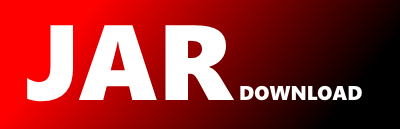
com.twilio.rest.notify.v1.service.Binding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.notify.v1.service;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.DateConverter;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class Binding extends Resource {
private static final long serialVersionUID = 19604548272115L;
public enum BindingType {
APN("apn"),
GCM("gcm"),
SMS("sms"),
FCM("fcm"),
FACEBOOK_MESSENGER("facebook-messenger"),
ALEXA("alexa");
private final String value;
private BindingType(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a BindingType from a string.
* @param value string value
* @return generated BindingType
*/
@JsonCreator
public static BindingType forValue(final String value) {
return Promoter.enumFromString(value, BindingType.values());
}
}
/**
* Create a BindingFetcher to execute fetch.
*
* @param pathServiceSid The service_sid
* @param pathSid The sid
* @return BindingFetcher capable of executing the fetch
*/
public static BindingFetcher fetcher(final String pathServiceSid,
final String pathSid) {
return new BindingFetcher(pathServiceSid, pathSid);
}
/**
* Create a BindingDeleter to execute delete.
*
* @param pathServiceSid The service_sid
* @param pathSid The sid
* @return BindingDeleter capable of executing the delete
*/
public static BindingDeleter deleter(final String pathServiceSid,
final String pathSid) {
return new BindingDeleter(pathServiceSid, pathSid);
}
/**
* Create a BindingCreator to execute create.
*
* @param pathServiceSid The service_sid
* @param identity The Identity to which this Binding belongs to.
* @param bindingType The type of the Binding.
* @param address The address specific to the channel.
* @return BindingCreator capable of executing the create
*/
public static BindingCreator creator(final String pathServiceSid,
final String identity,
final Binding.BindingType bindingType,
final String address) {
return new BindingCreator(pathServiceSid, identity, bindingType, address);
}
/**
* Create a BindingReader to execute read.
*
* @param pathServiceSid The service_sid
* @return BindingReader capable of executing the read
*/
public static BindingReader reader(final String pathServiceSid) {
return new BindingReader(pathServiceSid);
}
/**
* Converts a JSON String into a Binding object using the provided ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Binding object represented by the provided JSON
*/
public static Binding fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Binding.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Binding object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Binding object represented by the provided JSON
*/
public static Binding fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Binding.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String accountSid;
private final String serviceSid;
private final String credentialSid;
private final DateTime dateCreated;
private final DateTime dateUpdated;
private final String notificationProtocolVersion;
private final String endpoint;
private final String identity;
private final String bindingType;
private final String address;
private final List tags;
private final URI url;
private final Map links;
@JsonCreator
private Binding(@JsonProperty("sid")
final String sid,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("service_sid")
final String serviceSid,
@JsonProperty("credential_sid")
final String credentialSid,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("date_updated")
final String dateUpdated,
@JsonProperty("notification_protocol_version")
final String notificationProtocolVersion,
@JsonProperty("endpoint")
final String endpoint,
@JsonProperty("identity")
final String identity,
@JsonProperty("binding_type")
final String bindingType,
@JsonProperty("address")
final String address,
@JsonProperty("tags")
final List tags,
@JsonProperty("url")
final URI url,
@JsonProperty("links")
final Map links) {
this.sid = sid;
this.accountSid = accountSid;
this.serviceSid = serviceSid;
this.credentialSid = credentialSid;
this.dateCreated = DateConverter.iso8601DateTimeFromString(dateCreated);
this.dateUpdated = DateConverter.iso8601DateTimeFromString(dateUpdated);
this.notificationProtocolVersion = notificationProtocolVersion;
this.endpoint = endpoint;
this.identity = identity;
this.bindingType = bindingType;
this.address = address;
this.tags = tags;
this.url = url;
this.links = links;
}
/**
* Returns The The sid.
*
* @return The sid
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The The account_sid.
*
* @return The account_sid
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The The service_sid.
*
* @return The service_sid
*/
public final String getServiceSid() {
return this.serviceSid;
}
/**
* Returns The The unique identifier of the Credential resource to be used to
* send notifications to this Binding..
*
* @return The unique identifier of the Credential resource to be used to send
* notifications to this Binding.
*/
public final String getCredentialSid() {
return this.credentialSid;
}
/**
* Returns The The date_created.
*
* @return The date_created
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The The date_updated.
*
* @return The date_updated
*/
public final DateTime getDateUpdated() {
return this.dateUpdated;
}
/**
* Returns The The version of the protocol used to send the notification..
*
* @return The version of the protocol used to send the notification.
*/
public final String getNotificationProtocolVersion() {
return this.notificationProtocolVersion;
}
/**
* Returns The DEPRECATED*.
*
* @return DEPRECATED*
*/
public final String getEndpoint() {
return this.endpoint;
}
/**
* Returns The The Identity to which this Binding belongs to..
*
* @return The Identity to which this Binding belongs to.
*/
public final String getIdentity() {
return this.identity;
}
/**
* Returns The The type of the Binding..
*
* @return The type of the Binding.
*/
public final String getBindingType() {
return this.bindingType;
}
/**
* Returns The The address specific to the channel..
*
* @return The address specific to the channel.
*/
public final String getAddress() {
return this.address;
}
/**
* Returns The The list of tags associated with this Binding..
*
* @return The list of tags associated with this Binding.
*/
public final List getTags() {
return this.tags;
}
/**
* Returns The The url.
*
* @return The url
*/
public final URI getUrl() {
return this.url;
}
/**
* Returns The The links.
*
* @return The links
*/
public final Map getLinks() {
return this.links;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Binding other = (Binding) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(serviceSid, other.serviceSid) &&
Objects.equals(credentialSid, other.credentialSid) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(dateUpdated, other.dateUpdated) &&
Objects.equals(notificationProtocolVersion, other.notificationProtocolVersion) &&
Objects.equals(endpoint, other.endpoint) &&
Objects.equals(identity, other.identity) &&
Objects.equals(bindingType, other.bindingType) &&
Objects.equals(address, other.address) &&
Objects.equals(tags, other.tags) &&
Objects.equals(url, other.url) &&
Objects.equals(links, other.links);
}
@Override
public int hashCode() {
return Objects.hash(sid,
accountSid,
serviceSid,
credentialSid,
dateCreated,
dateUpdated,
notificationProtocolVersion,
endpoint,
identity,
bindingType,
address,
tags,
url,
links);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("accountSid", accountSid)
.add("serviceSid", serviceSid)
.add("credentialSid", credentialSid)
.add("dateCreated", dateCreated)
.add("dateUpdated", dateUpdated)
.add("notificationProtocolVersion", notificationProtocolVersion)
.add("endpoint", endpoint)
.add("identity", identity)
.add("bindingType", bindingType)
.add("address", address)
.add("tags", tags)
.add("url", url)
.add("links", links)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy