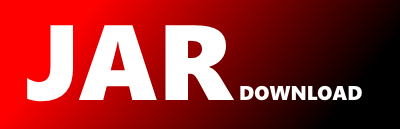
com.tsc9526.monalisa.tools.cache.CacheKey Maven / Gradle / Ivy
/*******************************************************************************************
* Copyright (c) 2016, zzg.zhou([email protected])
*
* Monalisa is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*******************************************************************************************/
package com.tsc9526.monalisa.tools.cache;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author zzg.zhou([email protected])
*/
public class CacheKey {
private static final int DEFAULT_MULTIPLYER = 37;
private static final int DEFAULT_HASHCODE = 17;
private int multiplier=DEFAULT_MULTIPLYER;
private int hashcode=DEFAULT_HASHCODE;
private int count=0;
private long checksum;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy