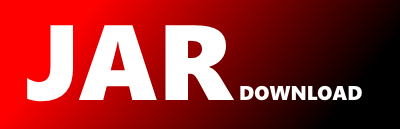
com.twilio.rest.ipmessaging.v2.ServiceUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.ipmessaging.v2;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
import java.util.List;
public class ServiceUpdater extends Updater {
private final String pathSid;
private String friendlyName;
private String defaultServiceRoleSid;
private String defaultChannelRoleSid;
private String defaultChannelCreatorRoleSid;
private Boolean readStatusEnabled;
private Boolean reachabilityEnabled;
private Integer typingIndicatorTimeout;
private Integer consumptionReportInterval;
private Boolean notificationsNewMessageEnabled;
private String notificationsNewMessageTemplate;
private String notificationsNewMessageSound;
private Boolean notificationsNewMessageBadgeCountEnabled;
private Boolean notificationsAddedToChannelEnabled;
private String notificationsAddedToChannelTemplate;
private String notificationsAddedToChannelSound;
private Boolean notificationsRemovedFromChannelEnabled;
private String notificationsRemovedFromChannelTemplate;
private String notificationsRemovedFromChannelSound;
private Boolean notificationsInvitedToChannelEnabled;
private String notificationsInvitedToChannelTemplate;
private String notificationsInvitedToChannelSound;
private URI preWebhookUrl;
private URI postWebhookUrl;
private HttpMethod webhookMethod;
private List webhookFilters;
private Integer limitsChannelMembers;
private Integer limitsUserChannels;
private String mediaCompatibilityMessage;
private Integer preWebhookRetryCount;
private Integer postWebhookRetryCount;
private Boolean notificationsLogEnabled;
/**
* Construct a new ServiceUpdater.
*
* @param pathSid The sid
*/
public ServiceUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* Human-readable name for this service instance.
*
* @param friendlyName Human-readable name for this service instance
* @return this
*/
public ServiceUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The default_service_role_sid.
*
* @param defaultServiceRoleSid The default_service_role_sid
* @return this
*/
public ServiceUpdater setDefaultServiceRoleSid(final String defaultServiceRoleSid) {
this.defaultServiceRoleSid = defaultServiceRoleSid;
return this;
}
/**
* Channel role assigned on channel join (see
* [Roles](https://www.twilio.com/docs/chat/api/roles) data model for the
* details).
*
* @param defaultChannelRoleSid Channel role assigned on channel join
* @return this
*/
public ServiceUpdater setDefaultChannelRoleSid(final String defaultChannelRoleSid) {
this.defaultChannelRoleSid = defaultChannelRoleSid;
return this;
}
/**
* Channel role assigned to creator of channel when joining for first time.
*
* @param defaultChannelCreatorRoleSid Channel role assigned to creator of
* channel when joining for first time
* @return this
*/
public ServiceUpdater setDefaultChannelCreatorRoleSid(final String defaultChannelCreatorRoleSid) {
this.defaultChannelCreatorRoleSid = defaultChannelCreatorRoleSid;
return this;
}
/**
* `true` if the member read status feature is enabled, `false` if not.
* Defaults to `true`..
*
* @param readStatusEnabled true if the member read status feature is enabled,
* false if not.
* @return this
*/
public ServiceUpdater setReadStatusEnabled(final Boolean readStatusEnabled) {
this.readStatusEnabled = readStatusEnabled;
return this;
}
/**
* `true` if the reachability feature should be enabled. Defaults to `false`.
*
* @param reachabilityEnabled true if the reachability feature should be
* enabled.
* @return this
*/
public ServiceUpdater setReachabilityEnabled(final Boolean reachabilityEnabled) {
this.reachabilityEnabled = reachabilityEnabled;
return this;
}
/**
* The duration in seconds indicating the timeout after "started typing" event
* when client should assume that user is not typing anymore even if no "ended
* typing" message received.
*
* @param typingIndicatorTimeout The duration in seconds indicating the timeout
* after "started typing" event when client should
* assume that user is not typing anymore even if
* no "ended typing" message received
* @return this
*/
public ServiceUpdater setTypingIndicatorTimeout(final Integer typingIndicatorTimeout) {
this.typingIndicatorTimeout = typingIndicatorTimeout;
return this;
}
/**
* The consumption_report_interval.
*
* @param consumptionReportInterval The consumption_report_interval
* @return this
*/
public ServiceUpdater setConsumptionReportInterval(final Integer consumptionReportInterval) {
this.consumptionReportInterval = consumptionReportInterval;
return this;
}
/**
* The notifications.new_message.enabled.
*
* @param notificationsNewMessageEnabled The notifications.new_message.enabled
* @return this
*/
public ServiceUpdater setNotificationsNewMessageEnabled(final Boolean notificationsNewMessageEnabled) {
this.notificationsNewMessageEnabled = notificationsNewMessageEnabled;
return this;
}
/**
* The notifications.new_message.template.
*
* @param notificationsNewMessageTemplate The notifications.new_message.template
* @return this
*/
public ServiceUpdater setNotificationsNewMessageTemplate(final String notificationsNewMessageTemplate) {
this.notificationsNewMessageTemplate = notificationsNewMessageTemplate;
return this;
}
/**
* The notifications.new_message.sound.
*
* @param notificationsNewMessageSound The notifications.new_message.sound
* @return this
*/
public ServiceUpdater setNotificationsNewMessageSound(final String notificationsNewMessageSound) {
this.notificationsNewMessageSound = notificationsNewMessageSound;
return this;
}
/**
* The notifications.new_message.badge_count_enabled.
*
* @param notificationsNewMessageBadgeCountEnabled The
* notifications.new_message.badge_count_enabled
* @return this
*/
public ServiceUpdater setNotificationsNewMessageBadgeCountEnabled(final Boolean notificationsNewMessageBadgeCountEnabled) {
this.notificationsNewMessageBadgeCountEnabled = notificationsNewMessageBadgeCountEnabled;
return this;
}
/**
* The notifications.added_to_channel.enabled.
*
* @param notificationsAddedToChannelEnabled The
* notifications.added_to_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelEnabled(final Boolean notificationsAddedToChannelEnabled) {
this.notificationsAddedToChannelEnabled = notificationsAddedToChannelEnabled;
return this;
}
/**
* The notifications.added_to_channel.template.
*
* @param notificationsAddedToChannelTemplate The
* notifications.added_to_channel.template
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelTemplate(final String notificationsAddedToChannelTemplate) {
this.notificationsAddedToChannelTemplate = notificationsAddedToChannelTemplate;
return this;
}
/**
* The notifications.added_to_channel.sound.
*
* @param notificationsAddedToChannelSound The
* notifications.added_to_channel.sound
* @return this
*/
public ServiceUpdater setNotificationsAddedToChannelSound(final String notificationsAddedToChannelSound) {
this.notificationsAddedToChannelSound = notificationsAddedToChannelSound;
return this;
}
/**
* The notifications.removed_from_channel.enabled.
*
* @param notificationsRemovedFromChannelEnabled The
* notifications.removed_from_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelEnabled(final Boolean notificationsRemovedFromChannelEnabled) {
this.notificationsRemovedFromChannelEnabled = notificationsRemovedFromChannelEnabled;
return this;
}
/**
* The notifications.removed_from_channel.template.
*
* @param notificationsRemovedFromChannelTemplate The
* notifications.removed_from_channel.template
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelTemplate(final String notificationsRemovedFromChannelTemplate) {
this.notificationsRemovedFromChannelTemplate = notificationsRemovedFromChannelTemplate;
return this;
}
/**
* The notifications.removed_from_channel.sound.
*
* @param notificationsRemovedFromChannelSound The
* notifications.removed_from_channel.sound
* @return this
*/
public ServiceUpdater setNotificationsRemovedFromChannelSound(final String notificationsRemovedFromChannelSound) {
this.notificationsRemovedFromChannelSound = notificationsRemovedFromChannelSound;
return this;
}
/**
* The notifications.invited_to_channel.enabled.
*
* @param notificationsInvitedToChannelEnabled The
* notifications.invited_to_channel.enabled
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelEnabled(final Boolean notificationsInvitedToChannelEnabled) {
this.notificationsInvitedToChannelEnabled = notificationsInvitedToChannelEnabled;
return this;
}
/**
* The notifications.invited_to_channel.template.
*
* @param notificationsInvitedToChannelTemplate The
* notifications.invited_to_channel.template
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelTemplate(final String notificationsInvitedToChannelTemplate) {
this.notificationsInvitedToChannelTemplate = notificationsInvitedToChannelTemplate;
return this;
}
/**
* The notifications.invited_to_channel.sound.
*
* @param notificationsInvitedToChannelSound The
* notifications.invited_to_channel.sound
* @return this
*/
public ServiceUpdater setNotificationsInvitedToChannelSound(final String notificationsInvitedToChannelSound) {
this.notificationsInvitedToChannelSound = notificationsInvitedToChannelSound;
return this;
}
/**
* The webhook URL for PRE-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/chat/webhook-events) for more details..
*
* @param preWebhookUrl The webhook URL for PRE-Event webhooks.
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final URI preWebhookUrl) {
this.preWebhookUrl = preWebhookUrl;
return this;
}
/**
* The webhook URL for PRE-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/chat/webhook-events) for more details..
*
* @param preWebhookUrl The webhook URL for PRE-Event webhooks.
* @return this
*/
public ServiceUpdater setPreWebhookUrl(final String preWebhookUrl) {
return setPreWebhookUrl(Promoter.uriFromString(preWebhookUrl));
}
/**
* The webhook URL for POST-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/chat/webhook-events) for more details..
*
* @param postWebhookUrl The webhook URL for POST-Event webhooks.
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final URI postWebhookUrl) {
this.postWebhookUrl = postWebhookUrl;
return this;
}
/**
* The webhook URL for POST-Event webhooks. See [Webhook
* Events](https://www.twilio.com/docs/chat/webhook-events) for more details..
*
* @param postWebhookUrl The webhook URL for POST-Event webhooks.
* @return this
*/
public ServiceUpdater setPostWebhookUrl(final String postWebhookUrl) {
return setPostWebhookUrl(Promoter.uriFromString(postWebhookUrl));
}
/**
* The webhook request format to use. Must be POST or GET. See [Webhook
* Events](https://www.twilio.com/docs/chat/webhook-events) for more details..
*
* @param webhookMethod The webhook request format to use.
* @return this
*/
public ServiceUpdater setWebhookMethod(final HttpMethod webhookMethod) {
this.webhookMethod = webhookMethod;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/chat/webhook-events) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance.
* @return this
*/
public ServiceUpdater setWebhookFilters(final List webhookFilters) {
this.webhookFilters = webhookFilters;
return this;
}
/**
* The list of WebHook events that are enabled for this Service instance. See
* [Webhook Events](https://www.twilio.com/docs/chat/webhook-events) for more
* details..
*
* @param webhookFilters The list of WebHook events that are enabled for this
* Service instance.
* @return this
*/
public ServiceUpdater setWebhookFilters(final String webhookFilters) {
return setWebhookFilters(Promoter.listOfOne(webhookFilters));
}
/**
* The maximum number of Members that can be added to Channels within this
* Service. The maximum allowed value is 1,000.
*
* @param limitsChannelMembers The maximum number of Members that can be added
* to Channels within this Service.
* @return this
*/
public ServiceUpdater setLimitsChannelMembers(final Integer limitsChannelMembers) {
this.limitsChannelMembers = limitsChannelMembers;
return this;
}
/**
* The maximum number of Channels Users can be a Member of within this Service.
* The maximum value allowed is 1,000.
*
* @param limitsUserChannels The maximum number of Channels Users can be a
* Member of within this Service.
* @return this
*/
public ServiceUpdater setLimitsUserChannels(final Integer limitsUserChannels) {
this.limitsUserChannels = limitsUserChannels;
return this;
}
/**
* The media.compatibility_message.
*
* @param mediaCompatibilityMessage The media.compatibility_message
* @return this
*/
public ServiceUpdater setMediaCompatibilityMessage(final String mediaCompatibilityMessage) {
this.mediaCompatibilityMessage = mediaCompatibilityMessage;
return this;
}
/**
* Count of times webhook will be retried in case of timeout (5 seconds) or
* 429/503/504 HTTP responses. Default retry count is 0 times..
*
* @param preWebhookRetryCount Count of times webhook will be retried in case
* of timeout or 429/503/504 HTTP responses.
* @return this
*/
public ServiceUpdater setPreWebhookRetryCount(final Integer preWebhookRetryCount) {
this.preWebhookRetryCount = preWebhookRetryCount;
return this;
}
/**
* Count of times webhook will be retried in case of timeout (5 seconds) or
* 429/503/504 HTTP responses. Default retry count is 0 times..
*
* @param postWebhookRetryCount Count of times webhook will be retried in case
* of timeout or 429/503/504 HTTP responses.
* @return this
*/
public ServiceUpdater setPostWebhookRetryCount(final Integer postWebhookRetryCount) {
this.postWebhookRetryCount = postWebhookRetryCount;
return this;
}
/**
* The notifications.log_enabled.
*
* @param notificationsLogEnabled The notifications.log_enabled
* @return this
*/
public ServiceUpdater setNotificationsLogEnabled(final Boolean notificationsLogEnabled) {
this.notificationsLogEnabled = notificationsLogEnabled;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Service
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Service update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.IPMESSAGING.toString(),
"/v2/Services/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Service update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Service.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (defaultServiceRoleSid != null) {
request.addPostParam("DefaultServiceRoleSid", defaultServiceRoleSid);
}
if (defaultChannelRoleSid != null) {
request.addPostParam("DefaultChannelRoleSid", defaultChannelRoleSid);
}
if (defaultChannelCreatorRoleSid != null) {
request.addPostParam("DefaultChannelCreatorRoleSid", defaultChannelCreatorRoleSid);
}
if (readStatusEnabled != null) {
request.addPostParam("ReadStatusEnabled", readStatusEnabled.toString());
}
if (reachabilityEnabled != null) {
request.addPostParam("ReachabilityEnabled", reachabilityEnabled.toString());
}
if (typingIndicatorTimeout != null) {
request.addPostParam("TypingIndicatorTimeout", typingIndicatorTimeout.toString());
}
if (consumptionReportInterval != null) {
request.addPostParam("ConsumptionReportInterval", consumptionReportInterval.toString());
}
if (notificationsNewMessageEnabled != null) {
request.addPostParam("Notifications.NewMessage.Enabled", notificationsNewMessageEnabled.toString());
}
if (notificationsNewMessageTemplate != null) {
request.addPostParam("Notifications.NewMessage.Template", notificationsNewMessageTemplate);
}
if (notificationsNewMessageSound != null) {
request.addPostParam("Notifications.NewMessage.Sound", notificationsNewMessageSound);
}
if (notificationsNewMessageBadgeCountEnabled != null) {
request.addPostParam("Notifications.NewMessage.BadgeCountEnabled", notificationsNewMessageBadgeCountEnabled.toString());
}
if (notificationsAddedToChannelEnabled != null) {
request.addPostParam("Notifications.AddedToChannel.Enabled", notificationsAddedToChannelEnabled.toString());
}
if (notificationsAddedToChannelTemplate != null) {
request.addPostParam("Notifications.AddedToChannel.Template", notificationsAddedToChannelTemplate);
}
if (notificationsAddedToChannelSound != null) {
request.addPostParam("Notifications.AddedToChannel.Sound", notificationsAddedToChannelSound);
}
if (notificationsRemovedFromChannelEnabled != null) {
request.addPostParam("Notifications.RemovedFromChannel.Enabled", notificationsRemovedFromChannelEnabled.toString());
}
if (notificationsRemovedFromChannelTemplate != null) {
request.addPostParam("Notifications.RemovedFromChannel.Template", notificationsRemovedFromChannelTemplate);
}
if (notificationsRemovedFromChannelSound != null) {
request.addPostParam("Notifications.RemovedFromChannel.Sound", notificationsRemovedFromChannelSound);
}
if (notificationsInvitedToChannelEnabled != null) {
request.addPostParam("Notifications.InvitedToChannel.Enabled", notificationsInvitedToChannelEnabled.toString());
}
if (notificationsInvitedToChannelTemplate != null) {
request.addPostParam("Notifications.InvitedToChannel.Template", notificationsInvitedToChannelTemplate);
}
if (notificationsInvitedToChannelSound != null) {
request.addPostParam("Notifications.InvitedToChannel.Sound", notificationsInvitedToChannelSound);
}
if (preWebhookUrl != null) {
request.addPostParam("PreWebhookUrl", preWebhookUrl.toString());
}
if (postWebhookUrl != null) {
request.addPostParam("PostWebhookUrl", postWebhookUrl.toString());
}
if (webhookMethod != null) {
request.addPostParam("WebhookMethod", webhookMethod.toString());
}
if (webhookFilters != null) {
for (String prop : webhookFilters) {
request.addPostParam("WebhookFilters", prop);
}
}
if (limitsChannelMembers != null) {
request.addPostParam("Limits.ChannelMembers", limitsChannelMembers.toString());
}
if (limitsUserChannels != null) {
request.addPostParam("Limits.UserChannels", limitsUserChannels.toString());
}
if (mediaCompatibilityMessage != null) {
request.addPostParam("Media.CompatibilityMessage", mediaCompatibilityMessage);
}
if (preWebhookRetryCount != null) {
request.addPostParam("PreWebhookRetryCount", preWebhookRetryCount.toString());
}
if (postWebhookRetryCount != null) {
request.addPostParam("PostWebhookRetryCount", postWebhookRetryCount.toString());
}
if (notificationsLogEnabled != null) {
request.addPostParam("Notifications.LogEnabled", notificationsLogEnabled.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy