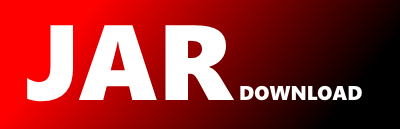
com.tsc9526.monalisa.orm.criteria.Field Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.orm.criteria;
import java.sql.Types;
import java.util.ArrayList;
import java.util.List;
import com.tsc9526.monalisa.orm.Query;
import com.tsc9526.monalisa.orm.datasource.DataSourceManager;
import com.tsc9526.monalisa.tools.clazz.MelpEnum;
import com.tsc9526.monalisa.tools.string.MelpTypes;
/**
*
* @author zzg.zhou([email protected])
*/
@SuppressWarnings({"unchecked"})
public class Field>{
private DataSourceManager dsm=DataSourceManager.getInstance();
protected Y criteria;
protected String name;
protected String columnName;
protected Query q;
protected String type;
public Field(String name,Y criteria){
this(name, criteria, Types.INTEGER);
}
public Field(String name,Y criteria,int jdbcType){
this.name=name;
this.criteria=criteria;
this.q=criteria.q;
this.type=MelpTypes.getJavaType(jdbcType);
}
/**
* SQL: =
*
* @param value the value
* @return this
*/
public Y eq(X value){
return add(" = ?", value);
}
/**
* SQL: <>
*
* @param value the value
* @return this
*/
public Y ne(X value){
return add(" <> ?", value);
}
/**
* SQL: >
*
* @param value the value
* @return this
*/
public Y gt(X value){
return add(" > ?", value);
}
/**
* SQL: >=
*
* @param value the value
* @return this
*/
public Y ge(X value){
return add(" >= ?", value);
}
/**
* SQL: <
*
* @param value the value
* @return this
*/
public Y lt(X value){
return add(" < ?", value);
}
/**
* SQL: <=
*
* @param value the value
* @return this
*/
public Y le(X value){
return add(" <= ?", value);
}
/**
* Example:
* like("%value%"); -> like '%value%'
* like("value%"); -> like 'value%'
* like("%value"); -> like '%value'
*
* @param value the value
* @return this
*/
public Y like(X value){
return add(" like ?", value);
}
/**
* SQL: IS NULL
*
* @return this
*/
public Y isNull(){
return add(" IS NULL");
}
/**
* SQL: IS NOT NULL
*
* @return this
*/
public Y isNotNull(){
return add(" IS NOT NULL");
}
/**
*
* SQL: BETWEEN ? AND ?
*
* @param from >= from
* @param to <= to
* @return this
*/
public Y between(X from,X to){
return add(" BETWEEN ? AND ?", from,to);
}
/**
* SQL: NOT BETWEEN ? AND ?
*
* @param from < from OR
* @param to > to
* @return this
*/
public Y notBetween(X from,X to){
return add(" NOT BETWEEN ? AND ?", from,to);
}
/**
* SQL: IN(...)
*
* @param value the value
* @param values other values
* @return this
*/
public Y in(X value,X... values){
if(isIngore(value)){
return criteria;
}
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).in(getValues(value,values));
return criteria;
}
/**
* SQL: NOT IN (...)
*
* @param value the value
* @param values other values
* @return this
*/
public Y notin(X value,X... values){
if(isIngore(value)){
return criteria;
}
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).notin(getValues(value,values));
return criteria;
}
/**
* SQL: IN(...)=
*
* @param values list values
* @return this
*/
public Y in(List values){
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).in(getValues(values));
return criteria;
}
/**
* SQL: NOT IN (...)
*
* @param values list values
* @return this
*/
public Y notin(List values){
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).notin(getValues(values));
return criteria;
}
/**
* SQL: [ORDER BY] ASC
*
* @return this
*/
public Y asc(){
criteria.addOrderByAsc(getColumnName());
return criteria;
}
/**
* SQL: [ORDER BY] DESC
*
* @return this
*/
public Y desc(){
criteria.addOrderByDesc(getColumnName());
return criteria;
}
private Y add(String op,X value,X... values){
if(isIngore(value)){
return criteria;
}
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).add(op, getValues(value,values));
return criteria;
}
private Y add(String op){
if(q.isEmpty()==false){
q.add(" AND ");
}
q.add(getColumnName()).add(op);
return criteria;
}
private List> getValues(List values){
if(values!=null && values.size()>0 && values.get(0).getClass().isEnum()){
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy