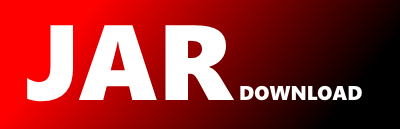
com.twilio.rest.api.v2010.account.sip.DomainUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account.sip;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
public class DomainUpdater extends Updater {
private String pathAccountSid;
private final String pathSid;
private String authType;
private String friendlyName;
private HttpMethod voiceFallbackMethod;
private URI voiceFallbackUrl;
private HttpMethod voiceMethod;
private HttpMethod voiceStatusCallbackMethod;
private URI voiceStatusCallbackUrl;
private URI voiceUrl;
private Boolean sipRegistration;
/**
* Construct a new DomainUpdater.
*
* @param pathSid The sid
*/
public DomainUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* Construct a new DomainUpdater.
*
* @param pathAccountSid The account_sid
* @param pathSid The sid
*/
public DomainUpdater(final String pathAccountSid,
final String pathSid) {
this.pathAccountSid = pathAccountSid;
this.pathSid = pathSid;
}
/**
* The auth_type.
*
* @param authType The auth_type
* @return this
*/
public DomainUpdater setAuthType(final String authType) {
this.authType = authType;
return this;
}
/**
* A user-specified, human-readable name for the trigger..
*
* @param friendlyName A user-specified, human-readable name for the trigger.
* @return this
*/
public DomainUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The voice_fallback_method.
*
* @param voiceFallbackMethod The voice_fallback_method
* @return this
*/
public DomainUpdater setVoiceFallbackMethod(final HttpMethod voiceFallbackMethod) {
this.voiceFallbackMethod = voiceFallbackMethod;
return this;
}
/**
* The voice_fallback_url.
*
* @param voiceFallbackUrl The voice_fallback_url
* @return this
*/
public DomainUpdater setVoiceFallbackUrl(final URI voiceFallbackUrl) {
this.voiceFallbackUrl = voiceFallbackUrl;
return this;
}
/**
* The voice_fallback_url.
*
* @param voiceFallbackUrl The voice_fallback_url
* @return this
*/
public DomainUpdater setVoiceFallbackUrl(final String voiceFallbackUrl) {
return setVoiceFallbackUrl(Promoter.uriFromString(voiceFallbackUrl));
}
/**
* The HTTP method to use with the voice_url.
*
* @param voiceMethod HTTP method to use with voice_url
* @return this
*/
public DomainUpdater setVoiceMethod(final HttpMethod voiceMethod) {
this.voiceMethod = voiceMethod;
return this;
}
/**
* The voice_status_callback_method.
*
* @param voiceStatusCallbackMethod The voice_status_callback_method
* @return this
*/
public DomainUpdater setVoiceStatusCallbackMethod(final HttpMethod voiceStatusCallbackMethod) {
this.voiceStatusCallbackMethod = voiceStatusCallbackMethod;
return this;
}
/**
* The voice_status_callback_url.
*
* @param voiceStatusCallbackUrl The voice_status_callback_url
* @return this
*/
public DomainUpdater setVoiceStatusCallbackUrl(final URI voiceStatusCallbackUrl) {
this.voiceStatusCallbackUrl = voiceStatusCallbackUrl;
return this;
}
/**
* The voice_status_callback_url.
*
* @param voiceStatusCallbackUrl The voice_status_callback_url
* @return this
*/
public DomainUpdater setVoiceStatusCallbackUrl(final String voiceStatusCallbackUrl) {
return setVoiceStatusCallbackUrl(Promoter.uriFromString(voiceStatusCallbackUrl));
}
/**
* The voice_url.
*
* @param voiceUrl The voice_url
* @return this
*/
public DomainUpdater setVoiceUrl(final URI voiceUrl) {
this.voiceUrl = voiceUrl;
return this;
}
/**
* The voice_url.
*
* @param voiceUrl The voice_url
* @return this
*/
public DomainUpdater setVoiceUrl(final String voiceUrl) {
return setVoiceUrl(Promoter.uriFromString(voiceUrl));
}
/**
* The sip_registration.
*
* @param sipRegistration The sip_registration
* @return this
*/
public DomainUpdater setSipRegistration(final Boolean sipRegistration) {
this.sipRegistration = sipRegistration;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Domain
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Domain update(final TwilioRestClient client) {
this.pathAccountSid = this.pathAccountSid == null ? client.getAccountSid() : this.pathAccountSid;
Request request = new Request(
HttpMethod.POST,
Domains.API.toString(),
"/2010-04-01/Accounts/" + this.pathAccountSid + "/SIP/Domains/" + this.pathSid + ".json",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Domain update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Domain.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (authType != null) {
request.addPostParam("AuthType", authType);
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (voiceFallbackMethod != null) {
request.addPostParam("VoiceFallbackMethod", voiceFallbackMethod.toString());
}
if (voiceFallbackUrl != null) {
request.addPostParam("VoiceFallbackUrl", voiceFallbackUrl.toString());
}
if (voiceMethod != null) {
request.addPostParam("VoiceMethod", voiceMethod.toString());
}
if (voiceStatusCallbackMethod != null) {
request.addPostParam("VoiceStatusCallbackMethod", voiceStatusCallbackMethod.toString());
}
if (voiceStatusCallbackUrl != null) {
request.addPostParam("VoiceStatusCallbackUrl", voiceStatusCallbackUrl.toString());
}
if (voiceUrl != null) {
request.addPostParam("VoiceUrl", voiceUrl.toString());
}
if (sipRegistration != null) {
request.addPostParam("SipRegistration", sipRegistration.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy