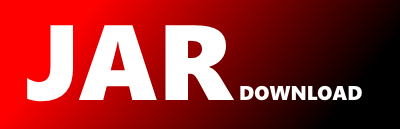
com.trimble.id.analytics.AnalyticsHttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id.analytics;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
/**
* A Http client for sending events to GA
*/
public class AnalyticsHttpClient {
private static OkHttpClient client = new OkHttpClient();
private static final String MEASUREMENT_ID = "G-9DHNE789XG";
private static final String API_SECRET = "7wd60wEeSnufb8FK6naHOQ";
private static final String CLIENT_ID = String.valueOf(UUID.randomUUID());
private static final String LANGUAGE = "language";
private static final String CLIENT_NAME = "client_name";
private static final String CLIENT_VERSION = "client_version";
private static final String EVENT_TYPE = "event_type";
private static final String APPLICATION_ID = "application_id";
private static final String EXCEPTION_MESSAGE = "exception_message";
/**
* Send initialization events
* For Java SDK
*
* @param name is the name of the event
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
* @param applicationID is the Client identifier of the application
*/
public static void sendInitEvent(String name, String clientName, String clientVersion, String applicationID) {
Map params = new HashMap<>();
putIfNotNull(params, LANGUAGE, "java_sdk");
putIfNotNull(params, CLIENT_NAME, clientName);
putIfNotNull(params, CLIENT_VERSION, clientVersion);
putIfNotNull(params, EVENT_TYPE, EventType.INIT.name());
putIfNotNull(params, APPLICATION_ID, applicationID);
sendEvent(name + "_init", params);
}
/**
* Send initialization events without application id
* For Java SDK
*
* @param name is the name of the event
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
*/
public static void sendInitEvent(String name, String clientName, String clientVersion) {
sendInitEvent(name, clientName, clientVersion, null);
}
/**
* Send method events
*
* @param name is the name of the event
* @param sdkType is the type of SDK(Android or Java) that uses analytics
* SDK
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
* @param applicationId is the Client identifier of the application
*/
public static void sendMethodEvent(String name, String sdkType, String clientName, String clientVersion,
String applicationId) {
Map params = new HashMap<>();
putIfNotNull(params, LANGUAGE, sdkType);
putIfNotNull(params, CLIENT_NAME, clientName);
putIfNotNull(params, CLIENT_VERSION, clientVersion);
putIfNotNull(params, APPLICATION_ID, applicationId);
putIfNotNull(params, EVENT_TYPE, EventType.METHOD_USAGE.name());
sendEvent(name + "_method", params);
}
/**
* Send method events without application id
*
* @param name is the name of the event
* @param sdkType is the type of SDK(Android or Java) that uses analytics
* SDK
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
*/
public static void sendMethodEvent(String name, String sdkType, String clientName, String clientVersion) {
sendMethodEvent(name, sdkType, clientName, clientVersion, null);
}
/**
* Send exception events
*
* @param name is the name of the event
* @param sdkType is the type of SDK(Android or Java) that uses
* analytics
* SDK
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
* @param exceptionMessage is the exception message
* @param applicationId is the Client identifier of the application
*/
public static void sendExceptionEvent(String name, String sdkType, String clientName, String clientVersion,
String exceptionMessage, String applicationId) {
Map params = new HashMap<>();
putIfNotNull(params, LANGUAGE, sdkType);
putIfNotNull(params, CLIENT_NAME, clientName);
putIfNotNull(params, CLIENT_VERSION, clientVersion);
putIfNotNull(params, EVENT_TYPE, EventType.EXCEPTION.name());
putIfNotNull(params, APPLICATION_ID, applicationId);
putIfNotNull(params, EXCEPTION_MESSAGE, exceptionMessage);
sendEvent(name + "_exception", params);
}
/**
* Send exception events without application id
*
* @param name is the name of the event
* @param sdkType is the type of SDK(Android or Java) that uses
* analytics
* SDK
* @param clientName is the name of the client SDK
* @param clientVersion is the version of the client SDK
* @param exceptionMessage is the exception message
*/
public static void sendExceptionEvent(String name, String sdkType, String clientName, String clientVersion,
String exceptionMessage) {
sendExceptionEvent(name, sdkType, clientName, clientVersion, exceptionMessage, null);
}
private static void sendEvent(String name, Map params) {
try {
Request request = createRequest(getTargetUrl(), name, params);
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call arg0, IOException e) {
}
@Override
public void onResponse(Call arg0, Response arg1) throws IOException {
}
});
} catch (Exception e) {
}
}
private static Request createRequest(String url, String name, Map params)
throws JsonProcessingException {
Map event = new HashMap<>();
event.put("name", name);
event.put("params", params);
// Create the events array
Map postDataMap = new HashMap<>();
postDataMap.put("client_id", CLIENT_ID);
postDataMap.put("events", Collections.singletonList(event));
// Convert map to JSON string
ObjectMapper objectMapper = new ObjectMapper();
String postData = objectMapper.writeValueAsString(postDataMap);
MediaType mediaType = MediaType.parse("application/json; charset=utf-8");
RequestBody body = RequestBody.create(postData, mediaType);
return new Request.Builder().url(url)
.addHeader("Content-Type", "application/json")
.addHeader("User-Agent", "TrimbleCloud.AnalyticsHttpClient/1.0.0")
.post(body).build();
}
private static String getTargetUrl() {
return "https://www.google-analytics.com/mp/collect?measurement_id=" + MEASUREMENT_ID + "&api_secret="
+ API_SECRET;
}
static void putIfNotNull(Map map, K key, V value) {
if (value != null) {
map.put(key, value);
}
}
}
enum EventType {
INIT,
METHOD_USAGE,
EXCEPTION
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy