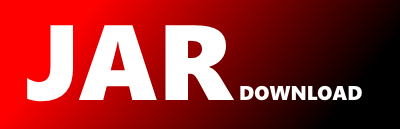
com.twilio.rest.proxy.v1.Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.proxy.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
import java.util.Objects;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class Service extends Resource {
private static final long serialVersionUID = 38310522618070L;
public enum GeoMatchLevel {
AREA_CODE("area-code"),
OVERLAY("overlay"),
RADIUS("radius"),
COUNTRY("country");
private final String value;
private GeoMatchLevel(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a GeoMatchLevel from a string.
* @param value string value
* @return generated GeoMatchLevel
*/
@JsonCreator
public static GeoMatchLevel forValue(final String value) {
return Promoter.enumFromString(value, GeoMatchLevel.values());
}
}
public enum NumberSelectionBehavior {
AVOID_STICKY("avoid-sticky"),
PREFER_STICKY("prefer-sticky");
private final String value;
private NumberSelectionBehavior(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a NumberSelectionBehavior from a string.
* @param value string value
* @return generated NumberSelectionBehavior
*/
@JsonCreator
public static NumberSelectionBehavior forValue(final String value) {
return Promoter.enumFromString(value, NumberSelectionBehavior.values());
}
}
/**
* Create a ServiceFetcher to execute fetch.
*
* @param pathSid A string that uniquely identifies this Service.
* @return ServiceFetcher capable of executing the fetch
*/
public static ServiceFetcher fetcher(final String pathSid) {
return new ServiceFetcher(pathSid);
}
/**
* Create a ServiceReader to execute read.
*
* @return ServiceReader capable of executing the read
*/
public static ServiceReader reader() {
return new ServiceReader();
}
/**
* Create a ServiceCreator to execute create.
*
* @param uniqueName The human-readable string that uniquely identifies this
* Service.
* @return ServiceCreator capable of executing the create
*/
public static ServiceCreator creator(final String uniqueName) {
return new ServiceCreator(uniqueName);
}
/**
* Create a ServiceDeleter to execute delete.
*
* @param pathSid A string that uniquely identifies this Service.
* @return ServiceDeleter capable of executing the delete
*/
public static ServiceDeleter deleter(final String pathSid) {
return new ServiceDeleter(pathSid);
}
/**
* Create a ServiceUpdater to execute update.
*
* @param pathSid A string that uniquely identifies this Service.
* @return ServiceUpdater capable of executing the update
*/
public static ServiceUpdater updater(final String pathSid) {
return new ServiceUpdater(pathSid);
}
/**
* Converts a JSON String into a Service object using the provided ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Service object represented by the provided JSON
*/
public static Service fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Service.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Service object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Service object represented by the provided JSON
*/
public static Service fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Service.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String uniqueName;
private final String accountSid;
private final URI callbackUrl;
private final Integer defaultTtl;
private final Service.NumberSelectionBehavior numberSelectionBehavior;
private final Service.GeoMatchLevel geoMatchLevel;
private final URI interceptCallbackUrl;
private final URI outOfSessionCallbackUrl;
private final DateTime dateCreated;
private final DateTime dateUpdated;
private final URI url;
private final Map links;
@JsonCreator
private Service(@JsonProperty("sid")
final String sid,
@JsonProperty("unique_name")
final String uniqueName,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("callback_url")
final URI callbackUrl,
@JsonProperty("default_ttl")
final Integer defaultTtl,
@JsonProperty("number_selection_behavior")
final Service.NumberSelectionBehavior numberSelectionBehavior,
@JsonProperty("geo_match_level")
final Service.GeoMatchLevel geoMatchLevel,
@JsonProperty("intercept_callback_url")
final URI interceptCallbackUrl,
@JsonProperty("out_of_session_callback_url")
final URI outOfSessionCallbackUrl,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("date_updated")
final String dateUpdated,
@JsonProperty("url")
final URI url,
@JsonProperty("links")
final Map links) {
this.sid = sid;
this.uniqueName = uniqueName;
this.accountSid = accountSid;
this.callbackUrl = callbackUrl;
this.defaultTtl = defaultTtl;
this.numberSelectionBehavior = numberSelectionBehavior;
this.geoMatchLevel = geoMatchLevel;
this.interceptCallbackUrl = interceptCallbackUrl;
this.outOfSessionCallbackUrl = outOfSessionCallbackUrl;
this.dateCreated = DateConverter.iso8601DateTimeFromString(dateCreated);
this.dateUpdated = DateConverter.iso8601DateTimeFromString(dateUpdated);
this.url = url;
this.links = links;
}
/**
* Returns The A string that uniquely identifies this Service..
*
* @return A string that uniquely identifies this Service.
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The A human-readable description of this resource..
*
* @return A human-readable description of this resource.
*/
public final String getUniqueName() {
return this.uniqueName;
}
/**
* Returns The Account Sid..
*
* @return Account Sid.
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The URL Twilio will send callbacks to.
*
* @return URL Twilio will send callbacks to
*/
public final URI getCallbackUrl() {
return this.callbackUrl;
}
/**
* Returns The Default TTL for a Session, in seconds..
*
* @return Default TTL for a Session, in seconds.
*/
public final Integer getDefaultTtl() {
return this.defaultTtl;
}
/**
* Returns The What behavior to use when choosing a proxy number..
*
* @return What behavior to use when choosing a proxy number.
*/
public final Service.NumberSelectionBehavior getNumberSelectionBehavior() {
return this.numberSelectionBehavior;
}
/**
* Returns The Whether proxy number selected must be in the same area code as
* the participant identifier..
*
* @return Whether proxy number selected must be in the same area code as the
* participant identifier.
*/
public final Service.GeoMatchLevel getGeoMatchLevel() {
return this.geoMatchLevel;
}
/**
* Returns The A URL for Twilio call before each Interaction..
*
* @return A URL for Twilio call before each Interaction.
*/
public final URI getInterceptCallbackUrl() {
return this.interceptCallbackUrl;
}
/**
* Returns The A URL for Twilio call when a new Interaction has no Session..
*
* @return A URL for Twilio call when a new Interaction has no Session.
*/
public final URI getOutOfSessionCallbackUrl() {
return this.outOfSessionCallbackUrl;
}
/**
* Returns The The date this Service was created.
*
* @return The date this Service was created
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The The date this Service was last updated.
*
* @return The date this Service was last updated
*/
public final DateTime getDateUpdated() {
return this.dateUpdated;
}
/**
* Returns The The URL of this resource..
*
* @return The URL of this resource.
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns The Nested resource URLs..
*
* @return Nested resource URLs.
*/
public final Map getLinks() {
return this.links;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Service other = (Service) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(uniqueName, other.uniqueName) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(callbackUrl, other.callbackUrl) &&
Objects.equals(defaultTtl, other.defaultTtl) &&
Objects.equals(numberSelectionBehavior, other.numberSelectionBehavior) &&
Objects.equals(geoMatchLevel, other.geoMatchLevel) &&
Objects.equals(interceptCallbackUrl, other.interceptCallbackUrl) &&
Objects.equals(outOfSessionCallbackUrl, other.outOfSessionCallbackUrl) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(dateUpdated, other.dateUpdated) &&
Objects.equals(url, other.url) &&
Objects.equals(links, other.links);
}
@Override
public int hashCode() {
return Objects.hash(sid,
uniqueName,
accountSid,
callbackUrl,
defaultTtl,
numberSelectionBehavior,
geoMatchLevel,
interceptCallbackUrl,
outOfSessionCallbackUrl,
dateCreated,
dateUpdated,
url,
links);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("uniqueName", uniqueName)
.add("accountSid", accountSid)
.add("callbackUrl", callbackUrl)
.add("defaultTtl", defaultTtl)
.add("numberSelectionBehavior", numberSelectionBehavior)
.add("geoMatchLevel", geoMatchLevel)
.add("interceptCallbackUrl", interceptCallbackUrl)
.add("outOfSessionCallbackUrl", outOfSessionCallbackUrl)
.add("dateCreated", dateCreated)
.add("dateUpdated", dateUpdated)
.add("url", url)
.add("links", links)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy