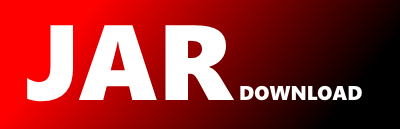
com.turbospaces.web3j.Web3jFactoryBean Maven / Gradle / Ivy
package com.turbospaces.web3j;
import org.springframework.beans.factory.config.AbstractFactoryBean;
import org.web3j.protocol.Web3j;
import org.web3j.protocol.http.HttpService;
import com.turbospaces.cfg.ApplicationProperties;
import com.turbospaces.ups.PlainServiceInfo;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import okhttp3.OkHttpClient;
import okhttp3.logging.HttpLoggingInterceptor;
@Slf4j
@RequiredArgsConstructor
public class Web3jFactoryBean extends AbstractFactoryBean {
private final ApplicationProperties props;
private final PlainServiceInfo serviceInfo;
@Override
public Class> getObjectType() {
return Web3j.class;
}
@Override
protected Web3j createInstance() throws Exception {
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.connectTimeout(props.TCP_CONNECTION_TIMEOUT.get());
builder.readTimeout(props.TCP_SOCKET_TIMEOUT.get());
HttpLoggingInterceptor logging = new HttpLoggingInterceptor(log::debug);
logging.setLevel(HttpLoggingInterceptor.Level.HEADERS);
builder.addInterceptor(logging);
OkHttpClient okhttp = builder.build();
String uri = serviceInfo.getUri();
HttpService httpService = new HttpService(uri, okhttp, false);
return Web3j.build(httpService);
}
@Override
protected void destroyInstance(Web3j instance) throws Exception {
if (instance != null) {
instance.shutdown();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy