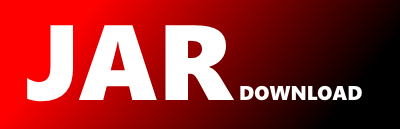
com.truelayer.java.merchantaccounts.IMerchantAccountsApi Maven / Gradle / Ivy
package com.truelayer.java.merchantaccounts;
import com.truelayer.java.entities.RequestScopes;
import com.truelayer.java.http.entities.ApiResponse;
import com.truelayer.java.merchantaccounts.entities.*;
import com.truelayer.java.merchantaccounts.entities.sweeping.SweepingSettings;
import com.truelayer.java.merchantaccounts.entities.transactions.TransactionTypeQuery;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import retrofit2.http.*;
import retrofit2.http.GET;
import retrofit2.http.Path;
import retrofit2.http.Query;
/**
* Exposes all the merchant accounts related capabilities of the library.
*
* @see Merchant Accounts API reference
*/
public interface IMerchantAccountsApi {
/**
* List all your TrueLayer's merchant accounts. There might be more than one account per currency.
* @param scopes the scopes to be used by the underlying Oauth token
* @return the list of all you TrueLayer's merchant accounts
* @see List Merchant Accounts API reference
*/
@GET("/merchant-accounts")
CompletableFuture> listMerchantAccounts(@Tag RequestScopes scopes);
/**
* Get the details of a single merchant account.
* @param scopes the scopes to be used by the underlying Oauth token
* @param merchantAccountId the id of the merchant account
* @return the details of the given merchant account
* @see Get Merchant Accounts API reference
*/
@GET("/merchant-accounts/{merchantAccountId}")
CompletableFuture> getMerchantAccountById(
@Tag RequestScopes scopes, @Path("merchantAccountId") String merchantAccountId);
/**
* Get the transactions of a single merchant account.
* @param scopes the scopes to be used by the underlying Oauth token
* @param headers map representing custom HTTP headers to be sent. In this case used for old clients to opt-in to paginated results
* @param merchantAccountId the id of the merchant account
* @param from Timestamp as a string for the start of the range you are querying. Mandatory
* @param to Timestamp as a string for the end of the range you are querying. Mandatory
* @param type Filter transactions by type. If omitted, both payments and payouts will be returned.
* @return the list of transactions matching the specified filters
* @see Get Transactions API reference
*/
@GET("/merchant-accounts/{merchantAccountId}/transactions")
CompletableFuture> listTransactions(
@Tag RequestScopes scopes,
@HeaderMap Map headers,
@Path("merchantAccountId") String merchantAccountId,
@Query("from") String from,
@Query("to") String to,
@Query("type") TransactionTypeQuery type,
@Query("cursor") String cursor);
/**
* Set the automatic sweeping settings for a merchant account. At regular intervals, any available balance in excess
* of the configured max_amount_in_minor
is withdrawn to a pre-configured IBAN.
* @param scopes the scopes to be used by the underlying Oauth token
* @param headers map representing custom HTTP headers to be sent
* @param merchantAccountId the id of the merchant account
* @param updateSweepingRequest the update/setup sweeping request
* @return the updated sweeping settings
* @see Setup/Update Sweeping API reference
*/
@POST("/merchant-accounts/{merchantAccountId}/sweeping")
CompletableFuture> updateSweeping(
@Tag RequestScopes scopes,
@HeaderMap Map headers,
@Path("merchantAccountId") String merchantAccountId,
@Body UpdateSweepingRequest updateSweepingRequest);
/**
* Get the automatic sweeping settings for a merchant account.
* @param scopes the scopes to be used by the underlying Oauth token
* @param merchantAccountId the id of the merchant account
* @return the sweeping settings for the given merchant account
* @see Get Sweeping Settings API reference
*/
@GET("/merchant-accounts/{merchantAccountId}/sweeping")
CompletableFuture> getSweepingSettings(
@Tag RequestScopes scopes, @Path("merchantAccountId") String merchantAccountId);
/**
* Disable automatic sweeping for a merchant account.
* @param scopes the scopes to be used by the underlying Oauth token
* @param headers map representing custom HTTP headers to be sent
* @param merchantAccountId the id of the merchant account
* @return an empty response in case of success
* @see Disable Sweeping API reference
*/
@DELETE("/merchant-accounts/{merchantAccountId}/sweeping")
CompletableFuture> disableSweeping(
@Tag RequestScopes scopes,
@HeaderMap Map headers,
@Path("merchantAccountId") String merchantAccountId);
/**
* Get the payment sources from which the merchant account has received payment
* @param scopes the scopes to be used by the underlying Oauth token
* @param merchantAccountId the id of the merchant account
* @param userId the id of the user
* @return The list of payment sources from which the merchant account has received payment
* @see Get payment sources API reference
*/
@GET("/merchant-accounts/{merchantAccountId}/payment-sources")
CompletableFuture> listPaymentSources(
@Tag RequestScopes scopes,
@Path("merchantAccountId") String merchantAccountId,
@Query("user_id") String userId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy