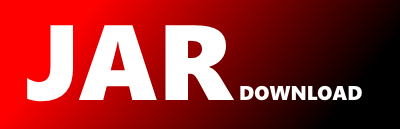
com.tsc9526.monalisa.tools.cache.impl.PerpetualCache Maven / Gradle / Ivy
package com.tsc9526.monalisa.tools.cache.impl;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import com.tsc9526.monalisa.tools.cache.Cache;
public class PerpetualCache implements Cache {
private String id;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy