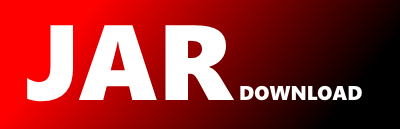
com.tryfinch.api.models.SandboxConnectionAccountCreateParams.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finch-java-core Show documentation
Show all versions of finch-java-core Show documentation
The Finch HRIS API provides a unified way to connect to a multitide of HRIS
systems. The API requires an access token issued by Finch.
By default, Organization and Payroll requests use Finch's
[Data Syncs](/developer-resources/Data-Syncs). If a request is made before the
initial sync has completed, Finch will request data live from the provider. The
latency on live requests may range from seconds to minutes depending on the
provider and batch size. For automated integrations, Deductions requests (both
read and write) are always made live to the provider. Latencies may range from
seconds to minutes depending on the provider and batch size.
Employer products are specified by the product parameter, a space-separated list
of products that your application requests from an employer authenticating
through Finch Connect. Valid product names are—
- `company`: Read basic company data
- `directory`: Read company directory and organization structure
- `individual`: Read individual data, excluding income and employment data
- `employment`: Read individual employment and income data
- `payment`: Read payroll and contractor related payments by the company
- `pay_statement`: Read detailed pay statements for each individual
- `benefits`: Create and manage deductions and contributions and enrollment for
an employer
[](https://god.gw.postman.com/run-collection/21027137-08db0929-883d-4094-a9ce-dbf5a9bee4a4?action=collection%2Ffork&collection-url=entityId%3D21027137-08db0929-883d-4094-a9ce-dbf5a9bee4a4%26entityType%3Dcollection%26workspaceId%3D1edf19bc-e0a8-41e9-ac55-481a4b50790b)
// File generated from our OpenAPI spec by Stainless.
package com.tryfinch.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonCreator
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.tryfinch.api.core.Enum
import com.tryfinch.api.core.ExcludeMissing
import com.tryfinch.api.core.JsonField
import com.tryfinch.api.core.JsonValue
import com.tryfinch.api.core.NoAutoDetect
import com.tryfinch.api.core.toUnmodifiable
import com.tryfinch.api.errors.FinchInvalidDataException
import com.tryfinch.api.models.*
import java.util.Objects
import java.util.Optional
class SandboxConnectionAccountCreateParams
constructor(
private val companyId: String,
private val providerId: String,
private val authenticationType: AuthenticationType?,
private val products: List?,
private val additionalQueryParams: Map>,
private val additionalHeaders: Map>,
private val additionalBodyProperties: Map,
) {
fun companyId(): String = companyId
fun providerId(): String = providerId
fun authenticationType(): Optional = Optional.ofNullable(authenticationType)
fun products(): Optional> = Optional.ofNullable(products)
@JvmSynthetic
internal fun getBody(): SandboxConnectionAccountCreateBody {
return SandboxConnectionAccountCreateBody(
companyId,
providerId,
authenticationType,
products,
additionalBodyProperties,
)
}
@JvmSynthetic internal fun getQueryParams(): Map> = additionalQueryParams
@JvmSynthetic internal fun getHeaders(): Map> = additionalHeaders
@JsonDeserialize(builder = SandboxConnectionAccountCreateBody.Builder::class)
@NoAutoDetect
class SandboxConnectionAccountCreateBody
internal constructor(
private val companyId: String?,
private val providerId: String?,
private val authenticationType: AuthenticationType?,
private val products: List?,
private val additionalProperties: Map,
) {
private var hashCode: Int = 0
@JsonProperty("company_id") fun companyId(): String? = companyId
@JsonProperty("provider_id") fun providerId(): String? = providerId
@JsonProperty("authentication_type")
fun authenticationType(): AuthenticationType? = authenticationType
/**
* Optional, defaults to Organization products (`company`, `directory`, `employment`,
* `individual`)
*/
@JsonProperty("products") fun products(): List? = products
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is SandboxConnectionAccountCreateBody &&
this.companyId == other.companyId &&
this.providerId == other.providerId &&
this.authenticationType == other.authenticationType &&
this.products == other.products &&
this.additionalProperties == other.additionalProperties
}
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode =
Objects.hash(
companyId,
providerId,
authenticationType,
products,
additionalProperties,
)
}
return hashCode
}
override fun toString() =
"SandboxConnectionAccountCreateBody{companyId=$companyId, providerId=$providerId, authenticationType=$authenticationType, products=$products, additionalProperties=$additionalProperties}"
companion object {
@JvmStatic fun builder() = Builder()
}
class Builder {
private var companyId: String? = null
private var providerId: String? = null
private var authenticationType: AuthenticationType? = null
private var products: List? = null
private var additionalProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(
sandboxConnectionAccountCreateBody: SandboxConnectionAccountCreateBody
) = apply {
this.companyId = sandboxConnectionAccountCreateBody.companyId
this.providerId = sandboxConnectionAccountCreateBody.providerId
this.authenticationType = sandboxConnectionAccountCreateBody.authenticationType
this.products = sandboxConnectionAccountCreateBody.products
additionalProperties(sandboxConnectionAccountCreateBody.additionalProperties)
}
@JsonProperty("company_id")
fun companyId(companyId: String) = apply { this.companyId = companyId }
@JsonProperty("provider_id")
fun providerId(providerId: String) = apply { this.providerId = providerId }
@JsonProperty("authentication_type")
fun authenticationType(authenticationType: AuthenticationType) = apply {
this.authenticationType = authenticationType
}
/**
* Optional, defaults to Organization products (`company`, `directory`, `employment`,
* `individual`)
*/
@JsonProperty("products")
fun products(products: List) = apply { this.products = products }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): SandboxConnectionAccountCreateBody =
SandboxConnectionAccountCreateBody(
checkNotNull(companyId) { "`companyId` is required but was not set" },
checkNotNull(providerId) { "`providerId` is required but was not set" },
authenticationType,
products?.toUnmodifiable(),
additionalProperties.toUnmodifiable(),
)
}
}
fun _additionalQueryParams(): Map> = additionalQueryParams
fun _additionalHeaders(): Map> = additionalHeaders
fun _additionalBodyProperties(): Map = additionalBodyProperties
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is SandboxConnectionAccountCreateParams &&
this.companyId == other.companyId &&
this.providerId == other.providerId &&
this.authenticationType == other.authenticationType &&
this.products == other.products &&
this.additionalQueryParams == other.additionalQueryParams &&
this.additionalHeaders == other.additionalHeaders &&
this.additionalBodyProperties == other.additionalBodyProperties
}
override fun hashCode(): Int {
return Objects.hash(
companyId,
providerId,
authenticationType,
products,
additionalQueryParams,
additionalHeaders,
additionalBodyProperties,
)
}
override fun toString() =
"SandboxConnectionAccountCreateParams{companyId=$companyId, providerId=$providerId, authenticationType=$authenticationType, products=$products, additionalQueryParams=$additionalQueryParams, additionalHeaders=$additionalHeaders, additionalBodyProperties=$additionalBodyProperties}"
fun toBuilder() = Builder().from(this)
companion object {
@JvmStatic fun builder() = Builder()
}
@NoAutoDetect
class Builder {
private var companyId: String? = null
private var providerId: String? = null
private var authenticationType: AuthenticationType? = null
private var products: MutableList = mutableListOf()
private var additionalQueryParams: MutableMap> = mutableMapOf()
private var additionalHeaders: MutableMap> = mutableMapOf()
private var additionalBodyProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(
sandboxConnectionAccountCreateParams: SandboxConnectionAccountCreateParams
) = apply {
this.companyId = sandboxConnectionAccountCreateParams.companyId
this.providerId = sandboxConnectionAccountCreateParams.providerId
this.authenticationType = sandboxConnectionAccountCreateParams.authenticationType
this.products(sandboxConnectionAccountCreateParams.products ?: listOf())
additionalQueryParams(sandboxConnectionAccountCreateParams.additionalQueryParams)
additionalHeaders(sandboxConnectionAccountCreateParams.additionalHeaders)
additionalBodyProperties(sandboxConnectionAccountCreateParams.additionalBodyProperties)
}
fun companyId(companyId: String) = apply { this.companyId = companyId }
fun providerId(providerId: String) = apply { this.providerId = providerId }
fun authenticationType(authenticationType: AuthenticationType) = apply {
this.authenticationType = authenticationType
}
/**
* Optional, defaults to Organization products (`company`, `directory`, `employment`,
* `individual`)
*/
fun products(products: List) = apply {
this.products.clear()
this.products.addAll(products)
}
/**
* Optional, defaults to Organization products (`company`, `directory`, `employment`,
* `individual`)
*/
fun addProduct(product: String) = apply { this.products.add(product) }
fun additionalQueryParams(additionalQueryParams: Map>) = apply {
this.additionalQueryParams.clear()
putAllQueryParams(additionalQueryParams)
}
fun putQueryParam(name: String, value: String) = apply {
this.additionalQueryParams.getOrPut(name) { mutableListOf() }.add(value)
}
fun putQueryParams(name: String, values: Iterable) = apply {
this.additionalQueryParams.getOrPut(name) { mutableListOf() }.addAll(values)
}
fun putAllQueryParams(additionalQueryParams: Map>) = apply {
additionalQueryParams.forEach(this::putQueryParams)
}
fun removeQueryParam(name: String) = apply {
this.additionalQueryParams.put(name, mutableListOf())
}
fun additionalHeaders(additionalHeaders: Map>) = apply {
this.additionalHeaders.clear()
putAllHeaders(additionalHeaders)
}
fun putHeader(name: String, value: String) = apply {
this.additionalHeaders.getOrPut(name) { mutableListOf() }.add(value)
}
fun putHeaders(name: String, values: Iterable) = apply {
this.additionalHeaders.getOrPut(name) { mutableListOf() }.addAll(values)
}
fun putAllHeaders(additionalHeaders: Map>) = apply {
additionalHeaders.forEach(this::putHeaders)
}
fun removeHeader(name: String) = apply { this.additionalHeaders.put(name, mutableListOf()) }
fun additionalBodyProperties(additionalBodyProperties: Map) = apply {
this.additionalBodyProperties.clear()
this.additionalBodyProperties.putAll(additionalBodyProperties)
}
fun putAdditionalBodyProperty(key: String, value: JsonValue) = apply {
this.additionalBodyProperties.put(key, value)
}
fun putAllAdditionalBodyProperties(additionalBodyProperties: Map) =
apply {
this.additionalBodyProperties.putAll(additionalBodyProperties)
}
fun build(): SandboxConnectionAccountCreateParams =
SandboxConnectionAccountCreateParams(
checkNotNull(companyId) { "`companyId` is required but was not set" },
checkNotNull(providerId) { "`providerId` is required but was not set" },
authenticationType,
if (products.size == 0) null else products.toUnmodifiable(),
additionalQueryParams.mapValues { it.value.toUnmodifiable() }.toUnmodifiable(),
additionalHeaders.mapValues { it.value.toUnmodifiable() }.toUnmodifiable(),
additionalBodyProperties.toUnmodifiable(),
)
}
class AuthenticationType
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is AuthenticationType && this.value == other.value
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
@JvmField val CREDENTIAL = AuthenticationType(JsonField.of("credential"))
@JvmField val API_TOKEN = AuthenticationType(JsonField.of("api_token"))
@JvmField val OAUTH = AuthenticationType(JsonField.of("oauth"))
@JvmField val ASSISTED = AuthenticationType(JsonField.of("assisted"))
@JvmStatic fun of(value: String) = AuthenticationType(JsonField.of(value))
}
enum class Known {
CREDENTIAL,
API_TOKEN,
OAUTH,
ASSISTED,
}
enum class Value {
CREDENTIAL,
API_TOKEN,
OAUTH,
ASSISTED,
_UNKNOWN,
}
fun value(): Value =
when (this) {
CREDENTIAL -> Value.CREDENTIAL
API_TOKEN -> Value.API_TOKEN
OAUTH -> Value.OAUTH
ASSISTED -> Value.ASSISTED
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
CREDENTIAL -> Known.CREDENTIAL
API_TOKEN -> Known.API_TOKEN
OAUTH -> Known.OAUTH
ASSISTED -> Known.ASSISTED
else -> throw FinchInvalidDataException("Unknown AuthenticationType: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy