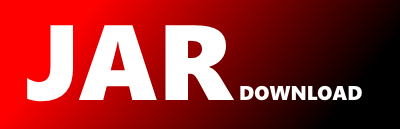
com.trimble.id.OpenIdEndpointProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import com.fasterxml.jackson.databind.ObjectMapper;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import okhttp3.ResponseBody;
import java.io.IOException;
import java.net.URI;
import java.util.concurrent.CompletableFuture;
import com.trimble.id.analytics.AnalyticsHttpClient;
import static com.trimble.id.AuthenticationConstants.SDK_VARIANT;
import static com.trimble.id.AuthenticationConstants.SDK_VERSION;
/** An endpoint provider that returns values from a OpenID well known configuration */
public class OpenIdEndpointProvider implements IEndpointProvider {
private URI openIdConfigurationEndpoint;
private URI authorizationEndpoint;
private URI tokenEndpoint;
private URI jwksEndpoint;
private URI revocationEndpoint;
private URI endSessionEndpoint;
/**
* Public constructor of OpenIdEndpointProvider
*
* @param openIdConfigurationEndpoint The OpenID well known configuration endpoint URI
*/
public OpenIdEndpointProvider(URI openIdConfigurationEndpoint) {
this.openIdConfigurationEndpoint = openIdConfigurationEndpoint;
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(), SDK_VERSION);
}
/**
* Get the authorization endpoint
*
* @return A CompletableFuture that contains the authorization endpoint
*/
public CompletableFuture getAuthorizationEndpoint() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getAuthorizationEndpoint", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION);
if (this.authorizationEndpoint != null)
return CompletableFuture.completedFuture(this.authorizationEndpoint);
return this.loadConfiguration().thenApply((success) -> this.authorizationEndpoint);
}
/**
* Get the token endpoint
*
* @return A CompletableFuture that contains the token endpoint
*/
public CompletableFuture getTokenEndpoint() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getTokenEndpoint", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION);
if (this.tokenEndpoint != null)
return CompletableFuture.completedFuture(this.tokenEndpoint);
return this.loadConfiguration().thenApply((success) -> this.tokenEndpoint);
}
/**
* Get the JSON Web Keyset endpoint
*
* @return A CompletableFuture that contains the JSON Web Keyset endpoint
*/
public CompletableFuture getJSONWebKeysetEndpoint() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getJSONWebKeysetEndpoint", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION);
if (this.jwksEndpoint != null)
return CompletableFuture.completedFuture(this.jwksEndpoint);
return this.loadConfiguration().thenApply((success) -> this.jwksEndpoint);
}
/**
* Get the revocation endpoint
*
* @return A CompletableFuture that contains the revocation endpoint
*/
public CompletableFuture getRevocationEndpoint() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getRevocationEndpoint", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION);
if (this.revocationEndpoint != null)
return CompletableFuture.completedFuture(this.revocationEndpoint);
return this.loadConfiguration().thenApply((success) -> this.revocationEndpoint);
}
/**
* Get the end session endpoint
*
* @return A CompletableFuture that contains the end session endpoint
*/
public CompletableFuture getEndSessionEndpoint() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("getEndSessionEndpoint", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION);
if (this.endSessionEndpoint != null)
return CompletableFuture.completedFuture(this.endSessionEndpoint);
return this.loadConfiguration().thenApply((success) -> this.endSessionEndpoint);
}
private CompletableFuture loadConfiguration() throws SDKClientException {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(getOpenIdConfigurationEndpoint().toString()).build();
ResponseBody responseBody = null;
try {
Response response = client.newCall(request).execute();
responseBody = response.body();
ObjectMapper mapper = new ObjectMapper();
OpenIdResponse configuration = mapper.readValue(responseBody.string(), OpenIdResponse.class);
setAuthorizationEndpoint(URI.create(configuration.authorizationEndpoint));
setTokenEndpoint(this.tokenEndpoint = URI.create(configuration.tokenEndpoint));
setJwksEndpoint(this.jwksEndpoint = URI.create((configuration.jwksUri)));
setRevocationEndpoint(this.revocationEndpoint = URI.create(configuration.revocationEndpoint));
setEndSessionEndpoint(this.endSessionEndpoint = URI.create(configuration.endSessionEndpoint));
} catch (IOException e) {
AnalyticsHttpClient.sendExceptionEvent("loadConfiguration", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION, e.getMessage());
throw new SDKClientException(e, e.getCause(), e.getMessage());
} finally {
if (responseBody != null)
responseBody.close();
}
return CompletableFuture.allOf();
}
/**
* Get the OpenID well known configuration endpoint URI
*
* @return The OpenID well known configuration endpoint URI
*/
public URI getOpenIdConfigurationEndpoint() {
return openIdConfigurationEndpoint;
}
public void setOpenIdConfigurationEndpoint(URI openIdConfigurationEndpoint) {
this.openIdConfigurationEndpoint = openIdConfigurationEndpoint;
}
public void setAuthorizationEndpoint(URI authorizationEndpoint) {
this.authorizationEndpoint = authorizationEndpoint;
}
public void setTokenEndpoint(URI tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
}
public void setJwksEndpoint(URI jwksEndpoint) {
this.jwksEndpoint = jwksEndpoint;
}
public void setRevocationEndpoint(URI revocationEndpoint) {
this.revocationEndpoint = revocationEndpoint;
}
public void setEndSessionEndpoint(URI endSessionEndpoint) {
this.endSessionEndpoint = endSessionEndpoint;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy