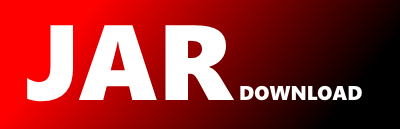
com.twilio.rest.taskrouter.v1.workspace.task.ReservationUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.taskrouter.v1.workspace.task;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
import java.util.List;
public class ReservationUpdater extends Updater {
private final String pathWorkspaceSid;
private final String pathTaskSid;
private final String pathSid;
private Reservation.Status reservationStatus;
private String workerActivitySid;
private String instruction;
private String dequeuePostWorkActivitySid;
private String dequeueFrom;
private String dequeueRecord;
private Integer dequeueTimeout;
private String dequeueTo;
private URI dequeueStatusCallbackUrl;
private String callFrom;
private String callRecord;
private Integer callTimeout;
private String callTo;
private URI callUrl;
private URI callStatusCallbackUrl;
private Boolean callAccept;
private String redirectCallSid;
private Boolean redirectAccept;
private URI redirectUrl;
private String to;
private String from;
private URI statusCallback;
private HttpMethod statusCallbackMethod;
private List statusCallbackEvent;
private Integer timeout;
private Boolean record;
private Boolean muted;
private String beep;
private Boolean startConferenceOnEnter;
private Boolean endConferenceOnExit;
private URI waitUrl;
private HttpMethod waitMethod;
private Boolean earlyMedia;
private Integer maxParticipants;
private URI conferenceStatusCallback;
private HttpMethod conferenceStatusCallbackMethod;
private List conferenceStatusCallbackEvent;
private String conferenceRecord;
private String conferenceTrim;
private String recordingChannels;
private URI recordingStatusCallback;
private HttpMethod recordingStatusCallbackMethod;
private URI conferenceRecordingStatusCallback;
private HttpMethod conferenceRecordingStatusCallbackMethod;
private String region;
private String sipAuthUsername;
private String sipAuthPassword;
private List dequeueStatusCallbackEvent;
private String postWorkActivitySid;
private Reservation.SupervisorMode supervisorMode;
private String supervisor;
/**
* Construct a new ReservationUpdater.
*
* @param pathWorkspaceSid The workspace_sid
* @param pathTaskSid The task_sid
* @param pathSid The sid
*/
public ReservationUpdater(final String pathWorkspaceSid,
final String pathTaskSid,
final String pathSid) {
this.pathWorkspaceSid = pathWorkspaceSid;
this.pathTaskSid = pathTaskSid;
this.pathSid = pathSid;
}
/**
* New reservation status.
*
* @param reservationStatus New reservation status
* @return this
*/
public ReservationUpdater setReservationStatus(final Reservation.Status reservationStatus) {
this.reservationStatus = reservationStatus;
return this;
}
/**
* New worker activity sid if rejecting a reservation.
*
* @param workerActivitySid New worker activity sid if rejecting a reservation
* @return this
*/
public ReservationUpdater setWorkerActivitySid(final String workerActivitySid) {
this.workerActivitySid = workerActivitySid;
return this;
}
/**
* Assignment instruction for reservation.
*
* @param instruction Assignment instruction for reservation
* @return this
*/
public ReservationUpdater setInstruction(final String instruction) {
this.instruction = instruction;
return this;
}
/**
* New worker activity sid after executing a Dequeue instruction.
*
* @param dequeuePostWorkActivitySid New worker activity sid after executing a
* Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeuePostWorkActivitySid(final String dequeuePostWorkActivitySid) {
this.dequeuePostWorkActivitySid = dequeuePostWorkActivitySid;
return this;
}
/**
* Caller ID for the call to the worker when executing a Dequeue instruction.
*
* @param dequeueFrom Caller ID for the call to the worker when executing a
* Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueFrom(final String dequeueFrom) {
this.dequeueFrom = dequeueFrom;
return this;
}
/**
* Attribute to record both legs of a call when executing a Dequeue instruction.
*
* @param dequeueRecord Attribute to record both legs of a call when executing
* a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueRecord(final String dequeueRecord) {
this.dequeueRecord = dequeueRecord;
return this;
}
/**
* Timeout for call when executing a Dequeue instruction.
*
* @param dequeueTimeout Timeout for call when executing a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueTimeout(final Integer dequeueTimeout) {
this.dequeueTimeout = dequeueTimeout;
return this;
}
/**
* Contact URI of the worker when executing a Dequeue instruction.
*
* @param dequeueTo Contact URI of the worker when executing a Dequeue
* instruction
* @return this
*/
public ReservationUpdater setDequeueTo(final String dequeueTo) {
this.dequeueTo = dequeueTo;
return this;
}
/**
* Callback URL for completed call event when executing a Dequeue instruction.
*
* @param dequeueStatusCallbackUrl Callback URL for completed call event when
* executing a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueStatusCallbackUrl(final URI dequeueStatusCallbackUrl) {
this.dequeueStatusCallbackUrl = dequeueStatusCallbackUrl;
return this;
}
/**
* Callback URL for completed call event when executing a Dequeue instruction.
*
* @param dequeueStatusCallbackUrl Callback URL for completed call event when
* executing a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueStatusCallbackUrl(final String dequeueStatusCallbackUrl) {
return setDequeueStatusCallbackUrl(Promoter.uriFromString(dequeueStatusCallbackUrl));
}
/**
* Caller ID for the outbound call when executing a Call instruction.
*
* @param callFrom Caller ID for the outbound call when executing a Call
* instruction
* @return this
*/
public ReservationUpdater setCallFrom(final String callFrom) {
this.callFrom = callFrom;
return this;
}
/**
* Attribute to record both legs of a call when executing a Call instruction.
*
* @param callRecord Attribute to record both legs of a call when executing a
* Call instruction
* @return this
*/
public ReservationUpdater setCallRecord(final String callRecord) {
this.callRecord = callRecord;
return this;
}
/**
* Timeout for call when executing a Call instruction.
*
* @param callTimeout Timeout for call when executing a Call instruction
* @return this
*/
public ReservationUpdater setCallTimeout(final Integer callTimeout) {
this.callTimeout = callTimeout;
return this;
}
/**
* Contact URI of the worker when executing a Call instruction.
*
* @param callTo Contact URI of the worker when executing a Call instruction
* @return this
*/
public ReservationUpdater setCallTo(final String callTo) {
this.callTo = callTo;
return this;
}
/**
* TwiML URI executed on answering the worker's leg as a result of the Call
* instruction.
*
* @param callUrl TwiML URI executed on answering the worker's leg as a result
* of the Call instruction
* @return this
*/
public ReservationUpdater setCallUrl(final URI callUrl) {
this.callUrl = callUrl;
return this;
}
/**
* TwiML URI executed on answering the worker's leg as a result of the Call
* instruction.
*
* @param callUrl TwiML URI executed on answering the worker's leg as a result
* of the Call instruction
* @return this
*/
public ReservationUpdater setCallUrl(final String callUrl) {
return setCallUrl(Promoter.uriFromString(callUrl));
}
/**
* Callback URL for completed call event when executing a Call instruction.
*
* @param callStatusCallbackUrl Callback URL for completed call event when
* executing a Call instruction
* @return this
*/
public ReservationUpdater setCallStatusCallbackUrl(final URI callStatusCallbackUrl) {
this.callStatusCallbackUrl = callStatusCallbackUrl;
return this;
}
/**
* Callback URL for completed call event when executing a Call instruction.
*
* @param callStatusCallbackUrl Callback URL for completed call event when
* executing a Call instruction
* @return this
*/
public ReservationUpdater setCallStatusCallbackUrl(final String callStatusCallbackUrl) {
return setCallStatusCallbackUrl(Promoter.uriFromString(callStatusCallbackUrl));
}
/**
* Flag to determine if reservation should be accepted when executing a Call
* instruction.
*
* @param callAccept Flag to determine if reservation should be accepted when
* executing a Call instruction
* @return this
*/
public ReservationUpdater setCallAccept(final Boolean callAccept) {
this.callAccept = callAccept;
return this;
}
/**
* Call sid of the call parked in the queue when executing a Redirect
* instruction.
*
* @param redirectCallSid Call sid of the call parked in the queue when
* executing a Redirect instruction
* @return this
*/
public ReservationUpdater setRedirectCallSid(final String redirectCallSid) {
this.redirectCallSid = redirectCallSid;
return this;
}
/**
* Flag to determine if reservation should be accepted when executing a Redirect
* instruction.
*
* @param redirectAccept Flag to determine if reservation should be accepted
* when executing a Redirect instruction
* @return this
*/
public ReservationUpdater setRedirectAccept(final Boolean redirectAccept) {
this.redirectAccept = redirectAccept;
return this;
}
/**
* TwiML URI to redirect the call to when executing the Redirect instruction.
*
* @param redirectUrl TwiML URI to redirect the call to when executing the
* Redirect instruction
* @return this
*/
public ReservationUpdater setRedirectUrl(final URI redirectUrl) {
this.redirectUrl = redirectUrl;
return this;
}
/**
* TwiML URI to redirect the call to when executing the Redirect instruction.
*
* @param redirectUrl TwiML URI to redirect the call to when executing the
* Redirect instruction
* @return this
*/
public ReservationUpdater setRedirectUrl(final String redirectUrl) {
return setRedirectUrl(Promoter.uriFromString(redirectUrl));
}
/**
* Contact URI of the worker when executing a Conference instruction.
*
* @param to Contact URI of the worker when executing a Conference instruction
* @return this
*/
public ReservationUpdater setTo(final String to) {
this.to = to;
return this;
}
/**
* Caller ID for the call to the worker when executing a Conference instruction.
*
* @param from Caller ID for the call to the worker when executing a Conference
* instruction
* @return this
*/
public ReservationUpdater setFrom(final String from) {
this.from = from;
return this;
}
/**
* The status_callback.
*
* @param statusCallback The status_callback
* @return this
*/
public ReservationUpdater setStatusCallback(final URI statusCallback) {
this.statusCallback = statusCallback;
return this;
}
/**
* The status_callback.
*
* @param statusCallback The status_callback
* @return this
*/
public ReservationUpdater setStatusCallback(final String statusCallback) {
return setStatusCallback(Promoter.uriFromString(statusCallback));
}
/**
* The status_callback_method.
*
* @param statusCallbackMethod The status_callback_method
* @return this
*/
public ReservationUpdater setStatusCallbackMethod(final HttpMethod statusCallbackMethod) {
this.statusCallbackMethod = statusCallbackMethod;
return this;
}
/**
* The status_callback_event.
*
* @param statusCallbackEvent The status_callback_event
* @return this
*/
public ReservationUpdater setStatusCallbackEvent(final List statusCallbackEvent) {
this.statusCallbackEvent = statusCallbackEvent;
return this;
}
/**
* The status_callback_event.
*
* @param statusCallbackEvent The status_callback_event
* @return this
*/
public ReservationUpdater setStatusCallbackEvent(final Reservation.CallStatus statusCallbackEvent) {
return setStatusCallbackEvent(Promoter.listOfOne(statusCallbackEvent));
}
/**
* Timeout for call when executing a Conference instruction.
*
* @param timeout Timeout for call when executing a Conference instruction
* @return this
*/
public ReservationUpdater setTimeout(final Integer timeout) {
this.timeout = timeout;
return this;
}
/**
* The record.
*
* @param record The record
* @return this
*/
public ReservationUpdater setRecord(final Boolean record) {
this.record = record;
return this;
}
/**
* The muted.
*
* @param muted The muted
* @return this
*/
public ReservationUpdater setMuted(final Boolean muted) {
this.muted = muted;
return this;
}
/**
* The beep.
*
* @param beep The beep
* @return this
*/
public ReservationUpdater setBeep(final String beep) {
this.beep = beep;
return this;
}
/**
* The start_conference_on_enter.
*
* @param startConferenceOnEnter The start_conference_on_enter
* @return this
*/
public ReservationUpdater setStartConferenceOnEnter(final Boolean startConferenceOnEnter) {
this.startConferenceOnEnter = startConferenceOnEnter;
return this;
}
/**
* The end_conference_on_exit.
*
* @param endConferenceOnExit The end_conference_on_exit
* @return this
*/
public ReservationUpdater setEndConferenceOnExit(final Boolean endConferenceOnExit) {
this.endConferenceOnExit = endConferenceOnExit;
return this;
}
/**
* The wait_url.
*
* @param waitUrl The wait_url
* @return this
*/
public ReservationUpdater setWaitUrl(final URI waitUrl) {
this.waitUrl = waitUrl;
return this;
}
/**
* The wait_url.
*
* @param waitUrl The wait_url
* @return this
*/
public ReservationUpdater setWaitUrl(final String waitUrl) {
return setWaitUrl(Promoter.uriFromString(waitUrl));
}
/**
* The wait_method.
*
* @param waitMethod The wait_method
* @return this
*/
public ReservationUpdater setWaitMethod(final HttpMethod waitMethod) {
this.waitMethod = waitMethod;
return this;
}
/**
* The early_media.
*
* @param earlyMedia The early_media
* @return this
*/
public ReservationUpdater setEarlyMedia(final Boolean earlyMedia) {
this.earlyMedia = earlyMedia;
return this;
}
/**
* The max_participants.
*
* @param maxParticipants The max_participants
* @return this
*/
public ReservationUpdater setMaxParticipants(final Integer maxParticipants) {
this.maxParticipants = maxParticipants;
return this;
}
/**
* The conference_status_callback.
*
* @param conferenceStatusCallback The conference_status_callback
* @return this
*/
public ReservationUpdater setConferenceStatusCallback(final URI conferenceStatusCallback) {
this.conferenceStatusCallback = conferenceStatusCallback;
return this;
}
/**
* The conference_status_callback.
*
* @param conferenceStatusCallback The conference_status_callback
* @return this
*/
public ReservationUpdater setConferenceStatusCallback(final String conferenceStatusCallback) {
return setConferenceStatusCallback(Promoter.uriFromString(conferenceStatusCallback));
}
/**
* The conference_status_callback_method.
*
* @param conferenceStatusCallbackMethod The conference_status_callback_method
* @return this
*/
public ReservationUpdater setConferenceStatusCallbackMethod(final HttpMethod conferenceStatusCallbackMethod) {
this.conferenceStatusCallbackMethod = conferenceStatusCallbackMethod;
return this;
}
/**
* The conference_status_callback_event.
*
* @param conferenceStatusCallbackEvent The conference_status_callback_event
* @return this
*/
public ReservationUpdater setConferenceStatusCallbackEvent(final List conferenceStatusCallbackEvent) {
this.conferenceStatusCallbackEvent = conferenceStatusCallbackEvent;
return this;
}
/**
* The conference_status_callback_event.
*
* @param conferenceStatusCallbackEvent The conference_status_callback_event
* @return this
*/
public ReservationUpdater setConferenceStatusCallbackEvent(final Reservation.ConferenceEvent conferenceStatusCallbackEvent) {
return setConferenceStatusCallbackEvent(Promoter.listOfOne(conferenceStatusCallbackEvent));
}
/**
* The conference_record.
*
* @param conferenceRecord The conference_record
* @return this
*/
public ReservationUpdater setConferenceRecord(final String conferenceRecord) {
this.conferenceRecord = conferenceRecord;
return this;
}
/**
* The conference_trim.
*
* @param conferenceTrim The conference_trim
* @return this
*/
public ReservationUpdater setConferenceTrim(final String conferenceTrim) {
this.conferenceTrim = conferenceTrim;
return this;
}
/**
* The recording_channels.
*
* @param recordingChannels The recording_channels
* @return this
*/
public ReservationUpdater setRecordingChannels(final String recordingChannels) {
this.recordingChannels = recordingChannels;
return this;
}
/**
* The recording_status_callback.
*
* @param recordingStatusCallback The recording_status_callback
* @return this
*/
public ReservationUpdater setRecordingStatusCallback(final URI recordingStatusCallback) {
this.recordingStatusCallback = recordingStatusCallback;
return this;
}
/**
* The recording_status_callback.
*
* @param recordingStatusCallback The recording_status_callback
* @return this
*/
public ReservationUpdater setRecordingStatusCallback(final String recordingStatusCallback) {
return setRecordingStatusCallback(Promoter.uriFromString(recordingStatusCallback));
}
/**
* The recording_status_callback_method.
*
* @param recordingStatusCallbackMethod The recording_status_callback_method
* @return this
*/
public ReservationUpdater setRecordingStatusCallbackMethod(final HttpMethod recordingStatusCallbackMethod) {
this.recordingStatusCallbackMethod = recordingStatusCallbackMethod;
return this;
}
/**
* The conference_recording_status_callback.
*
* @param conferenceRecordingStatusCallback The
* conference_recording_status_callback
* @return this
*/
public ReservationUpdater setConferenceRecordingStatusCallback(final URI conferenceRecordingStatusCallback) {
this.conferenceRecordingStatusCallback = conferenceRecordingStatusCallback;
return this;
}
/**
* The conference_recording_status_callback.
*
* @param conferenceRecordingStatusCallback The
* conference_recording_status_callback
* @return this
*/
public ReservationUpdater setConferenceRecordingStatusCallback(final String conferenceRecordingStatusCallback) {
return setConferenceRecordingStatusCallback(Promoter.uriFromString(conferenceRecordingStatusCallback));
}
/**
* The conference_recording_status_callback_method.
*
* @param conferenceRecordingStatusCallbackMethod The
* conference_recording_status_callback_method
* @return this
*/
public ReservationUpdater setConferenceRecordingStatusCallbackMethod(final HttpMethod conferenceRecordingStatusCallbackMethod) {
this.conferenceRecordingStatusCallbackMethod = conferenceRecordingStatusCallbackMethod;
return this;
}
/**
* The region.
*
* @param region The region
* @return this
*/
public ReservationUpdater setRegion(final String region) {
this.region = region;
return this;
}
/**
* The sip_auth_username.
*
* @param sipAuthUsername The sip_auth_username
* @return this
*/
public ReservationUpdater setSipAuthUsername(final String sipAuthUsername) {
this.sipAuthUsername = sipAuthUsername;
return this;
}
/**
* The sip_auth_password.
*
* @param sipAuthPassword The sip_auth_password
* @return this
*/
public ReservationUpdater setSipAuthPassword(final String sipAuthPassword) {
this.sipAuthPassword = sipAuthPassword;
return this;
}
/**
* Call progress events sent via webhooks as a result of a Dequeue instruction.
*
* @param dequeueStatusCallbackEvent Call progress events sent via webhooks as
* a result of a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueStatusCallbackEvent(final List dequeueStatusCallbackEvent) {
this.dequeueStatusCallbackEvent = dequeueStatusCallbackEvent;
return this;
}
/**
* Call progress events sent via webhooks as a result of a Dequeue instruction.
*
* @param dequeueStatusCallbackEvent Call progress events sent via webhooks as
* a result of a Dequeue instruction
* @return this
*/
public ReservationUpdater setDequeueStatusCallbackEvent(final String dequeueStatusCallbackEvent) {
return setDequeueStatusCallbackEvent(Promoter.listOfOne(dequeueStatusCallbackEvent));
}
/**
* New worker activity sid after executing a Conference instruction.
*
* @param postWorkActivitySid New worker activity sid after executing a
* Conference instruction
* @return this
*/
public ReservationUpdater setPostWorkActivitySid(final String postWorkActivitySid) {
this.postWorkActivitySid = postWorkActivitySid;
return this;
}
/**
* Supervisor mode when executing the Supervise instruction.
*
* @param supervisorMode Supervisor mode when executing the Supervise
* instruction
* @return this
*/
public ReservationUpdater setSupervisorMode(final Reservation.SupervisorMode supervisorMode) {
this.supervisorMode = supervisorMode;
return this;
}
/**
* Supervisor sid/uri when executing the Supervise instruction.
*
* @param supervisor Supervisor sid/uri when executing the Supervise instruction
* @return this
*/
public ReservationUpdater setSupervisor(final String supervisor) {
this.supervisor = supervisor;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Reservation
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Reservation update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.TASKROUTER.toString(),
"/v1/Workspaces/" + this.pathWorkspaceSid + "/Tasks/" + this.pathTaskSid + "/Reservations/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Reservation update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Reservation.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (reservationStatus != null) {
request.addPostParam("ReservationStatus", reservationStatus.toString());
}
if (workerActivitySid != null) {
request.addPostParam("WorkerActivitySid", workerActivitySid);
}
if (instruction != null) {
request.addPostParam("Instruction", instruction);
}
if (dequeuePostWorkActivitySid != null) {
request.addPostParam("DequeuePostWorkActivitySid", dequeuePostWorkActivitySid);
}
if (dequeueFrom != null) {
request.addPostParam("DequeueFrom", dequeueFrom);
}
if (dequeueRecord != null) {
request.addPostParam("DequeueRecord", dequeueRecord);
}
if (dequeueTimeout != null) {
request.addPostParam("DequeueTimeout", dequeueTimeout.toString());
}
if (dequeueTo != null) {
request.addPostParam("DequeueTo", dequeueTo);
}
if (dequeueStatusCallbackUrl != null) {
request.addPostParam("DequeueStatusCallbackUrl", dequeueStatusCallbackUrl.toString());
}
if (callFrom != null) {
request.addPostParam("CallFrom", callFrom);
}
if (callRecord != null) {
request.addPostParam("CallRecord", callRecord);
}
if (callTimeout != null) {
request.addPostParam("CallTimeout", callTimeout.toString());
}
if (callTo != null) {
request.addPostParam("CallTo", callTo);
}
if (callUrl != null) {
request.addPostParam("CallUrl", callUrl.toString());
}
if (callStatusCallbackUrl != null) {
request.addPostParam("CallStatusCallbackUrl", callStatusCallbackUrl.toString());
}
if (callAccept != null) {
request.addPostParam("CallAccept", callAccept.toString());
}
if (redirectCallSid != null) {
request.addPostParam("RedirectCallSid", redirectCallSid);
}
if (redirectAccept != null) {
request.addPostParam("RedirectAccept", redirectAccept.toString());
}
if (redirectUrl != null) {
request.addPostParam("RedirectUrl", redirectUrl.toString());
}
if (to != null) {
request.addPostParam("To", to);
}
if (from != null) {
request.addPostParam("From", from);
}
if (statusCallback != null) {
request.addPostParam("StatusCallback", statusCallback.toString());
}
if (statusCallbackMethod != null) {
request.addPostParam("StatusCallbackMethod", statusCallbackMethod.toString());
}
if (statusCallbackEvent != null) {
for (Reservation.CallStatus prop : statusCallbackEvent) {
request.addPostParam("StatusCallbackEvent", prop.toString());
}
}
if (timeout != null) {
request.addPostParam("Timeout", timeout.toString());
}
if (record != null) {
request.addPostParam("Record", record.toString());
}
if (muted != null) {
request.addPostParam("Muted", muted.toString());
}
if (beep != null) {
request.addPostParam("Beep", beep);
}
if (startConferenceOnEnter != null) {
request.addPostParam("StartConferenceOnEnter", startConferenceOnEnter.toString());
}
if (endConferenceOnExit != null) {
request.addPostParam("EndConferenceOnExit", endConferenceOnExit.toString());
}
if (waitUrl != null) {
request.addPostParam("WaitUrl", waitUrl.toString());
}
if (waitMethod != null) {
request.addPostParam("WaitMethod", waitMethod.toString());
}
if (earlyMedia != null) {
request.addPostParam("EarlyMedia", earlyMedia.toString());
}
if (maxParticipants != null) {
request.addPostParam("MaxParticipants", maxParticipants.toString());
}
if (conferenceStatusCallback != null) {
request.addPostParam("ConferenceStatusCallback", conferenceStatusCallback.toString());
}
if (conferenceStatusCallbackMethod != null) {
request.addPostParam("ConferenceStatusCallbackMethod", conferenceStatusCallbackMethod.toString());
}
if (conferenceStatusCallbackEvent != null) {
for (Reservation.ConferenceEvent prop : conferenceStatusCallbackEvent) {
request.addPostParam("ConferenceStatusCallbackEvent", prop.toString());
}
}
if (conferenceRecord != null) {
request.addPostParam("ConferenceRecord", conferenceRecord);
}
if (conferenceTrim != null) {
request.addPostParam("ConferenceTrim", conferenceTrim);
}
if (recordingChannels != null) {
request.addPostParam("RecordingChannels", recordingChannels);
}
if (recordingStatusCallback != null) {
request.addPostParam("RecordingStatusCallback", recordingStatusCallback.toString());
}
if (recordingStatusCallbackMethod != null) {
request.addPostParam("RecordingStatusCallbackMethod", recordingStatusCallbackMethod.toString());
}
if (conferenceRecordingStatusCallback != null) {
request.addPostParam("ConferenceRecordingStatusCallback", conferenceRecordingStatusCallback.toString());
}
if (conferenceRecordingStatusCallbackMethod != null) {
request.addPostParam("ConferenceRecordingStatusCallbackMethod", conferenceRecordingStatusCallbackMethod.toString());
}
if (region != null) {
request.addPostParam("Region", region);
}
if (sipAuthUsername != null) {
request.addPostParam("SipAuthUsername", sipAuthUsername);
}
if (sipAuthPassword != null) {
request.addPostParam("SipAuthPassword", sipAuthPassword);
}
if (dequeueStatusCallbackEvent != null) {
for (String prop : dequeueStatusCallbackEvent) {
request.addPostParam("DequeueStatusCallbackEvent", prop);
}
}
if (postWorkActivitySid != null) {
request.addPostParam("PostWorkActivitySid", postWorkActivitySid);
}
if (supervisorMode != null) {
request.addPostParam("SupervisorMode", supervisorMode.toString());
}
if (supervisor != null) {
request.addPostParam("Supervisor", supervisor);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy