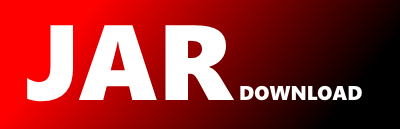
com.truward.orion.eolaire.model.EolaireModel Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: eolaire.proto
package com.truward.orion.eolaire.model;
public final class EolaireModel {
private EolaireModel() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code truward.orion.user.VariantType}
*/
public enum VariantType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NULL = 0;
*/
NULL(0, 0),
/**
* BOOL_FALSE = 1;
*/
BOOL_FALSE(1, 1),
/**
* BOOL_TRUE = 2;
*/
BOOL_TRUE(2, 2),
/**
* INT32_ZERO = 3;
*/
INT32_ZERO(3, 3),
/**
* INT64_ZERO = 4;
*/
INT64_ZERO(4, 4),
/**
* FLOAT_ZERO = 5;
*/
FLOAT_ZERO(5, 5),
/**
* DOUBLE_ZERO = 6;
*/
DOUBLE_ZERO(6, 6),
/**
* INT32 = 7;
*/
INT32(7, 7),
/**
* INT64 = 8;
*/
INT64(8, 8),
/**
* FLOAT = 9;
*/
FLOAT(9, 9),
/**
* DOUBLE = 10;
*/
DOUBLE(10, 10),
/**
* STRING = 11;
*/
STRING(11, 11),
/**
* BYTES = 12;
*/
BYTES(12, 12),
UNRECOGNIZED(-1, -1),
;
/**
* NULL = 0;
*/
public static final int NULL_VALUE = 0;
/**
* BOOL_FALSE = 1;
*/
public static final int BOOL_FALSE_VALUE = 1;
/**
* BOOL_TRUE = 2;
*/
public static final int BOOL_TRUE_VALUE = 2;
/**
* INT32_ZERO = 3;
*/
public static final int INT32_ZERO_VALUE = 3;
/**
* INT64_ZERO = 4;
*/
public static final int INT64_ZERO_VALUE = 4;
/**
* FLOAT_ZERO = 5;
*/
public static final int FLOAT_ZERO_VALUE = 5;
/**
* DOUBLE_ZERO = 6;
*/
public static final int DOUBLE_ZERO_VALUE = 6;
/**
* INT32 = 7;
*/
public static final int INT32_VALUE = 7;
/**
* INT64 = 8;
*/
public static final int INT64_VALUE = 8;
/**
* FLOAT = 9;
*/
public static final int FLOAT_VALUE = 9;
/**
* DOUBLE = 10;
*/
public static final int DOUBLE_VALUE = 10;
/**
* STRING = 11;
*/
public static final int STRING_VALUE = 11;
/**
* BYTES = 12;
*/
public static final int BYTES_VALUE = 12;
public final int getNumber() {
if (index == -1) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
public static VariantType valueOf(int value) {
switch (value) {
case 0: return NULL;
case 1: return BOOL_FALSE;
case 2: return BOOL_TRUE;
case 3: return INT32_ZERO;
case 4: return INT64_ZERO;
case 5: return FLOAT_ZERO;
case 6: return DOUBLE_ZERO;
case 7: return INT32;
case 8: return INT64;
case 9: return FLOAT;
case 10: return DOUBLE;
case 11: return STRING;
case 12: return BYTES;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
VariantType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public VariantType findValueByNumber(int number) {
return VariantType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.getDescriptor().getEnumTypes().get(0);
}
private static final VariantType[] VALUES = values();
public static VariantType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private VariantType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:truward.orion.user.VariantType)
}
public interface EntityTypeOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.EntityType)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 id = 1;
*/
long getId();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code truward.orion.user.EntityType}
*/
public static final class EntityType extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.EntityType)
EntityTypeOrBuilder {
// Use EntityType.newBuilder() to construct.
private EntityType(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private EntityType() {
id_ = 0L;
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private EntityType(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
id_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_EntityType_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_EntityType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.EntityType.class, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* optional int64 id = 1;
*/
public long getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0L) {
output.writeInt64(1, id_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.EntityType prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.EntityType}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.EntityType)
com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_EntityType_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_EntityType_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.EntityType.class, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.EntityType.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = 0L;
name_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_EntityType_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.EntityType getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.EntityType build() {
com.truward.orion.eolaire.model.EolaireModel.EntityType result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.EntityType buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.EntityType result = new com.truward.orion.eolaire.model.EolaireModel.EntityType(this);
result.id_ = id_;
result.name_ = name_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.EntityType) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.EntityType)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.EntityType other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance()) return this;
if (other.getId() != 0L) {
setId(other.getId());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.EntityType parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.EntityType) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long id_ ;
/**
* optional int64 id = 1;
*/
public long getId() {
return id_;
}
/**
* optional int64 id = 1;
*/
public Builder setId(long value) {
id_ = value;
onChanged();
return this;
}
/**
* optional int64 id = 1;
*/
public Builder clearId() {
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.EntityType)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.EntityType)
private static final com.truward.orion.eolaire.model.EolaireModel.EntityType DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.EntityType();
}
public static com.truward.orion.eolaire.model.EolaireModel.EntityType getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public EntityType parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new EntityType(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.EntityType getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.Item)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 id = 1;
*/
long getId();
/**
* optional string name = 2;
*/
java.lang.String getName();
/**
* optional string name = 2;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional int64 itemTypeId = 3;
*/
long getItemTypeId();
}
/**
* Protobuf type {@code truward.orion.user.Item}
*/
public static final class Item extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.Item)
ItemOrBuilder {
// Use Item.newBuilder() to construct.
private Item(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Item() {
id_ = 0L;
name_ = "";
itemTypeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Item(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
id_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 24: {
itemTypeId_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Item_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.Item.class, com.truward.orion.eolaire.model.EolaireModel.Item.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* optional int64 id = 1;
*/
public long getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEMTYPEID_FIELD_NUMBER = 3;
private long itemTypeId_;
/**
* optional int64 itemTypeId = 3;
*/
public long getItemTypeId() {
return itemTypeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != 0L) {
output.writeInt64(1, id_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, name_);
}
if (itemTypeId_ != 0L) {
output.writeInt64(3, itemTypeId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, name_);
}
if (itemTypeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, itemTypeId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Item parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.Item prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.Item}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.Item)
com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Item_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.Item.class, com.truward.orion.eolaire.model.EolaireModel.Item.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.Item.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = 0L;
name_ = "";
itemTypeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Item_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.Item getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.Item build() {
com.truward.orion.eolaire.model.EolaireModel.Item result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.Item buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.Item result = new com.truward.orion.eolaire.model.EolaireModel.Item(this);
result.id_ = id_;
result.name_ = name_;
result.itemTypeId_ = itemTypeId_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.Item) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.Item)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.Item other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance()) return this;
if (other.getId() != 0L) {
setId(other.getId());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.getItemTypeId() != 0L) {
setItemTypeId(other.getItemTypeId());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.Item parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.Item) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long id_ ;
/**
* optional int64 id = 1;
*/
public long getId() {
return id_;
}
/**
* optional int64 id = 1;
*/
public Builder setId(long value) {
id_ = value;
onChanged();
return this;
}
/**
* optional int64 id = 1;
*/
public Builder clearId() {
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* optional string name = 2;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 2;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 2;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 2;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private long itemTypeId_ ;
/**
* optional int64 itemTypeId = 3;
*/
public long getItemTypeId() {
return itemTypeId_;
}
/**
* optional int64 itemTypeId = 3;
*/
public Builder setItemTypeId(long value) {
itemTypeId_ = value;
onChanged();
return this;
}
/**
* optional int64 itemTypeId = 3;
*/
public Builder clearItemTypeId() {
itemTypeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.Item)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.Item)
private static final com.truward.orion.eolaire.model.EolaireModel.Item DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.Item();
}
public static com.truward.orion.eolaire.model.EolaireModel.Item getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser-
PARSER = new com.google.protobuf.AbstractParser
- () {
public Item parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Item(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser
- parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser
- getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.Item getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ItemRelationOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.ItemRelation)
com.google.protobuf.MessageOrBuilder {
/**
*
optional int64 targetItemId = 1;
*/
long getTargetItemId();
/**
* optional int64 relationTypeId = 2;
*/
long getRelationTypeId();
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
boolean hasMetadata();
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata();
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.ItemRelation}
*/
public static final class ItemRelation extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.ItemRelation)
ItemRelationOrBuilder {
// Use ItemRelation.newBuilder() to construct.
private ItemRelation(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ItemRelation() {
targetItemId_ = 0L;
relationTypeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ItemRelation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
targetItemId_ = input.readInt64();
break;
}
case 16: {
relationTypeId_ = input.readInt64();
break;
}
case 26: {
com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder subBuilder = null;
if (metadata_ != null) {
subBuilder = metadata_.toBuilder();
}
metadata_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.Metadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(metadata_);
metadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemRelation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemRelation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.class, com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder.class);
}
public static final int TARGETITEMID_FIELD_NUMBER = 1;
private long targetItemId_;
/**
* optional int64 targetItemId = 1;
*/
public long getTargetItemId() {
return targetItemId_;
}
public static final int RELATIONTYPEID_FIELD_NUMBER = 2;
private long relationTypeId_;
/**
* optional int64 relationTypeId = 2;
*/
public long getRelationTypeId() {
return relationTypeId_;
}
public static final int METADATA_FIELD_NUMBER = 3;
private com.truward.orion.eolaire.model.EolaireModel.Metadata metadata_;
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public boolean hasMetadata() {
return metadata_ != null;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata() {
return metadata_ == null ? com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder() {
return getMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (targetItemId_ != 0L) {
output.writeInt64(1, targetItemId_);
}
if (relationTypeId_ != 0L) {
output.writeInt64(2, relationTypeId_);
}
if (metadata_ != null) {
output.writeMessage(3, getMetadata());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (targetItemId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, targetItemId_);
}
if (relationTypeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, relationTypeId_);
}
if (metadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMetadata());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.ItemRelation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.ItemRelation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.ItemRelation)
com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemRelation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemRelation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.class, com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.ItemRelation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
targetItemId_ = 0L;
relationTypeId_ = 0L;
if (metadataBuilder_ == null) {
metadata_ = null;
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemRelation_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.ItemRelation.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation build() {
com.truward.orion.eolaire.model.EolaireModel.ItemRelation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.ItemRelation result = new com.truward.orion.eolaire.model.EolaireModel.ItemRelation(this);
result.targetItemId_ = targetItemId_;
result.relationTypeId_ = relationTypeId_;
if (metadataBuilder_ == null) {
result.metadata_ = metadata_;
} else {
result.metadata_ = metadataBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.ItemRelation) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.ItemRelation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.ItemRelation other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.ItemRelation.getDefaultInstance()) return this;
if (other.getTargetItemId() != 0L) {
setTargetItemId(other.getTargetItemId());
}
if (other.getRelationTypeId() != 0L) {
setRelationTypeId(other.getRelationTypeId());
}
if (other.hasMetadata()) {
mergeMetadata(other.getMetadata());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.ItemRelation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.ItemRelation) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long targetItemId_ ;
/**
* optional int64 targetItemId = 1;
*/
public long getTargetItemId() {
return targetItemId_;
}
/**
* optional int64 targetItemId = 1;
*/
public Builder setTargetItemId(long value) {
targetItemId_ = value;
onChanged();
return this;
}
/**
* optional int64 targetItemId = 1;
*/
public Builder clearTargetItemId() {
targetItemId_ = 0L;
onChanged();
return this;
}
private long relationTypeId_ ;
/**
* optional int64 relationTypeId = 2;
*/
public long getRelationTypeId() {
return relationTypeId_;
}
/**
* optional int64 relationTypeId = 2;
*/
public Builder setRelationTypeId(long value) {
relationTypeId_ = value;
onChanged();
return this;
}
/**
* optional int64 relationTypeId = 2;
*/
public Builder clearRelationTypeId() {
relationTypeId_ = 0L;
onChanged();
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.Metadata metadata_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder> metadataBuilder_;
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public boolean hasMetadata() {
return metadataBuilder_ != null || metadata_ != null;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata() {
if (metadataBuilder_ == null) {
return metadata_ == null ? com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
} else {
return metadataBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public Builder setMetadata(com.truward.orion.eolaire.model.EolaireModel.Metadata value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metadata_ = value;
onChanged();
} else {
metadataBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public Builder setMetadata(
com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder builderForValue) {
if (metadataBuilder_ == null) {
metadata_ = builderForValue.build();
onChanged();
} else {
metadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public Builder mergeMetadata(com.truward.orion.eolaire.model.EolaireModel.Metadata value) {
if (metadataBuilder_ == null) {
if (metadata_ != null) {
metadata_ =
com.truward.orion.eolaire.model.EolaireModel.Metadata.newBuilder(metadata_).mergeFrom(value).buildPartial();
} else {
metadata_ = value;
}
onChanged();
} else {
metadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public Builder clearMetadata() {
if (metadataBuilder_ == null) {
metadata_ = null;
onChanged();
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder getMetadataBuilder() {
onChanged();
return getMetadataFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder() {
if (metadataBuilder_ != null) {
return metadataBuilder_.getMessageOrBuilder();
} else {
return metadata_ == null ?
com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
}
}
/**
* optional .truward.orion.user.Metadata metadata = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder>
getMetadataFieldBuilder() {
if (metadataBuilder_ == null) {
metadataBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder>(
getMetadata(),
getParentForChildren(),
isClean());
metadata_ = null;
}
return metadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.ItemRelation)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.ItemRelation)
private static final com.truward.orion.eolaire.model.EolaireModel.ItemRelation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.ItemRelation();
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemRelation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ItemRelation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ItemRelation(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ItemGraphOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.ItemGraph)
com.google.protobuf.MessageOrBuilder {
/**
* optional .truward.orion.user.Item item = 1;
*/
boolean hasItem();
/**
* optional .truward.orion.user.Item item = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.Item getItem();
/**
* optional .truward.orion.user.Item item = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemOrBuilder();
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
boolean hasRelations();
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemRelation getRelations();
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder getRelationsOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.ItemGraph}
*/
public static final class ItemGraph extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.ItemGraph)
ItemGraphOrBuilder {
// Use ItemGraph.newBuilder() to construct.
private ItemGraph(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ItemGraph() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ItemGraph(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.truward.orion.eolaire.model.EolaireModel.Item.Builder subBuilder = null;
if (item_ != null) {
subBuilder = item_.toBuilder();
}
item_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.Item.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(item_);
item_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder subBuilder = null;
if (relations_ != null) {
subBuilder = relations_.toBuilder();
}
relations_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.ItemRelation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(relations_);
relations_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemGraph_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemGraph_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemGraph.class, com.truward.orion.eolaire.model.EolaireModel.ItemGraph.Builder.class);
}
public static final int ITEM_FIELD_NUMBER = 1;
private com.truward.orion.eolaire.model.EolaireModel.Item item_;
/**
* optional .truward.orion.user.Item item = 1;
*/
public boolean hasItem() {
return item_ != null;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item getItem() {
return item_ == null ? com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance() : item_;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemOrBuilder() {
return getItem();
}
public static final int RELATIONS_FIELD_NUMBER = 2;
private com.truward.orion.eolaire.model.EolaireModel.ItemRelation relations_;
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public boolean hasRelations() {
return relations_ != null;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation getRelations() {
return relations_ == null ? com.truward.orion.eolaire.model.EolaireModel.ItemRelation.getDefaultInstance() : relations_;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder getRelationsOrBuilder() {
return getRelations();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (item_ != null) {
output.writeMessage(1, getItem());
}
if (relations_ != null) {
output.writeMessage(2, getRelations());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (item_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getItem());
}
if (relations_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRelations());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.ItemGraph prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.ItemGraph}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.ItemGraph)
com.truward.orion.eolaire.model.EolaireModel.ItemGraphOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemGraph_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemGraph_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemGraph.class, com.truward.orion.eolaire.model.EolaireModel.ItemGraph.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.ItemGraph.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (itemBuilder_ == null) {
item_ = null;
} else {
item_ = null;
itemBuilder_ = null;
}
if (relationsBuilder_ == null) {
relations_ = null;
} else {
relations_ = null;
relationsBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemGraph_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemGraph getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.ItemGraph.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.ItemGraph build() {
com.truward.orion.eolaire.model.EolaireModel.ItemGraph result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemGraph buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.ItemGraph result = new com.truward.orion.eolaire.model.EolaireModel.ItemGraph(this);
if (itemBuilder_ == null) {
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
if (relationsBuilder_ == null) {
result.relations_ = relations_;
} else {
result.relations_ = relationsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.ItemGraph) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.ItemGraph)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.ItemGraph other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.ItemGraph.getDefaultInstance()) return this;
if (other.hasItem()) {
mergeItem(other.getItem());
}
if (other.hasRelations()) {
mergeRelations(other.getRelations());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.ItemGraph parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.ItemGraph) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.Item item_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder> itemBuilder_;
/**
* optional .truward.orion.user.Item item = 1;
*/
public boolean hasItem() {
return itemBuilder_ != null || item_ != null;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item getItem() {
if (itemBuilder_ == null) {
return item_ == null ? com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance() : item_;
} else {
return itemBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public Builder setItem(com.truward.orion.eolaire.model.EolaireModel.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
item_ = value;
onChanged();
} else {
itemBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public Builder setItem(
com.truward.orion.eolaire.model.EolaireModel.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
item_ = builderForValue.build();
onChanged();
} else {
itemBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public Builder mergeItem(com.truward.orion.eolaire.model.EolaireModel.Item value) {
if (itemBuilder_ == null) {
if (item_ != null) {
item_ =
com.truward.orion.eolaire.model.EolaireModel.Item.newBuilder(item_).mergeFrom(value).buildPartial();
} else {
item_ = value;
}
onChanged();
} else {
itemBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = null;
onChanged();
} else {
item_ = null;
itemBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item.Builder getItemBuilder() {
onChanged();
return getItemFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.Item item = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemOrBuilder() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilder();
} else {
return item_ == null ?
com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance() : item_;
}
}
/**
* optional .truward.orion.user.Item item = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>(
getItem(),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private com.truward.orion.eolaire.model.EolaireModel.ItemRelation relations_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemRelation, com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder> relationsBuilder_;
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public boolean hasRelations() {
return relationsBuilder_ != null || relations_ != null;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation getRelations() {
if (relationsBuilder_ == null) {
return relations_ == null ? com.truward.orion.eolaire.model.EolaireModel.ItemRelation.getDefaultInstance() : relations_;
} else {
return relationsBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public Builder setRelations(com.truward.orion.eolaire.model.EolaireModel.ItemRelation value) {
if (relationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
relations_ = value;
onChanged();
} else {
relationsBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public Builder setRelations(
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder builderForValue) {
if (relationsBuilder_ == null) {
relations_ = builderForValue.build();
onChanged();
} else {
relationsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public Builder mergeRelations(com.truward.orion.eolaire.model.EolaireModel.ItemRelation value) {
if (relationsBuilder_ == null) {
if (relations_ != null) {
relations_ =
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.newBuilder(relations_).mergeFrom(value).buildPartial();
} else {
relations_ = value;
}
onChanged();
} else {
relationsBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public Builder clearRelations() {
if (relationsBuilder_ == null) {
relations_ = null;
onChanged();
} else {
relations_ = null;
relationsBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder getRelationsBuilder() {
onChanged();
return getRelationsFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder getRelationsOrBuilder() {
if (relationsBuilder_ != null) {
return relationsBuilder_.getMessageOrBuilder();
} else {
return relations_ == null ?
com.truward.orion.eolaire.model.EolaireModel.ItemRelation.getDefaultInstance() : relations_;
}
}
/**
* optional .truward.orion.user.ItemRelation relations = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemRelation, com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder>
getRelationsFieldBuilder() {
if (relationsBuilder_ == null) {
relationsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemRelation, com.truward.orion.eolaire.model.EolaireModel.ItemRelation.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemRelationOrBuilder>(
getRelations(),
getParentForChildren(),
isClean());
relations_ = null;
}
return relationsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.ItemGraph)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.ItemGraph)
private static final com.truward.orion.eolaire.model.EolaireModel.ItemGraph DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.ItemGraph();
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemGraph getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ItemGraph parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ItemGraph(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemGraph getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VariantValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.VariantValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 intValue = 1;
*/
int getIntValue();
/**
* optional int64 longValue = 2;
*/
long getLongValue();
/**
* optional float floatValue = 3;
*/
float getFloatValue();
/**
* optional double doubleValue = 4;
*/
double getDoubleValue();
/**
* optional string strValue = 5;
*/
java.lang.String getStrValue();
/**
* optional string strValue = 5;
*/
com.google.protobuf.ByteString
getStrValueBytes();
/**
* optional bytes bytesValue = 7;
*/
com.google.protobuf.ByteString getBytesValue();
}
/**
* Protobuf type {@code truward.orion.user.VariantValue}
*/
public static final class VariantValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.VariantValue)
VariantValueOrBuilder {
// Use VariantValue.newBuilder() to construct.
private VariantValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private VariantValue() {
intValue_ = 0;
longValue_ = 0L;
floatValue_ = 0F;
doubleValue_ = 0D;
strValue_ = "";
bytesValue_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private VariantValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
intValue_ = input.readInt32();
break;
}
case 16: {
longValue_ = input.readInt64();
break;
}
case 29: {
floatValue_ = input.readFloat();
break;
}
case 33: {
doubleValue_ = input.readDouble();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
strValue_ = s;
break;
}
case 58: {
bytesValue_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_VariantValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_VariantValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.VariantValue.class, com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder.class);
}
public static final int INTVALUE_FIELD_NUMBER = 1;
private int intValue_;
/**
* optional int32 intValue = 1;
*/
public int getIntValue() {
return intValue_;
}
public static final int LONGVALUE_FIELD_NUMBER = 2;
private long longValue_;
/**
* optional int64 longValue = 2;
*/
public long getLongValue() {
return longValue_;
}
public static final int FLOATVALUE_FIELD_NUMBER = 3;
private float floatValue_;
/**
* optional float floatValue = 3;
*/
public float getFloatValue() {
return floatValue_;
}
public static final int DOUBLEVALUE_FIELD_NUMBER = 4;
private double doubleValue_;
/**
* optional double doubleValue = 4;
*/
public double getDoubleValue() {
return doubleValue_;
}
public static final int STRVALUE_FIELD_NUMBER = 5;
private volatile java.lang.Object strValue_;
/**
* optional string strValue = 5;
*/
public java.lang.String getStrValue() {
java.lang.Object ref = strValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
strValue_ = s;
return s;
}
}
/**
* optional string strValue = 5;
*/
public com.google.protobuf.ByteString
getStrValueBytes() {
java.lang.Object ref = strValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
strValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BYTESVALUE_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString bytesValue_;
/**
* optional bytes bytesValue = 7;
*/
public com.google.protobuf.ByteString getBytesValue() {
return bytesValue_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (intValue_ != 0) {
output.writeInt32(1, intValue_);
}
if (longValue_ != 0L) {
output.writeInt64(2, longValue_);
}
if (floatValue_ != 0F) {
output.writeFloat(3, floatValue_);
}
if (doubleValue_ != 0D) {
output.writeDouble(4, doubleValue_);
}
if (!getStrValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, strValue_);
}
if (!bytesValue_.isEmpty()) {
output.writeBytes(7, bytesValue_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (intValue_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, intValue_);
}
if (longValue_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, longValue_);
}
if (floatValue_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(3, floatValue_);
}
if (doubleValue_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, doubleValue_);
}
if (!getStrValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, strValue_);
}
if (!bytesValue_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, bytesValue_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.VariantValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.VariantValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.VariantValue)
com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_VariantValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_VariantValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.VariantValue.class, com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.VariantValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
intValue_ = 0;
longValue_ = 0L;
floatValue_ = 0F;
doubleValue_ = 0D;
strValue_ = "";
bytesValue_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_VariantValue_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.VariantValue getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.VariantValue.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.VariantValue build() {
com.truward.orion.eolaire.model.EolaireModel.VariantValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.VariantValue buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.VariantValue result = new com.truward.orion.eolaire.model.EolaireModel.VariantValue(this);
result.intValue_ = intValue_;
result.longValue_ = longValue_;
result.floatValue_ = floatValue_;
result.doubleValue_ = doubleValue_;
result.strValue_ = strValue_;
result.bytesValue_ = bytesValue_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.VariantValue) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.VariantValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.VariantValue other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.VariantValue.getDefaultInstance()) return this;
if (other.getIntValue() != 0) {
setIntValue(other.getIntValue());
}
if (other.getLongValue() != 0L) {
setLongValue(other.getLongValue());
}
if (other.getFloatValue() != 0F) {
setFloatValue(other.getFloatValue());
}
if (other.getDoubleValue() != 0D) {
setDoubleValue(other.getDoubleValue());
}
if (!other.getStrValue().isEmpty()) {
strValue_ = other.strValue_;
onChanged();
}
if (other.getBytesValue() != com.google.protobuf.ByteString.EMPTY) {
setBytesValue(other.getBytesValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.VariantValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.VariantValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int intValue_ ;
/**
* optional int32 intValue = 1;
*/
public int getIntValue() {
return intValue_;
}
/**
* optional int32 intValue = 1;
*/
public Builder setIntValue(int value) {
intValue_ = value;
onChanged();
return this;
}
/**
* optional int32 intValue = 1;
*/
public Builder clearIntValue() {
intValue_ = 0;
onChanged();
return this;
}
private long longValue_ ;
/**
* optional int64 longValue = 2;
*/
public long getLongValue() {
return longValue_;
}
/**
* optional int64 longValue = 2;
*/
public Builder setLongValue(long value) {
longValue_ = value;
onChanged();
return this;
}
/**
* optional int64 longValue = 2;
*/
public Builder clearLongValue() {
longValue_ = 0L;
onChanged();
return this;
}
private float floatValue_ ;
/**
* optional float floatValue = 3;
*/
public float getFloatValue() {
return floatValue_;
}
/**
* optional float floatValue = 3;
*/
public Builder setFloatValue(float value) {
floatValue_ = value;
onChanged();
return this;
}
/**
* optional float floatValue = 3;
*/
public Builder clearFloatValue() {
floatValue_ = 0F;
onChanged();
return this;
}
private double doubleValue_ ;
/**
* optional double doubleValue = 4;
*/
public double getDoubleValue() {
return doubleValue_;
}
/**
* optional double doubleValue = 4;
*/
public Builder setDoubleValue(double value) {
doubleValue_ = value;
onChanged();
return this;
}
/**
* optional double doubleValue = 4;
*/
public Builder clearDoubleValue() {
doubleValue_ = 0D;
onChanged();
return this;
}
private java.lang.Object strValue_ = "";
/**
* optional string strValue = 5;
*/
public java.lang.String getStrValue() {
java.lang.Object ref = strValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
strValue_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string strValue = 5;
*/
public com.google.protobuf.ByteString
getStrValueBytes() {
java.lang.Object ref = strValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
strValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string strValue = 5;
*/
public Builder setStrValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
strValue_ = value;
onChanged();
return this;
}
/**
* optional string strValue = 5;
*/
public Builder clearStrValue() {
strValue_ = getDefaultInstance().getStrValue();
onChanged();
return this;
}
/**
* optional string strValue = 5;
*/
public Builder setStrValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
strValue_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString bytesValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes bytesValue = 7;
*/
public com.google.protobuf.ByteString getBytesValue() {
return bytesValue_;
}
/**
* optional bytes bytesValue = 7;
*/
public Builder setBytesValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bytesValue_ = value;
onChanged();
return this;
}
/**
* optional bytes bytesValue = 7;
*/
public Builder clearBytesValue() {
bytesValue_ = getDefaultInstance().getBytesValue();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.VariantValue)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.VariantValue)
private static final com.truward.orion.eolaire.model.EolaireModel.VariantValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.VariantValue();
}
public static com.truward.orion.eolaire.model.EolaireModel.VariantValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public VariantValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new VariantValue(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.VariantValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MetadataEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.MetadataEntry)
com.google.protobuf.MessageOrBuilder {
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* optional .truward.orion.user.VariantType type = 2;
*/
int getTypeValue();
/**
* optional .truward.orion.user.VariantType type = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.VariantType getType();
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
boolean hasValue();
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
com.truward.orion.eolaire.model.EolaireModel.VariantValue getValue();
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder getValueOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.MetadataEntry}
*/
public static final class MetadataEntry extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.MetadataEntry)
MetadataEntryOrBuilder {
// Use MetadataEntry.newBuilder() to construct.
private MetadataEntry(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private MetadataEntry() {
key_ = "";
type_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private MetadataEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
key_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 26: {
com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder subBuilder = null;
if (value_ != null) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.VariantValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_MetadataEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_MetadataEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.class, com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder.class);
}
public static final int KEY_FIELD_NUMBER = 1;
private volatile java.lang.Object key_;
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantType getType() {
com.truward.orion.eolaire.model.EolaireModel.VariantType result = com.truward.orion.eolaire.model.EolaireModel.VariantType.valueOf(type_);
return result == null ? com.truward.orion.eolaire.model.EolaireModel.VariantType.UNRECOGNIZED : result;
}
public static final int VALUE_FIELD_NUMBER = 3;
private com.truward.orion.eolaire.model.EolaireModel.VariantValue value_;
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public boolean hasValue() {
return value_ != null;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantValue getValue() {
return value_ == null ? com.truward.orion.eolaire.model.EolaireModel.VariantValue.getDefaultInstance() : value_;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder getValueOrBuilder() {
return getValue();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, key_);
}
if (type_ != com.truward.orion.eolaire.model.EolaireModel.VariantType.NULL.getNumber()) {
output.writeEnum(2, type_);
}
if (value_ != null) {
output.writeMessage(3, getValue());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, key_);
}
if (type_ != com.truward.orion.eolaire.model.EolaireModel.VariantType.NULL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
if (value_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getValue());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.MetadataEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.MetadataEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.MetadataEntry)
com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_MetadataEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_MetadataEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.class, com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
key_ = "";
type_ = 0;
if (valueBuilder_ == null) {
value_ = null;
} else {
value_ = null;
valueBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_MetadataEntry_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry build() {
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry result = new com.truward.orion.eolaire.model.EolaireModel.MetadataEntry(this);
result.key_ = key_;
result.type_ = type_;
if (valueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.MetadataEntry) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.MetadataEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.MetadataEntry other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.getDefaultInstance()) return this;
if (!other.getKey().isEmpty()) {
key_ = other.key_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.MetadataEntry) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
key_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantType getType() {
com.truward.orion.eolaire.model.EolaireModel.VariantType result = com.truward.orion.eolaire.model.EolaireModel.VariantType.valueOf(type_);
return result == null ? com.truward.orion.eolaire.model.EolaireModel.VariantType.UNRECOGNIZED : result;
}
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public Builder setType(com.truward.orion.eolaire.model.EolaireModel.VariantType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .truward.orion.user.VariantType type = 2;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.VariantValue value_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.VariantValue, com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder, com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder> valueBuilder_;
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public boolean hasValue() {
return valueBuilder_ != null || value_ != null;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantValue getValue() {
if (valueBuilder_ == null) {
return value_ == null ? com.truward.orion.eolaire.model.EolaireModel.VariantValue.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public Builder setValue(com.truward.orion.eolaire.model.EolaireModel.VariantValue value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
valueBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public Builder setValue(
com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public Builder mergeValue(com.truward.orion.eolaire.model.EolaireModel.VariantValue value) {
if (valueBuilder_ == null) {
if (value_ != null) {
value_ =
com.truward.orion.eolaire.model.EolaireModel.VariantValue.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
valueBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = null;
onChanged();
} else {
value_ = null;
valueBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder getValueBuilder() {
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
public com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
com.truward.orion.eolaire.model.EolaireModel.VariantValue.getDefaultInstance() : value_;
}
}
/**
* optional .truward.orion.user.VariantValue value = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.VariantValue, com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder, com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.VariantValue, com.truward.orion.eolaire.model.EolaireModel.VariantValue.Builder, com.truward.orion.eolaire.model.EolaireModel.VariantValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.MetadataEntry)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.MetadataEntry)
private static final com.truward.orion.eolaire.model.EolaireModel.MetadataEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.MetadataEntry();
}
public static com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MetadataEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new MetadataEntry(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.Metadata)
com.google.protobuf.MessageOrBuilder {
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
boolean hasEntries();
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getEntries();
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder getEntriesOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.Metadata}
*/
public static final class Metadata extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.Metadata)
MetadataOrBuilder {
// Use Metadata.newBuilder() to construct.
private Metadata(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Metadata() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Metadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder subBuilder = null;
if (entries_ != null) {
subBuilder = entries_.toBuilder();
}
entries_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(entries_);
entries_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Metadata_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.Metadata.class, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder.class);
}
public static final int ENTRIES_FIELD_NUMBER = 1;
private com.truward.orion.eolaire.model.EolaireModel.MetadataEntry entries_;
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public boolean hasEntries() {
return entries_ != null;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getEntries() {
return entries_ == null ? com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.getDefaultInstance() : entries_;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder getEntriesOrBuilder() {
return getEntries();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (entries_ != null) {
output.writeMessage(1, getEntries());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (entries_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getEntries());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.Metadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.Metadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.Metadata)
com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Metadata_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Metadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.Metadata.class, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.Metadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (entriesBuilder_ == null) {
entries_ = null;
} else {
entries_ = null;
entriesBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_Metadata_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.Metadata getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.Metadata build() {
com.truward.orion.eolaire.model.EolaireModel.Metadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.Metadata buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.Metadata result = new com.truward.orion.eolaire.model.EolaireModel.Metadata(this);
if (entriesBuilder_ == null) {
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.Metadata) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.Metadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.Metadata other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance()) return this;
if (other.hasEntries()) {
mergeEntries(other.getEntries());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.Metadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.Metadata) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.MetadataEntry entries_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry, com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder> entriesBuilder_;
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public boolean hasEntries() {
return entriesBuilder_ != null || entries_ != null;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry getEntries() {
if (entriesBuilder_ == null) {
return entries_ == null ? com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.getDefaultInstance() : entries_;
} else {
return entriesBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public Builder setEntries(com.truward.orion.eolaire.model.EolaireModel.MetadataEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
entries_ = value;
onChanged();
} else {
entriesBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public Builder setEntries(
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
entries_ = builderForValue.build();
onChanged();
} else {
entriesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public Builder mergeEntries(com.truward.orion.eolaire.model.EolaireModel.MetadataEntry value) {
if (entriesBuilder_ == null) {
if (entries_ != null) {
entries_ =
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.newBuilder(entries_).mergeFrom(value).buildPartial();
} else {
entries_ = value;
}
onChanged();
} else {
entriesBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = null;
onChanged();
} else {
entries_ = null;
entriesBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder getEntriesBuilder() {
onChanged();
return getEntriesFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder getEntriesOrBuilder() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilder();
} else {
return entries_ == null ?
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.getDefaultInstance() : entries_;
}
}
/**
* optional .truward.orion.user.MetadataEntry entries = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry, com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.MetadataEntry, com.truward.orion.eolaire.model.EolaireModel.MetadataEntry.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataEntryOrBuilder>(
getEntries(),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.Metadata)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.Metadata)
private static final com.truward.orion.eolaire.model.EolaireModel.Metadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.Metadata();
}
public static com.truward.orion.eolaire.model.EolaireModel.Metadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Metadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new Metadata(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.Metadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ItemProfileOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.ItemProfile)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 itemId = 1;
*/
long getItemId();
/**
* optional string description = 2;
*/
java.lang.String getDescription();
/**
* optional string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* optional int64 created = 3;
*/
long getCreated();
/**
* optional int64 updated = 4;
*/
long getUpdated();
/**
* optional int64 flags = 5;
*/
long getFlags();
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
boolean hasMetadata();
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata();
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.ItemProfile}
*
*
**
* Optional profile
*
*/
public static final class ItemProfile extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.ItemProfile)
ItemProfileOrBuilder {
// Use ItemProfile.newBuilder() to construct.
private ItemProfile(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private ItemProfile() {
itemId_ = 0L;
description_ = "";
created_ = 0L;
updated_ = 0L;
flags_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private ItemProfile(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
itemId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 24: {
created_ = input.readInt64();
break;
}
case 32: {
updated_ = input.readInt64();
break;
}
case 40: {
flags_ = input.readInt64();
break;
}
case 50: {
com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder subBuilder = null;
if (metadata_ != null) {
subBuilder = metadata_.toBuilder();
}
metadata_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.Metadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(metadata_);
metadata_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemProfile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.class, com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder.class);
}
public static final int ITEMID_FIELD_NUMBER = 1;
private long itemId_;
/**
* optional int64 itemId = 1;
*/
public long getItemId() {
return itemId_;
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
* optional string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* optional string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATED_FIELD_NUMBER = 3;
private long created_;
/**
* optional int64 created = 3;
*/
public long getCreated() {
return created_;
}
public static final int UPDATED_FIELD_NUMBER = 4;
private long updated_;
/**
* optional int64 updated = 4;
*/
public long getUpdated() {
return updated_;
}
public static final int FLAGS_FIELD_NUMBER = 5;
private long flags_;
/**
* optional int64 flags = 5;
*/
public long getFlags() {
return flags_;
}
public static final int METADATA_FIELD_NUMBER = 6;
private com.truward.orion.eolaire.model.EolaireModel.Metadata metadata_;
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public boolean hasMetadata() {
return metadata_ != null;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata() {
return metadata_ == null ? com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder() {
return getMetadata();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (itemId_ != 0L) {
output.writeInt64(1, itemId_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, description_);
}
if (created_ != 0L) {
output.writeInt64(3, created_);
}
if (updated_ != 0L) {
output.writeInt64(4, updated_);
}
if (flags_ != 0L) {
output.writeInt64(5, flags_);
}
if (metadata_ != null) {
output.writeMessage(6, getMetadata());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (itemId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, itemId_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, description_);
}
if (created_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, created_);
}
if (updated_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, updated_);
}
if (flags_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, flags_);
}
if (metadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getMetadata());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.ItemProfile prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.ItemProfile}
*
*
**
* Optional profile
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.ItemProfile)
com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemProfile_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemProfile_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.class, com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.ItemProfile.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
itemId_ = 0L;
description_ = "";
created_ = 0L;
updated_ = 0L;
flags_ = 0L;
if (metadataBuilder_ == null) {
metadata_ = null;
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_ItemProfile_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.ItemProfile.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile build() {
com.truward.orion.eolaire.model.EolaireModel.ItemProfile result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.ItemProfile result = new com.truward.orion.eolaire.model.EolaireModel.ItemProfile(this);
result.itemId_ = itemId_;
result.description_ = description_;
result.created_ = created_;
result.updated_ = updated_;
result.flags_ = flags_;
if (metadataBuilder_ == null) {
result.metadata_ = metadata_;
} else {
result.metadata_ = metadataBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.ItemProfile) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.ItemProfile)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.ItemProfile other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.ItemProfile.getDefaultInstance()) return this;
if (other.getItemId() != 0L) {
setItemId(other.getItemId());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (other.getCreated() != 0L) {
setCreated(other.getCreated());
}
if (other.getUpdated() != 0L) {
setUpdated(other.getUpdated());
}
if (other.getFlags() != 0L) {
setFlags(other.getFlags());
}
if (other.hasMetadata()) {
mergeMetadata(other.getMetadata());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.ItemProfile parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.ItemProfile) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long itemId_ ;
/**
* optional int64 itemId = 1;
*/
public long getItemId() {
return itemId_;
}
/**
* optional int64 itemId = 1;
*/
public Builder setItemId(long value) {
itemId_ = value;
onChanged();
return this;
}
/**
* optional int64 itemId = 1;
*/
public Builder clearItemId() {
itemId_ = 0L;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* optional string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* optional string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* optional string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private long created_ ;
/**
* optional int64 created = 3;
*/
public long getCreated() {
return created_;
}
/**
* optional int64 created = 3;
*/
public Builder setCreated(long value) {
created_ = value;
onChanged();
return this;
}
/**
* optional int64 created = 3;
*/
public Builder clearCreated() {
created_ = 0L;
onChanged();
return this;
}
private long updated_ ;
/**
* optional int64 updated = 4;
*/
public long getUpdated() {
return updated_;
}
/**
* optional int64 updated = 4;
*/
public Builder setUpdated(long value) {
updated_ = value;
onChanged();
return this;
}
/**
* optional int64 updated = 4;
*/
public Builder clearUpdated() {
updated_ = 0L;
onChanged();
return this;
}
private long flags_ ;
/**
* optional int64 flags = 5;
*/
public long getFlags() {
return flags_;
}
/**
* optional int64 flags = 5;
*/
public Builder setFlags(long value) {
flags_ = value;
onChanged();
return this;
}
/**
* optional int64 flags = 5;
*/
public Builder clearFlags() {
flags_ = 0L;
onChanged();
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.Metadata metadata_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder> metadataBuilder_;
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public boolean hasMetadata() {
return metadataBuilder_ != null || metadata_ != null;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata getMetadata() {
if (metadataBuilder_ == null) {
return metadata_ == null ? com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
} else {
return metadataBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public Builder setMetadata(com.truward.orion.eolaire.model.EolaireModel.Metadata value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metadata_ = value;
onChanged();
} else {
metadataBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public Builder setMetadata(
com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder builderForValue) {
if (metadataBuilder_ == null) {
metadata_ = builderForValue.build();
onChanged();
} else {
metadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public Builder mergeMetadata(com.truward.orion.eolaire.model.EolaireModel.Metadata value) {
if (metadataBuilder_ == null) {
if (metadata_ != null) {
metadata_ =
com.truward.orion.eolaire.model.EolaireModel.Metadata.newBuilder(metadata_).mergeFrom(value).buildPartial();
} else {
metadata_ = value;
}
onChanged();
} else {
metadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public Builder clearMetadata() {
if (metadataBuilder_ == null) {
metadata_ = null;
onChanged();
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder getMetadataBuilder() {
onChanged();
return getMetadataFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
public com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder getMetadataOrBuilder() {
if (metadataBuilder_ != null) {
return metadataBuilder_.getMessageOrBuilder();
} else {
return metadata_ == null ?
com.truward.orion.eolaire.model.EolaireModel.Metadata.getDefaultInstance() : metadata_;
}
}
/**
* optional .truward.orion.user.Metadata metadata = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder>
getMetadataFieldBuilder() {
if (metadataBuilder_ == null) {
metadataBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Metadata, com.truward.orion.eolaire.model.EolaireModel.Metadata.Builder, com.truward.orion.eolaire.model.EolaireModel.MetadataOrBuilder>(
getMetadata(),
getParentForChildren(),
isClean());
metadata_ = null;
}
return metadataBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.ItemProfile)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.ItemProfile)
private static final com.truward.orion.eolaire.model.EolaireModel.ItemProfile DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.ItemProfile();
}
public static com.truward.orion.eolaire.model.EolaireModel.ItemProfile getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ItemProfile parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new ItemProfile(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated int64 itemIds = 1;
*/
java.util.List getItemIdsList();
/**
* repeated int64 itemIds = 1;
*/
int getItemIdsCount();
/**
* repeated int64 itemIds = 1;
*/
long getItemIds(int index);
}
/**
* Protobuf type {@code truward.orion.user.GetItemsRequest}
*/
public static final class GetItemsRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemsRequest)
GetItemsRequestOrBuilder {
// Use GetItemsRequest.newBuilder() to construct.
private GetItemsRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemsRequest() {
itemIds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
itemIds_.add(input.readInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
itemIds_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.Builder.class);
}
public static final int ITEMIDS_FIELD_NUMBER = 1;
private java.util.List itemIds_;
/**
* repeated int64 itemIds = 1;
*/
public java.util.List
getItemIdsList() {
return itemIds_;
}
/**
* repeated int64 itemIds = 1;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 1;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
private int itemIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getItemIdsList().size() > 0) {
output.writeRawVarint32(10);
output.writeRawVarint32(itemIdsMemoizedSerializedSize);
}
for (int i = 0; i < itemIds_.size(); i++) {
output.writeInt64NoTag(itemIds_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < itemIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(itemIds_.get(i));
}
size += dataSize;
if (!getItemIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
itemIdsMemoizedSerializedSize = dataSize;
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemsRequest)
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsRequest_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest result = new com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.itemIds_ = itemIds_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest.getDefaultInstance()) return this;
if (!other.itemIds_.isEmpty()) {
if (itemIds_.isEmpty()) {
itemIds_ = other.itemIds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemIdsIsMutable();
itemIds_.addAll(other.itemIds_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List itemIds_ = java.util.Collections.emptyList();
private void ensureItemIdsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
itemIds_ = new java.util.ArrayList(itemIds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 itemIds = 1;
*/
public java.util.List
getItemIdsList() {
return java.util.Collections.unmodifiableList(itemIds_);
}
/**
* repeated int64 itemIds = 1;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 1;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
/**
* repeated int64 itemIds = 1;
*/
public Builder setItemIds(
int index, long value) {
ensureItemIdsIsMutable();
itemIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 1;
*/
public Builder addItemIds(long value) {
ensureItemIdsIsMutable();
itemIds_.add(value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 1;
*/
public Builder addAllItemIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureItemIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, itemIds_);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 1;
*/
public Builder clearItemIds() {
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemsRequest)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemsRequest)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemsRequest(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .truward.orion.user.Item items = 2;
*/
java.util.List
getItemsList();
/**
* repeated .truward.orion.user.Item items = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.Item getItems(int index);
/**
* repeated .truward.orion.user.Item items = 2;
*/
int getItemsCount();
/**
* repeated .truward.orion.user.Item items = 2;
*/
java.util.List extends com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>
getItemsOrBuilderList();
/**
* repeated .truward.orion.user.Item items = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemsOrBuilder(
int index);
}
/**
* Protobuf type {@code truward.orion.user.GetItemsResponse}
*/
public static final class GetItemsResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemsResponse)
GetItemsResponseOrBuilder {
// Use GetItemsResponse.newBuilder() to construct.
private GetItemsResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemsResponse() {
items_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
items_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
items_.add(input.readMessage(com.truward.orion.eolaire.model.EolaireModel.Item.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
items_ = java.util.Collections.unmodifiableList(items_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.Builder.class);
}
public static final int ITEMS_FIELD_NUMBER = 2;
private java.util.List items_;
/**
* repeated .truward.orion.user.Item items = 2;
*/
public java.util.List getItemsList() {
return items_;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public java.util.List extends com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>
getItemsOrBuilderList() {
return items_;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public int getItemsCount() {
return items_.size();
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item getItems(int index) {
return items_.get(index);
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemsOrBuilder(
int index) {
return items_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < items_.size(); i++) {
output.writeMessage(2, items_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < items_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, items_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemsResponse)
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getItemsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (itemsBuilder_ == null) {
items_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
itemsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemsResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse(this);
int from_bitField0_ = bitField0_;
if (itemsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
items_ = java.util.Collections.unmodifiableList(items_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.items_ = items_;
} else {
result.items_ = itemsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse.getDefaultInstance()) return this;
if (itemsBuilder_ == null) {
if (!other.items_.isEmpty()) {
if (items_.isEmpty()) {
items_ = other.items_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemsIsMutable();
items_.addAll(other.items_);
}
onChanged();
}
} else {
if (!other.items_.isEmpty()) {
if (itemsBuilder_.isEmpty()) {
itemsBuilder_.dispose();
itemsBuilder_ = null;
items_ = other.items_;
bitField0_ = (bitField0_ & ~0x00000001);
itemsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getItemsFieldBuilder() : null;
} else {
itemsBuilder_.addAllMessages(other.items_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List items_ =
java.util.Collections.emptyList();
private void ensureItemsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
items_ = new java.util.ArrayList(items_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder> itemsBuilder_;
/**
* repeated .truward.orion.user.Item items = 2;
*/
public java.util.List getItemsList() {
if (itemsBuilder_ == null) {
return java.util.Collections.unmodifiableList(items_);
} else {
return itemsBuilder_.getMessageList();
}
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public int getItemsCount() {
if (itemsBuilder_ == null) {
return items_.size();
} else {
return itemsBuilder_.getCount();
}
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item getItems(int index) {
if (itemsBuilder_ == null) {
return items_.get(index);
} else {
return itemsBuilder_.getMessage(index);
}
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder setItems(
int index, com.truward.orion.eolaire.model.EolaireModel.Item value) {
if (itemsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemsIsMutable();
items_.set(index, value);
onChanged();
} else {
itemsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder setItems(
int index, com.truward.orion.eolaire.model.EolaireModel.Item.Builder builderForValue) {
if (itemsBuilder_ == null) {
ensureItemsIsMutable();
items_.set(index, builderForValue.build());
onChanged();
} else {
itemsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder addItems(com.truward.orion.eolaire.model.EolaireModel.Item value) {
if (itemsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemsIsMutable();
items_.add(value);
onChanged();
} else {
itemsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder addItems(
int index, com.truward.orion.eolaire.model.EolaireModel.Item value) {
if (itemsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemsIsMutable();
items_.add(index, value);
onChanged();
} else {
itemsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder addItems(
com.truward.orion.eolaire.model.EolaireModel.Item.Builder builderForValue) {
if (itemsBuilder_ == null) {
ensureItemsIsMutable();
items_.add(builderForValue.build());
onChanged();
} else {
itemsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder addItems(
int index, com.truward.orion.eolaire.model.EolaireModel.Item.Builder builderForValue) {
if (itemsBuilder_ == null) {
ensureItemsIsMutable();
items_.add(index, builderForValue.build());
onChanged();
} else {
itemsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder addAllItems(
java.lang.Iterable extends com.truward.orion.eolaire.model.EolaireModel.Item> values) {
if (itemsBuilder_ == null) {
ensureItemsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, items_);
onChanged();
} else {
itemsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder clearItems() {
if (itemsBuilder_ == null) {
items_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
itemsBuilder_.clear();
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public Builder removeItems(int index) {
if (itemsBuilder_ == null) {
ensureItemsIsMutable();
items_.remove(index);
onChanged();
} else {
itemsBuilder_.remove(index);
}
return this;
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item.Builder getItemsBuilder(
int index) {
return getItemsFieldBuilder().getBuilder(index);
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder getItemsOrBuilder(
int index) {
if (itemsBuilder_ == null) {
return items_.get(index); } else {
return itemsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public java.util.List extends com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>
getItemsOrBuilderList() {
if (itemsBuilder_ != null) {
return itemsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(items_);
}
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item.Builder addItemsBuilder() {
return getItemsFieldBuilder().addBuilder(
com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance());
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.Item.Builder addItemsBuilder(
int index) {
return getItemsFieldBuilder().addBuilder(
index, com.truward.orion.eolaire.model.EolaireModel.Item.getDefaultInstance());
}
/**
* repeated .truward.orion.user.Item items = 2;
*/
public java.util.List
getItemsBuilderList() {
return getItemsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>
getItemsFieldBuilder() {
if (itemsBuilder_ == null) {
itemsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.Item, com.truward.orion.eolaire.model.EolaireModel.Item.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemOrBuilder>(
items_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
items_ = null;
}
return itemsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemsResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemsResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemsResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemProfileResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemProfileResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
boolean hasProfile();
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemProfile getProfile();
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder getProfileOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.GetItemProfileResponse}
*/
public static final class GetItemProfileResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemProfileResponse)
GetItemProfileResponseOrBuilder {
// Use GetItemProfileResponse.newBuilder() to construct.
private GetItemProfileResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemProfileResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemProfileResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder subBuilder = null;
if (profile_ != null) {
subBuilder = profile_.toBuilder();
}
profile_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.ItemProfile.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(profile_);
profile_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemProfileResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.Builder.class);
}
public static final int PROFILE_FIELD_NUMBER = 1;
private com.truward.orion.eolaire.model.EolaireModel.ItemProfile profile_;
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public boolean hasProfile() {
return profile_ != null;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile getProfile() {
return profile_ == null ? com.truward.orion.eolaire.model.EolaireModel.ItemProfile.getDefaultInstance() : profile_;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder getProfileOrBuilder() {
return getProfile();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (profile_ != null) {
output.writeMessage(1, getProfile());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (profile_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getProfile());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemProfileResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemProfileResponse)
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemProfileResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (profileBuilder_ == null) {
profile_ = null;
} else {
profile_ = null;
profileBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemProfileResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse(this);
if (profileBuilder_ == null) {
result.profile_ = profile_;
} else {
result.profile_ = profileBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse.getDefaultInstance()) return this;
if (other.hasProfile()) {
mergeProfile(other.getProfile());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.ItemProfile profile_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemProfile, com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder> profileBuilder_;
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public boolean hasProfile() {
return profileBuilder_ != null || profile_ != null;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile getProfile() {
if (profileBuilder_ == null) {
return profile_ == null ? com.truward.orion.eolaire.model.EolaireModel.ItemProfile.getDefaultInstance() : profile_;
} else {
return profileBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public Builder setProfile(com.truward.orion.eolaire.model.EolaireModel.ItemProfile value) {
if (profileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
profile_ = value;
onChanged();
} else {
profileBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public Builder setProfile(
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder builderForValue) {
if (profileBuilder_ == null) {
profile_ = builderForValue.build();
onChanged();
} else {
profileBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public Builder mergeProfile(com.truward.orion.eolaire.model.EolaireModel.ItemProfile value) {
if (profileBuilder_ == null) {
if (profile_ != null) {
profile_ =
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.newBuilder(profile_).mergeFrom(value).buildPartial();
} else {
profile_ = value;
}
onChanged();
} else {
profileBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public Builder clearProfile() {
if (profileBuilder_ == null) {
profile_ = null;
onChanged();
} else {
profile_ = null;
profileBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder getProfileBuilder() {
onChanged();
return getProfileFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
public com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder getProfileOrBuilder() {
if (profileBuilder_ != null) {
return profileBuilder_.getMessageOrBuilder();
} else {
return profile_ == null ?
com.truward.orion.eolaire.model.EolaireModel.ItemProfile.getDefaultInstance() : profile_;
}
}
/**
* optional .truward.orion.user.ItemProfile profile = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemProfile, com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder>
getProfileFieldBuilder() {
if (profileBuilder_ == null) {
profileBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.ItemProfile, com.truward.orion.eolaire.model.EolaireModel.ItemProfile.Builder, com.truward.orion.eolaire.model.EolaireModel.ItemProfileOrBuilder>(
getProfile(),
getParentForChildren(),
isClean());
profile_ = null;
}
return profileBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemProfileResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemProfileResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemProfileResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemProfileResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemProfileResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetEntityTypeResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetEntityTypeResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .truward.orion.user.EntityType type = 2;
*/
boolean hasType();
/**
* optional .truward.orion.user.EntityType type = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.EntityType getType();
/**
* optional .truward.orion.user.EntityType type = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypeOrBuilder();
}
/**
* Protobuf type {@code truward.orion.user.GetEntityTypeResponse}
*/
public static final class GetEntityTypeResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetEntityTypeResponse)
GetEntityTypeResponseOrBuilder {
// Use GetEntityTypeResponse.newBuilder() to construct.
private GetEntityTypeResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetEntityTypeResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetEntityTypeResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 18: {
com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder subBuilder = null;
if (type_ != null) {
subBuilder = type_.toBuilder();
}
type_ = input.readMessage(com.truward.orion.eolaire.model.EolaireModel.EntityType.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(type_);
type_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetEntityTypeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetEntityTypeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.truward.orion.eolaire.model.EolaireModel.EntityType type_;
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public boolean hasType() {
return type_ != null;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType getType() {
return type_ == null ? com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance() : type_;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypeOrBuilder() {
return getType();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != null) {
output.writeMessage(2, getType());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getType());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetEntityTypeResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetEntityTypeResponse)
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetEntityTypeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetEntityTypeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (typeBuilder_ == null) {
type_ = null;
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetEntityTypeResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse(this);
if (typeBuilder_ == null) {
result.type_ = type_;
} else {
result.type_ = typeBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse.getDefaultInstance()) return this;
if (other.hasType()) {
mergeType(other.getType());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.truward.orion.eolaire.model.EolaireModel.EntityType type_ = null;
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder> typeBuilder_;
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public boolean hasType() {
return typeBuilder_ != null || type_ != null;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType getType() {
if (typeBuilder_ == null) {
return type_ == null ? com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance() : type_;
} else {
return typeBuilder_.getMessage();
}
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public Builder setType(com.truward.orion.eolaire.model.EolaireModel.EntityType value) {
if (typeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
} else {
typeBuilder_.setMessage(value);
}
return this;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public Builder setType(
com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder builderForValue) {
if (typeBuilder_ == null) {
type_ = builderForValue.build();
onChanged();
} else {
typeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public Builder mergeType(com.truward.orion.eolaire.model.EolaireModel.EntityType value) {
if (typeBuilder_ == null) {
if (type_ != null) {
type_ =
com.truward.orion.eolaire.model.EolaireModel.EntityType.newBuilder(type_).mergeFrom(value).buildPartial();
} else {
type_ = value;
}
onChanged();
} else {
typeBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public Builder clearType() {
if (typeBuilder_ == null) {
type_ = null;
onChanged();
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder getTypeBuilder() {
onChanged();
return getTypeFieldBuilder().getBuilder();
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypeOrBuilder() {
if (typeBuilder_ != null) {
return typeBuilder_.getMessageOrBuilder();
} else {
return type_ == null ?
com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance() : type_;
}
}
/**
* optional .truward.orion.user.EntityType type = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>
getTypeFieldBuilder() {
if (typeBuilder_ == null) {
typeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>(
getType(),
getParentForChildren(),
isClean());
type_ = null;
}
return typeBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetEntityTypeResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetEntityTypeResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetEntityTypeResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetEntityTypeResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetEntityTypeResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetAllEntityTypesRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetAllEntityTypesRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* optional int32 limit = 2;
*/
int getLimit();
}
/**
* Protobuf type {@code truward.orion.user.GetAllEntityTypesRequest}
*/
public static final class GetAllEntityTypesRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetAllEntityTypesRequest)
GetAllEntityTypesRequestOrBuilder {
// Use GetAllEntityTypesRequest.newBuilder() to construct.
private GetAllEntityTypesRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetAllEntityTypesRequest() {
offsetToken_ = "";
limit_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetAllEntityTypesRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 16: {
limit_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.Builder.class);
}
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LIMIT_FIELD_NUMBER = 2;
private int limit_;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
if (limit_ != 0) {
output.writeInt32(2, limit_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
if (limit_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, limit_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetAllEntityTypesRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetAllEntityTypesRequest)
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
limit_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest build() {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest result = new com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest(this);
result.offsetToken_ = offsetToken_;
result.limit_ = limit_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (other.getLimit() != 0) {
setLimit(other.getLimit());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 2;
*/
public Builder setLimit(int value) {
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 2;
*/
public Builder clearLimit() {
limit_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetAllEntityTypesRequest)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetAllEntityTypesRequest)
private static final com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetAllEntityTypesRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetAllEntityTypesRequest(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetAllEntityTypesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetAllEntityTypesResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
java.util.List
getTypesList();
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.EntityType getTypes(int index);
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
int getTypesCount();
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
java.util.List extends com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>
getTypesOrBuilderList();
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypesOrBuilder(
int index);
}
/**
* Protobuf type {@code truward.orion.user.GetAllEntityTypesResponse}
*/
public static final class GetAllEntityTypesResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetAllEntityTypesResponse)
GetAllEntityTypesResponseOrBuilder {
// Use GetAllEntityTypesResponse.newBuilder() to construct.
private GetAllEntityTypesResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetAllEntityTypesResponse() {
offsetToken_ = "";
types_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetAllEntityTypesResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
types_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
types_.add(input.readMessage(com.truward.orion.eolaire.model.EolaireModel.EntityType.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
types_ = java.util.Collections.unmodifiableList(types_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.Builder.class);
}
private int bitField0_;
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPES_FIELD_NUMBER = 2;
private java.util.List types_;
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public java.util.List getTypesList() {
return types_;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public java.util.List extends com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>
getTypesOrBuilderList() {
return types_;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public int getTypesCount() {
return types_.size();
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType getTypes(int index) {
return types_.get(index);
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypesOrBuilder(
int index) {
return types_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
for (int i = 0; i < types_.size(); i++) {
output.writeMessage(2, types_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
for (int i = 0; i < types_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, types_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetAllEntityTypesResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetAllEntityTypesResponse)
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTypesFieldBuilder();
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
if (typesBuilder_ == null) {
types_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
typesBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.offsetToken_ = offsetToken_;
if (typesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
types_ = java.util.Collections.unmodifiableList(types_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.types_ = types_;
} else {
result.types_ = typesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (typesBuilder_ == null) {
if (!other.types_.isEmpty()) {
if (types_.isEmpty()) {
types_ = other.types_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTypesIsMutable();
types_.addAll(other.types_);
}
onChanged();
}
} else {
if (!other.types_.isEmpty()) {
if (typesBuilder_.isEmpty()) {
typesBuilder_.dispose();
typesBuilder_ = null;
types_ = other.types_;
bitField0_ = (bitField0_ & ~0x00000002);
typesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getTypesFieldBuilder() : null;
} else {
typesBuilder_.addAllMessages(other.types_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private java.util.List types_ =
java.util.Collections.emptyList();
private void ensureTypesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
types_ = new java.util.ArrayList(types_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder> typesBuilder_;
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public java.util.List getTypesList() {
if (typesBuilder_ == null) {
return java.util.Collections.unmodifiableList(types_);
} else {
return typesBuilder_.getMessageList();
}
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public int getTypesCount() {
if (typesBuilder_ == null) {
return types_.size();
} else {
return typesBuilder_.getCount();
}
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType getTypes(int index) {
if (typesBuilder_ == null) {
return types_.get(index);
} else {
return typesBuilder_.getMessage(index);
}
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder setTypes(
int index, com.truward.orion.eolaire.model.EolaireModel.EntityType value) {
if (typesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTypesIsMutable();
types_.set(index, value);
onChanged();
} else {
typesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder setTypes(
int index, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder builderForValue) {
if (typesBuilder_ == null) {
ensureTypesIsMutable();
types_.set(index, builderForValue.build());
onChanged();
} else {
typesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder addTypes(com.truward.orion.eolaire.model.EolaireModel.EntityType value) {
if (typesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTypesIsMutable();
types_.add(value);
onChanged();
} else {
typesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder addTypes(
int index, com.truward.orion.eolaire.model.EolaireModel.EntityType value) {
if (typesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTypesIsMutable();
types_.add(index, value);
onChanged();
} else {
typesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder addTypes(
com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder builderForValue) {
if (typesBuilder_ == null) {
ensureTypesIsMutable();
types_.add(builderForValue.build());
onChanged();
} else {
typesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder addTypes(
int index, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder builderForValue) {
if (typesBuilder_ == null) {
ensureTypesIsMutable();
types_.add(index, builderForValue.build());
onChanged();
} else {
typesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder addAllTypes(
java.lang.Iterable extends com.truward.orion.eolaire.model.EolaireModel.EntityType> values) {
if (typesBuilder_ == null) {
ensureTypesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, types_);
onChanged();
} else {
typesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder clearTypes() {
if (typesBuilder_ == null) {
types_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
typesBuilder_.clear();
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public Builder removeTypes(int index) {
if (typesBuilder_ == null) {
ensureTypesIsMutable();
types_.remove(index);
onChanged();
} else {
typesBuilder_.remove(index);
}
return this;
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder getTypesBuilder(
int index) {
return getTypesFieldBuilder().getBuilder(index);
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder getTypesOrBuilder(
int index) {
if (typesBuilder_ == null) {
return types_.get(index); } else {
return typesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public java.util.List extends com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>
getTypesOrBuilderList() {
if (typesBuilder_ != null) {
return typesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(types_);
}
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder addTypesBuilder() {
return getTypesFieldBuilder().addBuilder(
com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance());
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder addTypesBuilder(
int index) {
return getTypesFieldBuilder().addBuilder(
index, com.truward.orion.eolaire.model.EolaireModel.EntityType.getDefaultInstance());
}
/**
* repeated .truward.orion.user.EntityType types = 2;
*/
public java.util.List
getTypesBuilderList() {
return getTypesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>
getTypesFieldBuilder() {
if (typesBuilder_ == null) {
typesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.truward.orion.eolaire.model.EolaireModel.EntityType, com.truward.orion.eolaire.model.EolaireModel.EntityType.Builder, com.truward.orion.eolaire.model.EolaireModel.EntityTypeOrBuilder>(
types_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
types_ = null;
}
return typesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetAllEntityTypesResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetAllEntityTypesResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetAllEntityTypesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetAllEntityTypesResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetAllEntityTypesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemByTypeRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemByTypeRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* optional int32 limit = 2;
*/
int getLimit();
/**
* optional int64 itemTypeId = 3;
*/
long getItemTypeId();
}
/**
* Protobuf type {@code truward.orion.user.GetItemByTypeRequest}
*/
public static final class GetItemByTypeRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemByTypeRequest)
GetItemByTypeRequestOrBuilder {
// Use GetItemByTypeRequest.newBuilder() to construct.
private GetItemByTypeRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemByTypeRequest() {
offsetToken_ = "";
limit_ = 0;
itemTypeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemByTypeRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 16: {
limit_ = input.readInt32();
break;
}
case 24: {
itemTypeId_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.Builder.class);
}
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LIMIT_FIELD_NUMBER = 2;
private int limit_;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
public static final int ITEMTYPEID_FIELD_NUMBER = 3;
private long itemTypeId_;
/**
* optional int64 itemTypeId = 3;
*/
public long getItemTypeId() {
return itemTypeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
if (limit_ != 0) {
output.writeInt32(2, limit_);
}
if (itemTypeId_ != 0L) {
output.writeInt64(3, itemTypeId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
if (limit_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, limit_);
}
if (itemTypeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, itemTypeId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemByTypeRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemByTypeRequest)
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
limit_ = 0;
itemTypeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeRequest_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest result = new com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest(this);
result.offsetToken_ = offsetToken_;
result.limit_ = limit_;
result.itemTypeId_ = itemTypeId_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (other.getLimit() != 0) {
setLimit(other.getLimit());
}
if (other.getItemTypeId() != 0L) {
setItemTypeId(other.getItemTypeId());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 2;
*/
public Builder setLimit(int value) {
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 2;
*/
public Builder clearLimit() {
limit_ = 0;
onChanged();
return this;
}
private long itemTypeId_ ;
/**
* optional int64 itemTypeId = 3;
*/
public long getItemTypeId() {
return itemTypeId_;
}
/**
* optional int64 itemTypeId = 3;
*/
public Builder setItemTypeId(long value) {
itemTypeId_ = value;
onChanged();
return this;
}
/**
* optional int64 itemTypeId = 3;
*/
public Builder clearItemTypeId() {
itemTypeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemByTypeRequest)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemByTypeRequest)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemByTypeRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemByTypeRequest(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemByTypeResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemByTypeResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* repeated int64 itemIds = 2;
*/
java.util.List getItemIdsList();
/**
* repeated int64 itemIds = 2;
*/
int getItemIdsCount();
/**
* repeated int64 itemIds = 2;
*/
long getItemIds(int index);
}
/**
* Protobuf type {@code truward.orion.user.GetItemByTypeResponse}
*/
public static final class GetItemByTypeResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemByTypeResponse)
GetItemByTypeResponseOrBuilder {
// Use GetItemByTypeResponse.newBuilder() to construct.
private GetItemByTypeResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemByTypeResponse() {
offsetToken_ = "";
itemIds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemByTypeResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
itemIds_.add(input.readInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
itemIds_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.Builder.class);
}
private int bitField0_;
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEMIDS_FIELD_NUMBER = 2;
private java.util.List itemIds_;
/**
* repeated int64 itemIds = 2;
*/
public java.util.List
getItemIdsList() {
return itemIds_;
}
/**
* repeated int64 itemIds = 2;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 2;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
private int itemIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
if (getItemIdsList().size() > 0) {
output.writeRawVarint32(18);
output.writeRawVarint32(itemIdsMemoizedSerializedSize);
}
for (int i = 0; i < itemIds_.size(); i++) {
output.writeInt64NoTag(itemIds_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
{
int dataSize = 0;
for (int i = 0; i < itemIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(itemIds_.get(i));
}
size += dataSize;
if (!getItemIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
itemIdsMemoizedSerializedSize = dataSize;
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemByTypeResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemByTypeResponse)
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByTypeResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.offsetToken_ = offsetToken_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.itemIds_ = itemIds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (!other.itemIds_.isEmpty()) {
if (itemIds_.isEmpty()) {
itemIds_ = other.itemIds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureItemIdsIsMutable();
itemIds_.addAll(other.itemIds_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private java.util.List itemIds_ = java.util.Collections.emptyList();
private void ensureItemIdsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = new java.util.ArrayList(itemIds_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated int64 itemIds = 2;
*/
public java.util.List
getItemIdsList() {
return java.util.Collections.unmodifiableList(itemIds_);
}
/**
* repeated int64 itemIds = 2;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 2;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
/**
* repeated int64 itemIds = 2;
*/
public Builder setItemIds(
int index, long value) {
ensureItemIdsIsMutable();
itemIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder addItemIds(long value) {
ensureItemIdsIsMutable();
itemIds_.add(value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder addAllItemIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureItemIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, itemIds_);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder clearItemIds() {
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemByTypeResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemByTypeResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemByTypeResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemByTypeResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByTypeResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemByRelationRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemByRelationRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* optional int32 limit = 2;
*/
int getLimit();
/**
* optional int64 itemId = 3;
*
*
* e.g. 'science_fiction'
*
*/
long getItemId();
/**
* optional int64 relationTypeId = 4;
*
*
* e.g. 'genre'
*
*/
long getRelationTypeId();
/**
* optional int64 relatedItemTypeId = 5;
*
*
* e.g. 'book' or just everything if omitted
*
*/
long getRelatedItemTypeId();
}
/**
* Protobuf type {@code truward.orion.user.GetItemByRelationRequest}
*/
public static final class GetItemByRelationRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemByRelationRequest)
GetItemByRelationRequestOrBuilder {
// Use GetItemByRelationRequest.newBuilder() to construct.
private GetItemByRelationRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemByRelationRequest() {
offsetToken_ = "";
limit_ = 0;
itemId_ = 0L;
relationTypeId_ = 0L;
relatedItemTypeId_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemByRelationRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 16: {
limit_ = input.readInt32();
break;
}
case 24: {
itemId_ = input.readInt64();
break;
}
case 32: {
relationTypeId_ = input.readInt64();
break;
}
case 40: {
relatedItemTypeId_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.Builder.class);
}
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LIMIT_FIELD_NUMBER = 2;
private int limit_;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
public static final int ITEMID_FIELD_NUMBER = 3;
private long itemId_;
/**
* optional int64 itemId = 3;
*
*
* e.g. 'science_fiction'
*
*/
public long getItemId() {
return itemId_;
}
public static final int RELATIONTYPEID_FIELD_NUMBER = 4;
private long relationTypeId_;
/**
* optional int64 relationTypeId = 4;
*
*
* e.g. 'genre'
*
*/
public long getRelationTypeId() {
return relationTypeId_;
}
public static final int RELATEDITEMTYPEID_FIELD_NUMBER = 5;
private long relatedItemTypeId_;
/**
* optional int64 relatedItemTypeId = 5;
*
*
* e.g. 'book' or just everything if omitted
*
*/
public long getRelatedItemTypeId() {
return relatedItemTypeId_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
if (limit_ != 0) {
output.writeInt32(2, limit_);
}
if (itemId_ != 0L) {
output.writeInt64(3, itemId_);
}
if (relationTypeId_ != 0L) {
output.writeInt64(4, relationTypeId_);
}
if (relatedItemTypeId_ != 0L) {
output.writeInt64(5, relatedItemTypeId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
if (limit_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, limit_);
}
if (itemId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, itemId_);
}
if (relationTypeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, relationTypeId_);
}
if (relatedItemTypeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, relatedItemTypeId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemByRelationRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemByRelationRequest)
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
limit_ = 0;
itemId_ = 0L;
relationTypeId_ = 0L;
relatedItemTypeId_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationRequest_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest result = new com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest(this);
result.offsetToken_ = offsetToken_;
result.limit_ = limit_;
result.itemId_ = itemId_;
result.relationTypeId_ = relationTypeId_;
result.relatedItemTypeId_ = relatedItemTypeId_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (other.getLimit() != 0) {
setLimit(other.getLimit());
}
if (other.getItemId() != 0L) {
setItemId(other.getItemId());
}
if (other.getRelationTypeId() != 0L) {
setRelationTypeId(other.getRelationTypeId());
}
if (other.getRelatedItemTypeId() != 0L) {
setRelatedItemTypeId(other.getRelatedItemTypeId());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 2;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 2;
*/
public Builder setLimit(int value) {
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 2;
*/
public Builder clearLimit() {
limit_ = 0;
onChanged();
return this;
}
private long itemId_ ;
/**
* optional int64 itemId = 3;
*
*
* e.g. 'science_fiction'
*
*/
public long getItemId() {
return itemId_;
}
/**
* optional int64 itemId = 3;
*
*
* e.g. 'science_fiction'
*
*/
public Builder setItemId(long value) {
itemId_ = value;
onChanged();
return this;
}
/**
* optional int64 itemId = 3;
*
*
* e.g. 'science_fiction'
*
*/
public Builder clearItemId() {
itemId_ = 0L;
onChanged();
return this;
}
private long relationTypeId_ ;
/**
* optional int64 relationTypeId = 4;
*
*
* e.g. 'genre'
*
*/
public long getRelationTypeId() {
return relationTypeId_;
}
/**
* optional int64 relationTypeId = 4;
*
*
* e.g. 'genre'
*
*/
public Builder setRelationTypeId(long value) {
relationTypeId_ = value;
onChanged();
return this;
}
/**
* optional int64 relationTypeId = 4;
*
*
* e.g. 'genre'
*
*/
public Builder clearRelationTypeId() {
relationTypeId_ = 0L;
onChanged();
return this;
}
private long relatedItemTypeId_ ;
/**
* optional int64 relatedItemTypeId = 5;
*
*
* e.g. 'book' or just everything if omitted
*
*/
public long getRelatedItemTypeId() {
return relatedItemTypeId_;
}
/**
* optional int64 relatedItemTypeId = 5;
*
*
* e.g. 'book' or just everything if omitted
*
*/
public Builder setRelatedItemTypeId(long value) {
relatedItemTypeId_ = value;
onChanged();
return this;
}
/**
* optional int64 relatedItemTypeId = 5;
*
*
* e.g. 'book' or just everything if omitted
*
*/
public Builder clearRelatedItemTypeId() {
relatedItemTypeId_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemByRelationRequest)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemByRelationRequest)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemByRelationRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemByRelationRequest(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetItemByRelationResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:truward.orion.user.GetItemByRelationResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional string offsetToken = 1;
*/
java.lang.String getOffsetToken();
/**
* optional string offsetToken = 1;
*/
com.google.protobuf.ByteString
getOffsetTokenBytes();
/**
* repeated int64 itemIds = 2;
*/
java.util.List getItemIdsList();
/**
* repeated int64 itemIds = 2;
*/
int getItemIdsCount();
/**
* repeated int64 itemIds = 2;
*/
long getItemIds(int index);
}
/**
* Protobuf type {@code truward.orion.user.GetItemByRelationResponse}
*/
public static final class GetItemByRelationResponse extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:truward.orion.user.GetItemByRelationResponse)
GetItemByRelationResponseOrBuilder {
// Use GetItemByRelationResponse.newBuilder() to construct.
private GetItemByRelationResponse(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private GetItemByRelationResponse() {
offsetToken_ = "";
itemIds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetItemByRelationResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry) {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
offsetToken_ = s;
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
itemIds_.add(input.readInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
itemIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
itemIds_.add(input.readInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.Builder.class);
}
private int bitField0_;
public static final int OFFSETTOKEN_FIELD_NUMBER = 1;
private volatile java.lang.Object offsetToken_;
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEMIDS_FIELD_NUMBER = 2;
private java.util.List itemIds_;
/**
* repeated int64 itemIds = 2;
*/
public java.util.List
getItemIdsList() {
return itemIds_;
}
/**
* repeated int64 itemIds = 2;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 2;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
private int itemIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getOffsetTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, offsetToken_);
}
if (getItemIdsList().size() > 0) {
output.writeRawVarint32(18);
output.writeRawVarint32(itemIdsMemoizedSerializedSize);
}
for (int i = 0; i < itemIds_.size(); i++) {
output.writeInt64NoTag(itemIds_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOffsetTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, offsetToken_);
}
{
int dataSize = 0;
for (int i = 0; i < itemIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(itemIds_.get(i));
}
size += dataSize;
if (!getItemIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
itemIdsMemoizedSerializedSize = dataSize;
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code truward.orion.user.GetItemByRelationResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:truward.orion.user.GetItemByRelationResponse)
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.class, com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.Builder.class);
}
// Construct using com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
offsetToken_ = "";
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.truward.orion.eolaire.model.EolaireModel.internal_static_truward_orion_user_GetItemByRelationResponse_descriptor;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse getDefaultInstanceForType() {
return com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.getDefaultInstance();
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse build() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse buildPartial() {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse result = new com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.offsetToken_ = offsetToken_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = java.util.Collections.unmodifiableList(itemIds_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.itemIds_ = itemIds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse) {
return mergeFrom((com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse other) {
if (other == com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse.getDefaultInstance()) return this;
if (!other.getOffsetToken().isEmpty()) {
offsetToken_ = other.offsetToken_;
onChanged();
}
if (!other.itemIds_.isEmpty()) {
if (itemIds_.isEmpty()) {
itemIds_ = other.itemIds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureItemIdsIsMutable();
itemIds_.addAll(other.itemIds_);
}
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object offsetToken_ = "";
/**
* optional string offsetToken = 1;
*/
public java.lang.String getOffsetToken() {
java.lang.Object ref = offsetToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
offsetToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public com.google.protobuf.ByteString
getOffsetTokenBytes() {
java.lang.Object ref = offsetToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
offsetToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
offsetToken_ = value;
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder clearOffsetToken() {
offsetToken_ = getDefaultInstance().getOffsetToken();
onChanged();
return this;
}
/**
* optional string offsetToken = 1;
*/
public Builder setOffsetTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
offsetToken_ = value;
onChanged();
return this;
}
private java.util.List itemIds_ = java.util.Collections.emptyList();
private void ensureItemIdsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
itemIds_ = new java.util.ArrayList(itemIds_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated int64 itemIds = 2;
*/
public java.util.List
getItemIdsList() {
return java.util.Collections.unmodifiableList(itemIds_);
}
/**
* repeated int64 itemIds = 2;
*/
public int getItemIdsCount() {
return itemIds_.size();
}
/**
* repeated int64 itemIds = 2;
*/
public long getItemIds(int index) {
return itemIds_.get(index);
}
/**
* repeated int64 itemIds = 2;
*/
public Builder setItemIds(
int index, long value) {
ensureItemIdsIsMutable();
itemIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder addItemIds(long value) {
ensureItemIdsIsMutable();
itemIds_.add(value);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder addAllItemIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureItemIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, itemIds_);
onChanged();
return this;
}
/**
* repeated int64 itemIds = 2;
*/
public Builder clearItemIds() {
itemIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:truward.orion.user.GetItemByRelationResponse)
}
// @@protoc_insertion_point(class_scope:truward.orion.user.GetItemByRelationResponse)
private static final com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse();
}
public static com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetItemByRelationResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
try {
return new GetItemByRelationResponse(input, extensionRegistry);
} catch (RuntimeException e) {
if (e.getCause() instanceof
com.google.protobuf.InvalidProtocolBufferException) {
throw (com.google.protobuf.InvalidProtocolBufferException)
e.getCause();
}
throw e;
}
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.truward.orion.eolaire.model.EolaireModel.GetItemByRelationResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_EntityType_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_EntityType_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_Item_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_Item_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_ItemRelation_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_ItemRelation_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_ItemGraph_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_ItemGraph_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_VariantValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_VariantValue_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_MetadataEntry_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_MetadataEntry_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_Metadata_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_Metadata_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_ItemProfile_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_ItemProfile_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemsRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemsRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemsResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemsResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemProfileResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemProfileResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetEntityTypeResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetEntityTypeResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetAllEntityTypesRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetAllEntityTypesResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemByTypeRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemByTypeRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemByTypeResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemByTypeResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemByRelationRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemByRelationRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_truward_orion_user_GetItemByRelationResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_truward_orion_user_GetItemByRelationResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\reolaire.proto\022\022truward.orion.user\"&\n\nE" +
"ntityType\022\n\n\002id\030\001 \001(\003\022\014\n\004name\030\002 \001(\t\"4\n\004I" +
"tem\022\n\n\002id\030\001 \001(\003\022\014\n\004name\030\002 \001(\t\022\022\n\nitemTyp" +
"eId\030\003 \001(\003\"l\n\014ItemRelation\022\024\n\014targetItemI" +
"d\030\001 \001(\003\022\026\n\016relationTypeId\030\002 \001(\003\022.\n\010metad" +
"ata\030\003 \001(\0132\034.truward.orion.user.Metadata\"" +
"h\n\tItemGraph\022&\n\004item\030\001 \001(\0132\030.truward.ori" +
"on.user.Item\0223\n\trelations\030\002 \001(\0132 .truwar" +
"d.orion.user.ItemRelation\"\202\001\n\014VariantVal" +
"ue\022\020\n\010intValue\030\001 \001(\005\022\021\n\tlongValue\030\002 \001(\003\022",
"\022\n\nfloatValue\030\003 \001(\002\022\023\n\013doubleValue\030\004 \001(\001" +
"\022\020\n\010strValue\030\005 \001(\t\022\022\n\nbytesValue\030\007 \001(\014\"|" +
"\n\rMetadataEntry\022\013\n\003key\030\001 \001(\t\022-\n\004type\030\002 \001" +
"(\0162\037.truward.orion.user.VariantType\022/\n\005v" +
"alue\030\003 \001(\0132 .truward.orion.user.VariantV" +
"alue\">\n\010Metadata\0222\n\007entries\030\001 \001(\0132!.truw" +
"ard.orion.user.MetadataEntry\"\223\001\n\013ItemPro" +
"file\022\016\n\006itemId\030\001 \001(\003\022\023\n\013description\030\002 \001(" +
"\t\022\017\n\007created\030\003 \001(\003\022\017\n\007updated\030\004 \001(\003\022\r\n\005f" +
"lags\030\005 \001(\003\022.\n\010metadata\030\006 \001(\0132\034.truward.o",
"rion.user.Metadata\"\"\n\017GetItemsRequest\022\017\n" +
"\007itemIds\030\001 \003(\003\";\n\020GetItemsResponse\022\'\n\005it" +
"ems\030\002 \003(\0132\030.truward.orion.user.Item\"J\n\026G" +
"etItemProfileResponse\0220\n\007profile\030\001 \001(\0132\037" +
".truward.orion.user.ItemProfile\"E\n\025GetEn" +
"tityTypeResponse\022,\n\004type\030\002 \001(\0132\036.truward" +
".orion.user.EntityType\">\n\030GetAllEntityTy" +
"pesRequest\022\023\n\013offsetToken\030\001 \001(\t\022\r\n\005limit" +
"\030\002 \001(\005\"_\n\031GetAllEntityTypesResponse\022\023\n\013o" +
"ffsetToken\030\001 \001(\t\022-\n\005types\030\002 \003(\0132\036.truwar",
"d.orion.user.EntityType\"N\n\024GetItemByType" +
"Request\022\023\n\013offsetToken\030\001 \001(\t\022\r\n\005limit\030\002 " +
"\001(\005\022\022\n\nitemTypeId\030\003 \001(\003\"=\n\025GetItemByType" +
"Response\022\023\n\013offsetToken\030\001 \001(\t\022\017\n\007itemIds" +
"\030\002 \003(\003\"\201\001\n\030GetItemByRelationRequest\022\023\n\013o" +
"ffsetToken\030\001 \001(\t\022\r\n\005limit\030\002 \001(\005\022\016\n\006itemI" +
"d\030\003 \001(\003\022\026\n\016relationTypeId\030\004 \001(\003\022\031\n\021relat" +
"edItemTypeId\030\005 \001(\003\"A\n\031GetItemByRelationR" +
"esponse\022\023\n\013offsetToken\030\001 \001(\t\022\017\n\007itemIds\030" +
"\002 \003(\003*\273\001\n\013VariantType\022\010\n\004NULL\020\000\022\016\n\nBOOL_",
"FALSE\020\001\022\r\n\tBOOL_TRUE\020\002\022\016\n\nINT32_ZERO\020\003\022\016" +
"\n\nINT64_ZERO\020\004\022\016\n\nFLOAT_ZERO\020\005\022\017\n\013DOUBLE" +
"_ZERO\020\006\022\t\n\005INT32\020\007\022\t\n\005INT64\020\010\022\t\n\005FLOAT\020\t" +
"\022\n\n\006DOUBLE\020\n\022\n\n\006STRING\020\013\022\t\n\005BYTES\020\014B/\n\037c" +
"om.truward.orion.eolaire.modelB\014EolaireM" +
"odelb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_truward_orion_user_EntityType_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_truward_orion_user_EntityType_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_EntityType_descriptor,
new java.lang.String[] { "Id", "Name", });
internal_static_truward_orion_user_Item_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_truward_orion_user_Item_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_Item_descriptor,
new java.lang.String[] { "Id", "Name", "ItemTypeId", });
internal_static_truward_orion_user_ItemRelation_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_truward_orion_user_ItemRelation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_ItemRelation_descriptor,
new java.lang.String[] { "TargetItemId", "RelationTypeId", "Metadata", });
internal_static_truward_orion_user_ItemGraph_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_truward_orion_user_ItemGraph_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_ItemGraph_descriptor,
new java.lang.String[] { "Item", "Relations", });
internal_static_truward_orion_user_VariantValue_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_truward_orion_user_VariantValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_VariantValue_descriptor,
new java.lang.String[] { "IntValue", "LongValue", "FloatValue", "DoubleValue", "StrValue", "BytesValue", });
internal_static_truward_orion_user_MetadataEntry_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_truward_orion_user_MetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_MetadataEntry_descriptor,
new java.lang.String[] { "Key", "Type", "Value", });
internal_static_truward_orion_user_Metadata_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_truward_orion_user_Metadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_Metadata_descriptor,
new java.lang.String[] { "Entries", });
internal_static_truward_orion_user_ItemProfile_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_truward_orion_user_ItemProfile_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_ItemProfile_descriptor,
new java.lang.String[] { "ItemId", "Description", "Created", "Updated", "Flags", "Metadata", });
internal_static_truward_orion_user_GetItemsRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_truward_orion_user_GetItemsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemsRequest_descriptor,
new java.lang.String[] { "ItemIds", });
internal_static_truward_orion_user_GetItemsResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_truward_orion_user_GetItemsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemsResponse_descriptor,
new java.lang.String[] { "Items", });
internal_static_truward_orion_user_GetItemProfileResponse_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_truward_orion_user_GetItemProfileResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemProfileResponse_descriptor,
new java.lang.String[] { "Profile", });
internal_static_truward_orion_user_GetEntityTypeResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_truward_orion_user_GetEntityTypeResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetEntityTypeResponse_descriptor,
new java.lang.String[] { "Type", });
internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_truward_orion_user_GetAllEntityTypesRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetAllEntityTypesRequest_descriptor,
new java.lang.String[] { "OffsetToken", "Limit", });
internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_truward_orion_user_GetAllEntityTypesResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetAllEntityTypesResponse_descriptor,
new java.lang.String[] { "OffsetToken", "Types", });
internal_static_truward_orion_user_GetItemByTypeRequest_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_truward_orion_user_GetItemByTypeRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemByTypeRequest_descriptor,
new java.lang.String[] { "OffsetToken", "Limit", "ItemTypeId", });
internal_static_truward_orion_user_GetItemByTypeResponse_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_truward_orion_user_GetItemByTypeResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemByTypeResponse_descriptor,
new java.lang.String[] { "OffsetToken", "ItemIds", });
internal_static_truward_orion_user_GetItemByRelationRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_truward_orion_user_GetItemByRelationRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemByRelationRequest_descriptor,
new java.lang.String[] { "OffsetToken", "Limit", "ItemId", "RelationTypeId", "RelatedItemTypeId", });
internal_static_truward_orion_user_GetItemByRelationResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_truward_orion_user_GetItemByRelationResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_truward_orion_user_GetItemByRelationResponse_descriptor,
new java.lang.String[] { "OffsetToken", "ItemIds", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy