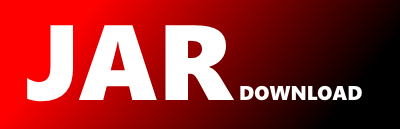
com.twilio.rest.notify.v1.service.UserCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.notify.v1.service;
import com.twilio.base.Creator;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.util.List;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
public class UserCreator extends Creator {
private final String pathServiceSid;
private final String identity;
private List segment;
/**
* Construct a new UserCreator.
*
* @param pathServiceSid The service_sid
* @param identity The identifier of the User, defined by your application.
*/
public UserCreator(final String pathServiceSid,
final String identity) {
this.pathServiceSid = pathServiceSid;
this.identity = identity;
}
/**
* The list of segments this User belongs to. Segments can be used to select
* recipients of a notification. Maximum 20 Segments per User allowed..
*
* @param segment The list of segments this User belongs to. Segments can be
* used to select recipients of a notification. Maximum 20
* Segments per User allowed.
* @return this
*/
public UserCreator setSegment(final List segment) {
this.segment = segment;
return this;
}
/**
* The list of segments this User belongs to. Segments can be used to select
* recipients of a notification. Maximum 20 Segments per User allowed..
*
* @param segment The list of segments this User belongs to. Segments can be
* used to select recipients of a notification. Maximum 20
* Segments per User allowed.
* @return this
*/
public UserCreator setSegment(final String segment) {
return setSegment(Promoter.listOfOne(segment));
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created User
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public User create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.NOTIFY.toString(),
"/v1/Services/" + this.pathServiceSid + "/Users",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("User creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return User.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (identity != null) {
request.addPostParam("Identity", identity);
}
if (segment != null) {
for (String prop : segment) {
request.addPostParam("Segment", prop);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy