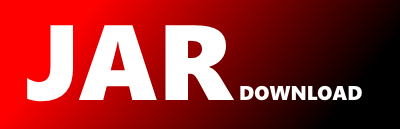
com.tukeof.common.crypto.HmacEnum Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-core Show documentation
Show all versions of common-core Show documentation
a common and useful pure java library
The newest version!
package com.tukeof.common.crypto;
import com.tukeof.common.util.Base64Util;
import com.tukeof.common.util.ByteUtil;
import lombok.extern.slf4j.Slf4j;
import javax.crypto.KeyGenerator;
import javax.crypto.Mac;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
// Message Authentication Code
@Slf4j
public enum HmacEnum {
HMAC_MD5("HmacMD5"),
HMAC_SHA_1("HmacSHA1"),
HMAC_SHA_224("HmacSHA224"),
HMAC_SHA_256("HmacSHA256"),
HMAC_SHA_384("HmacSHA384"),
HMAC_SHA_512("HmacSHA512");
// ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ====
private final String algorithm;
private volatile int bufferSize;
HmacEnum(String algorithm) {
this.algorithm = algorithm;
this.bufferSize = 4096;
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public byte[] digest(byte[] data, byte[] key) throws Exception {
Mac mac = generateMac(key);
mac.update(data);
return mac.doFinal();
}
public byte[] digest(InputStream data, byte[] key) throws Exception {
Mac mac = generateMac(key);
final int size = bufferSize;
final byte[] buffer = new byte[size];
int read = data.read(buffer, 0, size);
while (read > -1) {
mac.update(buffer, 0, read);
read = data.read(buffer, 0, bufferSize);
}
return mac.doFinal();
}
public byte[] digest(ByteBuffer data, byte[] key) throws Exception {
Mac mac = generateMac(key);
mac.update(data);
return mac.doFinal();
}
public String digestToHex(byte[] data, byte[] key) throws Exception {
return ByteUtil.hex(digest(data, key));
}
public String digestToHex(InputStream data, byte[] key) throws Exception {
return ByteUtil.hex(digest(data, key));
}
public String digestToBase64(String base64Data, String base64Key) throws Exception {
byte[] keyBytes = Base64Util.decodeBase64(base64Key);
return digestToBase64(Base64Util.decodeBase64(base64Data), keyBytes);
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public String digestToBase64(byte[] data, String base64Key) throws Exception {
byte[] keyBytes = Base64Util.decodeBase64(base64Key);
return digestToBase64(data, keyBytes);
}
public String digestToBase64(byte[] data, byte[] key) throws Exception {
return Base64Util.encodeBase64ToString(digest(data, key));
}
// ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ====
public String digestToBase64(InputStream data, String key) throws Exception {
byte[] keyBytes = Base64Util.decodeBase64(key);
return Base64Util.encodeBase64ToString(digest(data, keyBytes));
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public byte[] generateKey() {
KeyGenerator keyGenerator;
try {
keyGenerator = KeyGenerator.getInstance(algorithm);
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
SecretKey secretKey = keyGenerator.generateKey();
return secretKey.getEncoded();
}
public String generateBase64Key() {
return Base64Util.encodeBase64ToString(generateKey());
}
private Mac generateMac(byte[] key) throws InvalidKeyException, NoSuchAlgorithmException {
Mac mac = Mac.getInstance(algorithm);
SecretKeySpec secret_key = new SecretKeySpec(key, algorithm);
mac.init(secret_key);
return mac;
}
public HmacEnum withBufferSize(int bufferSize) {
this.bufferSize = bufferSize;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy