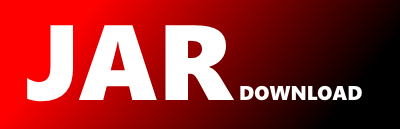
com.tryfinch.api.models.Introspection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finch-java-core Show documentation
Show all versions of finch-java-core Show documentation
The Finch HRIS API provides a unified way to connect to a multitide of HRIS
systems. The API requires an access token issued by Finch.
By default, Organization and Payroll requests use Finch's
[Data Syncs](/developer-resources/Data-Syncs). If a request is made before the
initial sync has completed, Finch will request data live from the provider. The
latency on live requests may range from seconds to minutes depending on the
provider and batch size. For automated integrations, Deductions requests (both
read and write) are always made live to the provider. Latencies may range from
seconds to minutes depending on the provider and batch size.
Employer products are specified by the product parameter, a space-separated list
of products that your application requests from an employer authenticating
through Finch Connect. Valid product names are—
- `company`: Read basic company data
- `directory`: Read company directory and organization structure
- `individual`: Read individual data, excluding income and employment data
- `employment`: Read individual employment and income data
- `payment`: Read payroll and contractor related payments by the company
- `pay_statement`: Read detailed pay statements for each individual
- `benefits`: Create and manage deductions and contributions and enrollment for
an employer
[](https://god.gw.postman.com/run-collection/21027137-08db0929-883d-4094-a9ce-dbf5a9bee4a4?action=collection%2Ffork&collection-url=entityId%3D21027137-08db0929-883d-4094-a9ce-dbf5a9bee4a4%26entityType%3Dcollection%26workspaceId%3D1edf19bc-e0a8-41e9-ac55-481a4b50790b)
// File generated from our OpenAPI spec by Stainless.
package com.tryfinch.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonCreator
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.tryfinch.api.core.Enum
import com.tryfinch.api.core.ExcludeMissing
import com.tryfinch.api.core.JsonField
import com.tryfinch.api.core.JsonMissing
import com.tryfinch.api.core.JsonValue
import com.tryfinch.api.core.NoAutoDetect
import com.tryfinch.api.core.toUnmodifiable
import com.tryfinch.api.errors.FinchInvalidDataException
import java.util.Objects
import java.util.Optional
@JsonDeserialize(builder = Introspection.Builder::class)
@NoAutoDetect
class Introspection
private constructor(
private val connectionId: JsonField,
private val connectionStatus: JsonField,
private val clientId: JsonField,
private val clientType: JsonField,
private val connectionType: JsonField,
private val companyId: JsonField,
private val accountId: JsonField,
private val authenticationMethods: JsonField>,
private val products: JsonField>,
private val username: JsonField,
private val providerId: JsonField,
private val payrollProviderId: JsonField,
private val manual: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
private var hashCode: Int = 0
/** The Finch UUID of the connection associated with the `access_token`. */
fun connectionId(): String = connectionId.getRequired("connection_id")
fun connectionStatus(): ConnectionStatus = connectionStatus.getRequired("connection_status")
/** The client ID of the application associated with the `access_token`. */
fun clientId(): String = clientId.getRequired("client_id")
/** The type of application associated with a token. */
fun clientType(): ClientType = clientType.getRequired("client_type")
/**
* The type of the connection associated with the token.
* - `provider` - connection to an external provider
* - `finch` - finch-generated data.
*/
fun connectionType(): ConnectionType = connectionType.getRequired("connection_type")
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of this
* company ID.
*/
fun companyId(): String = companyId.getRequired("company_id")
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of this
* account ID.
*/
fun accountId(): String = accountId.getRequired("account_id")
fun authenticationMethods(): List =
authenticationMethods.getRequired("authentication_methods")
/** An array of the authorized products associated with the `access_token`. */
fun products(): List = products.getRequired("products")
/** The account username used for login associated with the `access_token`. */
fun username(): String = username.getRequired("username")
/** The ID of the provider associated with the `access_token`. */
fun providerId(): String = providerId.getRequired("provider_id")
/**
* [DEPRECATED] Use `provider_id` to identify the provider instead of this payroll provider ID.
*/
fun payrollProviderId(): String = payrollProviderId.getRequired("payroll_provider_id")
/**
* Whether the connection associated with the `access_token` uses the Assisted Connect Flow.
* (`true` if using Assisted Connect, `false` if connection is automated)
*/
fun manual(): Boolean = manual.getRequired("manual")
/** The Finch UUID of the connection associated with the `access_token`. */
@JsonProperty("connection_id") @ExcludeMissing fun _connectionId() = connectionId
@JsonProperty("connection_status") @ExcludeMissing fun _connectionStatus() = connectionStatus
/** The client ID of the application associated with the `access_token`. */
@JsonProperty("client_id") @ExcludeMissing fun _clientId() = clientId
/** The type of application associated with a token. */
@JsonProperty("client_type") @ExcludeMissing fun _clientType() = clientType
/**
* The type of the connection associated with the token.
* - `provider` - connection to an external provider
* - `finch` - finch-generated data.
*/
@JsonProperty("connection_type") @ExcludeMissing fun _connectionType() = connectionType
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of this
* company ID.
*/
@JsonProperty("company_id") @ExcludeMissing fun _companyId() = companyId
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of this
* account ID.
*/
@JsonProperty("account_id") @ExcludeMissing fun _accountId() = accountId
@JsonProperty("authentication_methods")
@ExcludeMissing
fun _authenticationMethods() = authenticationMethods
/** An array of the authorized products associated with the `access_token`. */
@JsonProperty("products") @ExcludeMissing fun _products() = products
/** The account username used for login associated with the `access_token`. */
@JsonProperty("username") @ExcludeMissing fun _username() = username
/** The ID of the provider associated with the `access_token`. */
@JsonProperty("provider_id") @ExcludeMissing fun _providerId() = providerId
/**
* [DEPRECATED] Use `provider_id` to identify the provider instead of this payroll provider ID.
*/
@JsonProperty("payroll_provider_id")
@ExcludeMissing
fun _payrollProviderId() = payrollProviderId
/**
* Whether the connection associated with the `access_token` uses the Assisted Connect Flow.
* (`true` if using Assisted Connect, `false` if connection is automated)
*/
@JsonProperty("manual") @ExcludeMissing fun _manual() = manual
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Introspection = apply {
if (!validated) {
connectionId()
connectionStatus().validate()
clientId()
clientType()
connectionType()
companyId()
accountId()
authenticationMethods().forEach { it.validate() }
products()
username()
providerId()
payrollProviderId()
manual()
validated = true
}
}
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is Introspection &&
this.connectionId == other.connectionId &&
this.connectionStatus == other.connectionStatus &&
this.clientId == other.clientId &&
this.clientType == other.clientType &&
this.connectionType == other.connectionType &&
this.companyId == other.companyId &&
this.accountId == other.accountId &&
this.authenticationMethods == other.authenticationMethods &&
this.products == other.products &&
this.username == other.username &&
this.providerId == other.providerId &&
this.payrollProviderId == other.payrollProviderId &&
this.manual == other.manual &&
this.additionalProperties == other.additionalProperties
}
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode =
Objects.hash(
connectionId,
connectionStatus,
clientId,
clientType,
connectionType,
companyId,
accountId,
authenticationMethods,
products,
username,
providerId,
payrollProviderId,
manual,
additionalProperties,
)
}
return hashCode
}
override fun toString() =
"Introspection{connectionId=$connectionId, connectionStatus=$connectionStatus, clientId=$clientId, clientType=$clientType, connectionType=$connectionType, companyId=$companyId, accountId=$accountId, authenticationMethods=$authenticationMethods, products=$products, username=$username, providerId=$providerId, payrollProviderId=$payrollProviderId, manual=$manual, additionalProperties=$additionalProperties}"
companion object {
@JvmStatic fun builder() = Builder()
}
class Builder {
private var connectionId: JsonField = JsonMissing.of()
private var connectionStatus: JsonField = JsonMissing.of()
private var clientId: JsonField = JsonMissing.of()
private var clientType: JsonField = JsonMissing.of()
private var connectionType: JsonField = JsonMissing.of()
private var companyId: JsonField = JsonMissing.of()
private var accountId: JsonField = JsonMissing.of()
private var authenticationMethods: JsonField> = JsonMissing.of()
private var products: JsonField> = JsonMissing.of()
private var username: JsonField = JsonMissing.of()
private var providerId: JsonField = JsonMissing.of()
private var payrollProviderId: JsonField = JsonMissing.of()
private var manual: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(introspection: Introspection) = apply {
this.connectionId = introspection.connectionId
this.connectionStatus = introspection.connectionStatus
this.clientId = introspection.clientId
this.clientType = introspection.clientType
this.connectionType = introspection.connectionType
this.companyId = introspection.companyId
this.accountId = introspection.accountId
this.authenticationMethods = introspection.authenticationMethods
this.products = introspection.products
this.username = introspection.username
this.providerId = introspection.providerId
this.payrollProviderId = introspection.payrollProviderId
this.manual = introspection.manual
additionalProperties(introspection.additionalProperties)
}
/** The Finch UUID of the connection associated with the `access_token`. */
fun connectionId(connectionId: String) = connectionId(JsonField.of(connectionId))
/** The Finch UUID of the connection associated with the `access_token`. */
@JsonProperty("connection_id")
@ExcludeMissing
fun connectionId(connectionId: JsonField) = apply {
this.connectionId = connectionId
}
fun connectionStatus(connectionStatus: ConnectionStatus) =
connectionStatus(JsonField.of(connectionStatus))
@JsonProperty("connection_status")
@ExcludeMissing
fun connectionStatus(connectionStatus: JsonField) = apply {
this.connectionStatus = connectionStatus
}
/** The client ID of the application associated with the `access_token`. */
fun clientId(clientId: String) = clientId(JsonField.of(clientId))
/** The client ID of the application associated with the `access_token`. */
@JsonProperty("client_id")
@ExcludeMissing
fun clientId(clientId: JsonField) = apply { this.clientId = clientId }
/** The type of application associated with a token. */
fun clientType(clientType: ClientType) = clientType(JsonField.of(clientType))
/** The type of application associated with a token. */
@JsonProperty("client_type")
@ExcludeMissing
fun clientType(clientType: JsonField) = apply { this.clientType = clientType }
/**
* The type of the connection associated with the token.
* - `provider` - connection to an external provider
* - `finch` - finch-generated data.
*/
fun connectionType(connectionType: ConnectionType) =
connectionType(JsonField.of(connectionType))
/**
* The type of the connection associated with the token.
* - `provider` - connection to an external provider
* - `finch` - finch-generated data.
*/
@JsonProperty("connection_type")
@ExcludeMissing
fun connectionType(connectionType: JsonField) = apply {
this.connectionType = connectionType
}
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of
* this company ID.
*/
fun companyId(companyId: String) = companyId(JsonField.of(companyId))
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of
* this company ID.
*/
@JsonProperty("company_id")
@ExcludeMissing
fun companyId(companyId: JsonField) = apply { this.companyId = companyId }
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of
* this account ID.
*/
fun accountId(accountId: String) = accountId(JsonField.of(accountId))
/**
* [DEPRECATED] Use `connection_id` to associate tokens with a Finch connection instead of
* this account ID.
*/
@JsonProperty("account_id")
@ExcludeMissing
fun accountId(accountId: JsonField) = apply { this.accountId = accountId }
fun authenticationMethods(authenticationMethods: List) =
authenticationMethods(JsonField.of(authenticationMethods))
@JsonProperty("authentication_methods")
@ExcludeMissing
fun authenticationMethods(authenticationMethods: JsonField>) =
apply {
this.authenticationMethods = authenticationMethods
}
/** An array of the authorized products associated with the `access_token`. */
fun products(products: List) = products(JsonField.of(products))
/** An array of the authorized products associated with the `access_token`. */
@JsonProperty("products")
@ExcludeMissing
fun products(products: JsonField>) = apply { this.products = products }
/** The account username used for login associated with the `access_token`. */
fun username(username: String) = username(JsonField.of(username))
/** The account username used for login associated with the `access_token`. */
@JsonProperty("username")
@ExcludeMissing
fun username(username: JsonField) = apply { this.username = username }
/** The ID of the provider associated with the `access_token`. */
fun providerId(providerId: String) = providerId(JsonField.of(providerId))
/** The ID of the provider associated with the `access_token`. */
@JsonProperty("provider_id")
@ExcludeMissing
fun providerId(providerId: JsonField) = apply { this.providerId = providerId }
/**
* [DEPRECATED] Use `provider_id` to identify the provider instead of this payroll provider
* ID.
*/
fun payrollProviderId(payrollProviderId: String) =
payrollProviderId(JsonField.of(payrollProviderId))
/**
* [DEPRECATED] Use `provider_id` to identify the provider instead of this payroll provider
* ID.
*/
@JsonProperty("payroll_provider_id")
@ExcludeMissing
fun payrollProviderId(payrollProviderId: JsonField) = apply {
this.payrollProviderId = payrollProviderId
}
/**
* Whether the connection associated with the `access_token` uses the Assisted Connect Flow.
* (`true` if using Assisted Connect, `false` if connection is automated)
*/
fun manual(manual: Boolean) = manual(JsonField.of(manual))
/**
* Whether the connection associated with the `access_token` uses the Assisted Connect Flow.
* (`true` if using Assisted Connect, `false` if connection is automated)
*/
@JsonProperty("manual")
@ExcludeMissing
fun manual(manual: JsonField) = apply { this.manual = manual }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Introspection =
Introspection(
connectionId,
connectionStatus,
clientId,
clientType,
connectionType,
companyId,
accountId,
authenticationMethods.map { it.toUnmodifiable() },
products.map { it.toUnmodifiable() },
username,
providerId,
payrollProviderId,
manual,
additionalProperties.toUnmodifiable(),
)
}
@JsonDeserialize(builder = AuthenticationMethod.Builder::class)
@NoAutoDetect
class AuthenticationMethod
private constructor(
private val type: JsonField,
private val connectionStatus: JsonField,
private val products: JsonField>,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
private var hashCode: Int = 0
/** The type of authentication method. */
fun type(): Optional = Optional.ofNullable(type.getNullable("type"))
fun connectionStatus(): Optional =
Optional.ofNullable(connectionStatus.getNullable("connection_status"))
/** An array of the authorized products associated with the `access_token`. */
fun products(): Optional> =
Optional.ofNullable(products.getNullable("products"))
/** The type of authentication method. */
@JsonProperty("type") @ExcludeMissing fun _type() = type
@JsonProperty("connection_status")
@ExcludeMissing
fun _connectionStatus() = connectionStatus
/** An array of the authorized products associated with the `access_token`. */
@JsonProperty("products") @ExcludeMissing fun _products() = products
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): AuthenticationMethod = apply {
if (!validated) {
type()
connectionStatus().map { it.validate() }
products()
validated = true
}
}
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is AuthenticationMethod &&
this.type == other.type &&
this.connectionStatus == other.connectionStatus &&
this.products == other.products &&
this.additionalProperties == other.additionalProperties
}
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode =
Objects.hash(
type,
connectionStatus,
products,
additionalProperties,
)
}
return hashCode
}
override fun toString() =
"AuthenticationMethod{type=$type, connectionStatus=$connectionStatus, products=$products, additionalProperties=$additionalProperties}"
companion object {
@JvmStatic fun builder() = Builder()
}
class Builder {
private var type: JsonField = JsonMissing.of()
private var connectionStatus: JsonField = JsonMissing.of()
private var products: JsonField> = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(authenticationMethod: AuthenticationMethod) = apply {
this.type = authenticationMethod.type
this.connectionStatus = authenticationMethod.connectionStatus
this.products = authenticationMethod.products
additionalProperties(authenticationMethod.additionalProperties)
}
/** The type of authentication method. */
fun type(type: Type) = type(JsonField.of(type))
/** The type of authentication method. */
@JsonProperty("type")
@ExcludeMissing
fun type(type: JsonField) = apply { this.type = type }
fun connectionStatus(connectionStatus: ConnectionStatus) =
connectionStatus(JsonField.of(connectionStatus))
@JsonProperty("connection_status")
@ExcludeMissing
fun connectionStatus(connectionStatus: JsonField) = apply {
this.connectionStatus = connectionStatus
}
/** An array of the authorized products associated with the `access_token`. */
fun products(products: List) = products(JsonField.of(products))
/** An array of the authorized products associated with the `access_token`. */
@JsonProperty("products")
@ExcludeMissing
fun products(products: JsonField>) = apply { this.products = products }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): AuthenticationMethod =
AuthenticationMethod(
type,
connectionStatus,
products.map { it.toUnmodifiable() },
additionalProperties.toUnmodifiable(),
)
}
@JsonDeserialize(builder = ConnectionStatus.Builder::class)
@NoAutoDetect
class ConnectionStatus
private constructor(
private val status: JsonField,
private val message: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
private var hashCode: Int = 0
fun status(): Optional =
Optional.ofNullable(status.getNullable("status"))
fun message(): Optional = Optional.ofNullable(message.getNullable("message"))
@JsonProperty("status") @ExcludeMissing fun _status() = status
@JsonProperty("message") @ExcludeMissing fun _message() = message
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): ConnectionStatus = apply {
if (!validated) {
status()
message()
validated = true
}
}
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is ConnectionStatus &&
this.status == other.status &&
this.message == other.message &&
this.additionalProperties == other.additionalProperties
}
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode =
Objects.hash(
status,
message,
additionalProperties,
)
}
return hashCode
}
override fun toString() =
"ConnectionStatus{status=$status, message=$message, additionalProperties=$additionalProperties}"
companion object {
@JvmStatic fun builder() = Builder()
}
class Builder {
private var status: JsonField = JsonMissing.of()
private var message: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(connectionStatus: ConnectionStatus) = apply {
this.status = connectionStatus.status
this.message = connectionStatus.message
additionalProperties(connectionStatus.additionalProperties)
}
fun status(status: ConnectionStatusType) = status(JsonField.of(status))
@JsonProperty("status")
@ExcludeMissing
fun status(status: JsonField) = apply { this.status = status }
fun message(message: String) = message(JsonField.of(message))
@JsonProperty("message")
@ExcludeMissing
fun message(message: JsonField) = apply { this.message = message }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) =
apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): ConnectionStatus =
ConnectionStatus(
status,
message,
additionalProperties.toUnmodifiable(),
)
}
}
class Type
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is Type && this.value == other.value
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
@JvmField val ASSISTED = Type(JsonField.of("assisted"))
@JvmField val CREDENTIAL = Type(JsonField.of("credential"))
@JvmField val API_TOKEN = Type(JsonField.of("api_token"))
@JvmField val API_CREDENTIAL = Type(JsonField.of("api_credential"))
@JvmField val OAUTH = Type(JsonField.of("oauth"))
@JvmStatic fun of(value: String) = Type(JsonField.of(value))
}
enum class Known {
ASSISTED,
CREDENTIAL,
API_TOKEN,
API_CREDENTIAL,
OAUTH,
}
enum class Value {
ASSISTED,
CREDENTIAL,
API_TOKEN,
API_CREDENTIAL,
OAUTH,
_UNKNOWN,
}
fun value(): Value =
when (this) {
ASSISTED -> Value.ASSISTED
CREDENTIAL -> Value.CREDENTIAL
API_TOKEN -> Value.API_TOKEN
API_CREDENTIAL -> Value.API_CREDENTIAL
OAUTH -> Value.OAUTH
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
ASSISTED -> Known.ASSISTED
CREDENTIAL -> Known.CREDENTIAL
API_TOKEN -> Known.API_TOKEN
API_CREDENTIAL -> Known.API_CREDENTIAL
OAUTH -> Known.OAUTH
else -> throw FinchInvalidDataException("Unknown Type: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
}
class ClientType
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is ClientType && this.value == other.value
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
@JvmField val PRODUCTION = ClientType(JsonField.of("production"))
@JvmField val DEVELOPMENT = ClientType(JsonField.of("development"))
@JvmField val SANDBOX = ClientType(JsonField.of("sandbox"))
@JvmStatic fun of(value: String) = ClientType(JsonField.of(value))
}
enum class Known {
PRODUCTION,
DEVELOPMENT,
SANDBOX,
}
enum class Value {
PRODUCTION,
DEVELOPMENT,
SANDBOX,
_UNKNOWN,
}
fun value(): Value =
when (this) {
PRODUCTION -> Value.PRODUCTION
DEVELOPMENT -> Value.DEVELOPMENT
SANDBOX -> Value.SANDBOX
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
PRODUCTION -> Known.PRODUCTION
DEVELOPMENT -> Known.DEVELOPMENT
SANDBOX -> Known.SANDBOX
else -> throw FinchInvalidDataException("Unknown ClientType: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
@JsonDeserialize(builder = ConnectionStatus.Builder::class)
@NoAutoDetect
class ConnectionStatus
private constructor(
private val status: JsonField,
private val message: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
private var hashCode: Int = 0
fun status(): Optional =
Optional.ofNullable(status.getNullable("status"))
fun message(): Optional = Optional.ofNullable(message.getNullable("message"))
@JsonProperty("status") @ExcludeMissing fun _status() = status
@JsonProperty("message") @ExcludeMissing fun _message() = message
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): ConnectionStatus = apply {
if (!validated) {
status()
message()
validated = true
}
}
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is ConnectionStatus &&
this.status == other.status &&
this.message == other.message &&
this.additionalProperties == other.additionalProperties
}
override fun hashCode(): Int {
if (hashCode == 0) {
hashCode =
Objects.hash(
status,
message,
additionalProperties,
)
}
return hashCode
}
override fun toString() =
"ConnectionStatus{status=$status, message=$message, additionalProperties=$additionalProperties}"
companion object {
@JvmStatic fun builder() = Builder()
}
class Builder {
private var status: JsonField = JsonMissing.of()
private var message: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
@JvmSynthetic
internal fun from(connectionStatus: ConnectionStatus) = apply {
this.status = connectionStatus.status
this.message = connectionStatus.message
additionalProperties(connectionStatus.additionalProperties)
}
fun status(status: ConnectionStatusType) = status(JsonField.of(status))
@JsonProperty("status")
@ExcludeMissing
fun status(status: JsonField) = apply { this.status = status }
fun message(message: String) = message(JsonField.of(message))
@JsonProperty("message")
@ExcludeMissing
fun message(message: JsonField) = apply { this.message = message }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): ConnectionStatus =
ConnectionStatus(
status,
message,
additionalProperties.toUnmodifiable(),
)
}
}
class ConnectionType
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return other is ConnectionType && this.value == other.value
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
@JvmField val PROVIDER = ConnectionType(JsonField.of("provider"))
@JvmField val FINCH = ConnectionType(JsonField.of("finch"))
@JvmStatic fun of(value: String) = ConnectionType(JsonField.of(value))
}
enum class Known {
PROVIDER,
FINCH,
}
enum class Value {
PROVIDER,
FINCH,
_UNKNOWN,
}
fun value(): Value =
when (this) {
PROVIDER -> Value.PROVIDER
FINCH -> Value.FINCH
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
PROVIDER -> Known.PROVIDER
FINCH -> Known.FINCH
else -> throw FinchInvalidDataException("Unknown ConnectionType: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy