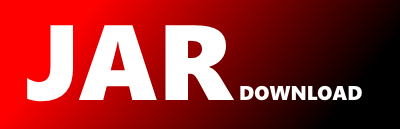
com.twilio.rest.notify.v1.ServiceCreator Maven / Gradle / Ivy
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.notify.v1;
import com.twilio.base.Creator;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
public class ServiceCreator extends Creator {
private String friendlyName;
private String apnCredentialSid;
private String gcmCredentialSid;
private String messagingServiceSid;
private String facebookMessengerPageId;
private String defaultApnNotificationProtocolVersion;
private String defaultGcmNotificationProtocolVersion;
private String fcmCredentialSid;
private String defaultFcmNotificationProtocolVersion;
private Boolean logEnabled;
private String alexaSkillId;
private String defaultAlexaNotificationProtocolVersion;
/**
* Human-readable name for this service instance.
*
* @param friendlyName Human-readable name for this service instance
* @return this
*/
public ServiceCreator setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The SID of the
* [Credential](https://www.twilio.com/docs/notify/api/credentials) to be used
* for APN Bindings..
*
* @param apnCredentialSid The SID of the Credential to be used for APN
* Bindings.
* @return this
*/
public ServiceCreator setApnCredentialSid(final String apnCredentialSid) {
this.apnCredentialSid = apnCredentialSid;
return this;
}
/**
* The SID of the
* [Credential](https://www.twilio.com/docs/notify/api/credentials) to be used
* for GCM Bindings..
*
* @param gcmCredentialSid The SID of the Credential to be used for GCM
* Bindings.
* @return this
*/
public ServiceCreator setGcmCredentialSid(final String gcmCredentialSid) {
this.gcmCredentialSid = gcmCredentialSid;
return this;
}
/**
* The SID of the [Messaging
* Service](https://www.twilio.com/docs/api/rest/sending-messages#messaging-services) to be used for SMS Bindings. In order to send SMS notifications this parameter has to be set..
*
* @param messagingServiceSid The SID of the Messaging Service to be used for
* SMS Bindings.
* @return this
*/
public ServiceCreator setMessagingServiceSid(final String messagingServiceSid) {
this.messagingServiceSid = messagingServiceSid;
return this;
}
/**
* The Page ID to be used to send for Facebook Messenger Bindings. It has to
* match the Page ID you configured when you [enabled Facebook
* Messaging](https://www.twilio.com/console/sms/settings) on your account..
*
* @param facebookMessengerPageId The Page ID to be used to send for Facebook
* Messenger Bindings.
* @return this
*/
public ServiceCreator setFacebookMessengerPageId(final String facebookMessengerPageId) {
this.facebookMessengerPageId = facebookMessengerPageId;
return this;
}
/**
* The version of the protocol to be used for sending APNS notifications. Can be
* overriden on a Binding by Binding basis when creating a
* [Binding](https://www.twilio.com/docs/notify/api/bindings) resource..
*
* @param defaultApnNotificationProtocolVersion The version of the protocol to
* be used for sending APNS
* notifications.
* @return this
*/
public ServiceCreator setDefaultApnNotificationProtocolVersion(final String defaultApnNotificationProtocolVersion) {
this.defaultApnNotificationProtocolVersion = defaultApnNotificationProtocolVersion;
return this;
}
/**
* The version of the protocol to be used for sending GCM notifications. Can be
* overriden on a Binding by Binding basis when creating a
* [Binding](https://www.twilio.com/docs/notify/api/bindings) resource..
*
* @param defaultGcmNotificationProtocolVersion The version of the protocol to
* be used for sending GCM
* notifications.
* @return this
*/
public ServiceCreator setDefaultGcmNotificationProtocolVersion(final String defaultGcmNotificationProtocolVersion) {
this.defaultGcmNotificationProtocolVersion = defaultGcmNotificationProtocolVersion;
return this;
}
/**
* The SID of the
* [Credential](https://www.twilio.com/docs/notify/api/credentials) to be used
* for FCM Bindings..
*
* @param fcmCredentialSid The SID of the Credential to be used for FCM
* Bindings.
* @return this
*/
public ServiceCreator setFcmCredentialSid(final String fcmCredentialSid) {
this.fcmCredentialSid = fcmCredentialSid;
return this;
}
/**
* The version of the protocol to be used for sending FCM notifications. Can be
* overriden on a Binding by Binding basis when creating a
* [Binding](https://www.twilio.com/docs/notify/api/bindings) resource..
*
* @param defaultFcmNotificationProtocolVersion The version of the protocol to
* be used for sending FCM
* notifications.
* @return this
*/
public ServiceCreator setDefaultFcmNotificationProtocolVersion(final String defaultFcmNotificationProtocolVersion) {
this.defaultFcmNotificationProtocolVersion = defaultFcmNotificationProtocolVersion;
return this;
}
/**
* The log_enabled.
*
* @param logEnabled The log_enabled
* @return this
*/
public ServiceCreator setLogEnabled(final Boolean logEnabled) {
this.logEnabled = logEnabled;
return this;
}
/**
* The alexa_skill_id.
*
* @param alexaSkillId The alexa_skill_id
* @return this
*/
public ServiceCreator setAlexaSkillId(final String alexaSkillId) {
this.alexaSkillId = alexaSkillId;
return this;
}
/**
* The default_alexa_notification_protocol_version.
*
* @param defaultAlexaNotificationProtocolVersion The
* default_alexa_notification_protocol_version
* @return this
*/
public ServiceCreator setDefaultAlexaNotificationProtocolVersion(final String defaultAlexaNotificationProtocolVersion) {
this.defaultAlexaNotificationProtocolVersion = defaultAlexaNotificationProtocolVersion;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created Service
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Service create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.NOTIFY.toString(),
"/v1/Services",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Service creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Service.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (apnCredentialSid != null) {
request.addPostParam("ApnCredentialSid", apnCredentialSid);
}
if (gcmCredentialSid != null) {
request.addPostParam("GcmCredentialSid", gcmCredentialSid);
}
if (messagingServiceSid != null) {
request.addPostParam("MessagingServiceSid", messagingServiceSid);
}
if (facebookMessengerPageId != null) {
request.addPostParam("FacebookMessengerPageId", facebookMessengerPageId);
}
if (defaultApnNotificationProtocolVersion != null) {
request.addPostParam("DefaultApnNotificationProtocolVersion", defaultApnNotificationProtocolVersion);
}
if (defaultGcmNotificationProtocolVersion != null) {
request.addPostParam("DefaultGcmNotificationProtocolVersion", defaultGcmNotificationProtocolVersion);
}
if (fcmCredentialSid != null) {
request.addPostParam("FcmCredentialSid", fcmCredentialSid);
}
if (defaultFcmNotificationProtocolVersion != null) {
request.addPostParam("DefaultFcmNotificationProtocolVersion", defaultFcmNotificationProtocolVersion);
}
if (logEnabled != null) {
request.addPostParam("LogEnabled", logEnabled.toString());
}
if (alexaSkillId != null) {
request.addPostParam("AlexaSkillId", alexaSkillId);
}
if (defaultAlexaNotificationProtocolVersion != null) {
request.addPostParam("DefaultAlexaNotificationProtocolVersion", defaultAlexaNotificationProtocolVersion);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy