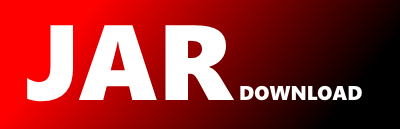
com.trustev.domain.entities.Transaction Maven / Gradle / Ivy
package com.trustev.domain.entities;
import java.math.BigDecimal;
import java.util.Collection;
import java.util.Date;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.annotate.JsonProperty;
import org.codehaus.jackson.map.annotate.JsonSerialize;
/**
* Transaction Object - includes details such as Transaction Amount, Currency, Items and Transaction delivery/billing address.
*/
@JsonSerialize(include=JsonSerialize.Inclusion.NON_NULL)
public class Transaction extends BaseObject {
/**
* @return Total Value of the Transaction.
*/
public double getTotalTransactionValue() {
return totalTransactionValue;
}
/**
* @param totalTransactionValue Total Value of the Transaction.
*/
@JsonProperty("TotalTransactionValue")
public void setTotalTransactionValue(double totalTransactionValue) {
this.totalTransactionValue = totalTransactionValue;
}
/**
* @return Currency Type Code. Standard Currency Type codes can be found at http://www.xe.com/currency
*/
public String getCurrency() {
return currency;
}
/**
* @param currency Currency Type Code. Standard Currency Type codes can be found at http://www.xe.com/currency
*/
@JsonProperty("Currency")
public void setCurrency(String currency) {
this.currency = currency;
}
/**
* @return Current Timestamp.
*/
@JsonIgnore()
public Date getTimestamp() {
return timestamp;
}
@JsonProperty("Timestamp")
String getTimestampString() {
return FormatTimeStamp(timestamp);
}
/**
* @param timestamp Current Timestamp.
*/
@JsonProperty("Timestamp")
public void setTimestamp(Date timestamp) {
this.timestamp = timestamp;
}
/**
* @return Addresses Object Contains standard/delivery/billing information. Please see Address Object for further parameter information.
*/
public Collection getAddresses() {
return addresses;
}
/**
* @param addresses Addresses Object Contains standard/delivery/billing information. Please see Address Object for further parameter information.
*/
@JsonProperty("Addresses")
public void setAddresses(Collection addresses) {
this.addresses = addresses;
}
/**
* @return Items Object contains details on Item Name, Quantity and Item Value. Please see Items Object for further parameter information.
*/
public Collection getItems() {
return items;
}
/**
* @param items Items Object contains details on Item Name, Quantity and Item Value. Please see Items Object for further parameter information.
*/
@JsonProperty("Items")
public void setItems(Collection items) {
this.items = items;
}
/**
* @return Email Object
*/
public Collection getEmails() {
return this.emails;
}
/**
* @param emails Email Object
*/
@JsonProperty("Emails")
public void setEmails(Collection emails) {
this.emails = emails;
}
private double totalTransactionValue;
private String currency;
private Date timestamp;
private Collection addresses;
private Collection items;
public Collection emails;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy