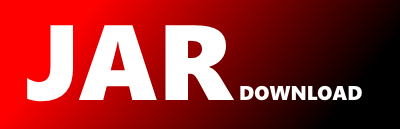
com.tvd12.ezydata.elasticsearch.action.EzyEsSimpleIndexAction Maven / Gradle / Ivy
The newest version!
package com.tvd12.ezydata.elasticsearch.action;
import lombok.Getter;
import org.elasticsearch.client.RequestOptions;
import java.util.*;
@Getter
public class EzyEsSimpleIndexAction implements EzyEsIndexAction {
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy