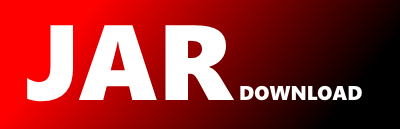
com.twilio.rest.messaging.v1.service.UsAppToPersonCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/*
* This code was generated by
* ___ _ _ _ _ _ _ ____ ____ ____ _ ____ ____ _ _ ____ ____ ____ ___ __ __
* | | | | | | | | | __ | | |__| | __ | __ |___ |\ | |___ |__/ |__| | | | |__/
* | |_|_| | |___ | |__| |__| | | | |__] |___ | \| |___ | \ | | | |__| | \
*
* Twilio - Messaging
* This is the public Twilio REST API.
*
* NOTE: This class is auto generated by OpenAPI Generator.
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.twilio.rest.messaging.v1.service;
import com.twilio.base.Creator;
import com.twilio.constant.EnumConstants;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.util.List;
import java.util.List;
public class UsAppToPersonCreator extends Creator {
private String pathMessagingServiceSid;
private String brandRegistrationSid;
private String description;
private String messageFlow;
private List messageSamples;
private String usAppToPersonUsecase;
private Boolean hasEmbeddedLinks;
private Boolean hasEmbeddedPhone;
private String optInMessage;
private String optOutMessage;
private String helpMessage;
private List optInKeywords;
private List optOutKeywords;
private List helpKeywords;
public UsAppToPersonCreator(
final String pathMessagingServiceSid,
final String brandRegistrationSid,
final String description,
final String messageFlow,
final List messageSamples,
final String usAppToPersonUsecase,
final Boolean hasEmbeddedLinks,
final Boolean hasEmbeddedPhone
) {
this.pathMessagingServiceSid = pathMessagingServiceSid;
this.brandRegistrationSid = brandRegistrationSid;
this.description = description;
this.messageFlow = messageFlow;
this.messageSamples = messageSamples;
this.usAppToPersonUsecase = usAppToPersonUsecase;
this.hasEmbeddedLinks = hasEmbeddedLinks;
this.hasEmbeddedPhone = hasEmbeddedPhone;
}
public UsAppToPersonCreator setBrandRegistrationSid(
final String brandRegistrationSid
) {
this.brandRegistrationSid = brandRegistrationSid;
return this;
}
public UsAppToPersonCreator setDescription(final String description) {
this.description = description;
return this;
}
public UsAppToPersonCreator setMessageFlow(final String messageFlow) {
this.messageFlow = messageFlow;
return this;
}
public UsAppToPersonCreator setMessageSamples(
final List messageSamples
) {
this.messageSamples = messageSamples;
return this;
}
public UsAppToPersonCreator setMessageSamples(final String messageSamples) {
return setMessageSamples(Promoter.listOfOne(messageSamples));
}
public UsAppToPersonCreator setUsAppToPersonUsecase(
final String usAppToPersonUsecase
) {
this.usAppToPersonUsecase = usAppToPersonUsecase;
return this;
}
public UsAppToPersonCreator setHasEmbeddedLinks(
final Boolean hasEmbeddedLinks
) {
this.hasEmbeddedLinks = hasEmbeddedLinks;
return this;
}
public UsAppToPersonCreator setHasEmbeddedPhone(
final Boolean hasEmbeddedPhone
) {
this.hasEmbeddedPhone = hasEmbeddedPhone;
return this;
}
public UsAppToPersonCreator setOptInMessage(final String optInMessage) {
this.optInMessage = optInMessage;
return this;
}
public UsAppToPersonCreator setOptOutMessage(final String optOutMessage) {
this.optOutMessage = optOutMessage;
return this;
}
public UsAppToPersonCreator setHelpMessage(final String helpMessage) {
this.helpMessage = helpMessage;
return this;
}
public UsAppToPersonCreator setOptInKeywords(
final List optInKeywords
) {
this.optInKeywords = optInKeywords;
return this;
}
public UsAppToPersonCreator setOptInKeywords(final String optInKeywords) {
return setOptInKeywords(Promoter.listOfOne(optInKeywords));
}
public UsAppToPersonCreator setOptOutKeywords(
final List optOutKeywords
) {
this.optOutKeywords = optOutKeywords;
return this;
}
public UsAppToPersonCreator setOptOutKeywords(final String optOutKeywords) {
return setOptOutKeywords(Promoter.listOfOne(optOutKeywords));
}
public UsAppToPersonCreator setHelpKeywords(
final List helpKeywords
) {
this.helpKeywords = helpKeywords;
return this;
}
public UsAppToPersonCreator setHelpKeywords(final String helpKeywords) {
return setHelpKeywords(Promoter.listOfOne(helpKeywords));
}
@Override
public UsAppToPerson create(final TwilioRestClient client) {
String path = "/v1/Services/{MessagingServiceSid}/Compliance/Usa2p";
path =
path.replace(
"{" + "MessagingServiceSid" + "}",
this.pathMessagingServiceSid.toString()
);
path =
path.replace(
"{" + "BrandRegistrationSid" + "}",
this.brandRegistrationSid.toString()
);
path =
path.replace(
"{" + "Description" + "}",
this.description.toString()
);
path =
path.replace(
"{" + "MessageFlow" + "}",
this.messageFlow.toString()
);
path =
path.replace(
"{" + "MessageSamples" + "}",
this.messageSamples.toString()
);
path =
path.replace(
"{" + "UsAppToPersonUsecase" + "}",
this.usAppToPersonUsecase.toString()
);
path =
path.replace(
"{" + "HasEmbeddedLinks" + "}",
this.hasEmbeddedLinks.toString()
);
path =
path.replace(
"{" + "HasEmbeddedPhone" + "}",
this.hasEmbeddedPhone.toString()
);
Request request = new Request(
HttpMethod.POST,
Domains.MESSAGING.toString(),
path
);
request.setContentType(EnumConstants.ContentType.FORM_URLENCODED);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException(
"UsAppToPerson creation failed: Unable to connect to server"
);
} else if (!TwilioRestClient.SUCCESS.test(response.getStatusCode())) {
RestException restException = RestException.fromJson(
response.getStream(),
client.getObjectMapper()
);
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(restException);
}
return UsAppToPerson.fromJson(
response.getStream(),
client.getObjectMapper()
);
}
private void addPostParams(final Request request) {
if (brandRegistrationSid != null) {
request.addPostParam("BrandRegistrationSid", brandRegistrationSid);
}
if (description != null) {
request.addPostParam("Description", description);
}
if (messageFlow != null) {
request.addPostParam("MessageFlow", messageFlow);
}
if (messageSamples != null) {
for (String prop : messageSamples) {
request.addPostParam("MessageSamples", prop);
}
}
if (usAppToPersonUsecase != null) {
request.addPostParam("UsAppToPersonUsecase", usAppToPersonUsecase);
}
if (hasEmbeddedLinks != null) {
request.addPostParam(
"HasEmbeddedLinks",
hasEmbeddedLinks.toString()
);
}
if (hasEmbeddedPhone != null) {
request.addPostParam(
"HasEmbeddedPhone",
hasEmbeddedPhone.toString()
);
}
if (optInMessage != null) {
request.addPostParam("OptInMessage", optInMessage);
}
if (optOutMessage != null) {
request.addPostParam("OptOutMessage", optOutMessage);
}
if (helpMessage != null) {
request.addPostParam("HelpMessage", helpMessage);
}
if (optInKeywords != null) {
for (String prop : optInKeywords) {
request.addPostParam("OptInKeywords", prop);
}
}
if (optOutKeywords != null) {
for (String prop : optOutKeywords) {
request.addPostParam("OptOutKeywords", prop);
}
}
if (helpKeywords != null) {
for (String prop : helpKeywords) {
request.addPostParam("HelpKeywords", prop);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy