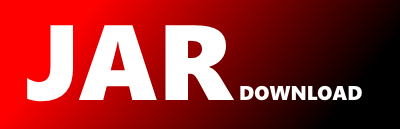
com.tsc9526.monalisa.orm.datasource.DbProp Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.orm.datasource;
import com.tsc9526.monalisa.orm.dialect.Dialect;
/**
* Database properties:
- sql.debug = false [scope: DB]
* If show running SQL statements
*
- url = [scope: DB]
* the JDBC url, for example: jdbc:mysql://127.0.0.1:3306/world
*
- driver = com.mysql.jdbc.Driver [scope: DB]
* the JDBC driver class
*
* - username = root [scope: DB]
* the database username
*
* - password = [scope: DB]
* the database password
*
* - catalog = [scope: DB]
* the database catalog
*
* - schema = [scope: DB]
* the database schema
*
* - tables = % [scope: DB]
* Indicates the table names to generate the model classes, "%": means all of tables.
* For example: pre_%: all tables with the prefix: "pre_"
*
* - mapping = [scope: DB]
* Table name's mapping, For example: table_123=ModelX;table123=ModelY...
*
* - partitions = [scope: DB]
* Define partition tables
* - datasourceClass = [scope: DB]
* Data source class, the value can be C3p0DataSource or DruidDataSource
* or other class which implementations of the class:
* {@link com.tsc9526.monalisa.orm.datasource.PooledDataSource}
*
* - datasourceDelayClose = [scope: DB]
* After database configuration reload, delay closing data source that has been opened
*
* - history.db = [scope: DB]
* The history table is saved in the database.
*
* - history.prefix.table = history_ [scope: DB]
* Prefix of history tables
*
* - history.prefix.column = history_ [scope: DB]
* Column's prefix in the history tables
*
* - history.tables = [scope: DB]
* Those tables need to record changes in history. %: means all of tables
*
* - multi.resultset.deepth = 100 [scope: DB]
* Useful when only the SQL query return multiple results
*
* - cache.tables = [scope: DB]
* Which tables will be cached
*
* - version.name = version [scope: TABLE]
* The version field's name
*
* - modelClass = [scope: TABLE]
* A base mode class, which inherits from {@link com.tsc9526.monalisa.orm.model.Model}
*
* - modelListener = [scope: TABLE]
* A listen class when model changed
*
* - validate = false [scope: TABLE]
* Whether you need to verify the data before you save it
*
* - validator = [scope: TABLE]
* The model validator class
*
* - cache.class = [scope: TABLE]
* The cache class
*
* - cache.name = default [scope: TABLE]
* The name of cache setting
*
* - cache.eviction = LRU [scope: TABLE]
* The cache eviction's algorithm
*
* - auto.create_time = create_time [scope: TABLE]
* When calling Model.save(), auto set model field's value if exists: create_time
*
* - auto.update_time = update_time [scope: TABLE]
* When calling Model.update(), auto set model field's value if exists: update_time
*
* - auto.create_by = create_by [scope: TABLE]
* When calling Model.save(), auto set model field's value if exists: create_by
* You need to set up thread context by call:
* Tx.put(CONTEXT_CURRENT_USERID,"the_operate_user_id");
*
* - auto.update_by = update_by [scope: TABLE]
* When calling Model.update(), auto set model field's value if exists: update_by
* You need to set up thread context by call:
* Tx.put(CONTEXT_CURRENT_USERID,"the_operate_user_id");
*
* - exception_if_set_field_not_found = false [scope: TABLE]
* If true, throw a exception if set a not exists field in a model, otherwise false.
*
*
*
*
scope:
* - DB
* Meaning: this property is a configuration item for a database scope level
* - TABLE
* Meaning: this property is a configuration item for a table scope level,
* you can specify a value for each table. for example:
*
* DB.cfg.modelClass = MyBaseModelClassName
* DB.cfg.modelClass.user = MyUserModelClassName
*
* All generated model classes which inherits from MyBaseModelClassName except for model: "user",
* the model: "user" will inherits from MyUserModelClassName
*
*
* @author zzg.zhou([email protected])
*/
public class DbProp {
public static boolean ProcessingEnvironment=false;
public final static DbProp PROP_DB_SQL_DEBUG = new DbProp("sql.debug",false);
public final static DbProp PROP_DB_URL = new DbProp("url");
public final static DbProp PROP_DB_DRIVER = new DbProp("driver");
public final static DbProp PROP_DB_CATALOG = new DbProp("catalog");
public final static DbProp PROP_DB_SCHEMA = new DbProp("schema");
public final static DbProp PROP_DB_USERNAME = new DbProp("username");
public final static DbProp PROP_DB_PASSWORD = new DbProp("password");
public final static DbProp PROP_DB_DBS = new DbProp("dbs");
public final static DbProp PROP_DB_DBS_EXCEPTION = new DbProp("dbs.exception");
public final static DbProp PROP_DB_DBS_AUTH_USERS = new DbProp("dbs.auth.users");
public final static DbProp PROP_DB_TABLES = new DbProp("tables");
public final static DbProp PROP_DB_MAPPING = new DbProp("mapping");
public final static DbProp PROP_DB_PARTITIONS = new DbProp("partitions");
public final static DbProp PROP_DB_DATASOURCE_CLASS = new DbProp("datasourceClass");
public final static DbProp PROP_DB_DATASOURCE_DELAY_CLOSE = new DbProp("datasourceDelayClose",30);
public final static DbProp PROP_DB_HISTORY_DB = new DbProp("history.db");
public final static DbProp PROP_DB_HISTORY_PREFIX_TABLE = new DbProp("history.prefix.table", "history_");
public final static DbProp PROP_DB_HISTORY_PREFIX_COLUMN = new DbProp("history.prefix.column","history_");
public final static DbProp PROP_DB_HISTORY_TABLES = new DbProp("history.tables");
public final static DbProp PROP_DB_MULTI_RESULTSET_DEEPTH = new DbProp("multi.resultset.deepth",100);
public final static DbProp PROP_DB_CACHE_TABLES = new DbProp("cache.tables");
public final static DbProp PROP_TABLE_VERSION_FIELD = new DbProp("version.name","VERSION");
public final static DbProp PROP_TABLE_MODEL_CLASS = new DbProp("modelClass");
public final static DbProp PROP_TABLE_MODEL_LISTENER = new DbProp("modelListener");
public final static DbProp PROP_TABLE_VALIDATE = new DbProp("validate",false);
public final static DbProp PROP_TABLE_VALIDATOR = new DbProp("validator");
public final static DbProp PROP_TABLE_SEQ = new DbProp("seq");
public final static DbProp PROP_TABLE_CACHE_CLASS = new DbProp("cache.class");
public final static DbProp PROP_TABLE_CACHE_NAME = new DbProp("cache.name","default");
public final static DbProp PROP_TABLE_CACHE_EVICTION = new DbProp("cache.eviction","LRU");
public final static DbProp PROP_TABLE_AUTO_SET_CREATE_TIME=new DbProp("auto.create_time","create_time");
public final static DbProp PROP_TABLE_AUTO_SET_UPDATE_TIME=new DbProp("auto.update_time","update_time");
public final static DbProp PROP_TABLE_AUTO_SET_CREATE_BY =new DbProp("auto.create_by","create_by");
public final static DbProp PROP_TABLE_AUTO_SET_UPDATE_BY =new DbProp("auto.update_by","update_by");
public final static DbProp PROP_TABLE_EXCEPTION_IF_SET_FIELD_NOT_FOUND=new DbProp("exception_if_set_field_not_found",false);
public final static DbProp PROP_TABLE_DBS_MAX_ROWS =new DbProp("dbs.max.rows",10000);
public static String CFG_FIELD_VERSION ="$VERSION";
public static String CFG_DATATABLE_KEY_SPLIT ="&";
/**
* CFG_PATH= ".";
* The file path for DB.configFile() is :
* System.getProperty("DB@"+DB.key(),CFG_PATH)+"/"+configFile;
*/
public static String CFG_ROOT_PATH = System.getProperty("monalisa.path",".");
/**
* Reloadable java directory
*/
public static String CFG_AGENT_PATH = CFG_ROOT_PATH+"/monalisa/agent";
public static String CFG_LIB_PATH = CFG_ROOT_PATH+"/monalisa/lib";
public static String TMP_ROOT_PATH = CFG_ROOT_PATH+"/monalisa/tmp";
public static String TMP_WORK_DIR_JSP = TMP_ROOT_PATH+"/_jsp";
public static String TMP_WORK_DIR_JAVA = TMP_ROOT_PATH+"/_java";
public static String TMP_WORK_DIR_METATABLE = TMP_ROOT_PATH+"/_meta";
public static String TMP_WORK_DIR_GEN = TMP_ROOT_PATH+"/_gen";
public static int CFG_RELOAD_CLASS_INTERVAL =15;
public static int CFG_RELOAD_MODEL_INTERVAL =15;
/**
* 全局Cache开关, 一个Query在未指定Cache时,允许读取另一个Query对象的缓存数据. 默认关闭
*/
public static boolean CFG_CACHE_GLOABLE_ENABLE=false;
/**
* 默认连接空闲1分钟时,执行保持连接检查的SQL
*/
public static int CFG_CONNECT_IDLE_INTERVALS= 60;
public static boolean CFG_LOG_JARLOCATION_DETAIL=true;
public static String SET_CFG_ROOT_PATH(String cfgRootPath) {
CFG_ROOT_PATH=cfgRootPath;
CFG_AGENT_PATH = CFG_ROOT_PATH+"/monalisa/agent";
CFG_LIB_PATH = CFG_ROOT_PATH+"/monalisa/lib";
SET_TMP_ROOT_PATH(CFG_ROOT_PATH+"/monalisa/tmp");
return CFG_ROOT_PATH;
};
public static String SET_TMP_ROOT_PATH(String tmpRootPath){
TMP_ROOT_PATH=tmpRootPath;
TMP_WORK_DIR_JSP = TMP_ROOT_PATH+"/_jsp";
TMP_WORK_DIR_JAVA = TMP_ROOT_PATH+"/_java";
TMP_WORK_DIR_METATABLE = TMP_ROOT_PATH+"/_meta";
TMP_WORK_DIR_GEN = TMP_ROOT_PATH+"/_gen";
return TMP_ROOT_PATH;
}
private String key;
private String value;
public DbProp(String key){
this.key=key;
}
public DbProp(String key,String value){
this.key=key;
this.value=value;
}
public DbProp(String key,boolean value){
this.key=key;
this.value=value?"true":"false";
}
public DbProp(String key,int value){
this.key=key;
this.value=""+value;
}
public String getKey(){
return key;
}
public String getFullKey(){
return DBConfig.PREFIX_DB+"."+DBConfig.CFG_DEFAULT_NAME+"."+key;
}
public String getFullKey(String configName){
return DBConfig.PREFIX_DB+"."+configName+"."+key;
}
public String getValue(DBConfig db){
return db.getCfg().getProperty(key, value);
}
public int getIntValue(DBConfig db,int defaultValue){
String v=db.getCfg().getProperty(key, value);
if(v!=null && v.trim().length()>0){
return Integer.parseInt(v);
}else{
return defaultValue;
}
}
public int getIntValue(DBConfig db,String tableName, int defaultValue){
if(tableName==null || tableName.length()==0){
return getIntValue(db,defaultValue);
}else{
String v=getValue(db, tableName);
if(v!=null && v.trim().length()>0){
return Integer.parseInt(v);
}else{
return defaultValue;
}
}
}
public String getValue(DBConfig db,String tableName,String defaultValue){
String v=getValue(db, tableName);
if(v==null){
v=defaultValue;
}
return v;
}
public String getValue(DBConfig db,String theTableName){
String tableName=Dialect.getRealname(theTableName);
String v=db.getCfg().getProperty(key+"."+tableName);
if(v!=null){
return v;
}else{
return db.getCfg().getProperty(key, value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy