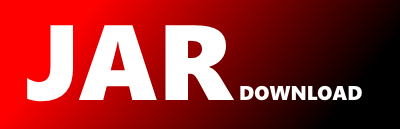
com.twilio.rest.preview.marketplace.AvailableAddOn Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.preview.marketplace;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.Converter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@JsonIgnoreProperties(ignoreUnknown = true)
public class AvailableAddOn extends Resource {
private static final long serialVersionUID = 22306216855466L;
/**
* Create a AvailableAddOnFetcher to execute fetch.
*
* @param pathSid The unique Available Add-on Sid
* @return AvailableAddOnFetcher capable of executing the fetch
*/
public static AvailableAddOnFetcher fetcher(final String pathSid) {
return new AvailableAddOnFetcher(pathSid);
}
/**
* Create a AvailableAddOnReader to execute read.
*
* @return AvailableAddOnReader capable of executing the read
*/
public static AvailableAddOnReader reader() {
return new AvailableAddOnReader();
}
/**
* Converts a JSON String into a AvailableAddOn object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return AvailableAddOn object represented by the provided JSON
*/
public static AvailableAddOn fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, AvailableAddOn.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a AvailableAddOn object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return AvailableAddOn object represented by the provided JSON
*/
public static AvailableAddOn fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, AvailableAddOn.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String friendlyName;
private final String description;
private final String pricingType;
private final Map configurationSchema;
private final URI url;
private final Map links;
@JsonCreator
private AvailableAddOn(@JsonProperty("sid")
final String sid,
@JsonProperty("friendly_name")
final String friendlyName,
@JsonProperty("description")
final String description,
@JsonProperty("pricing_type")
final String pricingType,
@JsonProperty("configuration_schema")
final Map configurationSchema,
@JsonProperty("url")
final URI url,
@JsonProperty("links")
final Map links) {
this.sid = sid;
this.friendlyName = friendlyName;
this.description = description;
this.pricingType = pricingType;
this.configurationSchema = configurationSchema;
this.url = url;
this.links = links;
}
/**
* Returns The A string that uniquely identifies this Add-on.
*
* @return A string that uniquely identifies this Add-on
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The A description of this Add-on.
*
* @return A description of this Add-on
*/
public final String getFriendlyName() {
return this.friendlyName;
}
/**
* Returns The A short description of the Add-on functionality.
*
* @return A short description of the Add-on functionality
*/
public final String getDescription() {
return this.description;
}
/**
* Returns The The way customers are charged for using this Add-on.
*
* @return The way customers are charged for using this Add-on
*/
public final String getPricingType() {
return this.pricingType;
}
/**
* Returns The The JSON Schema describing the Add-on's configuration.
*
* @return The JSON Schema describing the Add-on's configuration
*/
public final Map getConfigurationSchema() {
return this.configurationSchema;
}
/**
* Returns The The url.
*
* @return The url
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns The The links.
*
* @return The links
*/
public final Map getLinks() {
return this.links;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AvailableAddOn other = (AvailableAddOn) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(friendlyName, other.friendlyName) &&
Objects.equals(description, other.description) &&
Objects.equals(pricingType, other.pricingType) &&
Objects.equals(configurationSchema, other.configurationSchema) &&
Objects.equals(url, other.url) &&
Objects.equals(links, other.links);
}
@Override
public int hashCode() {
return Objects.hash(sid,
friendlyName,
description,
pricingType,
configurationSchema,
url,
links);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("friendlyName", friendlyName)
.add("description", description)
.add("pricingType", pricingType)
.add("configurationSchema", configurationSchema)
.add("url", url)
.add("links", links)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy