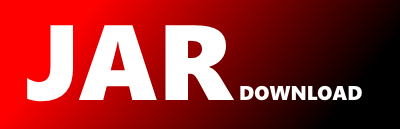
com.tvd12.ezydata.elasticsearch.handler.EzyEsIndexActionHandler Maven / Gradle / Ivy
The newest version!
package com.tvd12.ezydata.elasticsearch.handler;
import com.tvd12.ezydata.elasticsearch.action.EzyEsIndexAction;
import com.tvd12.ezyfox.entity.EzyObject;
import com.tvd12.ezyfox.identifier.EzyIdFetcher;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class EzyEsIndexActionHandler extends EzyEsAbstractActionHandler {
@SuppressWarnings("unchecked")
@Override
public BulkResponse handle(EzyEsIndexAction action) throws Exception {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy