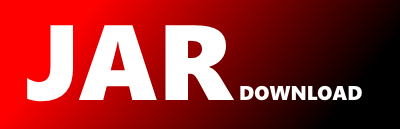
com.tsc9526.monalisa.orm.annotation.DB Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.orm.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import com.tsc9526.monalisa.orm.datasource.ConfigClass;
/**
*
* @DB(url="jdbc:mysql://127.0.0.1:3306/test", username="root", password="root")
* public interface Test {
* public final static DBConfig DB=DBConfig.fromClass(Test.class)
* }
*
* Call example:
* Test.DB.select("SELECT * FROM user");
*
*
*
* More properties setup, see: {@link #configFile}
*
* @see com.tsc9526.monalisa.main.DBModelGenerateMain
*
* @author zzg.zhou([email protected])
*
*/
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface DB{
/**
* @return JDBC-URL. This is an example for mysql:
* jdbc:mysql://127.0.0.1:3306/world
*
* Multi databases:
* jdbc:mysql://[127.0.0.1:3306,127.0.0.1:3307]/world
*
* Host: [name1@host1:port1,name2@host2:port2 ...]
*/
String url() default "";
/**
* @return Database driver.
*/
String driver() default "";
/**
*
* @return Database's catalog. default is ""
*/
String catalog() default "";
/**
*
* @return Database's schema. default is ""
*/
String schema() default "";
/**
* @return Database's user name. default is: root
*/
String username() default "root";
/**
* @return Database's password, default is: ""
*/
String password() default "";
/**
* default is "", means: disable database service
* if set, you can access database via url: $web_app_uri/dbs/$this_dbs_name/$your_table_name
* @return name of the database service.
*/
String dbs() default "";
/**
* default "monalisa:monalisa",
* if set, you can access database via url: http://username:password@host:port/$web_app_path/dbs/$this_dbs_name/$your_table_name
* @return username:password to access the database service. set to "none" means no need username and password
*/
String dbsAuthUsers() default "";
/**
* Indicates the table name to generate the model class, default is "%" (means is all tables).
* For example: pre_%: all tables with the prefix: "pre_"
* @return table’s name
*/
String tables() default "%";
/**
* Define partition tables. format: table_prefix{partition_class(arg1,arg2,arg3 ...)}; ...
* partition_class: DatePartitionTable, arg1: date format, arg2: date field
* For example, storage of the log table every day:
* log_access_{DatePartitionTable(yyyyMMdd,log_time)}
*
*
* Json format:
* {prefix:'log_access_', class='DatePartitionTable', args=['yyyyMMdd','log_time']}
or
* [{prefix:'log_access_', class='DatePartitionTable', args=['yyyyMMdd','log_time']},...]
*
* @return the define of partition tables
*/
String partitions() default "";
/**
* @return Table name's mapping, default is: ""
* For example: table_123=ModelX;table123=ModelY
*/
String mapping() default "";
/**
* Specifies the parent class of the mode class. The Class must be inherited from {@link com.tsc9526.monalisa.orm.model.Model}
*
* @return parent class, default is ""
*/
String modelClass() default "";
/**
* Mode listener, he Class must be implements {@link com.tsc9526.monalisa.orm.model.ModelListener}
*
* @return mode listener
*/
String modelListener() default "";
/**
* Data source class, the value can be C3p0DataSource or DruidDataSource or other class which implementations of the class:
* {@link com.tsc9526.monalisa.orm.datasource.PooledDataSource}
*
* @see com.tsc9526.monalisa.orm.datasource.PooledDataSource
* @return data source class
*/
String datasourceClass() default "";
/**
* Unique identifier for this db, Use the standard JAVA package class naming style, e.g.: x.y.z
* The default value is the class name annotated with @DB
*
* @return Unique identifier for this db
*
*/
String key() default "";
/**
*
* the configuration name. For example: TEST, The prefix of property xxx is: DB.TEST.xxx
* Note: "cfg" is a generic configuration name, not as a configuration name.
*
* @return config name of this db
*/
String configName() default "";
/**
* Database's properties are stored in this configuration file.
* Each of property with prefix: "DB.cfg."
* For example: url, the property name is:
DB.cfg.url = jdbc:mysql://127.0.0.1:3306/world
*
* Full properties see: {@link com.tsc9526.monalisa.orm.datasource.DbProp}
*
* Default configuration file is: full class name annotated with @DB
* For example:
*
* package test;
@DB public interface UserDB{}
*
The configuration file will be: test.UserDB.cfg
*
* Another example:
*
* package test;
@DB(configFile="test.cfg") public interface UserDB{}
*
The configuration file will be: test.cfg
*
* It's priority is lower than configClass()
* @return path of the config file
*/
String configFile() default "";
/**
* Database's properties are stored in this class.
* It's priority is higer than configFile()
* @return ConfigClass.class
*/
Class extends ConfigClass> configClass() default ConfigClass.class;
String[] properties() default {};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy