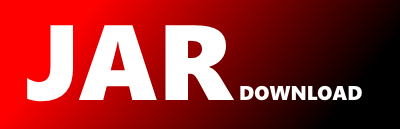
com.turbospaces.http.UnexpectedHttpClientException Maven / Gradle / Ivy
package com.turbospaces.http;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.apache.commons.lang3.StringUtils;
import org.apache.http.Header;
import org.apache.http.StatusLine;
import com.google.common.collect.ImmutableList;
import lombok.Getter;
@SuppressWarnings("serial")
public class UnexpectedHttpClientException extends UnexpectedHttpStatusException {
@Getter
private final Header[] headers;
public UnexpectedHttpClientException(StatusLine status, String entity, Header[] headers) {
super(status.toString(), entity, status.getStatusCode());
this.headers = headers;
}
@Override
public List getHeaderValues(String key) {
ImmutableList.Builder l = ImmutableList.builder();
if (Objects.nonNull(headers)) {
for (Header header : headers) {
if (StringUtils.equalsIgnoreCase(header.getName(), key)) {
l.add(header.getValue());
}
}
}
return l.build();
}
@Override
public Optional getFirstHeaderValue(String key) {
if (Objects.nonNull(headers)) {
for (Header header : headers) {
if (StringUtils.equalsIgnoreCase(header.getName(), key)) {
return Optional.of(header.getValue());
}
}
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy