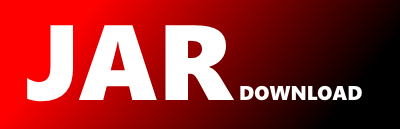
com.twilio.base.Reader Maven / Gradle / Ivy
package com.twilio.base;
import com.google.common.util.concurrent.ListenableFuture;
import com.twilio.Twilio;
import com.twilio.http.TwilioRestClient;
import java.util.concurrent.Callable;
/**
* Executor for listing of a resource.
*
* @param type of the resource
*/
public abstract class Reader {
private Integer pageSize;
private Long limit;
/**
* Execute a request using default client.
*
* @return ResourceSet of objects
*/
public ResourceSet read() {
return read(Twilio.getRestClient());
}
/**
* Execute a request using specified client.
*
* @param client client used to make request
* @return ResourceSet of objects
*/
public abstract ResourceSet read(final TwilioRestClient client);
/**
* Execute an async request using default client.
*
* @return future that resolves to the ResourceSet of objects
*/
public ListenableFuture> readAsync() {
return readAsync(Twilio.getRestClient());
}
/**
* Execute an async request using specified client.
*
* @param client client used to make request
* @return future that resolves to the ResourceSet of objects
*/
public ListenableFuture> readAsync(final TwilioRestClient client) {
return Twilio.getExecutorService().submit(new Callable>() {
public ResourceSet call() {
return read(client);
}
});
}
/**
* Fetch the first page of resources.
*
* @return Page containing the first pageSize of resources
*/
public Page firstPage() {
return firstPage(Twilio.getRestClient());
}
/**
* Fetch the first page of resources using specified client.
*
* @param client client used to fetch
* @return Page containing the first pageSize of resources
*/
public abstract Page firstPage(final TwilioRestClient client);
/**
* Retrieve the target page of resources.
*
* @param targetUrl API-generated URL for the requested results page
* @return Page containing the target pageSize of resources
*/
public Page getPage(final String targetUrl) {
return getPage(targetUrl, Twilio.getRestClient());
}
/**
* Retrieve the target page of resources.
*
* @param targetUrl API-generated URL for the requested results page
* @param client client used to fetch
* @return Page containing the target pageSize of resources
*/
public abstract Page getPage(final String targetUrl, final TwilioRestClient client);
/**
* Fetch the following page of resources.
*
* @param page current page of resources
* @return Page containing the next pageSize of resources
*/
public Page nextPage(final Page page) {
return nextPage(page, Twilio.getRestClient());
}
/**
* Fetch the following page of resources using specified client.
*
* @param page current page of resources
* @param client client used to fetch
* @return Page containing the next pageSize of resources
*/
public abstract Page nextPage(final Page page, final TwilioRestClient client);
/**
* Fetch the prior page of resources.
*
* @param page current page of resources
* @return Page containing the previous pageSize of resources
*/
public Page previousPage(final Page page) {
return previousPage(page, Twilio.getRestClient());
}
/**
* Fetch the prior page of resources using specified client.
*
* @param page current page of resources
* @param client client used to fetch
* @return Page containing the previous pageSize of resources
*/
public abstract Page previousPage(final Page page, final TwilioRestClient client);
public Integer getPageSize() {
return pageSize;
}
public Reader pageSize(final int pageSize) {
this.pageSize = pageSize;
return this;
}
public Long getLimit() {
return limit;
}
/**
* Sets the max number of records to read.
*
* @param limit max number of records to read
* @return this reader
*/
public Reader limit(final long limit) {
this.limit = limit;
if (this.pageSize == null) {
this.pageSize = (new Long(this.limit)).intValue();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy