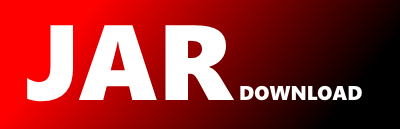
com.tryfinch.api.models.IndividualInDirectory.kt Maven / Gradle / Ivy
// File generated from our OpenAPI spec by Stainless.
package com.tryfinch.api.models
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonAnySetter
import com.fasterxml.jackson.annotation.JsonProperty
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.tryfinch.api.core.ExcludeMissing
import com.tryfinch.api.core.JsonField
import com.tryfinch.api.core.JsonMissing
import com.tryfinch.api.core.JsonValue
import com.tryfinch.api.core.NoAutoDetect
import com.tryfinch.api.core.toImmutable
import java.util.Objects
@JsonDeserialize(builder = IndividualInDirectory.Builder::class)
@NoAutoDetect
class IndividualInDirectory
private constructor(
private val id: JsonField,
private val firstName: JsonField,
private val middleName: JsonField,
private val lastName: JsonField,
private val manager: JsonField,
private val department: JsonField,
private val isActive: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** A stable Finch id (UUID v4) for an individual in the company. */
fun id(): String? = id.getNullable("id")
/** The legal first name of the individual. */
fun firstName(): String? = firstName.getNullable("first_name")
/** The legal middle name of the individual. */
fun middleName(): String? = middleName.getNullable("middle_name")
/** The legal last name of the individual. */
fun lastName(): String? = lastName.getNullable("last_name")
/** The manager object. */
fun manager(): Manager? = manager.getNullable("manager")
/** The department object. */
fun department(): Department? = department.getNullable("department")
/** `true` if the individual is an active employee or contractor at the company. */
fun isActive(): Boolean? = isActive.getNullable("is_active")
/** A stable Finch id (UUID v4) for an individual in the company. */
@JsonProperty("id") @ExcludeMissing fun _id() = id
/** The legal first name of the individual. */
@JsonProperty("first_name") @ExcludeMissing fun _firstName() = firstName
/** The legal middle name of the individual. */
@JsonProperty("middle_name") @ExcludeMissing fun _middleName() = middleName
/** The legal last name of the individual. */
@JsonProperty("last_name") @ExcludeMissing fun _lastName() = lastName
/** The manager object. */
@JsonProperty("manager") @ExcludeMissing fun _manager() = manager
/** The department object. */
@JsonProperty("department") @ExcludeMissing fun _department() = department
/** `true` if the individual is an active employee or contractor at the company. */
@JsonProperty("is_active") @ExcludeMissing fun _isActive() = isActive
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): IndividualInDirectory = apply {
if (!validated) {
id()
firstName()
middleName()
lastName()
manager()?.validate()
department()?.validate()
isActive()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var id: JsonField = JsonMissing.of()
private var firstName: JsonField = JsonMissing.of()
private var middleName: JsonField = JsonMissing.of()
private var lastName: JsonField = JsonMissing.of()
private var manager: JsonField = JsonMissing.of()
private var department: JsonField = JsonMissing.of()
private var isActive: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(individualInDirectory: IndividualInDirectory) = apply {
this.id = individualInDirectory.id
this.firstName = individualInDirectory.firstName
this.middleName = individualInDirectory.middleName
this.lastName = individualInDirectory.lastName
this.manager = individualInDirectory.manager
this.department = individualInDirectory.department
this.isActive = individualInDirectory.isActive
additionalProperties(individualInDirectory.additionalProperties)
}
/** A stable Finch id (UUID v4) for an individual in the company. */
fun id(id: String) = id(JsonField.of(id))
/** A stable Finch id (UUID v4) for an individual in the company. */
@JsonProperty("id") @ExcludeMissing fun id(id: JsonField) = apply { this.id = id }
/** The legal first name of the individual. */
fun firstName(firstName: String) = firstName(JsonField.of(firstName))
/** The legal first name of the individual. */
@JsonProperty("first_name")
@ExcludeMissing
fun firstName(firstName: JsonField) = apply { this.firstName = firstName }
/** The legal middle name of the individual. */
fun middleName(middleName: String) = middleName(JsonField.of(middleName))
/** The legal middle name of the individual. */
@JsonProperty("middle_name")
@ExcludeMissing
fun middleName(middleName: JsonField) = apply { this.middleName = middleName }
/** The legal last name of the individual. */
fun lastName(lastName: String) = lastName(JsonField.of(lastName))
/** The legal last name of the individual. */
@JsonProperty("last_name")
@ExcludeMissing
fun lastName(lastName: JsonField) = apply { this.lastName = lastName }
/** The manager object. */
fun manager(manager: Manager) = manager(JsonField.of(manager))
/** The manager object. */
@JsonProperty("manager")
@ExcludeMissing
fun manager(manager: JsonField) = apply { this.manager = manager }
/** The department object. */
fun department(department: Department) = department(JsonField.of(department))
/** The department object. */
@JsonProperty("department")
@ExcludeMissing
fun department(department: JsonField) = apply { this.department = department }
/** `true` if the individual is an active employee or contractor at the company. */
fun isActive(isActive: Boolean) = isActive(JsonField.of(isActive))
/** `true` if the individual is an active employee or contractor at the company. */
@JsonProperty("is_active")
@ExcludeMissing
fun isActive(isActive: JsonField) = apply { this.isActive = isActive }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): IndividualInDirectory =
IndividualInDirectory(
id,
firstName,
middleName,
lastName,
manager,
department,
isActive,
additionalProperties.toImmutable(),
)
}
/** The department object. */
@JsonDeserialize(builder = Department.Builder::class)
@NoAutoDetect
class Department
private constructor(
private val name: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** The name of the department. */
fun name(): String? = name.getNullable("name")
/** The name of the department. */
@JsonProperty("name") @ExcludeMissing fun _name() = name
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Department = apply {
if (!validated) {
name()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var name: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(department: Department) = apply {
this.name = department.name
additionalProperties(department.additionalProperties)
}
/** The name of the department. */
fun name(name: String) = name(JsonField.of(name))
/** The name of the department. */
@JsonProperty("name")
@ExcludeMissing
fun name(name: JsonField) = apply { this.name = name }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Department = Department(name, additionalProperties.toImmutable())
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Department && name == other.name && additionalProperties == other.additionalProperties /* spotless:on */
}
/* spotless:off */
private val hashCode: Int by lazy { Objects.hash(name, additionalProperties) }
/* spotless:on */
override fun hashCode(): Int = hashCode
override fun toString() =
"Department{name=$name, additionalProperties=$additionalProperties}"
}
/** The manager object. */
@JsonDeserialize(builder = Manager.Builder::class)
@NoAutoDetect
class Manager
private constructor(
private val id: JsonField,
private val additionalProperties: Map,
) {
private var validated: Boolean = false
/** A stable Finch `id` (UUID v4) for an individual in the company. */
fun id(): String? = id.getNullable("id")
/** A stable Finch `id` (UUID v4) for an individual in the company. */
@JsonProperty("id") @ExcludeMissing fun _id() = id
@JsonAnyGetter
@ExcludeMissing
fun _additionalProperties(): Map = additionalProperties
fun validate(): Manager = apply {
if (!validated) {
id()
validated = true
}
}
fun toBuilder() = Builder().from(this)
companion object {
fun builder() = Builder()
}
class Builder {
private var id: JsonField = JsonMissing.of()
private var additionalProperties: MutableMap = mutableMapOf()
internal fun from(manager: Manager) = apply {
this.id = manager.id
additionalProperties(manager.additionalProperties)
}
/** A stable Finch `id` (UUID v4) for an individual in the company. */
fun id(id: String) = id(JsonField.of(id))
/** A stable Finch `id` (UUID v4) for an individual in the company. */
@JsonProperty("id")
@ExcludeMissing
fun id(id: JsonField) = apply { this.id = id }
fun additionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.clear()
this.additionalProperties.putAll(additionalProperties)
}
@JsonAnySetter
fun putAdditionalProperty(key: String, value: JsonValue) = apply {
this.additionalProperties.put(key, value)
}
fun putAllAdditionalProperties(additionalProperties: Map) = apply {
this.additionalProperties.putAll(additionalProperties)
}
fun build(): Manager = Manager(id, additionalProperties.toImmutable())
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is Manager && id == other.id && additionalProperties == other.additionalProperties /* spotless:on */
}
/* spotless:off */
private val hashCode: Int by lazy { Objects.hash(id, additionalProperties) }
/* spotless:on */
override fun hashCode(): Int = hashCode
override fun toString() = "Manager{id=$id, additionalProperties=$additionalProperties}"
}
override fun equals(other: Any?): Boolean {
if (this === other) {
return true
}
return /* spotless:off */ other is IndividualInDirectory && id == other.id && firstName == other.firstName && middleName == other.middleName && lastName == other.lastName && manager == other.manager && department == other.department && isActive == other.isActive && additionalProperties == other.additionalProperties /* spotless:on */
}
/* spotless:off */
private val hashCode: Int by lazy { Objects.hash(id, firstName, middleName, lastName, manager, department, isActive, additionalProperties) }
/* spotless:on */
override fun hashCode(): Int = hashCode
override fun toString() =
"IndividualInDirectory{id=$id, firstName=$firstName, middleName=$middleName, lastName=$lastName, manager=$manager, department=$department, isActive=$isActive, additionalProperties=$additionalProperties}"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy