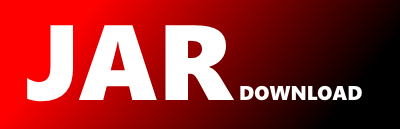
com.twilio.rest.api.v2010.account.usage.TriggerUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.api.v2010.account.usage;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
public class TriggerUpdater extends Updater {
private String pathAccountSid;
private final String pathSid;
private HttpMethod callbackMethod;
private URI callbackUrl;
private String friendlyName;
/**
* Construct a new TriggerUpdater.
*
* @param pathSid The sid
*/
public TriggerUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* Construct a new TriggerUpdater.
*
* @param pathAccountSid The account_sid
* @param pathSid The sid
*/
public TriggerUpdater(final String pathAccountSid,
final String pathSid) {
this.pathAccountSid = pathAccountSid;
this.pathSid = pathSid;
}
/**
* The HTTP method Twilio will use when making a request to the CallbackUrl.
* `GET` or `POST`..
*
* @param callbackMethod HTTP method to use with callback_url
* @return this
*/
public TriggerUpdater setCallbackMethod(final HttpMethod callbackMethod) {
this.callbackMethod = callbackMethod;
return this;
}
/**
* Twilio will make a request to this url when the trigger fires..
*
* @param callbackUrl URL Twilio will request when the trigger fires
* @return this
*/
public TriggerUpdater setCallbackUrl(final URI callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
/**
* Twilio will make a request to this url when the trigger fires..
*
* @param callbackUrl URL Twilio will request when the trigger fires
* @return this
*/
public TriggerUpdater setCallbackUrl(final String callbackUrl) {
return setCallbackUrl(Promoter.uriFromString(callbackUrl));
}
/**
* A user-specified, human-readable name for the trigger..
*
* @param friendlyName A user-specified, human-readable name for the trigger.
* @return this
*/
public TriggerUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated Trigger
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Trigger update(final TwilioRestClient client) {
this.pathAccountSid = this.pathAccountSid == null ? client.getAccountSid() : this.pathAccountSid;
Request request = new Request(
HttpMethod.POST,
Domains.API.toString(),
"/2010-04-01/Accounts/" + this.pathAccountSid + "/Usage/Triggers/" + this.pathSid + ".json",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Trigger update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Trigger.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (callbackMethod != null) {
request.addPostParam("CallbackMethod", callbackMethod.toString());
}
if (callbackUrl != null) {
request.addPostParam("CallbackUrl", callbackUrl.toString());
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy