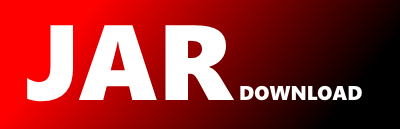
com.tryfinch.api.models.HrisBenefitIndividualRetrieveManyBenefitsPageAsync.kt Maven / Gradle / Ivy
// File generated from our OpenAPI spec by Stainless.
package com.tryfinch.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.tryfinch.api.core.ExcludeMissing
import com.tryfinch.api.core.JsonField
import com.tryfinch.api.core.JsonMissing
import com.tryfinch.api.core.JsonValue
import com.tryfinch.api.core.NoAutoDetect
import com.tryfinch.api.core.toImmutable
import com.tryfinch.api.services.async.hris.benefits.IndividualServiceAsync
import java.util.Objects
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.FlowCollector
class HrisBenefitIndividualRetrieveManyBenefitsPageAsync
private constructor(
private val individualsService: IndividualServiceAsync,
private val params: HrisBenefitIndividualRetrieveManyBenefitsParams,
private val response: Response,
) {
fun response(): Response = response
fun items(): List = response().items()
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is HrisBenefitIndividualRetrieveManyBenefitsPageAsync && individualsService == other.individualsService && params == other.params && response == other.response /* spotless:on */
}
override fun hashCode(): Int = /* spotless:off */ Objects.hash(individualsService, params, response) /* spotless:on */
override fun toString() =
"HrisBenefitIndividualRetrieveManyBenefitsPageAsync{individualsService=$individualsService, params=$params, response=$response}"
fun hasNextPage(): Boolean {
return !items().isEmpty()
}
fun getNextPageParams(): HrisBenefitIndividualRetrieveManyBenefitsParams? {
return null
}
suspend fun getNextPage(): HrisBenefitIndividualRetrieveManyBenefitsPageAsync? {
return getNextPageParams()?.let { individualsService.retrieveManyBenefits(it) }
}
fun autoPager(): AutoPager = AutoPager(this)
companion object {
fun of(
individualsService: IndividualServiceAsync,
params: HrisBenefitIndividualRetrieveManyBenefitsParams,
response: Response
) =
HrisBenefitIndividualRetrieveManyBenefitsPageAsync(
individualsService,
params,
response,
)
}
@JsonDeserialize(builder = Response.Builder::class)
@NoAutoDetect
class Response
constructor(
private val items: JsonField>,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
fun items(): List = items.getNullable("items") ?: listOf()
@JsonProperty("items") fun _items(): JsonField>? = items
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Response = apply {
if (!validated) {
items().map { it.validate() }
validated = true
}
}
fun toBuilder() = Builder().from(this)
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Response && items == other.items && additionalProperties == other.additionalProperties /* spotless:on */
}
override fun hashCode(): Int = /* spotless:off */ Objects.hash(items, additionalProperties) /* spotless:on */
override fun toString() =
"Response{items=$items, additionalProperties=$additionalProperties}"
companion object {
fun builder() = Builder()
}
class Builder {
private var items: JsonField> = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(page: Response) = apply {
this.items = page.items
this.additionalProperties.putAll(page.additionalProperties)
}
fun items(items: List) = items(JsonField.of(items))
@JsonProperty("items")
fun items(items: JsonField>) = apply { this.items = items }
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun build() = Response(items, additionalProperties.toImmutable())
}
}
class AutoPager
constructor(
private val firstPage: HrisBenefitIndividualRetrieveManyBenefitsPageAsync,
) : Flow {
override suspend fun collect(collector: FlowCollector) {
var page = firstPage
var index = 0
while (true) {
while (index < page.items().size) {
collector.emit(page.items()[index++])
}
page = page.getNextPage() ?: break
index = 0
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy