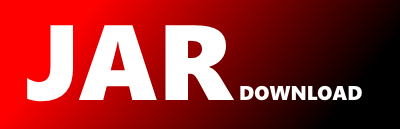
com.tvd12.gamebox.octree.SynchronizedOcTree Maven / Gradle / Ivy
The newest version!
package com.tvd12.gamebox.octree;
import com.tvd12.gamebox.entity.PositionAware;
import com.tvd12.gamebox.math.Vec3;
import java.util.List;
public class SynchronizedOcTree extends OcTree {
public SynchronizedOcTree(
Vec3 leftBottomBack,
Vec3 rightTopFront,
int maxItemsPerNode,
float minNodeSize
) {
super(leftBottomBack, rightTopFront, maxItemsPerNode, minNodeSize);
}
public boolean insert(T item) {
synchronized (this) {
return super.insert(item);
}
}
public boolean remove(T item) {
synchronized (this) {
return super.remove(item);
}
}
public List search(T item, float range) {
synchronized (this) {
return super.search(item, range);
}
}
public boolean contains(T item) {
synchronized (this) {
return super.contains(item);
}
}
public OcTreeNode findNodeContainingPosition(Vec3 position) {
synchronized (this) {
return super.findNodeContainingPosition(position);
}
}
public boolean isItemRemainingAtSameNode(T item, Vec3 newPosition) {
synchronized (this) {
return super.isItemRemainingAtSameNode(item, newPosition);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy