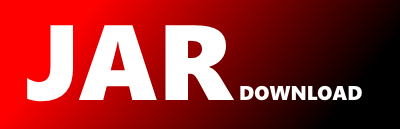
com.twilio.rest.api.v2010.account.IncomingPhoneNumberUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/*
* This code was generated by
* ___ _ _ _ _ _ _ ____ ____ ____ _ ____ ____ _ _ ____ ____ ____ ___ __ __
* | | | | | | | | | __ | | |__| | __ | __ |___ |\ | |___ |__/ |__| | | | |__/
* | |_|_| | |___ | |__| |__| | | | |__] |___ | \| |___ | \ | | | |__| | \
*
* Twilio - Api
* This is the public Twilio REST API.
*
* NOTE: This class is auto generated by OpenAPI Generator.
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.twilio.rest.api.v2010.account;
import com.twilio.base.Updater;
import com.twilio.constant.EnumConstants;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
public class IncomingPhoneNumberUpdater extends Updater {
private String pathSid;
private String pathAccountSid;
private String accountSid;
private String apiVersion;
private String friendlyName;
private String smsApplicationSid;
private HttpMethod smsFallbackMethod;
private URI smsFallbackUrl;
private HttpMethod smsMethod;
private URI smsUrl;
private URI statusCallback;
private HttpMethod statusCallbackMethod;
private String voiceApplicationSid;
private Boolean voiceCallerIdLookup;
private HttpMethod voiceFallbackMethod;
private URI voiceFallbackUrl;
private HttpMethod voiceMethod;
private URI voiceUrl;
private IncomingPhoneNumber.EmergencyStatus emergencyStatus;
private String emergencyAddressSid;
private String trunkSid;
private IncomingPhoneNumber.VoiceReceiveMode voiceReceiveMode;
private String identitySid;
private String addressSid;
private String bundleSid;
public IncomingPhoneNumberUpdater(final String pathSid) {
this.pathSid = pathSid;
}
public IncomingPhoneNumberUpdater(
final String pathAccountSid,
final String pathSid
) {
this.pathAccountSid = pathAccountSid;
this.pathSid = pathSid;
}
public IncomingPhoneNumberUpdater setAccountSid(final String accountSid) {
this.accountSid = accountSid;
return this;
}
public IncomingPhoneNumberUpdater setApiVersion(final String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
public IncomingPhoneNumberUpdater setFriendlyName(
final String friendlyName
) {
this.friendlyName = friendlyName;
return this;
}
public IncomingPhoneNumberUpdater setSmsApplicationSid(
final String smsApplicationSid
) {
this.smsApplicationSid = smsApplicationSid;
return this;
}
public IncomingPhoneNumberUpdater setSmsFallbackMethod(
final HttpMethod smsFallbackMethod
) {
this.smsFallbackMethod = smsFallbackMethod;
return this;
}
public IncomingPhoneNumberUpdater setSmsFallbackUrl(
final URI smsFallbackUrl
) {
this.smsFallbackUrl = smsFallbackUrl;
return this;
}
public IncomingPhoneNumberUpdater setSmsFallbackUrl(
final String smsFallbackUrl
) {
return setSmsFallbackUrl(Promoter.uriFromString(smsFallbackUrl));
}
public IncomingPhoneNumberUpdater setSmsMethod(final HttpMethod smsMethod) {
this.smsMethod = smsMethod;
return this;
}
public IncomingPhoneNumberUpdater setSmsUrl(final URI smsUrl) {
this.smsUrl = smsUrl;
return this;
}
public IncomingPhoneNumberUpdater setSmsUrl(final String smsUrl) {
return setSmsUrl(Promoter.uriFromString(smsUrl));
}
public IncomingPhoneNumberUpdater setStatusCallback(
final URI statusCallback
) {
this.statusCallback = statusCallback;
return this;
}
public IncomingPhoneNumberUpdater setStatusCallback(
final String statusCallback
) {
return setStatusCallback(Promoter.uriFromString(statusCallback));
}
public IncomingPhoneNumberUpdater setStatusCallbackMethod(
final HttpMethod statusCallbackMethod
) {
this.statusCallbackMethod = statusCallbackMethod;
return this;
}
public IncomingPhoneNumberUpdater setVoiceApplicationSid(
final String voiceApplicationSid
) {
this.voiceApplicationSid = voiceApplicationSid;
return this;
}
public IncomingPhoneNumberUpdater setVoiceCallerIdLookup(
final Boolean voiceCallerIdLookup
) {
this.voiceCallerIdLookup = voiceCallerIdLookup;
return this;
}
public IncomingPhoneNumberUpdater setVoiceFallbackMethod(
final HttpMethod voiceFallbackMethod
) {
this.voiceFallbackMethod = voiceFallbackMethod;
return this;
}
public IncomingPhoneNumberUpdater setVoiceFallbackUrl(
final URI voiceFallbackUrl
) {
this.voiceFallbackUrl = voiceFallbackUrl;
return this;
}
public IncomingPhoneNumberUpdater setVoiceFallbackUrl(
final String voiceFallbackUrl
) {
return setVoiceFallbackUrl(Promoter.uriFromString(voiceFallbackUrl));
}
public IncomingPhoneNumberUpdater setVoiceMethod(
final HttpMethod voiceMethod
) {
this.voiceMethod = voiceMethod;
return this;
}
public IncomingPhoneNumberUpdater setVoiceUrl(final URI voiceUrl) {
this.voiceUrl = voiceUrl;
return this;
}
public IncomingPhoneNumberUpdater setVoiceUrl(final String voiceUrl) {
return setVoiceUrl(Promoter.uriFromString(voiceUrl));
}
public IncomingPhoneNumberUpdater setEmergencyStatus(
final IncomingPhoneNumber.EmergencyStatus emergencyStatus
) {
this.emergencyStatus = emergencyStatus;
return this;
}
public IncomingPhoneNumberUpdater setEmergencyAddressSid(
final String emergencyAddressSid
) {
this.emergencyAddressSid = emergencyAddressSid;
return this;
}
public IncomingPhoneNumberUpdater setTrunkSid(final String trunkSid) {
this.trunkSid = trunkSid;
return this;
}
public IncomingPhoneNumberUpdater setVoiceReceiveMode(
final IncomingPhoneNumber.VoiceReceiveMode voiceReceiveMode
) {
this.voiceReceiveMode = voiceReceiveMode;
return this;
}
public IncomingPhoneNumberUpdater setIdentitySid(final String identitySid) {
this.identitySid = identitySid;
return this;
}
public IncomingPhoneNumberUpdater setAddressSid(final String addressSid) {
this.addressSid = addressSid;
return this;
}
public IncomingPhoneNumberUpdater setBundleSid(final String bundleSid) {
this.bundleSid = bundleSid;
return this;
}
@Override
public IncomingPhoneNumber update(final TwilioRestClient client) {
String path =
"/2010-04-01/Accounts/{AccountSid}/IncomingPhoneNumbers/{Sid}.json";
this.pathAccountSid =
this.pathAccountSid == null
? client.getAccountSid()
: this.pathAccountSid;
path =
path.replace(
"{" + "AccountSid" + "}",
this.pathAccountSid.toString()
);
path = path.replace("{" + "Sid" + "}", this.pathSid.toString());
Request request = new Request(
HttpMethod.POST,
Domains.API.toString(),
path
);
request.setContentType(EnumConstants.ContentType.FORM_URLENCODED);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException(
"IncomingPhoneNumber update failed: Unable to connect to server"
);
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(
response.getStream(),
client.getObjectMapper()
);
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return IncomingPhoneNumber.fromJson(
response.getStream(),
client.getObjectMapper()
);
}
private void addPostParams(final Request request) {
if (accountSid != null) {
request.addPostParam("AccountSid", accountSid);
}
if (apiVersion != null) {
request.addPostParam("ApiVersion", apiVersion);
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (smsApplicationSid != null) {
request.addPostParam("SmsApplicationSid", smsApplicationSid);
}
if (smsFallbackMethod != null) {
request.addPostParam(
"SmsFallbackMethod",
smsFallbackMethod.toString()
);
}
if (smsFallbackUrl != null) {
request.addPostParam("SmsFallbackUrl", smsFallbackUrl.toString());
}
if (smsMethod != null) {
request.addPostParam("SmsMethod", smsMethod.toString());
}
if (smsUrl != null) {
request.addPostParam("SmsUrl", smsUrl.toString());
}
if (statusCallback != null) {
request.addPostParam("StatusCallback", statusCallback.toString());
}
if (statusCallbackMethod != null) {
request.addPostParam(
"StatusCallbackMethod",
statusCallbackMethod.toString()
);
}
if (voiceApplicationSid != null) {
request.addPostParam("VoiceApplicationSid", voiceApplicationSid);
}
if (voiceCallerIdLookup != null) {
request.addPostParam(
"VoiceCallerIdLookup",
voiceCallerIdLookup.toString()
);
}
if (voiceFallbackMethod != null) {
request.addPostParam(
"VoiceFallbackMethod",
voiceFallbackMethod.toString()
);
}
if (voiceFallbackUrl != null) {
request.addPostParam(
"VoiceFallbackUrl",
voiceFallbackUrl.toString()
);
}
if (voiceMethod != null) {
request.addPostParam("VoiceMethod", voiceMethod.toString());
}
if (voiceUrl != null) {
request.addPostParam("VoiceUrl", voiceUrl.toString());
}
if (emergencyStatus != null) {
request.addPostParam("EmergencyStatus", emergencyStatus.toString());
}
if (emergencyAddressSid != null) {
request.addPostParam("EmergencyAddressSid", emergencyAddressSid);
}
if (trunkSid != null) {
request.addPostParam("TrunkSid", trunkSid);
}
if (voiceReceiveMode != null) {
request.addPostParam(
"VoiceReceiveMode",
voiceReceiveMode.toString()
);
}
if (identitySid != null) {
request.addPostParam("IdentitySid", identitySid);
}
if (addressSid != null) {
request.addPostParam("AddressSid", addressSid);
}
if (bundleSid != null) {
request.addPostParam("BundleSid", bundleSid);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy