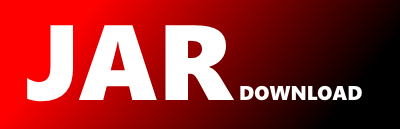
com.tsc9526.monalisa.orm.meta.MetaTable Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.orm.meta;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author zzg.zhou([email protected])
*/
public class MetaTable extends Name implements Cloneable{
private static final long serialVersionUID = 5069827028195702141L;
private List columns=new ArrayList();
private List keyColumns=new ArrayList();
private List indexes=new ArrayList();
private String javaPackage;
private long serialID=0L;
private MetaPartition partition;
private CreateTable createTable;
public MetaTable(){
super(true);
}
public MetaTable(String name){
super(true);
this.setName(name);
}
public void addIndex(MetaIndex index){
indexes.add(index);
}
public MetaIndex getIndex(String indexName){
for(MetaIndex index:indexes){
if(indexName.equalsIgnoreCase(index.getName())){
return index;
}
}
return null;
}
public List getIndexes(){
return this.indexes;
}
public long getSerialID(){
if(serialID==0){
StringBuffer sb=new StringBuffer();
sb.append(name).append("-").append(getJavaName()).append("-").append(getJavaPackage()).append("-").append(": {\r\n");
for(MetaColumn c:columns){
sb.append(c.name).append(c.getJavaType()).append("\r\n");
}
sb.append("}");
serialID=( ((long)sb.length()) << 32 ) + Math.abs(sb.toString().hashCode());
}
return serialID;
}
public List getColumns() {
return columns;
}
public List getKeyColumns() {
return keyColumns;
}
public MetaColumn addColumn(MetaColumn column) {
columns.add(column);
return column;
}
public void addKeyColumn(MetaColumn column) {
keyColumns.add(column);
}
public MetaColumn getColumn(String columnName){
for(MetaColumn c:columns){
if(c.getName().equalsIgnoreCase(columnName)){
return c;
}
}
return null;
}
public String toString(){
StringBuffer sb=new StringBuffer();
sb.append(name).append(": {\r\n");
for(MetaColumn c:columns){
sb.append(c.toString()).append("\r\n");
}
sb.append("}");
return sb.toString();
}
public String getJavaPackage() {
return javaPackage;
}
public void setJavaPackage(String javaPackage) {
this.javaPackage = javaPackage;
}
public MetaPartition getPartition() {
return partition;
}
public void setPartition(MetaPartition partition) {
this.partition = partition;
}
public void setCreateTable(CreateTable createTable) {
this.createTable = createTable;
}
public CreateTable getCreateTable() {
return this.createTable;
}
public String getNamePrefix() {
if(partition!=null){
return partition.getTablePrefix();
}else{
return this.name;
}
}
public MetaTable clone() throws CloneNotSupportedException{
return (MetaTable)super.clone();
}
public static enum TableType{
NORMAL,
PARTITION,
HISTORY,
}
public static class CreateTable implements Serializable{
private static final long serialVersionUID = 4435179643490161535L;
public final static String TABLE_VAR="#{table}";
public final static String FILE_NAME="create_table.sql";
private String tableName;
private String createSQL;
private TableType tableType;
private CreateTable refTable;
public CreateTable (String tableName,String createSQL){
this(tableName, createSQL, TableType.NORMAL);
}
public CreateTable (String tableName,String createSQL,TableType tableType){
this.tableName=tableName;
this.createSQL=createSQL;
this.tableType=tableType;
}
public CreateTable getRefTable(){
return this.refTable;
}
public String getTableName() {
return this.tableName;
}
public TableType getTableType(){
return this.tableType;
}
public String getOriginSQL() {
return createSQL;
}
public String getCreateSQL() {
int p=createSQL.indexOf(TABLE_VAR);
if(p>0){
return createSQL.substring(0,p)+tableName+createSQL.substring(p+TABLE_VAR.length());
}else{
return createSQL;
}
}
public CreateTable setCreateSQL(String createSQL){
this.createSQL=createSQL;
return this;
}
public CreateTable createTable(TableType theTableType,String theTableName){
CreateTable table=new CreateTable(theTableName,createSQL,theTableType);
table.refTable=this;
return table;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy