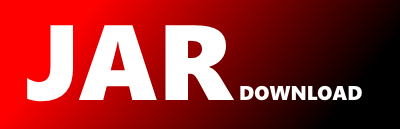
com.twilio.rest.ipmessaging.v1.ServiceUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.ipmessaging.v1;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
import java.util.List;
public class ServiceUpdater extends Updater {
private final String pathSid;
private String friendlyName;
private String defaultServiceRoleSid;
private String defaultChannelRoleSid;
private String defaultChannelCreatorRoleSid;
private Boolean readStatusEnabled;
private Boolean reachabilityEnabled;
private Integer typingIndicatorTimeout;
private Integer consumptionReportInterval;
private Boolean notificationsNewMessageEnabled;
private String notificationsNewMessageTemplate;
private Boolean notificationsAddedToChannelEnabled;
private String notificationsAddedToChannelTemplate;
private Boolean notificationsRemovedFromChannelEnabled;
private String notificationsRemovedFromChannelTemplate;
private Boolean notificationsInvitedToChannelEnabled;
private String notificationsInvitedToChannelTemplate;
private URI preWebhookUrl;
private URI postWebhookUrl;
private HttpMethod webhookMethod;
private List webhookFilters;
private URI webhooksOnMessageSendUrl;
private HttpMethod webhooksOnMessageSendMethod;
private String webhooksOnMessageSendFormat;
private URI webhooksOnMessageUpdateUrl;
private HttpMethod webhooksOnMessageUpdateMethod;
private String webhooksOnMessageUpdateFormat;
private URI webhooksOnMessageRemoveUrl;
private HttpMethod webhooksOnMessageRemoveMethod;
private String webhooksOnMessageRemoveFormat;
private URI webhooksOnChannelAddUrl;
private HttpMethod webhooksOnChannelAddMethod;
private String webhooksOnChannelAddFormat;
private URI webhooksOnChannelDestroyUrl;
private HttpMethod webhooksOnChannelDestroyMethod;
private String webhooksOnChannelDestroyFormat;
private URI webhooksOnChannelUpdateUrl;
private HttpMethod webhooksOnChannelUpdateMethod;
private String webhooksOnChannelUpdateFormat;
private URI webhooksOnMemberAddUrl;
private HttpMethod webhooksOnMemberAddMethod;
private String webhooksOnMemberAddFormat;
private URI webhooksOnMemberRemoveUrl;
private HttpMethod webhooksOnMemberRemoveMethod;
private String webhooksOnMemberRemoveFormat;
private URI webhooksOnMessageSentUrl;
private HttpMethod webhooksOnMessageSentMethod;
private String webhooksOnMessageSentFormat;
private URI webhooksOnMessageUpdatedUrl;
private HttpMethod webhooksOnMessageUpdatedMethod;
private String webhooksOnMessageUpdatedFormat;
private URI webhooksOnMessageRemovedUrl;
private HttpMethod webhooksOnMessageRemovedMethod;
private String webhooksOnMessageRemovedFormat;
private URI webhooksOnChannelAddedUrl;
private HttpMethod webhooksOnChannelAddedMethod;
private String webhooksOnChannelAddedFormat;
private URI webhooksOnChannelDestroyedUrl;
private HttpMethod webhooksOnChannelDestroyedMethod;
private String webhooksOnChannelDestroyedFormat;
private URI webhooksOnChannelUpdatedUrl;
private HttpMethod webhooksOnChannelUpdatedMethod;
private String webhooksOnChannelUpdatedFormat;
private URI webhooksOnMemberAddedUrl;
private HttpMethod webhooksOnMemberAddedMethod;
private String webhooksOnMemberAddedFormat;
private URI webhooksOnMemberRemovedUrl;
private HttpMethod webhooksOnMemberRemovedMethod;
private String webhooksOnMemberRemovedFormat;
private Integer limitsChannelMembers;
private Integer limitsUserChannels;
/**
* Construct a new ServiceUpdater.
*
* @param pathSid The sid
*/
public ServiceUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* Human-readable name for this service instance.
*
* @param friendlyName Human-readable name for this service instance
* @return this
*/
public ServiceUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The default_service_role_sid.
*
* @param defaultServiceRoleSid The default_service_role_sid
* @return this
*/
public ServiceUpdater setDefaultServiceRoleSid(final String defaultServiceRoleSid) {
this.defaultServiceRoleSid = defaultServiceRoleSid;
return this;
}
/**
* Channel role assigned on channel join (see
* [Roles](https://www.twilio.com/docs/api/chat/rest/v1/roles) data model for
* the details).
*
* @param defaultChannelRoleSid Channel role assigned on channel join
* @return this
*/
public ServiceUpdater setDefaultChannelRoleSid(final String defaultChannelRoleSid) {
this.defaultChannelRoleSid = defaultChannelRoleSid;
return this;
}
/**
* Channel role assigned to creator of channel when joining for first time.
*
* @param defaultChannelCreatorRoleSid Channel role assigned to creator of
* channel when joining for first time
* @return this
*/
public ServiceUpdater setDefaultChannelCreatorRoleSid(final String defaultChannelCreatorRoleSid) {
this.defaultChannelCreatorRoleSid = defaultChannelCreatorRoleSid;
return this;
}
/**
* `true` if the member read status feature is enabled, `false` if not.
* Defaults to `true`..
*
* @param readStatusEnabled true if the member read status feature is enabled,
* false if not.
* @return this
*/
public ServiceUpdater setReadStatusEnabled(final Boolean readStatusEnabled) {
this.readStatusEnabled = readStatusEnabled;
return this;
}
/**
* `true` if the reachability feature should be enabled. Defaults to `false`.
*
* @param reachabilityEnabled true if the reachability feature should be
* enabled.
* @return this
*/
public ServiceUpdater setReachabilityEnabled(final Boolean reachabilityEnabled) {
this.reachabilityEnabled = reachabilityEnabled;
return this;
}
/**
* ISO 8601 duration indicating the timeout after "started typing" event when
* client should assume that user is not typing anymore even if no "ended
* typing" message received.
*
* @param typingIndicatorTimeout ISO 8601 duration indicating the timeout after
* "started typing" event when client should
* assume that user is not typing anymore even if
* no "ended typing" message received
* @return this
*/
public ServiceUpdater setTypingIndicatorTimeout(final Integer typingIndicatorTimeout) {
this.typingIndicatorTimeout = typingIndicatorTimeout;
return this;
}
/**
* ISO 8601 duration indicating the interval between consumption reports sent
* from client endpoints..
*
* @param consumptionReportInterval ISO 8601 duration indicating the interval
* between consumption reports sent from client
* endpoints.
* @return this
*/
public ServiceUpdater setConsumptionReportInterval(final Integer consumptionReportInterval) {
this.consumptionReportInterval = consumptionReportInterval;
return this;
}
/**
* The notifications.new_message.enabled.
*
* @param notificationsNewMessageEnabled The notifications.new_message.enabled
* @return this
*/
public ServiceUpdater setNotificationsNewMessageEnabled(final Boolean notificationsNewMessageEnabled) {
this.notificationsNewMessageEnabled = notificationsNewMessageEnabled;
return this;
}
/**
* The notifications.new_message.template.
*
* @param notificationsNewMessageTemplate The notifications.new_message.template
* @return this
*/
public ServiceUpdater setNotificationsNewMessageTemplate(final String notificationsNewMessageTemplate) {
this.notificationsNewMessageTemplate = notificationsNewMessageTemplate;
return this;
}
/**
* The notifications.added_to_channel.enabled.
*
* @param notificationsAddedToChannelEnabled The
* notifications.added_to_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelEnabled(final Boolean notificationsAddedToChannelEnabled) {
this.notificationsAddedToChannelEnabled = notificationsAddedToChannelEnabled;
return this;
}
/**
* The notifications.added_to_channel.template.
*
* @param notificationsAddedToChannelTemplate The
* notifications.added_to_channel.template
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelTemplate(final String notificationsAddedToChannelTemplate) {
this.notificationsAddedToChannelTemplate = notificationsAddedToChannelTemplate;
return this;
}
/**
* The notifications.removed_from_channel.enabled.
*
* @param notificationsRemovedFromChannelEnabled The
* notifications.removed_from_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelEnabled(final Boolean notificationsRemovedFromChannelEnabled) {
this.notificationsRemovedFromChannelEnabled = notificationsRemovedFromChannelEnabled;
return this;
}
/**
* The notifications.removed_from_channel.template.
*
* @param notificationsRemovedFromChannelTemplate The
* notifications.removed_from_channel.template
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelTemplate(final String notificationsRemovedFromChannelTemplate) {
this.notificationsRemovedFromChannelTemplate = notificationsRemovedFromChannelTemplate;
return this;
}
/**
* The notifications.invited_to_channel.enabled.
*
* @param notificationsInvitedToChannelEnabled The
* notifications.invited_to_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelEnabled(final Boolean notificationsInvitedToChannelEnabled) {
this.notificationsInvitedToChannelEnabled = notificationsInvitedToChannelEnabled;
return this;
}
/**
* The notifications.invited_to_channel.template.
*
* @param notificationsInvitedToChannelTemplate The
* notifications.invited_to_channel.template
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelTemplate(final String notificationsInvitedToChannelTemplate) {
this.notificationsInvitedToChannelTemplate = notificationsInvitedToChannelTemplate;
return this;
}
/**
* The webhook URL for PRE-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param preWebhookUrl The webhook URL for PRE-Event webhooks.
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final URI preWebhookUrl) {
this.preWebhookUrl = preWebhookUrl;
return this;
}
/**
* The webhook URL for PRE-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param preWebhookUrl The webhook URL for PRE-Event webhooks.
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final String preWebhookUrl) {
return setPreWebhookUrl(Promoter.uriFromString(preWebhookUrl));
}
/**
* The webhook URL for POST-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param postWebhookUrl The webhook URL for POST-Event webhooks.
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final URI postWebhookUrl) {
this.postWebhookUrl = postWebhookUrl;
return this;
}
/**
* The webhook URL for POST-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param postWebhookUrl The webhook URL for POST-Event webhooks.
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final String postWebhookUrl) {
return setPostWebhookUrl(Promoter.uriFromString(postWebhookUrl));
}
/**
* The webhook request format to use. Must be POST or GET. See [Webhook
* Events](https://www.twilio.com/docs/api/chat/webhooks) for more details..
*
* @param webhookMethod The webhook request format to use.
* @return this
*/
public ServiceUpdater setWebhookMethod(final HttpMethod webhookMethod) {
this.webhookMethod = webhookMethod;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/api/chat/webhooks) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance.
* @return this
*/
public ServiceUpdater setWebhookFilters(final List webhookFilters) {
this.webhookFilters = webhookFilters;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/api/chat/webhooks) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance.
* @return this
*/
public ServiceUpdater setWebhookFilters(final String webhookFilters) {
return setWebhookFilters(Promoter.listOfOne(webhookFilters));
}
/**
* The webhooks.on_message_send.url.
*
* @param webhooksOnMessageSendUrl The webhooks.on_message_send.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendUrl(final URI webhooksOnMessageSendUrl) {
this.webhooksOnMessageSendUrl = webhooksOnMessageSendUrl;
return this;
}
/**
* The webhooks.on_message_send.url.
*
* @param webhooksOnMessageSendUrl The webhooks.on_message_send.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendUrl(final String webhooksOnMessageSendUrl) {
return setWebhooksOnMessageSendUrl(Promoter.uriFromString(webhooksOnMessageSendUrl));
}
/**
* The webhooks.on_message_send.method.
*
* @param webhooksOnMessageSendMethod The webhooks.on_message_send.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendMethod(final HttpMethod webhooksOnMessageSendMethod) {
this.webhooksOnMessageSendMethod = webhooksOnMessageSendMethod;
return this;
}
/**
* The webhooks.on_message_send.format.
*
* @param webhooksOnMessageSendFormat The webhooks.on_message_send.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSendFormat(final String webhooksOnMessageSendFormat) {
this.webhooksOnMessageSendFormat = webhooksOnMessageSendFormat;
return this;
}
/**
* The webhooks.on_message_update.url.
*
* @param webhooksOnMessageUpdateUrl The webhooks.on_message_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateUrl(final URI webhooksOnMessageUpdateUrl) {
this.webhooksOnMessageUpdateUrl = webhooksOnMessageUpdateUrl;
return this;
}
/**
* The webhooks.on_message_update.url.
*
* @param webhooksOnMessageUpdateUrl The webhooks.on_message_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateUrl(final String webhooksOnMessageUpdateUrl) {
return setWebhooksOnMessageUpdateUrl(Promoter.uriFromString(webhooksOnMessageUpdateUrl));
}
/**
* The webhooks.on_message_update.method.
*
* @param webhooksOnMessageUpdateMethod The webhooks.on_message_update.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateMethod(final HttpMethod webhooksOnMessageUpdateMethod) {
this.webhooksOnMessageUpdateMethod = webhooksOnMessageUpdateMethod;
return this;
}
/**
* The webhooks.on_message_update.format.
*
* @param webhooksOnMessageUpdateFormat The webhooks.on_message_update.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdateFormat(final String webhooksOnMessageUpdateFormat) {
this.webhooksOnMessageUpdateFormat = webhooksOnMessageUpdateFormat;
return this;
}
/**
* The webhooks.on_message_remove.url.
*
* @param webhooksOnMessageRemoveUrl The webhooks.on_message_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveUrl(final URI webhooksOnMessageRemoveUrl) {
this.webhooksOnMessageRemoveUrl = webhooksOnMessageRemoveUrl;
return this;
}
/**
* The webhooks.on_message_remove.url.
*
* @param webhooksOnMessageRemoveUrl The webhooks.on_message_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveUrl(final String webhooksOnMessageRemoveUrl) {
return setWebhooksOnMessageRemoveUrl(Promoter.uriFromString(webhooksOnMessageRemoveUrl));
}
/**
* The webhooks.on_message_remove.method.
*
* @param webhooksOnMessageRemoveMethod The webhooks.on_message_remove.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveMethod(final HttpMethod webhooksOnMessageRemoveMethod) {
this.webhooksOnMessageRemoveMethod = webhooksOnMessageRemoveMethod;
return this;
}
/**
* The webhooks.on_message_remove.format.
*
* @param webhooksOnMessageRemoveFormat The webhooks.on_message_remove.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemoveFormat(final String webhooksOnMessageRemoveFormat) {
this.webhooksOnMessageRemoveFormat = webhooksOnMessageRemoveFormat;
return this;
}
/**
* The webhooks.on_channel_add.url.
*
* @param webhooksOnChannelAddUrl The webhooks.on_channel_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddUrl(final URI webhooksOnChannelAddUrl) {
this.webhooksOnChannelAddUrl = webhooksOnChannelAddUrl;
return this;
}
/**
* The webhooks.on_channel_add.url.
*
* @param webhooksOnChannelAddUrl The webhooks.on_channel_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddUrl(final String webhooksOnChannelAddUrl) {
return setWebhooksOnChannelAddUrl(Promoter.uriFromString(webhooksOnChannelAddUrl));
}
/**
* The webhooks.on_channel_add.method.
*
* @param webhooksOnChannelAddMethod The webhooks.on_channel_add.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddMethod(final HttpMethod webhooksOnChannelAddMethod) {
this.webhooksOnChannelAddMethod = webhooksOnChannelAddMethod;
return this;
}
/**
* The webhooks.on_channel_add.format.
*
* @param webhooksOnChannelAddFormat The webhooks.on_channel_add.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddFormat(final String webhooksOnChannelAddFormat) {
this.webhooksOnChannelAddFormat = webhooksOnChannelAddFormat;
return this;
}
/**
* The webhooks.on_channel_destroy.url.
*
* @param webhooksOnChannelDestroyUrl The webhooks.on_channel_destroy.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyUrl(final URI webhooksOnChannelDestroyUrl) {
this.webhooksOnChannelDestroyUrl = webhooksOnChannelDestroyUrl;
return this;
}
/**
* The webhooks.on_channel_destroy.url.
*
* @param webhooksOnChannelDestroyUrl The webhooks.on_channel_destroy.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyUrl(final String webhooksOnChannelDestroyUrl) {
return setWebhooksOnChannelDestroyUrl(Promoter.uriFromString(webhooksOnChannelDestroyUrl));
}
/**
* The webhooks.on_channel_destroy.method.
*
* @param webhooksOnChannelDestroyMethod The webhooks.on_channel_destroy.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyMethod(final HttpMethod webhooksOnChannelDestroyMethod) {
this.webhooksOnChannelDestroyMethod = webhooksOnChannelDestroyMethod;
return this;
}
/**
* The webhooks.on_channel_destroy.format.
*
* @param webhooksOnChannelDestroyFormat The webhooks.on_channel_destroy.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyFormat(final String webhooksOnChannelDestroyFormat) {
this.webhooksOnChannelDestroyFormat = webhooksOnChannelDestroyFormat;
return this;
}
/**
* The webhooks.on_channel_update.url.
*
* @param webhooksOnChannelUpdateUrl The webhooks.on_channel_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateUrl(final URI webhooksOnChannelUpdateUrl) {
this.webhooksOnChannelUpdateUrl = webhooksOnChannelUpdateUrl;
return this;
}
/**
* The webhooks.on_channel_update.url.
*
* @param webhooksOnChannelUpdateUrl The webhooks.on_channel_update.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateUrl(final String webhooksOnChannelUpdateUrl) {
return setWebhooksOnChannelUpdateUrl(Promoter.uriFromString(webhooksOnChannelUpdateUrl));
}
/**
* The webhooks.on_channel_update.method.
*
* @param webhooksOnChannelUpdateMethod The webhooks.on_channel_update.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateMethod(final HttpMethod webhooksOnChannelUpdateMethod) {
this.webhooksOnChannelUpdateMethod = webhooksOnChannelUpdateMethod;
return this;
}
/**
* The webhooks.on_channel_update.format.
*
* @param webhooksOnChannelUpdateFormat The webhooks.on_channel_update.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdateFormat(final String webhooksOnChannelUpdateFormat) {
this.webhooksOnChannelUpdateFormat = webhooksOnChannelUpdateFormat;
return this;
}
/**
* The webhooks.on_member_add.url.
*
* @param webhooksOnMemberAddUrl The webhooks.on_member_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddUrl(final URI webhooksOnMemberAddUrl) {
this.webhooksOnMemberAddUrl = webhooksOnMemberAddUrl;
return this;
}
/**
* The webhooks.on_member_add.url.
*
* @param webhooksOnMemberAddUrl The webhooks.on_member_add.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddUrl(final String webhooksOnMemberAddUrl) {
return setWebhooksOnMemberAddUrl(Promoter.uriFromString(webhooksOnMemberAddUrl));
}
/**
* The webhooks.on_member_add.method.
*
* @param webhooksOnMemberAddMethod The webhooks.on_member_add.method
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddMethod(final HttpMethod webhooksOnMemberAddMethod) {
this.webhooksOnMemberAddMethod = webhooksOnMemberAddMethod;
return this;
}
/**
* The webhooks.on_member_add.format.
*
* @param webhooksOnMemberAddFormat The webhooks.on_member_add.format
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddFormat(final String webhooksOnMemberAddFormat) {
this.webhooksOnMemberAddFormat = webhooksOnMemberAddFormat;
return this;
}
/**
* The webhooks.on_member_remove.url.
*
* @param webhooksOnMemberRemoveUrl The webhooks.on_member_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveUrl(final URI webhooksOnMemberRemoveUrl) {
this.webhooksOnMemberRemoveUrl = webhooksOnMemberRemoveUrl;
return this;
}
/**
* The webhooks.on_member_remove.url.
*
* @param webhooksOnMemberRemoveUrl The webhooks.on_member_remove.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveUrl(final String webhooksOnMemberRemoveUrl) {
return setWebhooksOnMemberRemoveUrl(Promoter.uriFromString(webhooksOnMemberRemoveUrl));
}
/**
* The webhooks.on_member_remove.method.
*
* @param webhooksOnMemberRemoveMethod The webhooks.on_member_remove.method
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveMethod(final HttpMethod webhooksOnMemberRemoveMethod) {
this.webhooksOnMemberRemoveMethod = webhooksOnMemberRemoveMethod;
return this;
}
/**
* The webhooks.on_member_remove.format.
*
* @param webhooksOnMemberRemoveFormat The webhooks.on_member_remove.format
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemoveFormat(final String webhooksOnMemberRemoveFormat) {
this.webhooksOnMemberRemoveFormat = webhooksOnMemberRemoveFormat;
return this;
}
/**
* The webhooks.on_message_sent.url.
*
* @param webhooksOnMessageSentUrl The webhooks.on_message_sent.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentUrl(final URI webhooksOnMessageSentUrl) {
this.webhooksOnMessageSentUrl = webhooksOnMessageSentUrl;
return this;
}
/**
* The webhooks.on_message_sent.url.
*
* @param webhooksOnMessageSentUrl The webhooks.on_message_sent.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentUrl(final String webhooksOnMessageSentUrl) {
return setWebhooksOnMessageSentUrl(Promoter.uriFromString(webhooksOnMessageSentUrl));
}
/**
* The webhooks.on_message_sent.method.
*
* @param webhooksOnMessageSentMethod The webhooks.on_message_sent.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentMethod(final HttpMethod webhooksOnMessageSentMethod) {
this.webhooksOnMessageSentMethod = webhooksOnMessageSentMethod;
return this;
}
/**
* The webhooks.on_message_sent.format.
*
* @param webhooksOnMessageSentFormat The webhooks.on_message_sent.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageSentFormat(final String webhooksOnMessageSentFormat) {
this.webhooksOnMessageSentFormat = webhooksOnMessageSentFormat;
return this;
}
/**
* The webhooks.on_message_updated.url.
*
* @param webhooksOnMessageUpdatedUrl The webhooks.on_message_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedUrl(final URI webhooksOnMessageUpdatedUrl) {
this.webhooksOnMessageUpdatedUrl = webhooksOnMessageUpdatedUrl;
return this;
}
/**
* The webhooks.on_message_updated.url.
*
* @param webhooksOnMessageUpdatedUrl The webhooks.on_message_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedUrl(final String webhooksOnMessageUpdatedUrl) {
return setWebhooksOnMessageUpdatedUrl(Promoter.uriFromString(webhooksOnMessageUpdatedUrl));
}
/**
* The webhooks.on_message_updated.method.
*
* @param webhooksOnMessageUpdatedMethod The webhooks.on_message_updated.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedMethod(final HttpMethod webhooksOnMessageUpdatedMethod) {
this.webhooksOnMessageUpdatedMethod = webhooksOnMessageUpdatedMethod;
return this;
}
/**
* The webhooks.on_message_updated.format.
*
* @param webhooksOnMessageUpdatedFormat The webhooks.on_message_updated.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageUpdatedFormat(final String webhooksOnMessageUpdatedFormat) {
this.webhooksOnMessageUpdatedFormat = webhooksOnMessageUpdatedFormat;
return this;
}
/**
* The webhooks.on_message_removed.url.
*
* @param webhooksOnMessageRemovedUrl The webhooks.on_message_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedUrl(final URI webhooksOnMessageRemovedUrl) {
this.webhooksOnMessageRemovedUrl = webhooksOnMessageRemovedUrl;
return this;
}
/**
* The webhooks.on_message_removed.url.
*
* @param webhooksOnMessageRemovedUrl The webhooks.on_message_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedUrl(final String webhooksOnMessageRemovedUrl) {
return setWebhooksOnMessageRemovedUrl(Promoter.uriFromString(webhooksOnMessageRemovedUrl));
}
/**
* The webhooks.on_message_removed.method.
*
* @param webhooksOnMessageRemovedMethod The webhooks.on_message_removed.method
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedMethod(final HttpMethod webhooksOnMessageRemovedMethod) {
this.webhooksOnMessageRemovedMethod = webhooksOnMessageRemovedMethod;
return this;
}
/**
* The webhooks.on_message_removed.format.
*
* @param webhooksOnMessageRemovedFormat The webhooks.on_message_removed.format
* @return this
*/
public ServiceUpdater setWebhooksOnMessageRemovedFormat(final String webhooksOnMessageRemovedFormat) {
this.webhooksOnMessageRemovedFormat = webhooksOnMessageRemovedFormat;
return this;
}
/**
* The webhooks.on_channel_added.url.
*
* @param webhooksOnChannelAddedUrl The webhooks.on_channel_added.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedUrl(final URI webhooksOnChannelAddedUrl) {
this.webhooksOnChannelAddedUrl = webhooksOnChannelAddedUrl;
return this;
}
/**
* The webhooks.on_channel_added.url.
*
* @param webhooksOnChannelAddedUrl The webhooks.on_channel_added.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedUrl(final String webhooksOnChannelAddedUrl) {
return setWebhooksOnChannelAddedUrl(Promoter.uriFromString(webhooksOnChannelAddedUrl));
}
/**
* The webhooks.on_channel_added.method.
*
* @param webhooksOnChannelAddedMethod The webhooks.on_channel_added.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedMethod(final HttpMethod webhooksOnChannelAddedMethod) {
this.webhooksOnChannelAddedMethod = webhooksOnChannelAddedMethod;
return this;
}
/**
* The webhooks.on_channel_added.format.
*
* @param webhooksOnChannelAddedFormat The webhooks.on_channel_added.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelAddedFormat(final String webhooksOnChannelAddedFormat) {
this.webhooksOnChannelAddedFormat = webhooksOnChannelAddedFormat;
return this;
}
/**
* The webhooks.on_channel_destroyed.url.
*
* @param webhooksOnChannelDestroyedUrl The webhooks.on_channel_destroyed.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedUrl(final URI webhooksOnChannelDestroyedUrl) {
this.webhooksOnChannelDestroyedUrl = webhooksOnChannelDestroyedUrl;
return this;
}
/**
* The webhooks.on_channel_destroyed.url.
*
* @param webhooksOnChannelDestroyedUrl The webhooks.on_channel_destroyed.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedUrl(final String webhooksOnChannelDestroyedUrl) {
return setWebhooksOnChannelDestroyedUrl(Promoter.uriFromString(webhooksOnChannelDestroyedUrl));
}
/**
* The webhooks.on_channel_destroyed.method.
*
* @param webhooksOnChannelDestroyedMethod The
* webhooks.on_channel_destroyed.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedMethod(final HttpMethod webhooksOnChannelDestroyedMethod) {
this.webhooksOnChannelDestroyedMethod = webhooksOnChannelDestroyedMethod;
return this;
}
/**
* The webhooks.on_channel_destroyed.format.
*
* @param webhooksOnChannelDestroyedFormat The
* webhooks.on_channel_destroyed.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelDestroyedFormat(final String webhooksOnChannelDestroyedFormat) {
this.webhooksOnChannelDestroyedFormat = webhooksOnChannelDestroyedFormat;
return this;
}
/**
* The webhooks.on_channel_updated.url.
*
* @param webhooksOnChannelUpdatedUrl The webhooks.on_channel_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedUrl(final URI webhooksOnChannelUpdatedUrl) {
this.webhooksOnChannelUpdatedUrl = webhooksOnChannelUpdatedUrl;
return this;
}
/**
* The webhooks.on_channel_updated.url.
*
* @param webhooksOnChannelUpdatedUrl The webhooks.on_channel_updated.url
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedUrl(final String webhooksOnChannelUpdatedUrl) {
return setWebhooksOnChannelUpdatedUrl(Promoter.uriFromString(webhooksOnChannelUpdatedUrl));
}
/**
* The webhooks.on_channel_updated.method.
*
* @param webhooksOnChannelUpdatedMethod The webhooks.on_channel_updated.method
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedMethod(final HttpMethod webhooksOnChannelUpdatedMethod) {
this.webhooksOnChannelUpdatedMethod = webhooksOnChannelUpdatedMethod;
return this;
}
/**
* The webhooks.on_channel_updated.format.
*
* @param webhooksOnChannelUpdatedFormat The webhooks.on_channel_updated.format
* @return this
*/
public ServiceUpdater setWebhooksOnChannelUpdatedFormat(final String webhooksOnChannelUpdatedFormat) {
this.webhooksOnChannelUpdatedFormat = webhooksOnChannelUpdatedFormat;
return this;
}
/**
* The webhooks.on_member_added.url.
*
* @param webhooksOnMemberAddedUrl The webhooks.on_member_added.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedUrl(final URI webhooksOnMemberAddedUrl) {
this.webhooksOnMemberAddedUrl = webhooksOnMemberAddedUrl;
return this;
}
/**
* The webhooks.on_member_added.url.
*
* @param webhooksOnMemberAddedUrl The webhooks.on_member_added.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedUrl(final String webhooksOnMemberAddedUrl) {
return setWebhooksOnMemberAddedUrl(Promoter.uriFromString(webhooksOnMemberAddedUrl));
}
/**
* The webhooks.on_member_added.method.
*
* @param webhooksOnMemberAddedMethod The webhooks.on_member_added.method
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedMethod(final HttpMethod webhooksOnMemberAddedMethod) {
this.webhooksOnMemberAddedMethod = webhooksOnMemberAddedMethod;
return this;
}
/**
* The webhooks.on_member_added.format.
*
* @param webhooksOnMemberAddedFormat The webhooks.on_member_added.format
* @return this
*/
public ServiceUpdater setWebhooksOnMemberAddedFormat(final String webhooksOnMemberAddedFormat) {
this.webhooksOnMemberAddedFormat = webhooksOnMemberAddedFormat;
return this;
}
/**
* The webhooks.on_member_removed.url.
*
* @param webhooksOnMemberRemovedUrl The webhooks.on_member_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedUrl(final URI webhooksOnMemberRemovedUrl) {
this.webhooksOnMemberRemovedUrl = webhooksOnMemberRemovedUrl;
return this;
}
/**
* The webhooks.on_member_removed.url.
*
* @param webhooksOnMemberRemovedUrl The webhooks.on_member_removed.url
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedUrl(final String webhooksOnMemberRemovedUrl) {
return setWebhooksOnMemberRemovedUrl(Promoter.uriFromString(webhooksOnMemberRemovedUrl));
}
/**
* The webhooks.on_member_removed.method.
*
* @param webhooksOnMemberRemovedMethod The webhooks.on_member_removed.method
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedMethod(final HttpMethod webhooksOnMemberRemovedMethod) {
this.webhooksOnMemberRemovedMethod = webhooksOnMemberRemovedMethod;
return this;
}
/**
* The webhooks.on_member_removed.format.
*
* @param webhooksOnMemberRemovedFormat The webhooks.on_member_removed.format
* @return this
*/
public ServiceUpdater setWebhooksOnMemberRemovedFormat(final String webhooksOnMemberRemovedFormat) {
this.webhooksOnMemberRemovedFormat = webhooksOnMemberRemovedFormat;
return this;
}
/**
* The limits.channel_members.
*
* @param limitsChannelMembers The limits.channel_members
* @return this
*/
public ServiceUpdater setLimitsChannelMembers(final Integer limitsChannelMembers) {
this.limitsChannelMembers = limitsChannelMembers;
return this;
}
/**
* The limits.user_channels.
*
* @param limitsUserChannels The limits.user_channels
* @return this
*/
public ServiceUpdater setLimitsUserChannels(final Integer limitsUserChannels) {
this.limitsUserChannels = limitsUserChannels;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Service
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Service update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.IPMESSAGING.toString(),
"/v1/Services/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Service update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Service.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (defaultServiceRoleSid != null) {
request.addPostParam("DefaultServiceRoleSid", defaultServiceRoleSid);
}
if (defaultChannelRoleSid != null) {
request.addPostParam("DefaultChannelRoleSid", defaultChannelRoleSid);
}
if (defaultChannelCreatorRoleSid != null) {
request.addPostParam("DefaultChannelCreatorRoleSid", defaultChannelCreatorRoleSid);
}
if (readStatusEnabled != null) {
request.addPostParam("ReadStatusEnabled", readStatusEnabled.toString());
}
if (reachabilityEnabled != null) {
request.addPostParam("ReachabilityEnabled", reachabilityEnabled.toString());
}
if (typingIndicatorTimeout != null) {
request.addPostParam("TypingIndicatorTimeout", typingIndicatorTimeout.toString());
}
if (consumptionReportInterval != null) {
request.addPostParam("ConsumptionReportInterval", consumptionReportInterval.toString());
}
if (notificationsNewMessageEnabled != null) {
request.addPostParam("Notifications.NewMessage.Enabled", notificationsNewMessageEnabled.toString());
}
if (notificationsNewMessageTemplate != null) {
request.addPostParam("Notifications.NewMessage.Template", notificationsNewMessageTemplate);
}
if (notificationsAddedToChannelEnabled != null) {
request.addPostParam("Notifications.AddedToChannel.Enabled", notificationsAddedToChannelEnabled.toString());
}
if (notificationsAddedToChannelTemplate != null) {
request.addPostParam("Notifications.AddedToChannel.Template", notificationsAddedToChannelTemplate);
}
if (notificationsRemovedFromChannelEnabled != null) {
request.addPostParam("Notifications.RemovedFromChannel.Enabled", notificationsRemovedFromChannelEnabled.toString());
}
if (notificationsRemovedFromChannelTemplate != null) {
request.addPostParam("Notifications.RemovedFromChannel.Template", notificationsRemovedFromChannelTemplate);
}
if (notificationsInvitedToChannelEnabled != null) {
request.addPostParam("Notifications.InvitedToChannel.Enabled", notificationsInvitedToChannelEnabled.toString());
}
if (notificationsInvitedToChannelTemplate != null) {
request.addPostParam("Notifications.InvitedToChannel.Template", notificationsInvitedToChannelTemplate);
}
if (preWebhookUrl != null) {
request.addPostParam("PreWebhookUrl", preWebhookUrl.toString());
}
if (postWebhookUrl != null) {
request.addPostParam("PostWebhookUrl", postWebhookUrl.toString());
}
if (webhookMethod != null) {
request.addPostParam("WebhookMethod", webhookMethod.toString());
}
if (webhookFilters != null) {
for (String prop : webhookFilters) {
request.addPostParam("WebhookFilters", prop);
}
}
if (webhooksOnMessageSendUrl != null) {
request.addPostParam("Webhooks.OnMessageSend.Url", webhooksOnMessageSendUrl.toString());
}
if (webhooksOnMessageSendMethod != null) {
request.addPostParam("Webhooks.OnMessageSend.Method", webhooksOnMessageSendMethod.toString());
}
if (webhooksOnMessageSendFormat != null) {
request.addPostParam("Webhooks.OnMessageSend.Format", webhooksOnMessageSendFormat);
}
if (webhooksOnMessageUpdateUrl != null) {
request.addPostParam("Webhooks.OnMessageUpdate.Url", webhooksOnMessageUpdateUrl.toString());
}
if (webhooksOnMessageUpdateMethod != null) {
request.addPostParam("Webhooks.OnMessageUpdate.Method", webhooksOnMessageUpdateMethod.toString());
}
if (webhooksOnMessageUpdateFormat != null) {
request.addPostParam("Webhooks.OnMessageUpdate.Format", webhooksOnMessageUpdateFormat);
}
if (webhooksOnMessageRemoveUrl != null) {
request.addPostParam("Webhooks.OnMessageRemove.Url", webhooksOnMessageRemoveUrl.toString());
}
if (webhooksOnMessageRemoveMethod != null) {
request.addPostParam("Webhooks.OnMessageRemove.Method", webhooksOnMessageRemoveMethod.toString());
}
if (webhooksOnMessageRemoveFormat != null) {
request.addPostParam("Webhooks.OnMessageRemove.Format", webhooksOnMessageRemoveFormat);
}
if (webhooksOnChannelAddUrl != null) {
request.addPostParam("Webhooks.OnChannelAdd.Url", webhooksOnChannelAddUrl.toString());
}
if (webhooksOnChannelAddMethod != null) {
request.addPostParam("Webhooks.OnChannelAdd.Method", webhooksOnChannelAddMethod.toString());
}
if (webhooksOnChannelAddFormat != null) {
request.addPostParam("Webhooks.OnChannelAdd.Format", webhooksOnChannelAddFormat);
}
if (webhooksOnChannelDestroyUrl != null) {
request.addPostParam("Webhooks.OnChannelDestroy.Url", webhooksOnChannelDestroyUrl.toString());
}
if (webhooksOnChannelDestroyMethod != null) {
request.addPostParam("Webhooks.OnChannelDestroy.Method", webhooksOnChannelDestroyMethod.toString());
}
if (webhooksOnChannelDestroyFormat != null) {
request.addPostParam("Webhooks.OnChannelDestroy.Format", webhooksOnChannelDestroyFormat);
}
if (webhooksOnChannelUpdateUrl != null) {
request.addPostParam("Webhooks.OnChannelUpdate.Url", webhooksOnChannelUpdateUrl.toString());
}
if (webhooksOnChannelUpdateMethod != null) {
request.addPostParam("Webhooks.OnChannelUpdate.Method", webhooksOnChannelUpdateMethod.toString());
}
if (webhooksOnChannelUpdateFormat != null) {
request.addPostParam("Webhooks.OnChannelUpdate.Format", webhooksOnChannelUpdateFormat);
}
if (webhooksOnMemberAddUrl != null) {
request.addPostParam("Webhooks.OnMemberAdd.Url", webhooksOnMemberAddUrl.toString());
}
if (webhooksOnMemberAddMethod != null) {
request.addPostParam("Webhooks.OnMemberAdd.Method", webhooksOnMemberAddMethod.toString());
}
if (webhooksOnMemberAddFormat != null) {
request.addPostParam("Webhooks.OnMemberAdd.Format", webhooksOnMemberAddFormat);
}
if (webhooksOnMemberRemoveUrl != null) {
request.addPostParam("Webhooks.OnMemberRemove.Url", webhooksOnMemberRemoveUrl.toString());
}
if (webhooksOnMemberRemoveMethod != null) {
request.addPostParam("Webhooks.OnMemberRemove.Method", webhooksOnMemberRemoveMethod.toString());
}
if (webhooksOnMemberRemoveFormat != null) {
request.addPostParam("Webhooks.OnMemberRemove.Format", webhooksOnMemberRemoveFormat);
}
if (webhooksOnMessageSentUrl != null) {
request.addPostParam("Webhooks.OnMessageSent.Url", webhooksOnMessageSentUrl.toString());
}
if (webhooksOnMessageSentMethod != null) {
request.addPostParam("Webhooks.OnMessageSent.Method", webhooksOnMessageSentMethod.toString());
}
if (webhooksOnMessageSentFormat != null) {
request.addPostParam("Webhooks.OnMessageSent.Format", webhooksOnMessageSentFormat);
}
if (webhooksOnMessageUpdatedUrl != null) {
request.addPostParam("Webhooks.OnMessageUpdated.Url", webhooksOnMessageUpdatedUrl.toString());
}
if (webhooksOnMessageUpdatedMethod != null) {
request.addPostParam("Webhooks.OnMessageUpdated.Method", webhooksOnMessageUpdatedMethod.toString());
}
if (webhooksOnMessageUpdatedFormat != null) {
request.addPostParam("Webhooks.OnMessageUpdated.Format", webhooksOnMessageUpdatedFormat);
}
if (webhooksOnMessageRemovedUrl != null) {
request.addPostParam("Webhooks.OnMessageRemoved.Url", webhooksOnMessageRemovedUrl.toString());
}
if (webhooksOnMessageRemovedMethod != null) {
request.addPostParam("Webhooks.OnMessageRemoved.Method", webhooksOnMessageRemovedMethod.toString());
}
if (webhooksOnMessageRemovedFormat != null) {
request.addPostParam("Webhooks.OnMessageRemoved.Format", webhooksOnMessageRemovedFormat);
}
if (webhooksOnChannelAddedUrl != null) {
request.addPostParam("Webhooks.OnChannelAdded.Url", webhooksOnChannelAddedUrl.toString());
}
if (webhooksOnChannelAddedMethod != null) {
request.addPostParam("Webhooks.OnChannelAdded.Method", webhooksOnChannelAddedMethod.toString());
}
if (webhooksOnChannelAddedFormat != null) {
request.addPostParam("Webhooks.OnChannelAdded.Format", webhooksOnChannelAddedFormat);
}
if (webhooksOnChannelDestroyedUrl != null) {
request.addPostParam("Webhooks.OnChannelDestroyed.Url", webhooksOnChannelDestroyedUrl.toString());
}
if (webhooksOnChannelDestroyedMethod != null) {
request.addPostParam("Webhooks.OnChannelDestroyed.Method", webhooksOnChannelDestroyedMethod.toString());
}
if (webhooksOnChannelDestroyedFormat != null) {
request.addPostParam("Webhooks.OnChannelDestroyed.Format", webhooksOnChannelDestroyedFormat);
}
if (webhooksOnChannelUpdatedUrl != null) {
request.addPostParam("Webhooks.OnChannelUpdated.Url", webhooksOnChannelUpdatedUrl.toString());
}
if (webhooksOnChannelUpdatedMethod != null) {
request.addPostParam("Webhooks.OnChannelUpdated.Method", webhooksOnChannelUpdatedMethod.toString());
}
if (webhooksOnChannelUpdatedFormat != null) {
request.addPostParam("Webhooks.OnChannelUpdated.Format", webhooksOnChannelUpdatedFormat);
}
if (webhooksOnMemberAddedUrl != null) {
request.addPostParam("Webhooks.OnMemberAdded.Url", webhooksOnMemberAddedUrl.toString());
}
if (webhooksOnMemberAddedMethod != null) {
request.addPostParam("Webhooks.OnMemberAdded.Method", webhooksOnMemberAddedMethod.toString());
}
if (webhooksOnMemberAddedFormat != null) {
request.addPostParam("Webhooks.OnMemberAdded.Format", webhooksOnMemberAddedFormat);
}
if (webhooksOnMemberRemovedUrl != null) {
request.addPostParam("Webhooks.OnMemberRemoved.Url", webhooksOnMemberRemovedUrl.toString());
}
if (webhooksOnMemberRemovedMethod != null) {
request.addPostParam("Webhooks.OnMemberRemoved.Method", webhooksOnMemberRemovedMethod.toString());
}
if (webhooksOnMemberRemovedFormat != null) {
request.addPostParam("Webhooks.OnMemberRemoved.Format", webhooksOnMemberRemovedFormat);
}
if (limitsChannelMembers != null) {
request.addPostParam("Limits.ChannelMembers", limitsChannelMembers.toString());
}
if (limitsUserChannels != null) {
request.addPostParam("Limits.UserChannels", limitsUserChannels.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy