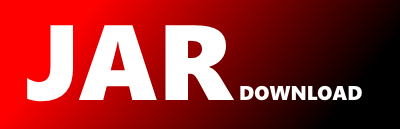
com.twilio.twiml.voice.Prompt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.twiml.voice;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlProperty;
import com.twilio.converter.Promoter;
import com.twilio.twiml.TwiML;
import com.twilio.twiml.TwiMLException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* TwiML wrapper for {@code }
*/
@JsonDeserialize(builder = Prompt.Builder.class)
public class Prompt extends TwiML {
public enum For {
PAYMENT_CARD_NUMBER("payment-card-number"),
EXPIRATION_DATE("expiration-date"),
SECURITY_CODE("security-code"),
POSTAL_CODE("postal-code"),
PAYMENT_PROCESSING("payment-processing"),
BANK_ACCOUNT_NUMBER("bank-account-number"),
BANK_ROUTING_NUMBER("bank-routing-number");
private final String value;
private For(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
public enum ErrorType {
TIMEOUT("timeout"),
INVALID_CARD_NUMBER("invalid-card-number"),
INVALID_CARD_TYPE("invalid-card-type"),
INVALID_DATE("invalid-date"),
INVALID_SECURITY_CODE("invalid-security-code"),
INTERNAL_ERROR("internal-error"),
INPUT_MATCHING_FAILED("input-matching-failed");
private final String value;
private ErrorType(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
public enum CardType {
VISA("visa"),
MASTERCARD("mastercard"),
AMEX("amex"),
MAESTRO("maestro"),
DISCOVER("discover"),
OPTIMA("optima"),
JCB("jcb"),
DINERS_CLUB("diners-club"),
ENROUTE("enroute");
private final String value;
private CardType(final String value) {
this.value = value;
}
public String toString() {
return value;
}
}
private final Prompt.For for_;
private final List errorType;
private final List cardType;
private final List attempt;
private final Boolean requireMatchingInputs;
/**
* For XML Serialization/Deserialization
*/
private Prompt() {
this(new Builder());
}
/**
* Create a new {@code } element
*/
private Prompt(Builder b) {
super("Prompt", b);
this.for_ = b.for_;
this.errorType = b.errorType;
this.cardType = b.cardType;
this.attempt = b.attempt;
this.requireMatchingInputs = b.requireMatchingInputs;
}
/**
* Attributes to set on the generated XML element
*
* @return A Map of attribute keys to values
*/
protected Map getElementAttributes() {
// Preserve order of attributes
Map attrs = new HashMap<>();
if (this.getFor_() != null) {
attrs.put("for_", this.getFor_().toString());
}
if (this.getErrorTypes() != null) {
attrs.put("errorType", this.getErrorTypesAsString());
}
if (this.getCardTypes() != null) {
attrs.put("cardType", this.getCardTypesAsString());
}
if (this.getAttempts() != null) {
attrs.put("attempt", this.getAttemptsAsString());
}
if (this.isRequireMatchingInputs() != null) {
attrs.put("requireMatchingInputs", this.isRequireMatchingInputs().toString());
}
return attrs;
}
/**
* Name of the payment source data element
*
* @return Name of the payment source data element
*/
public Prompt.For getFor_() {
return for_;
}
/**
* Type of error
*
* @return Type of error
*/
public List getErrorTypes() {
return errorType;
}
protected String getErrorTypesAsString() {
StringBuilder sb = new StringBuilder();
Iterator iter = this.getErrorTypes().iterator();
while (iter.hasNext()) {
sb.append(iter.next().toString());
if (iter.hasNext()) {
sb.append(" ");
}
}
return sb.toString();
}
/**
* Type of the credit card
*
* @return Type of the credit card
*/
public List getCardTypes() {
return cardType;
}
protected String getCardTypesAsString() {
StringBuilder sb = new StringBuilder();
Iterator iter = this.getCardTypes().iterator();
while (iter.hasNext()) {
sb.append(iter.next().toString());
if (iter.hasNext()) {
sb.append(" ");
}
}
return sb.toString();
}
/**
* Current attempt count
*
* @return Current attempt count
*/
public List getAttempts() {
return attempt;
}
protected String getAttemptsAsString() {
StringBuilder sb = new StringBuilder();
Iterator iter = this.getAttempts().iterator();
while (iter.hasNext()) {
sb.append(iter.next().toString());
if (iter.hasNext()) {
sb.append(" ");
}
}
return sb.toString();
}
/**
* Require customer to input requested information twice and verify matching.
*
* @return Require customer to input requested information twice and verify
* matching.
*/
public Boolean isRequireMatchingInputs() {
return requireMatchingInputs;
}
/**
* Create a new {@code } element
*/
@JsonPOJOBuilder(withPrefix = "")
public static class Builder extends TwiML.Builder {
/**
* Create and return a {@code } from an XML string
*/
public static Builder fromXml(final String xml) throws TwiMLException {
try {
return OBJECT_MAPPER.readValue(xml, Builder.class);
} catch (final JsonProcessingException jpe) {
throw new TwiMLException(
"Failed to deserialize a Prompt.Builder from the provided XML string: " + jpe.getMessage());
} catch (final Exception e) {
throw new TwiMLException("Unhandled exception: " + e.getMessage());
}
}
private Prompt.For for_;
private List errorType;
private List cardType;
private List attempt;
private Boolean requireMatchingInputs;
/**
* Name of the payment source data element
*/
@JacksonXmlProperty(isAttribute = true, localName = "for")
public Builder for_(Prompt.For for_) {
this.for_ = for_;
return this;
}
/**
* Type of error
*/
@JacksonXmlProperty(isAttribute = true, localName = "errorType")
public Builder errorTypes(List errorType) {
this.errorType = errorType;
return this;
}
/**
* Type of error
*/
public Builder errorTypes(Prompt.ErrorType errorType) {
this.errorType = Promoter.listOfOne(errorType);
return this;
}
/**
* Type of the credit card
*/
@JacksonXmlProperty(isAttribute = true, localName = "cardType")
public Builder cardTypes(List cardType) {
this.cardType = cardType;
return this;
}
/**
* Type of the credit card
*/
public Builder cardTypes(Prompt.CardType cardType) {
this.cardType = Promoter.listOfOne(cardType);
return this;
}
/**
* Current attempt count
*/
@JacksonXmlProperty(isAttribute = true, localName = "attempt")
public Builder attempts(List attempt) {
this.attempt = attempt;
return this;
}
/**
* Current attempt count
*/
public Builder attempts(Integer attempt) {
this.attempt = Promoter.listOfOne(attempt);
return this;
}
/**
* Require customer to input requested information twice and verify matching.
*/
@JacksonXmlProperty(isAttribute = true, localName = "requireMatchingInputs")
public Builder requireMatchingInputs(Boolean requireMatchingInputs) {
this.requireMatchingInputs = requireMatchingInputs;
return this;
}
/**
* Add a child {@code } element
*/
@JacksonXmlProperty(isAttribute = false, localName = "Say")
public Builder say(Say say) {
this.children.add(say);
return this;
}
/**
* Add a child {@code } element
*/
@JacksonXmlProperty(isAttribute = false, localName = "Play")
public Builder play(Play play) {
this.children.add(play);
return this;
}
/**
* Add a child {@code } element
*/
@JacksonXmlProperty(isAttribute = false, localName = "Pause")
public Builder pause(Pause pause) {
this.children.add(pause);
return this;
}
/**
* Create and return resulting {@code } element
*/
public Prompt build() {
return new Prompt(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy