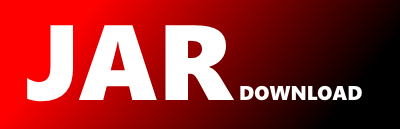
com.tryfinch.api.models.IndividualBenefit.kt Maven / Gradle / Ivy
// File generated from our OpenAPI spec by Stainless.
package com.tryfinch.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonCreator
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.tryfinch.api.core.Enum
import com.tryfinch.api.core.ExcludeMissing
import com.tryfinch.api.core.JsonField
import com.tryfinch.api.core.JsonMissing
import com.tryfinch.api.core.JsonValue
import com.tryfinch.api.core.NoAutoDetect
import com.tryfinch.api.core.toImmutable
import com.tryfinch.api.errors.FinchInvalidDataException
import java.util.Objects
@JsonDeserialize(builder = IndividualBenefit.Builder::class)
@NoAutoDetect
class IndividualBenefit
private constructor(
private val individualId: JsonField,
private val code: JsonField,
private val body: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
fun individualId(): String? = individualId.getNullable("individual_id")
fun code(): Long? = code.getNullable("code")
fun body(): Body? = body.getNullable("body")
@JsonProperty("individual_id") @ExcludeMissing fun _individualId() = individualId
@JsonProperty("code") @ExcludeMissing fun _code() = code
@JsonProperty("body") @ExcludeMissing fun _body() = body
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): IndividualBenefit = apply {
if (!validated) {
individualId()
code()
body()?.validate()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var individualId: JsonField = JsonMissing.of()
private var code: JsonField = JsonMissing.of()
private var body: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(individualBenefit: IndividualBenefit) = apply {
this.individualId = individualBenefit.individualId
this.code = individualBenefit.code
this.body = individualBenefit.body
additionalProperties(individualBenefit.additionalProperties)
}
fun individualId(individualId: String) = individualId(JsonField.of(individualId))
@JsonProperty("individual_id")
@ExcludeMissing
fun individualId(individualId: JsonField) = apply {
this.individualId = individualId
}
fun code(code: Long) = code(JsonField.of(code))
@JsonProperty("code")
@ExcludeMissing
fun code(code: JsonField) = apply { this.code = code }
fun body(body: Body) = body(JsonField.of(body))
@JsonProperty("body")
@ExcludeMissing
fun body(body: JsonField) = apply { this.body = body }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): IndividualBenefit =
IndividualBenefit(
individualId,
code,
body,
additionalProperties.toImmutable(),
)
}
@JsonDeserialize(builder = Body.Builder::class)
@NoAutoDetect
class Body
private constructor(
private val employeeDeduction: JsonField,
private val companyContribution: JsonField,
private val annualMaximum: JsonField,
private val catchUp: JsonField,
private val hsaContributionLimit: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
fun employeeDeduction(): BenefitContribution? =
employeeDeduction.getNullable("employee_deduction")
fun companyContribution(): BenefitContribution? =
companyContribution.getNullable("company_contribution")
/** If the benefit supports annual maximum, the amount in cents for this individual. */
fun annualMaximum(): Long? = annualMaximum.getNullable("annual_maximum")
/**
* If the benefit supports catch up (401k, 403b, etc.), whether catch up is enabled for this
* individual.
*/
fun catchUp(): Boolean? = catchUp.getNullable("catch_up")
/** Type for HSA contribution limit if the benefit is a HSA. */
fun hsaContributionLimit(): HsaContributionLimit? =
hsaContributionLimit.getNullable("hsa_contribution_limit")
@JsonProperty("employee_deduction")
@ExcludeMissing
fun _employeeDeduction() = employeeDeduction
@JsonProperty("company_contribution")
@ExcludeMissing
fun _companyContribution() = companyContribution
/** If the benefit supports annual maximum, the amount in cents for this individual. */
@JsonProperty("annual_maximum") @ExcludeMissing fun _annualMaximum() = annualMaximum
/**
* If the benefit supports catch up (401k, 403b, etc.), whether catch up is enabled for this
* individual.
*/
@JsonProperty("catch_up") @ExcludeMissing fun _catchUp() = catchUp
/** Type for HSA contribution limit if the benefit is a HSA. */
@JsonProperty("hsa_contribution_limit")
@ExcludeMissing
fun _hsaContributionLimit() = hsaContributionLimit
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Body = apply {
if (!validated) {
employeeDeduction()?.validate()
companyContribution()?.validate()
annualMaximum()
catchUp()
hsaContributionLimit()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var employeeDeduction: JsonField = JsonMissing.of()
private var companyContribution: JsonField = JsonMissing.of()
private var annualMaximum: JsonField = JsonMissing.of()
private var catchUp: JsonField = JsonMissing.of()
private var hsaContributionLimit: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(body: Body) = apply {
this.employeeDeduction = body.employeeDeduction
this.companyContribution = body.companyContribution
this.annualMaximum = body.annualMaximum
this.catchUp = body.catchUp
this.hsaContributionLimit = body.hsaContributionLimit
additionalProperties(body.additionalProperties)
}
fun employeeDeduction(employeeDeduction: BenefitContribution) =
employeeDeduction(JsonField.of(employeeDeduction))
@JsonProperty("employee_deduction")
@ExcludeMissing
fun employeeDeduction(employeeDeduction: JsonField) = apply {
this.employeeDeduction = employeeDeduction
}
fun companyContribution(companyContribution: BenefitContribution) =
companyContribution(JsonField.of(companyContribution))
@JsonProperty("company_contribution")
@ExcludeMissing
fun companyContribution(companyContribution: JsonField) = apply {
this.companyContribution = companyContribution
}
/** If the benefit supports annual maximum, the amount in cents for this individual. */
fun annualMaximum(annualMaximum: Long) = annualMaximum(JsonField.of(annualMaximum))
/** If the benefit supports annual maximum, the amount in cents for this individual. */
@JsonProperty("annual_maximum")
@ExcludeMissing
fun annualMaximum(annualMaximum: JsonField) = apply {
this.annualMaximum = annualMaximum
}
/**
* If the benefit supports catch up (401k, 403b, etc.), whether catch up is enabled for
* this individual.
*/
fun catchUp(catchUp: Boolean) = catchUp(JsonField.of(catchUp))
/**
* If the benefit supports catch up (401k, 403b, etc.), whether catch up is enabled for
* this individual.
*/
@JsonProperty("catch_up")
@ExcludeMissing
fun catchUp(catchUp: JsonField) = apply { this.catchUp = catchUp }
/** Type for HSA contribution limit if the benefit is a HSA. */
fun hsaContributionLimit(hsaContributionLimit: HsaContributionLimit) =
hsaContributionLimit(JsonField.of(hsaContributionLimit))
/** Type for HSA contribution limit if the benefit is a HSA. */
@JsonProperty("hsa_contribution_limit")
@ExcludeMissing
fun hsaContributionLimit(hsaContributionLimit: JsonField) =
apply {
this.hsaContributionLimit = hsaContributionLimit
}
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Body =
Body(
employeeDeduction,
companyContribution,
annualMaximum,
catchUp,
hsaContributionLimit,
additionalProperties.toImmutable(),
)
}
class HsaContributionLimit
@JsonCreator
private constructor(
private val value: JsonField,
) : Enum {
@com.fasterxml.jackson.annotation.JsonValue fun _value(): JsonField = value
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is HsaContributionLimit && value == other.value /* spotless:on */
}
override fun hashCode() = value.hashCode()
override fun toString() = value.toString()
companion object {
val INDIVIDUAL = HsaContributionLimit(JsonField.of("individual"))
val FAMILY = HsaContributionLimit(JsonField.of("family"))
fun of(value: String) = HsaContributionLimit(JsonField.of(value))
}
enum class Known {
INDIVIDUAL,
FAMILY,
}
enum class Value {
INDIVIDUAL,
FAMILY,
_UNKNOWN,
}
fun value(): Value =
when (this) {
INDIVIDUAL -> Value.INDIVIDUAL
FAMILY -> Value.FAMILY
else -> Value._UNKNOWN
}
fun known(): Known =
when (this) {
INDIVIDUAL -> Known.INDIVIDUAL
FAMILY -> Known.FAMILY
else -> throw FinchInvalidDataException("Unknown HsaContributionLimit: $value")
}
fun asString(): String = _value().asStringOrThrow()
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Body && employeeDeduction == other.employeeDeduction && companyContribution == other.companyContribution && annualMaximum == other.annualMaximum && catchUp == other.catchUp && hsaContributionLimit == other.hsaContributionLimit && additionalProperties == other.additionalProperties /* spotless:on */
}
/* spotless:off */
private val hashCode: Int by lazy { Objects.hash(employeeDeduction, companyContribution, annualMaximum, catchUp, hsaContributionLimit, additionalProperties) }
/* spotless:on */
override fun hashCode(): Int = hashCode
override fun toString() =
"Body{employeeDeduction=$employeeDeduction, companyContribution=$companyContribution, annualMaximum=$annualMaximum, catchUp=$catchUp, hsaContributionLimit=$hsaContributionLimit, additionalProperties=$additionalProperties}"
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is IndividualBenefit && individualId == other.individualId && code == other.code && body == other.body && additionalProperties == other.additionalProperties /* spotless:on */
}
/* spotless:off */
private val hashCode: Int by lazy { Objects.hash(individualId, code, body, additionalProperties) }
/* spotless:on */
override fun hashCode(): Int = hashCode
override fun toString() =
"IndividualBenefit{individualId=$individualId, code=$code, body=$body, additionalProperties=$additionalProperties}"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy