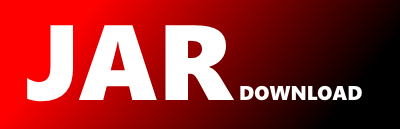
com.trimble.id.OnBehalfGrantTokenProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import static com.trimble.id.AuthenticationConstants.GRANT_TYPE;
import static com.trimble.id.AuthenticationConstants.JWT_TOKEN_TYPE;
import static com.trimble.id.AuthenticationConstants.SCOPE;
import static com.trimble.id.AuthenticationConstants.SDK_VARIANT;
import static com.trimble.id.AuthenticationConstants.SDK_VERSION;
import static com.trimble.id.AuthenticationConstants.SPACE;
import static com.trimble.id.AuthenticationConstants.SUBJECT_TOKEN;
import static com.trimble.id.AuthenticationConstants.SUBJECT_TOKEN_TYPE;
import static com.trimble.id.AuthenticationConstants.TOKEN_EXCHANGE;
import com.trimble.id.analytics.AnalyticsHttpClient;
/** A token provider based on the OAuth On Behalf grant type */
public class OnBehalfGrantTokenProvider implements ITokenProvider {
private IHttpTokenClient httpTokenClient;
private String clientId;
private String clientSecret;
private List scopes;
private String exchangeToken;
private String accessToken;
private Long tokenExpiry;
/**
* Public constructor of OnBehalfGrantTokenProvider
*
* @param endpointProvider The endpoint provider that will be used to get the
* OAuth endpoints
* @param consumerKey The consumer key of the application
* @param consumerSecret The consumer secret of the application
* @param exchangeToken The exchange token of the user
*/
public OnBehalfGrantTokenProvider(IEndpointProvider endpointProvider, String consumerKey, String consumerSecret,
String exchangeToken) {
this.httpTokenClient = new HttpTokenClient(endpointProvider);
this.clientId = consumerKey;
this.clientSecret = consumerSecret;
this.scopes = new ArrayList();
this.exchangeToken = exchangeToken;
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(),
SDK_VERSION, clientId);
}
/**
* Fluent extension to add scopes to the token provider
*
* @param scopes The scopes to be added
* @return The token provider with the scopes added
*/
public OnBehalfGrantTokenProvider withScopes(String[] scopes) {
this.scopes.addAll(Arrays.asList(scopes));
return this;
}
/**
* Get the access token
*
* @return A CompletableFuture that contains the access token
*/
@Override
public CompletableFuture getAccessToken() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getAccessToken", SDK_VARIANT, this.getClass().getPackage().getName(),
SDK_VERSION, clientId);
if (this.accessToken == null
|| (this.getTokenExpiry() != null && this.getTokenExpiry() < new Date().getTime())) {
List nameValuePairs = new ArrayList();
nameValuePairs.add(new NameValuePair(GRANT_TYPE, TOKEN_EXCHANGE));
try {
nameValuePairs.add(new NameValuePair(SCOPE,
URLEncoder.encode(String.join(SPACE, this.scopes), StandardCharsets.UTF_8.name())));
} catch (Exception e) {
throw new SDKClientException(e, e.getCause(), e.getMessage());
}
nameValuePairs.add(new NameValuePair(SUBJECT_TOKEN_TYPE, JWT_TOKEN_TYPE));
nameValuePairs.add(new NameValuePair(SUBJECT_TOKEN, this.exchangeToken));
return this.httpTokenClient
.getOauthTokens(new TokenRequest(this.clientId, this.clientSecret, nameValuePairs))
.thenApply((tokenResponse) -> {
if (tokenResponse.error_description != null) {
AnalyticsHttpClient.sendExceptionEvent("getAccessToken", SDK_VARIANT,
this.getClass().getPackage().getName(), SDK_VERSION,
tokenResponse.error_description, clientId);
throw new SDKClientException(tokenResponse.error, tokenResponse.error_description);
}
setAccessToken(tokenResponse.accessToken);
setTokenExpiry(Long.valueOf(new Date().getTime()) + (tokenResponse.expiresIn * 1000));
return this.accessToken;
});
}
return CompletableFuture.completedFuture(this.accessToken);
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
/**
* Get the token expiry
*
* @return The token expiry
*/
public Long getTokenExpiry() {
return this.tokenExpiry;
}
public void setTokenExpiry(Long tokenExpiry) {
this.tokenExpiry = tokenExpiry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy