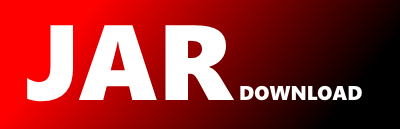
com.trustev.domain.entities.Customer Maven / Gradle / Ivy
package com.trustev.domain.entities;
import java.util.Collection;
import java.util.Date;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.annotate.JsonProperty;
import org.codehaus.jackson.map.annotate.JsonSerialize;
/**
* The Customer object includes the information on a Customer such as Address, Names, Email, Phone Number and Social Account information.
*/
@JsonSerialize(include=JsonSerialize.Inclusion.NON_NULL)
public class Customer extends BaseObject{
/**
* @return The First Name of the Customer.
*/
public String getFirstName() {
return firstName;
}
/**
* @param firstName The First Name of the Customer.
*/
@JsonProperty("FirstName")
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* @return The Last Name of the Customer.
*/
public String getLastName() {
return lastName;
}
/**
* @param lastName The Last Name of the Customer.
*/
@JsonProperty("LastName")
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return A collection of Emails. Please see Emails object for further parameter information.
*/
public Collection getEmail() {
return email;
}
/**
* @param email A collection of Emails. Please see Emails object for further parameter information.
*/
@JsonProperty("Emails")
public void setEmail(Collection email) {
this.email = email;
}
/**
* @return The Phone Number for the Customer.
*/
public String getPhoneNumber() {
return phoneNumber;
}
/**
* @param phoneNumber The Phone Number for the Customer.
*/
@JsonProperty("PhoneNumber")
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
/**
* @return The Date of Birth of the Customer.
*/
@JsonIgnore()
public Date getDateOfBirth() {
return dateOfBirth;
}
@JsonProperty("DateOfBirth")
String getDateOfBirthString() {
return FormatTimeStamp(dateOfBirth);
}
/**
* @param dateOfBirth The Date of Birth of the Customer.
*/
@JsonProperty("DateOfBirth")
public void setDateOfBirth(Date dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
/**
* @return Addresses Object Contains standard/delivery/billing information. Please see Address Object for further parameter information.
*/
public Collection getAddresses() {
return addresses;
}
/**
*
* @param addresses Addresses Object Contains standard/delivery/billing information. Please see Address Object for further parameter information.
*/
@JsonProperty("Addresses")
public void setAddresses(Collection addresses) {
this.addresses = addresses;
}
/**
*
* @return Social Account Object Contains Short Term and Long Term Access Tokens, along with Social Account Ids and Types. See Trustev Integration Documentation, http://developers.trustev.com/v2 for more information.
*/
public Collection getSocialAccounts() {
return socialAccounts;
}
/**
*
* @param socialAccounts Social Account Object Contains Short Term and Long Term Access Tokens, along with Social Account Ids and Types. See Trustev Integration Documentation, http://developers.trustev.com/v2 for more information.
*/
@JsonProperty("SocialAccounts")
public void setSocialAccounts(Collection socialAccounts) {
this.socialAccounts = socialAccounts;
}
/**
*
* @return The Account number of the Customer.
*/
public String getAccountNumber() {
return accountNumber;
}
/**
*
* @param accountNumber The Account number of the Customer.
*/
@JsonProperty("AccountNumber")
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
/**
*
* @return The Account number of the Customer.
*/
public String getSocialSecurityNumber() {
return this.socialSecurityNumber;
}
/**
*
* @param socialSecurityNumber The Social Security Number of the customer
*/
@JsonProperty("SocialSecurityNumber")
public void setSocialSecurityNumber(String socialSecurityNumber) {
this.socialSecurityNumber = socialSecurityNumber;
}
private String firstName;
private String lastName;
private Collection email;
private String phoneNumber;
private Date dateOfBirth;
private Collection addresses;
private Collection socialAccounts;
private String accountNumber;
private String socialSecurityNumber;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy