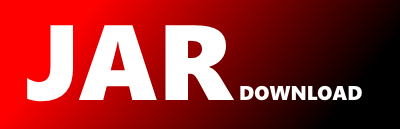
com.twilio.base.bearertoken.Reader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
package com.twilio.base.bearertoken;
import com.twilio.TwilioOrgsTokenAuth;
import com.twilio.http.bearertoken.BearerTokenTwilioRestClient;
import java.util.concurrent.CompletableFuture;
/**
* Executor for listing of a resource.
*
* @param type of the resource
*/
public abstract class Reader {
private Integer pageSize;
private Long limit;
/**
* Execute a request using default client.
*
* @return ResourceSet of objects
*/
public ResourceSet read() {
return read(TwilioOrgsTokenAuth.getRestClient());
}
/**
* Execute a request using specified client.
*
* @param client client used to make request
* @return ResourceSet of objects
*/
public abstract ResourceSet read(final BearerTokenTwilioRestClient client);
/**
* Execute an async request using default client.
*
* @return future that resolves to the ResourceSet of objects
*/
public CompletableFuture> readAsync() {
return readAsync(TwilioOrgsTokenAuth.getRestClient());
}
/**
* Execute an async request using specified client.
*
* @param client client used to make request
* @return future that resolves to the ResourceSet of objects
*/
public CompletableFuture> readAsync(final BearerTokenTwilioRestClient client) {
return CompletableFuture.supplyAsync(() -> read(client), TwilioOrgsTokenAuth.getExecutorService());
}
/**
* Fetch the first page of resources.
*
* @return Page containing the first pageSize of resources
*/
public Page firstPage() {
return firstPage(TwilioOrgsTokenAuth.getRestClient());
}
/**
* Fetch the first page of resources using specified client.
*
* @param client client used to fetch
* @return Page containing the first pageSize of resources
*/
public abstract Page firstPage(final BearerTokenTwilioRestClient client);
/**
* Retrieve the target page of resources.
*
* @param targetUrl API-generated URL for the requested results page
* @return Page containing the target pageSize of resources
*/
public Page getPage(final String targetUrl) {
return getPage(targetUrl, TwilioOrgsTokenAuth.getRestClient());
}
/**
* Retrieve the target page of resources.
*
* @param targetUrl API-generated URL for the requested results page
* @param client client used to fetch
* @return Page containing the target pageSize of resources
*/
public abstract Page getPage(final String targetUrl, final BearerTokenTwilioRestClient client);
/**
* Fetch the following page of resources.
*
* @param page current page of resources
* @return Page containing the next pageSize of resources
*/
public Page nextPage(final Page page) {
return nextPage(page, TwilioOrgsTokenAuth.getRestClient());
}
/**
* Fetch the following page of resources using specified client.
*
* @param page current page of resources
* @param client client used to fetch
* @return Page containing the next pageSize of resources
*/
public abstract Page nextPage(final Page page, final BearerTokenTwilioRestClient client);
/**
* Fetch the prior page of resources.
*
* @param page current page of resources
* @return Page containing the previous pageSize of resources
*/
public Page previousPage(final Page page) {
return previousPage(page, TwilioOrgsTokenAuth.getRestClient());
}
/**
* Fetch the prior page of resources using specified client.
*
* @param page current page of resources
* @param client client used to fetch
* @return Page containing the previous pageSize of resources
*/
public abstract Page previousPage(final Page page, final BearerTokenTwilioRestClient client);
public Integer getPageSize() {
return pageSize;
}
public Reader pageSize(final int pageSize) {
this.pageSize = pageSize;
return this;
}
public Long getLimit() {
return limit;
}
/**
* Sets the max number of records to read.
*
* @param limit max number of records to read
* @return this reader
*/
public Reader limit(final long limit) {
this.limit = limit;
if (this.pageSize == null) {
this.pageSize = this.limit.intValue();
}
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy