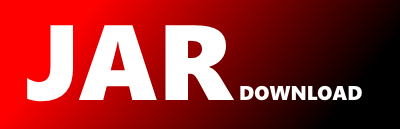
com.tsc9526.monalisa.tools.json.MelpJson Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.tools.json;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.google.gson.ExclusionStrategy;
import com.google.gson.FieldAttributes;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.JsonParser;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.stream.JsonWriter;
import com.tsc9526.monalisa.tools.clazz.MelpClass;
import com.tsc9526.monalisa.tools.clazz.MelpLib;
import com.tsc9526.monalisa.tools.clazz.MelpClass.FGS;
import com.tsc9526.monalisa.tools.clazz.MelpClass.ClassHelper;
import com.tsc9526.monalisa.tools.converters.Conversion;
import com.tsc9526.monalisa.tools.datatable.DataColumn;
import com.tsc9526.monalisa.tools.datatable.DataMap;
import com.tsc9526.monalisa.tools.datatable.DataTable;
import com.tsc9526.monalisa.tools.datatable.Page;
/**
*
* @author zzg.zhou([email protected])
*/
public class MelpJson {
static{
MelpLib.tryLoadGson();
}
private static GsonBuilder gb=createGsonBuilder();
public static GsonBuilder createGsonBuilder(){
return new GsonBuilder()
.registerTypeAdapter(Double.class, new JsonSerializer() {
public JsonElement serialize(Double src, Type typeOfSrc, JsonSerializationContext context) {
if(src == src.longValue()){
return new JsonPrimitive(src.longValue());
}
return new JsonPrimitive(src);
}
})
.setExclusionStrategies(new ExclusionStrategy() {
public boolean shouldSkipField(FieldAttributes fa) {
return fa.getName().startsWith("$");
}
public boolean shouldSkipClass(Class> clazz) {
return false;
}
})
.setDateFormat(Conversion.DEFAULT_DATETIME_FORMAT);
}
public static Gson getGson(){
return gb.create();
}
public static String toJson(Gson gson,Object bean) {
JsonObject json=new JsonObject();
ClassHelper mc=MelpClass.getClassHelper(bean);
for (FGS fgs : mc.getFields()) {
String name=fgs.getFieldName();
Object v = fgs.getObject(bean);
if (v != null) {
JsonElement e=null;
if(v instanceof JsonElement){
e=(JsonElement)v;
}else{
e=gson.toJsonTree(v);
}
json.add(name, e);
}
}
return gson.toJson(json);
}
public static String getString(JsonObject json,String name){
return getString(json, name,null);
}
public static String getString(JsonObject json,String name,String defaultValue){
JsonElement e=json.get(name);
if(e==null || e.isJsonNull()){
return defaultValue;
}else{
return e.getAsString();
}
}
public static int getInt(JsonObject json,String name,int defaultValue){
JsonElement e=json.get(name);
if(e==null || e.isJsonNull()){
return defaultValue;
}else{
return e.getAsInt();
}
}
public static double getDouble(JsonObject json,String name,double defaultValue){
JsonElement e=json.get(name);
if(e==null || e.isJsonNull()){
return defaultValue;
}else{
return e.getAsDouble();
}
}
public static DataTable parseToDataTable(JsonArray array){
DataTable table=new DataTable();
for(int i=0;i parseToPage(JsonObject json){
DataTable rows=parseToDataTable(json.get("rows").getAsJsonArray());
long records=json.get("records").getAsLong();
long size =json.get("size").getAsLong();
long page =json.get("page").getAsLong();
long offset=(page-1)*size;
Page r= new Page(rows,records,size,offset);
return r;
}
public static DataMap parseToDataMap(String json){
JsonElement je=new JsonParser().parse(json);
if(je.isJsonObject()){
return parseToDataMap(je.getAsJsonObject());
}else{
return null;
}
}
public static DataMap parseToDataMap(JsonObject jsonObject) throws JsonParseException {
DataMap map = new DataMap();
Set> entrySet = jsonObject.entrySet();
for (Map.Entry entry : entrySet) {
JsonElement e=entry.getValue();
Object v=toObject(e);
map.put(entry.getKey(),v);
}
return map;
}
private static Object toObject(JsonElement e){
if(e==null || e.isJsonNull()){
return null;
}else if(e.isJsonPrimitive()){
JsonPrimitive x=(JsonPrimitive)e;
if(x.isNumber()){
return e.getAsNumber();
}else{
return e.getAsString();
}
}else if(e.isJsonObject()){
return parseToDataMap(e.getAsJsonObject());
}else if(e.isJsonArray()){
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy