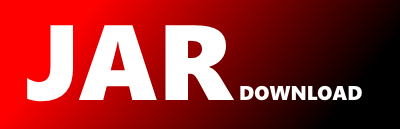
com.twilio.rest.api.v2010.account.conference.ParticipantCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account.conference;
import com.twilio.base.Creator;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
import java.util.List;
public class ParticipantCreator extends Creator {
private String pathAccountSid;
private final String pathConferenceSid;
private final com.twilio.type.PhoneNumber from;
private final com.twilio.type.PhoneNumber to;
private URI statusCallback;
private HttpMethod statusCallbackMethod;
private List statusCallbackEvent;
private Integer timeout;
private Boolean record;
private Boolean muted;
private String beep;
private Boolean startConferenceOnEnter;
private Boolean endConferenceOnExit;
private URI waitUrl;
private HttpMethod waitMethod;
private Boolean earlyMedia;
private Integer maxParticipants;
private String conferenceRecord;
private String conferenceTrim;
private URI conferenceStatusCallback;
private HttpMethod conferenceStatusCallbackMethod;
private List conferenceStatusCallbackEvent;
private String recordingChannels;
private URI recordingStatusCallback;
private HttpMethod recordingStatusCallbackMethod;
private String sipAuthUsername;
private String sipAuthPassword;
private String region;
private URI conferenceRecordingStatusCallback;
private HttpMethod conferenceRecordingStatusCallbackMethod;
private List recordingStatusCallbackEvent;
private List conferenceRecordingStatusCallbackEvent;
/**
* Construct a new ParticipantCreator.
*
* @param pathConferenceSid The conference_sid
* @param from The `from` phone number used to invite a participant.
* @param to The number, client id, or sip address of the new participant.
*/
public ParticipantCreator(final String pathConferenceSid,
final com.twilio.type.PhoneNumber from,
final com.twilio.type.PhoneNumber to) {
this.pathConferenceSid = pathConferenceSid;
this.from = from;
this.to = to;
}
/**
* Construct a new ParticipantCreator.
*
* @param pathAccountSid The account_sid
* @param pathConferenceSid The conference_sid
* @param from The `from` phone number used to invite a participant.
* @param to The number, client id, or sip address of the new participant.
*/
public ParticipantCreator(final String pathAccountSid,
final String pathConferenceSid,
final com.twilio.type.PhoneNumber from,
final com.twilio.type.PhoneNumber to) {
this.pathAccountSid = pathAccountSid;
this.pathConferenceSid = pathConferenceSid;
this.from = from;
this.to = to;
}
/**
* The absolute URL where Twilio should send a webhook with conference event
* information that you request with the `StatusCallbackEvent` parameter..
*
* @param statusCallback URL for conference event callback.
* @return this
*/
public ParticipantCreator setStatusCallback(final URI statusCallback) {
this.statusCallback = statusCallback;
return this;
}
/**
* The absolute URL where Twilio should send a webhook with conference event
* information that you request with the `StatusCallbackEvent` parameter..
*
* @param statusCallback URL for conference event callback.
* @return this
*/
public ParticipantCreator setStatusCallback(final String statusCallback) {
return setStatusCallback(Promoter.uriFromString(statusCallback));
}
/**
* The method Twilio should use when requesting your `StatusCallback` URL.
* Options are `GET` and `POST`. Defaults to `POST`..
*
* @param statusCallbackMethod Method Twilio should use to reach the status
* callback URL.
* @return this
*/
public ParticipantCreator setStatusCallbackMethod(final HttpMethod statusCallbackMethod) {
this.statusCallbackMethod = statusCallbackMethod;
return this;
}
/**
* Specifies which conference state changes should generate a webhook to the
* `StatusCallback` URL. Options are `initiated`, `ringing`, `answered`, and
* `completed`. To specify multiple values, separate each with a space. Defaults
* to `completed`..
*
* @param statusCallbackEvent Set state change events that will trigger a
* callback.
* @return this
*/
public ParticipantCreator setStatusCallbackEvent(final List statusCallbackEvent) {
this.statusCallbackEvent = statusCallbackEvent;
return this;
}
/**
* Specifies which conference state changes should generate a webhook to the
* `StatusCallback` URL. Options are `initiated`, `ringing`, `answered`, and
* `completed`. To specify multiple values, separate each with a space. Defaults
* to `completed`..
*
* @param statusCallbackEvent Set state change events that will trigger a
* callback.
* @return this
*/
public ParticipantCreator setStatusCallbackEvent(final String statusCallbackEvent) {
return setStatusCallbackEvent(Promoter.listOfOne(statusCallbackEvent));
}
/**
* The number of seconds (integer) that Twilio should allow the phone to ring
* before assuming there is no answer. Defaults to 60 seconds. Minimum allowed
* timeout is `5`, max is `600`. Twilio always adds a 5-second timeout buffer to
* outgoing calls, so if you enter a timeout value of 10 seconds, you will see
* an actual timeout closer to 15 seconds..
*
* @param timeout Number of seconds Twilio will wait for an answer.
* @return this
*/
public ParticipantCreator setTimeout(final Integer timeout) {
this.timeout = timeout;
return this;
}
/**
* Records the agent and their conferences, including downtime between
* conferences. Values may be `true` or `false`. Defaults to `false`..
*
* @param record Record the agent and their conferences.
* @return this
*/
public ParticipantCreator setRecord(final Boolean record) {
this.record = record;
return this;
}
/**
* Specify whether the agent can speak in the conference. Values can be `true`
* or `false`. Defaults to `false`..
*
* @param muted Mute the agent.
* @return this
*/
public ParticipantCreator setMuted(final Boolean muted) {
this.muted = muted;
return this;
}
/**
* Play a notification beep to the conference when this participant joins.
* Options are `true`, `false`, `onEnter`, or `onExit`. Defaults to `true`..
*
* @param beep Play a beep when the participant joins the conference.
* @return this
*/
public ParticipantCreator setBeep(final String beep) {
this.beep = beep;
return this;
}
/**
* If the conference has not already begun, `true` will start the conference
* when this participant joins. Specifying `false` will mute the participant and
* play background music until the conference begins. Defaults to `true`..
*
* @param startConferenceOnEnter Begin the conference when the participant
* joins.
* @return this
*/
public ParticipantCreator setStartConferenceOnEnter(final Boolean startConferenceOnEnter) {
this.startConferenceOnEnter = startConferenceOnEnter;
return this;
}
/**
* If `true`, will end the conference when this participant leaves. Defaults to
* `false`..
*
* @param endConferenceOnExit End the conference when the participant leaves.
* @return this
*/
public ParticipantCreator setEndConferenceOnExit(final Boolean endConferenceOnExit) {
this.endConferenceOnExit = endConferenceOnExit;
return this;
}
/**
* Specify an absolute URL that hosts music to play before the conference
* starts. Defualts to Twilio's standard [hold
* music](https://www.twilio.com/labs/twimlets/holdmusic)..
*
* @param waitUrl URL that hosts pre-conference hold music
* @return this
*/
public ParticipantCreator setWaitUrl(final URI waitUrl) {
this.waitUrl = waitUrl;
return this;
}
/**
* Specify an absolute URL that hosts music to play before the conference
* starts. Defualts to Twilio's standard [hold
* music](https://www.twilio.com/labs/twimlets/holdmusic)..
*
* @param waitUrl URL that hosts pre-conference hold music
* @return this
*/
public ParticipantCreator setWaitUrl(final String waitUrl) {
return setWaitUrl(Promoter.uriFromString(waitUrl));
}
/**
* Specify which method, `GET` or `POST`, Twilio should use to request the
* `WaitUrl` for this conference. Be sure to use `GET` if you are directly
* requesting static audio files so that Twilio properly caches the files.
* Defaults to `POST`..
*
* @param waitMethod The method Twilio should use to request `WaitUrl`.
* @return this
*/
public ParticipantCreator setWaitMethod(final HttpMethod waitMethod) {
this.waitMethod = waitMethod;
return this;
}
/**
* Allow an agent to hear the state of the outbound call, including ringing or
* disconnect messages. Can be `true` or `false`. Defaults to `true`..
*
* @param earlyMedia Agents can hear the state of the outbound call.
* @return this
*/
public ParticipantCreator setEarlyMedia(final Boolean earlyMedia) {
this.earlyMedia = earlyMedia;
return this;
}
/**
* The maximum number of participants within this agent conference. Values can
* be positive integers from `2`-`10`. Defaults to `10`..
*
* @param maxParticipants Maximum number of agent conference participants.
* @return this
*/
public ParticipantCreator setMaxParticipants(final Integer maxParticipants) {
this.maxParticipants = maxParticipants;
return this;
}
/**
* Records the conference that this participant is joining. Options are `true`,
* `false`, `record-from-start`, and `do-not-record`. Deafults to `false`.
*
* @param conferenceRecord Record the conference.
* @return this
*/
public ParticipantCreator setConferenceRecord(final String conferenceRecord) {
this.conferenceRecord = conferenceRecord;
return this;
}
/**
* Specify whether to trim leading and trailing silence from your recorded
* conference audio files. Options are `trim-silence` and `do-not-trim`.
* Defaults to `trim-silence`.
*
* @param conferenceTrim Trim silence from audio files.
* @return this
*/
public ParticipantCreator setConferenceTrim(final String conferenceTrim) {
this.conferenceTrim = conferenceTrim;
return this;
}
/**
* The absolute URL Twilio should request with conference events specified in
* `ConferenceStatusCallbackEvent`. This value is set by the first Participant
* to join the conference, and subsequent callback URLs will be ignored..
*
* @param conferenceStatusCallback Callback URL for conference events.
* @return this
*/
public ParticipantCreator setConferenceStatusCallback(final URI conferenceStatusCallback) {
this.conferenceStatusCallback = conferenceStatusCallback;
return this;
}
/**
* The absolute URL Twilio should request with conference events specified in
* `ConferenceStatusCallbackEvent`. This value is set by the first Participant
* to join the conference, and subsequent callback URLs will be ignored..
*
* @param conferenceStatusCallback Callback URL for conference events.
* @return this
*/
public ParticipantCreator setConferenceStatusCallback(final String conferenceStatusCallback) {
return setConferenceStatusCallback(Promoter.uriFromString(conferenceStatusCallback));
}
/**
* The HTTP method Twilio should use when requesting the
* `ConferenceStatusCallback` URL. Either `GET` or `POST`. Defaults to `POST`..
*
* @param conferenceStatusCallbackMethod HTTP method for requesting
* `ConferenceStatusCallback` URL.
* @return this
*/
public ParticipantCreator setConferenceStatusCallbackMethod(final HttpMethod conferenceStatusCallbackMethod) {
this.conferenceStatusCallbackMethod = conferenceStatusCallbackMethod;
return this;
}
/**
* Specifies which conference state changes should generate a webhook to the URL
* specified in the `ConferenceStatusCallback` attribute. Available values are
* `start`, `end`, `join`, `leave`, `mute`, `hold`, and `speaker`. To specify
* multiple values, separate them with a space. Defaults to `start` and `end`..
*
* @param conferenceStatusCallbackEvent Set which conference state changes
* should webhook to the
* `ConferenceStatusCallback`
* @return this
*/
public ParticipantCreator setConferenceStatusCallbackEvent(final List conferenceStatusCallbackEvent) {
this.conferenceStatusCallbackEvent = conferenceStatusCallbackEvent;
return this;
}
/**
* Specifies which conference state changes should generate a webhook to the URL
* specified in the `ConferenceStatusCallback` attribute. Available values are
* `start`, `end`, `join`, `leave`, `mute`, `hold`, and `speaker`. To specify
* multiple values, separate them with a space. Defaults to `start` and `end`..
*
* @param conferenceStatusCallbackEvent Set which conference state changes
* should webhook to the
* `ConferenceStatusCallback`
* @return this
*/
public ParticipantCreator setConferenceStatusCallbackEvent(final String conferenceStatusCallbackEvent) {
return setConferenceStatusCallbackEvent(Promoter.listOfOne(conferenceStatusCallbackEvent));
}
/**
* Set the recording channels for the final agent/conference recording. Either
* `mono` or `dual`. Defaults to `mono`..
*
* @param recordingChannels Specify `mono` or `dual` recording channels.
* @return this
*/
public ParticipantCreator setRecordingChannels(final String recordingChannels) {
this.recordingChannels = recordingChannels;
return this;
}
/**
* Specifies the `absolute URL` that Twilio should request when the recording is
* available if the agent and conference are recorded..
*
* @param recordingStatusCallback The absolute URL for Twilio's webhook with
* recording status information.
* @return this
*/
public ParticipantCreator setRecordingStatusCallback(final URI recordingStatusCallback) {
this.recordingStatusCallback = recordingStatusCallback;
return this;
}
/**
* Specifies the `absolute URL` that Twilio should request when the recording is
* available if the agent and conference are recorded..
*
* @param recordingStatusCallback The absolute URL for Twilio's webhook with
* recording status information.
* @return this
*/
public ParticipantCreator setRecordingStatusCallback(final String recordingStatusCallback) {
return setRecordingStatusCallback(Promoter.uriFromString(recordingStatusCallback));
}
/**
* The HTTP method Twilio should use when requesting the
* `RecordingStatusCallback`. `GET` or `POST`. Defaults to `POST`..
*
* @param recordingStatusCallbackMethod HTTP method for
* `RecordingStatusCallback`
* @return this
*/
public ParticipantCreator setRecordingStatusCallbackMethod(final HttpMethod recordingStatusCallbackMethod) {
this.recordingStatusCallbackMethod = recordingStatusCallbackMethod;
return this;
}
/**
* SIP username used for authenticating..
*
* @param sipAuthUsername SIP username used for authenticating.
* @return this
*/
public ParticipantCreator setSipAuthUsername(final String sipAuthUsername) {
this.sipAuthUsername = sipAuthUsername;
return this;
}
/**
* SIP password for authentication..
*
* @param sipAuthPassword SIP password for authentication.
* @return this
*/
public ParticipantCreator setSipAuthPassword(final String sipAuthPassword) {
this.sipAuthPassword = sipAuthPassword;
return this;
}
/**
* Specifies the
* [region](https://support.twilio.com/hc/en-us/articles/223132167-How-global-low-latency-routing-and-region-selection-work-for-conferences-and-Client-calls) where Twilio should mix the recorded audio. Options are `us1`, `ie1`, `de1`, `sg1`, `br1`, `au1`, `jp1`..
*
* @param region The region where Twilio should mix the conference audio.
* @return this
*/
public ParticipantCreator setRegion(final String region) {
this.region = region;
return this;
}
/**
* The `absolute URL` Twilio should request when the conference recording is
* available..
*
* @param conferenceRecordingStatusCallback Conference recording callback URL.
* @return this
*/
public ParticipantCreator setConferenceRecordingStatusCallback(final URI conferenceRecordingStatusCallback) {
this.conferenceRecordingStatusCallback = conferenceRecordingStatusCallback;
return this;
}
/**
* The `absolute URL` Twilio should request when the conference recording is
* available..
*
* @param conferenceRecordingStatusCallback Conference recording callback URL.
* @return this
*/
public ParticipantCreator setConferenceRecordingStatusCallback(final String conferenceRecordingStatusCallback) {
return setConferenceRecordingStatusCallback(Promoter.uriFromString(conferenceRecordingStatusCallback));
}
/**
* The HTTP method Twilio should use when requesting your recording status
* callback URL, either `GET` or `POST`. Defaults to `POST`..
*
* @param conferenceRecordingStatusCallbackMethod Method Twilio should use to
* request the
* `ConferenceRecordingStatusCallback` URL.
* @return this
*/
public ParticipantCreator setConferenceRecordingStatusCallbackMethod(final HttpMethod conferenceRecordingStatusCallbackMethod) {
this.conferenceRecordingStatusCallbackMethod = conferenceRecordingStatusCallbackMethod;
return this;
}
/**
* The recording_status_callback_event.
*
* @param recordingStatusCallbackEvent The recording_status_callback_event
* @return this
*/
public ParticipantCreator setRecordingStatusCallbackEvent(final List recordingStatusCallbackEvent) {
this.recordingStatusCallbackEvent = recordingStatusCallbackEvent;
return this;
}
/**
* The recording_status_callback_event.
*
* @param recordingStatusCallbackEvent The recording_status_callback_event
* @return this
*/
public ParticipantCreator setRecordingStatusCallbackEvent(final String recordingStatusCallbackEvent) {
return setRecordingStatusCallbackEvent(Promoter.listOfOne(recordingStatusCallbackEvent));
}
/**
* The conference_recording_status_callback_event.
*
* @param conferenceRecordingStatusCallbackEvent The
* conference_recording_status_callback_event
* @return this
*/
public ParticipantCreator setConferenceRecordingStatusCallbackEvent(final List conferenceRecordingStatusCallbackEvent) {
this.conferenceRecordingStatusCallbackEvent = conferenceRecordingStatusCallbackEvent;
return this;
}
/**
* The conference_recording_status_callback_event.
*
* @param conferenceRecordingStatusCallbackEvent The
* conference_recording_status_callback_event
* @return this
*/
public ParticipantCreator setConferenceRecordingStatusCallbackEvent(final String conferenceRecordingStatusCallbackEvent) {
return setConferenceRecordingStatusCallbackEvent(Promoter.listOfOne(conferenceRecordingStatusCallbackEvent));
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created Participant
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Participant create(final TwilioRestClient client) {
this.pathAccountSid = this.pathAccountSid == null ? client.getAccountSid() : this.pathAccountSid;
Request request = new Request(
HttpMethod.POST,
Domains.API.toString(),
"/2010-04-01/Accounts/" + this.pathAccountSid + "/Conferences/" + this.pathConferenceSid + "/Participants.json",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Participant creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Participant.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (from != null) {
request.addPostParam("From", from.toString());
}
if (to != null) {
request.addPostParam("To", to.toString());
}
if (statusCallback != null) {
request.addPostParam("StatusCallback", statusCallback.toString());
}
if (statusCallbackMethod != null) {
request.addPostParam("StatusCallbackMethod", statusCallbackMethod.toString());
}
if (statusCallbackEvent != null) {
for (String prop : statusCallbackEvent) {
request.addPostParam("StatusCallbackEvent", prop);
}
}
if (timeout != null) {
request.addPostParam("Timeout", timeout.toString());
}
if (record != null) {
request.addPostParam("Record", record.toString());
}
if (muted != null) {
request.addPostParam("Muted", muted.toString());
}
if (beep != null) {
request.addPostParam("Beep", beep);
}
if (startConferenceOnEnter != null) {
request.addPostParam("StartConferenceOnEnter", startConferenceOnEnter.toString());
}
if (endConferenceOnExit != null) {
request.addPostParam("EndConferenceOnExit", endConferenceOnExit.toString());
}
if (waitUrl != null) {
request.addPostParam("WaitUrl", waitUrl.toString());
}
if (waitMethod != null) {
request.addPostParam("WaitMethod", waitMethod.toString());
}
if (earlyMedia != null) {
request.addPostParam("EarlyMedia", earlyMedia.toString());
}
if (maxParticipants != null) {
request.addPostParam("MaxParticipants", maxParticipants.toString());
}
if (conferenceRecord != null) {
request.addPostParam("ConferenceRecord", conferenceRecord);
}
if (conferenceTrim != null) {
request.addPostParam("ConferenceTrim", conferenceTrim);
}
if (conferenceStatusCallback != null) {
request.addPostParam("ConferenceStatusCallback", conferenceStatusCallback.toString());
}
if (conferenceStatusCallbackMethod != null) {
request.addPostParam("ConferenceStatusCallbackMethod", conferenceStatusCallbackMethod.toString());
}
if (conferenceStatusCallbackEvent != null) {
for (String prop : conferenceStatusCallbackEvent) {
request.addPostParam("ConferenceStatusCallbackEvent", prop);
}
}
if (recordingChannels != null) {
request.addPostParam("RecordingChannels", recordingChannels);
}
if (recordingStatusCallback != null) {
request.addPostParam("RecordingStatusCallback", recordingStatusCallback.toString());
}
if (recordingStatusCallbackMethod != null) {
request.addPostParam("RecordingStatusCallbackMethod", recordingStatusCallbackMethod.toString());
}
if (sipAuthUsername != null) {
request.addPostParam("SipAuthUsername", sipAuthUsername);
}
if (sipAuthPassword != null) {
request.addPostParam("SipAuthPassword", sipAuthPassword);
}
if (region != null) {
request.addPostParam("Region", region);
}
if (conferenceRecordingStatusCallback != null) {
request.addPostParam("ConferenceRecordingStatusCallback", conferenceRecordingStatusCallback.toString());
}
if (conferenceRecordingStatusCallbackMethod != null) {
request.addPostParam("ConferenceRecordingStatusCallbackMethod", conferenceRecordingStatusCallbackMethod.toString());
}
if (recordingStatusCallbackEvent != null) {
for (String prop : recordingStatusCallbackEvent) {
request.addPostParam("RecordingStatusCallbackEvent", prop);
}
}
if (conferenceRecordingStatusCallbackEvent != null) {
for (String prop : conferenceRecordingStatusCallbackEvent) {
request.addPostParam("ConferenceRecordingStatusCallbackEvent", prop);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy