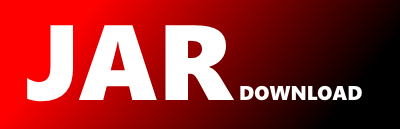
com.tukeof.common.crypto.MessageDigestEnum Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-core Show documentation
Show all versions of common-core Show documentation
a common and useful pure java library
The newest version!
package com.tukeof.common.crypto;
import com.tukeof.common.util.Base64Util;
import com.tukeof.common.util.ByteUtil;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
/**
* Create by tuke on 2019-02-17
*/
public enum MessageDigestEnum {
MD2("MD2"),
MD5("MD5"),
SHA_1("SHA-1"),
SHA_224("SHA-224"),
SHA_256("SHA-256"),
SHA_384("SHA-384"),
SHA_512("SHA-512"),
SHA3_224("SHA3-224"),
SHA3_256("SHA3-256"),
SHA3_384("SHA3-384"),
SHA3_512("SHA3-512");
// ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ====
private final String algorithm;
private volatile int bufferSize;
MessageDigestEnum(String algorithm) {
this.algorithm = algorithm;
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public byte[] digest(byte[] input) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance(algorithm);
return md.digest(input);
}
public byte[] digest(InputStream input) throws NoSuchAlgorithmException, IOException {
MessageDigest md = MessageDigest.getInstance(algorithm);
final int size = bufferSize;
final byte[] buffer = new byte[size];
int read = input.read(buffer, 0, size);
while (read > -1) {
md.update(buffer, 0, read);
read = input.read(buffer, 0, size);
}
return md.digest();
}
public byte[] digest(ByteBuffer input) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance(algorithm);
md.update(input);
return md.digest();
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public String digestToHex(byte[] input) throws NoSuchAlgorithmException {
return ByteUtil.hex(digest(input));
}
public String digestToHex(InputStream input) throws NoSuchAlgorithmException, IOException {
return ByteUtil.hex(digest(input));
}
public String digestToHex(ByteBuffer input) throws NoSuchAlgorithmException {
return ByteUtil.hex(digest(input));
}
// ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ---- ----
public String digestToBase64(byte[] input) throws NoSuchAlgorithmException {
return Base64Util.encodeBase64ToString(digest(input));
}
public String digestToBase64(InputStream input) throws NoSuchAlgorithmException, IOException {
return Base64Util.encodeBase64ToString(digest(input));
}
// ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ==== ====
public String digestToBase64(ByteBuffer input) throws NoSuchAlgorithmException {
return Base64Util.encodeBase64ToString(digest(input));
}
public String digestToString(byte[] input) throws NoSuchAlgorithmException {
return new String(digest(input), StandardCharsets.ISO_8859_1);
}
public String digestToString(String input) throws NoSuchAlgorithmException {
return digestToString(input.getBytes(StandardCharsets.ISO_8859_1));
}
public MessageDigestEnum withBufferSize(int bufferSize) {
this.bufferSize = bufferSize;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy