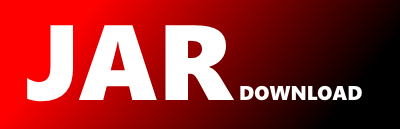
com.trimble.id.RefreshableTokenProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import static com.trimble.id.AuthenticationConstants.CLIENT_ID;
import static com.trimble.id.AuthenticationConstants.CODE_CHALLENGE_METHOD_PARAM;
import static com.trimble.id.AuthenticationConstants.CODE_CHALLENGE_PARAM;
import static com.trimble.id.AuthenticationConstants.CODE_VERIFIER;
import static com.trimble.id.AuthenticationConstants.GRANT_TYPE;
import static com.trimble.id.AuthenticationConstants.REFRESH_TOKEN;
import static com.trimble.id.AuthenticationConstants.S256;
import static com.trimble.id.AuthenticationConstants.SDK_VARIANT;
import static com.trimble.id.AuthenticationConstants.SDK_VERSION;
import static com.trimble.id.AuthenticationConstants.TOKEN;
import static com.trimble.id.AuthenticationConstants.TOKEN_TYPE_HINT;
import static com.trimble.id.CryptographicHelper.createCodeChallenge;
import static com.trimble.id.CryptographicHelper.generateCodeVerifier;
import com.trimble.id.analytics.AnalyticsHttpClient;
public class RefreshableTokenProvider implements ITokenProvider {
private HttpTokenClient tokenHttpClient;
private String clientId;
private String clientSecret;
private String accessToken;
private String idToken;
private Long tokenExpiry;
private String refreshToken;
private String codeVerifier;
private String codeVerifierForSerialRefresh;
public RefreshableTokenProvider(IEndpointProvider endpointProvider, String clientId, String clientSecret,
String accessToken, Long tokenExpiry, String refreshToken, String idToken, String codeVerifier) {
this.tokenHttpClient = new HttpTokenClient(endpointProvider);
this.clientId = clientId;
this.clientSecret = clientSecret;
this.accessToken = accessToken;
this.tokenExpiry = tokenExpiry;
this.refreshToken = refreshToken;
this.idToken = idToken;
this.codeVerifier = codeVerifier;
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(), SDK_VERSION, clientId);
}
@Override
public CompletableFuture getAccessToken() throws SDKClientException {
if (this.getTokenExpiry() != null && this.getTokenExpiry() < new Date().getTime()) {
if (this.codeVerifier != null) {
return refreshTokensUsingPKCE().thenApply((isSuccessful) -> {
return this.accessToken;
});
}
else {
return refreshTokens().thenApply((isSuccessful) -> {
return this.accessToken;
});
}
}
return CompletableFuture.completedFuture(this.accessToken);
}
@Override
public CompletableFuture getRefreshToken() throws SDKClientException {
return CompletableFuture.completedFuture(refreshToken);
}
@Override
public CompletableFuture getIdToken() throws SDKClientException {
if (this.getTokenExpiry() != null && this.getTokenExpiry() < new Date().getTime()) {
if (this.codeVerifier != null) {
return refreshTokensUsingPKCE().thenApply((isSuccessful) -> {
return this.idToken;
});
}
else {
return refreshTokens().thenApply((isSuccessful) -> {
return this.idToken;
});
}
}
return CompletableFuture.completedFuture(idToken);
}
@Override
public CompletableFuture revokeRefreshToken() throws SDKClientException {
List nameValuePairs = new ArrayList();
nameValuePairs.add(new NameValuePair(TOKEN, this.refreshToken));
nameValuePairs.add(new NameValuePair(TOKEN_TYPE_HINT, REFRESH_TOKEN));
return tokenHttpClient.revokeRefreshToken(new TokenRequest(this.clientId, this.clientSecret, nameValuePairs))
.thenApply((responseCode) -> {
return responseCode;
});
}
private CompletableFuture refreshTokens() throws SDKClientException {
List nameValuePairs = new ArrayList();
nameValuePairs.add(new NameValuePair(GRANT_TYPE, REFRESH_TOKEN));
nameValuePairs.add(new NameValuePair(REFRESH_TOKEN, this.refreshToken));
return tokenHttpClient.getOauthTokens(new TokenRequest(this.clientId, this.clientSecret, nameValuePairs))
.thenApply((tokenResponse) -> {
if (tokenResponse.error_description != null) {
AnalyticsHttpClient.sendExceptionEvent("refreshTokens", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION, tokenResponse.error_description, clientId);
throw new SDKClientException(tokenResponse.error, tokenResponse.error_description);
}
setAccessToken(tokenResponse.accessToken);
setTokenExpiry(Long.valueOf(new Date().getTime()) + (tokenResponse.expiresIn * 1000));
setRefreshToken(tokenResponse.refreshToken);
setIdToken(tokenResponse.idToken);
return true;
});
}
private CompletableFuture refreshTokensUsingPKCE() throws SDKClientException {
List nameValuePairs = new ArrayList();
nameValuePairs.add(new NameValuePair(CLIENT_ID, this.clientId));
nameValuePairs.add(new NameValuePair(GRANT_TYPE, REFRESH_TOKEN));
nameValuePairs.add(new NameValuePair(REFRESH_TOKEN, this.refreshToken));
nameValuePairs.add(new NameValuePair(CODE_VERIFIER, this.codeVerifier));
this.codeVerifierForSerialRefresh = generateCodeVerifier();
nameValuePairs
.add(new NameValuePair(CODE_CHALLENGE_PARAM, createCodeChallenge(this.codeVerifierForSerialRefresh)));
nameValuePairs.add(new NameValuePair(CODE_CHALLENGE_METHOD_PARAM, S256));
return tokenHttpClient.getOauthTokens(new TokenRequest(this.clientId, nameValuePairs))
.thenApply((tokenResponse) -> {
if (tokenResponse.error_description != null) {
AnalyticsHttpClient.sendExceptionEvent("refreshTokensUsingPKCE", SDK_VARIANT, this.getClass().getPackage().getName(), SDK_VERSION, tokenResponse.error_description, clientId);
throw new SDKClientException(tokenResponse.error, tokenResponse.error_description);
}
setAccessToken(tokenResponse.accessToken);
setTokenExpiry(Long.valueOf(new Date().getTime()) + (tokenResponse.expiresIn * 1000));
setRefreshToken(tokenResponse.refreshToken);
setIdToken(tokenResponse.idToken);
setCodeVerifier(this.codeVerifierForSerialRefresh);
return true;
});
}
public String getCodeVerifier() {
return codeVerifier;
}
public void setCodeVerifier(String codeVerifier) {
this.codeVerifier = codeVerifier;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public void setRefreshToken(String refreshToken) {
this.refreshToken = refreshToken;
}
public void setTokenExpiry(Long tokenExpiry) {
this.tokenExpiry = tokenExpiry;
}
public void setIdToken(String idToken) {
this.idToken = idToken;
}
public Long getTokenExpiry() {
return tokenExpiry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy