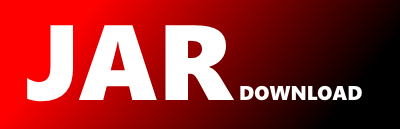
com.twilio.rest.api.v2010.account.CallCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account;
import com.twilio.base.Creator;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import com.twilio.type.Endpoint;
import java.net.URI;
import java.util.List;
public class CallCreator extends Creator {
private String pathAccountSid;
private final com.twilio.type.Endpoint to;
private final com.twilio.type.PhoneNumber from;
private URI url;
private String applicationSid;
private HttpMethod method;
private URI fallbackUrl;
private HttpMethod fallbackMethod;
private URI statusCallback;
private List statusCallbackEvent;
private HttpMethod statusCallbackMethod;
private String sendDigits;
private String ifMachine;
private Integer timeout;
private Boolean record;
private String recordingChannels;
private String recordingStatusCallback;
private HttpMethod recordingStatusCallbackMethod;
private String sipAuthUsername;
private String sipAuthPassword;
private String machineDetection;
private Integer machineDetectionTimeout;
private List recordingStatusCallbackEvent;
private String trim;
private String callerId;
/**
* Construct a new CallCreator.
*
* @param to Phone number, SIP address, or client identifier to call
* @param from Twilio number from which to originate the call
* @param url Url from which to fetch TwiML
*/
public CallCreator(final com.twilio.type.Endpoint to,
final com.twilio.type.PhoneNumber from,
final URI url) {
this.to = to;
this.from = from;
this.url = url;
}
/**
* Construct a new CallCreator.
*
* @param pathAccountSid The account_sid
* @param to Phone number, SIP address, or client identifier to call
* @param from Twilio number from which to originate the call
* @param url Url from which to fetch TwiML
*/
public CallCreator(final String pathAccountSid,
final com.twilio.type.Endpoint to,
final com.twilio.type.PhoneNumber from,
final URI url) {
this.pathAccountSid = pathAccountSid;
this.to = to;
this.from = from;
this.url = url;
}
/**
* Construct a new CallCreator.
*
* @param to Phone number, SIP address, or client identifier to call
* @param from Twilio number from which to originate the call
* @param applicationSid ApplicationSid that configures from where to fetch
* TwiML
*/
public CallCreator(final com.twilio.type.Endpoint to,
final com.twilio.type.PhoneNumber from,
final String applicationSid) {
this.to = to;
this.from = from;
this.applicationSid = applicationSid;
}
/**
* Construct a new CallCreator.
*
* @param pathAccountSid The account_sid
* @param to Phone number, SIP address, or client identifier to call
* @param from Twilio number from which to originate the call
* @param applicationSid ApplicationSid that configures from where to fetch
* TwiML
*/
public CallCreator(final String pathAccountSid,
final com.twilio.type.Endpoint to,
final com.twilio.type.PhoneNumber from,
final String applicationSid) {
this.pathAccountSid = pathAccountSid;
this.to = to;
this.from = from;
this.applicationSid = applicationSid;
}
/**
* The HTTP method Twilio should use when making its request to the `Url`
* parameter's value. Defaults to `POST`. If an `ApplicationSid` parameter is
* present, this parameter is ignored..
*
* @param method HTTP method to use to fetch TwiML
* @return this
*/
public CallCreator setMethod(final HttpMethod method) {
this.method = method;
return this;
}
/**
* A URL that Twilio will request if an error occurs requesting or executing the
* TwiML at `Url`. If an `ApplicationSid` parameter is present, this parameter
* is ignored..
*
* @param fallbackUrl Fallback URL in case of error
* @return this
*/
public CallCreator setFallbackUrl(final URI fallbackUrl) {
this.fallbackUrl = fallbackUrl;
return this;
}
/**
* A URL that Twilio will request if an error occurs requesting or executing the
* TwiML at `Url`. If an `ApplicationSid` parameter is present, this parameter
* is ignored..
*
* @param fallbackUrl Fallback URL in case of error
* @return this
*/
public CallCreator setFallbackUrl(final String fallbackUrl) {
return setFallbackUrl(Promoter.uriFromString(fallbackUrl));
}
/**
* The HTTP method that Twilio should use to request the `FallbackUrl`. Must be
* either `GET` or `POST`. Defaults to `POST`. If an `ApplicationSid` parameter
* is present, this parameter is ignored..
*
* @param fallbackMethod HTTP Method to use with FallbackUrl
* @return this
*/
public CallCreator setFallbackMethod(final HttpMethod fallbackMethod) {
this.fallbackMethod = fallbackMethod;
return this;
}
/**
* A URL that Twilio will send asynchronous webhook requests to on every call
* event specified in the `StatusCallbackEvent` parameter. If no event is
* specified, Twilio will send `completed` by default. If an `ApplicationSid`
* parameter is present, this parameter is ignored. URLs must contain a valid
* hostname (underscores are not permitted)..
*
* @param statusCallback Status Callback URL
* @return this
*/
public CallCreator setStatusCallback(final URI statusCallback) {
this.statusCallback = statusCallback;
return this;
}
/**
* A URL that Twilio will send asynchronous webhook requests to on every call
* event specified in the `StatusCallbackEvent` parameter. If no event is
* specified, Twilio will send `completed` by default. If an `ApplicationSid`
* parameter is present, this parameter is ignored. URLs must contain a valid
* hostname (underscores are not permitted)..
*
* @param statusCallback Status Callback URL
* @return this
*/
public CallCreator setStatusCallback(final String statusCallback) {
return setStatusCallback(Promoter.uriFromString(statusCallback));
}
/**
* The call progress events that will trigger a webhook from Twilio. Available
* values are `initiated`, `ringing`, `answered`, and `completed`. If no event
* is specified, defaults to `completed`. If you want to receive multiple
* events, please provide multiple `StatusCallbackEvent` values as individual
* parameters in the `POST` request. See the code sample for [monitoring call
* progress](https://www.twilio.com/docs/voice/api/call?code-sample=code-create-a-call-and-specify-a-statuscallbackevent). If an `ApplicationSid` is present, this parameter is ignored..
*
* @param statusCallbackEvent The call progress events that Twilio will send
* webhooks on.
* @return this
*/
public CallCreator setStatusCallbackEvent(final List statusCallbackEvent) {
this.statusCallbackEvent = statusCallbackEvent;
return this;
}
/**
* The call progress events that will trigger a webhook from Twilio. Available
* values are `initiated`, `ringing`, `answered`, and `completed`. If no event
* is specified, defaults to `completed`. If you want to receive multiple
* events, please provide multiple `StatusCallbackEvent` values as individual
* parameters in the `POST` request. See the code sample for [monitoring call
* progress](https://www.twilio.com/docs/voice/api/call?code-sample=code-create-a-call-and-specify-a-statuscallbackevent). If an `ApplicationSid` is present, this parameter is ignored..
*
* @param statusCallbackEvent The call progress events that Twilio will send
* webhooks on.
* @return this
*/
public CallCreator setStatusCallbackEvent(final String statusCallbackEvent) {
return setStatusCallbackEvent(Promoter.listOfOne(statusCallbackEvent));
}
/**
* The HTTP method Twilio should use when requesting the `StatusCallback` URL.
* Defaults to `POST`. If an `ApplicationSid` parameter is present, this
* parameter is ignored..
*
* @param statusCallbackMethod HTTP Method to use with StatusCallback
* @return this
*/
public CallCreator setStatusCallbackMethod(final HttpMethod statusCallbackMethod) {
this.statusCallbackMethod = statusCallbackMethod;
return this;
}
/**
* A string of keys to dial after connecting to the number, maximum of 32
* digits. Valid digits in the string include: any digit (`0`-`9`), '`#`', '`*`'
* and '`w`' (to insert a half second pause). For example, if you connected to a
* company phone number and wanted to pause for one second, dial extension 1234,
* and then the pound key, use `SendDigits=ww1234#`. Remember to URL-encode this
* string, since the '`#`' character has special meaning in a URL. If both
* `SendDigits` and `MachineDetection` parameters are provided, then
* `MachineDetection` will be ignored..
*
* @param sendDigits Digits to send
* @return this
*/
public CallCreator setSendDigits(final String sendDigits) {
this.sendDigits = sendDigits;
return this;
}
/**
* The if_machine.
*
* @param ifMachine The if_machine
* @return this
*/
public CallCreator setIfMachine(final String ifMachine) {
this.ifMachine = ifMachine;
return this;
}
/**
* The integer number of seconds that Twilio should allow the phone to ring
* before assuming there is no answer. Default is `60` seconds, the maximum is
* `600` seconds. For some call flows, Twilio will add a 5-second buffer to the
* timeout value provided, so if you enter a timeout value of 10 seconds, you
* could see actual timeout closer to 15 seconds. Note, you could set this to a
* low value like `15` to hang up before reaching an answering machine or
* voicemail..
*
* @param timeout Number of seconds to wait for an answer
* @return this
*/
public CallCreator setTimeout(final Integer timeout) {
this.timeout = timeout;
return this;
}
/**
* Set this parameter to `true` to record the entirety of a phone call. The
* `RecordingUrl` will be sent to the `StatusCallback` URL. Defaults to false..
*
* @param record Whether or not to record the Call
* @return this
*/
public CallCreator setRecord(final Boolean record) {
this.record = record;
return this;
}
/**
* `mono` or `dual` – defaults to `mono`. Set this parameter to specify the
* number of channels in the final recording. In a mono-channel recording, both
* legs of the call are mixed down into a single channel within a single
* recording file. With dual-channel recording, both legs use separate channels
* within a single recording file. In dual-channel recordings, the parent call
* will always be in the first channel, and the child call will always be in the
* second channel..
*
* @param recordingChannels mono or dualSet this parameter to specify the
* number of channels in the final recording.
* @return this
*/
public CallCreator setRecordingChannels(final String recordingChannels) {
this.recordingChannels = recordingChannels;
return this;
}
/**
* A URL that Twilio will send a webhook request to when the recording is
* available for access..
*
* @param recordingStatusCallback A URL that Twilio will send a webhook request
* to when the recording is available for access.
* @return this
*/
public CallCreator setRecordingStatusCallback(final String recordingStatusCallback) {
this.recordingStatusCallback = recordingStatusCallback;
return this;
}
/**
* The HTTP method Twilio should use when requesting the
* `RecordingStatusCallback` URL. Defaults to `POST`..
*
* @param recordingStatusCallbackMethod The HTTP method Twilio should use when
* requesting the `RecordingStatusCallback`
* URL.
* @return this
*/
public CallCreator setRecordingStatusCallbackMethod(final HttpMethod recordingStatusCallbackMethod) {
this.recordingStatusCallbackMethod = recordingStatusCallbackMethod;
return this;
}
/**
* The sip_auth_username.
*
* @param sipAuthUsername The sip_auth_username
* @return this
*/
public CallCreator setSipAuthUsername(final String sipAuthUsername) {
this.sipAuthUsername = sipAuthUsername;
return this;
}
/**
* The sip_auth_password.
*
* @param sipAuthPassword The sip_auth_password
* @return this
*/
public CallCreator setSipAuthPassword(final String sipAuthPassword) {
this.sipAuthPassword = sipAuthPassword;
return this;
}
/**
* Detect if a human, answering machine, or fax has picked up the call. Use
* `Enable` if you would like Twilio to return an `AnsweredBy` value as soon as
* it identifies the called party. If you would like to leave a message on an
* answering machine, specify `DetectMessageEnd`. If both `SendDigits` and
* `MachineDetection` parameters are provided, then MachineDetection will be
* ignored. [Detailed documentation is
* here](https://www.twilio.com/docs/voice/answering-machine-detection)..
*
* @param machineDetection Enable machine detection or end of greeting detection
* @return this
*/
public CallCreator setMachineDetection(final String machineDetection) {
this.machineDetection = machineDetection;
return this;
}
/**
* The number of seconds that Twilio should attempt to perform answering machine
* detection before timing out and firing a voice request with `AnsweredBy` of
* `unknown`. Defaults to 30 seconds..
*
* @param machineDetectionTimeout Number of miliseconds to wait for machine
* detection
* @return this
*/
public CallCreator setMachineDetectionTimeout(final Integer machineDetectionTimeout) {
this.machineDetectionTimeout = machineDetectionTimeout;
return this;
}
/**
* The recording status events that will trigger Twilio to send webhooks on to
* the URL specified in `RecordingStatusCallback`. The available values are
* `in-progress`, `completed`, and `failed`. Defaults are `completed` and
* `failed`. To specify multiple values, separate them with a space. If any
* values are specified, the defaults are no longer applicable..
*
* @param recordingStatusCallbackEvent The recording status changes that Twilio
* will send webhooks on to the URL
* specified in RecordingStatusCallback.
* @return this
*/
public CallCreator setRecordingStatusCallbackEvent(final List recordingStatusCallbackEvent) {
this.recordingStatusCallbackEvent = recordingStatusCallbackEvent;
return this;
}
/**
* The recording status events that will trigger Twilio to send webhooks on to
* the URL specified in `RecordingStatusCallback`. The available values are
* `in-progress`, `completed`, and `failed`. Defaults are `completed` and
* `failed`. To specify multiple values, separate them with a space. If any
* values are specified, the defaults are no longer applicable..
*
* @param recordingStatusCallbackEvent The recording status changes that Twilio
* will send webhooks on to the URL
* specified in RecordingStatusCallback.
* @return this
*/
public CallCreator setRecordingStatusCallbackEvent(final String recordingStatusCallbackEvent) {
return setRecordingStatusCallbackEvent(Promoter.listOfOne(recordingStatusCallbackEvent));
}
/**
* `trim-silence` or `do-not-trim`. Set this parameter to define whether leading
* and trailing silence is trimmed from the recording. Defaults to
* `trim-silence`..
*
* @param trim Set this parameter to control trimming of silence on the
* recording.
* @return this
*/
public CallCreator setTrim(final String trim) {
this.trim = trim;
return this;
}
/**
* The phone number, SIP address, or Client identifier that made this Call.
* Phone numbers are in [E.164
* format](https://www.twilio.com/docs/glossary/what-e164) (e.g., +16175551212).
* SIP addresses are formatted as `[email protected]`..
*
* @param callerId The phone number, SIP address, or Client identifier that
* made this Call. Phone numbers are in E.164 format (e.g.,
* +16175551212). SIP addresses are formatted as
* `[email protected]`.
* @return this
*/
public CallCreator setCallerId(final String callerId) {
this.callerId = callerId;
return this;
}
/**
* The fully qualified URL that hosts instructions for the call. Twilio will
* consult this URL when the call connects. See the [Url
* Parameter](https://www.twilio.com/docs/voice/make-calls#specify-a-url-parameter) details in [Making Calls](https://www.twilio.com/docs/voice/make-calls) for more details..
*
* @param url Url from which to fetch TwiML
* @return this
*/
public CallCreator setUrl(final URI url) {
this.url = url;
return this;
}
/**
* The fully qualified URL that hosts instructions for the call. Twilio will
* consult this URL when the call connects. See the [Url
* Parameter](https://www.twilio.com/docs/voice/make-calls#specify-a-url-parameter) details in [Making Calls](https://www.twilio.com/docs/voice/make-calls) for more details..
*
* @param url Url from which to fetch TwiML
* @return this
*/
public CallCreator setUrl(final String url) {
return setUrl(Promoter.uriFromString(url));
}
/**
* The 34-character SID of the application Twilio should use to handle this
* phone call. If this parameter is present, Twilio will ignore all of the voice
* URLs passed and use the URLs set on the application. See the [ApplicationSid
* Parameter](https://www.twilio.com/docs/voice/make-calls#specify-an-applicationsid-parameter) section in [Making Calls](https://www.twilio.com/docs/voice/make-calls) for more details..
*
* @param applicationSid ApplicationSid that configures from where to fetch
* TwiML
* @return this
*/
public CallCreator setApplicationSid(final String applicationSid) {
this.applicationSid = applicationSid;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created Call
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Call create(final TwilioRestClient client) {
this.pathAccountSid = this.pathAccountSid == null ? client.getAccountSid() : this.pathAccountSid;
Request request = new Request(
HttpMethod.POST,
Domains.API.toString(),
"/2010-04-01/Accounts/" + this.pathAccountSid + "/Calls.json",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Call creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Call.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (to != null) {
request.addPostParam("To", to.getEndpoint());
}
if (from != null) {
request.addPostParam("From", from.toString());
}
if (url != null) {
request.addPostParam("Url", url.toString());
}
if (applicationSid != null) {
request.addPostParam("ApplicationSid", applicationSid);
}
if (method != null) {
request.addPostParam("Method", method.toString());
}
if (fallbackUrl != null) {
request.addPostParam("FallbackUrl", fallbackUrl.toString());
}
if (fallbackMethod != null) {
request.addPostParam("FallbackMethod", fallbackMethod.toString());
}
if (statusCallback != null) {
request.addPostParam("StatusCallback", statusCallback.toString());
}
if (statusCallbackEvent != null) {
for (String prop : statusCallbackEvent) {
request.addPostParam("StatusCallbackEvent", prop);
}
}
if (statusCallbackMethod != null) {
request.addPostParam("StatusCallbackMethod", statusCallbackMethod.toString());
}
if (sendDigits != null) {
request.addPostParam("SendDigits", sendDigits);
}
if (ifMachine != null) {
request.addPostParam("IfMachine", ifMachine);
}
if (timeout != null) {
request.addPostParam("Timeout", timeout.toString());
}
if (record != null) {
request.addPostParam("Record", record.toString());
}
if (recordingChannels != null) {
request.addPostParam("RecordingChannels", recordingChannels);
}
if (recordingStatusCallback != null) {
request.addPostParam("RecordingStatusCallback", recordingStatusCallback);
}
if (recordingStatusCallbackMethod != null) {
request.addPostParam("RecordingStatusCallbackMethod", recordingStatusCallbackMethod.toString());
}
if (sipAuthUsername != null) {
request.addPostParam("SipAuthUsername", sipAuthUsername);
}
if (sipAuthPassword != null) {
request.addPostParam("SipAuthPassword", sipAuthPassword);
}
if (machineDetection != null) {
request.addPostParam("MachineDetection", machineDetection);
}
if (machineDetectionTimeout != null) {
request.addPostParam("MachineDetectionTimeout", machineDetectionTimeout.toString());
}
if (recordingStatusCallbackEvent != null) {
for (String prop : recordingStatusCallbackEvent) {
request.addPostParam("RecordingStatusCallbackEvent", prop);
}
}
if (trim != null) {
request.addPostParam("Trim", trim);
}
if (callerId != null) {
request.addPostParam("CallerId", callerId);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy