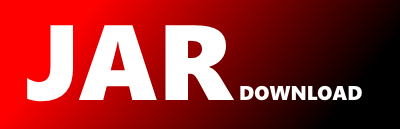
com.twilio.rest.video.v1.CompositionSettingsCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.video.v1;
import com.twilio.base.Creator;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
/**
* PLEASE NOTE that this class contains preview products that are subject to
* change. Use them with caution. If you currently do not have developer preview
* access, please contact [email protected].
*/
public class CompositionSettingsCreator extends Creator {
private final String friendlyName;
private String awsCredentialsSid;
private String encryptionKeySid;
private URI awsS3Url;
private Boolean awsStorageEnabled;
private Boolean encryptionEnabled;
/**
* Construct a new CompositionSettingsCreator.
*
* @param friendlyName Friendly name of the configuration to be shown in the
* console
*/
public CompositionSettingsCreator(final String friendlyName) {
this.friendlyName = friendlyName;
}
/**
* SID of the Stored Credential resource `CRxx`.
*
* @param awsCredentialsSid SID of the Stored Credential resource CRxx
* @return this
*/
public CompositionSettingsCreator setAwsCredentialsSid(final String awsCredentialsSid) {
this.awsCredentialsSid = awsCredentialsSid;
return this;
}
/**
* SID of the Public Key resource `CRxx`.
*
* @param encryptionKeySid SID of the Public Key resource CRxx
* @return this
*/
public CompositionSettingsCreator setEncryptionKeySid(final String encryptionKeySid) {
this.encryptionKeySid = encryptionKeySid;
return this;
}
/**
* Identity of the external location where the compositions should be stored. We
* only support DNS-compliant URLs like
* `http://<my-bucket>.s3-<aws-region>.amazonaws.com/compositions`,
* where `compositions` is the path where you want compositions to be stored..
*
* @param awsS3Url Identity of the external location where the compositions
* should be stored. We only support DNS-compliant URLs like
* http://<my-bucket>.s3-<aws-region>.amazonaws.com/compositions, where compositions is the path where you want compositions to be stored.
* @return this
*/
public CompositionSettingsCreator setAwsS3Url(final URI awsS3Url) {
this.awsS3Url = awsS3Url;
return this;
}
/**
* Identity of the external location where the compositions should be stored. We
* only support DNS-compliant URLs like
* `http://<my-bucket>.s3-<aws-region>.amazonaws.com/compositions`,
* where `compositions` is the path where you want compositions to be stored..
*
* @param awsS3Url Identity of the external location where the compositions
* should be stored. We only support DNS-compliant URLs like
* http://<my-bucket>.s3-<aws-region>.amazonaws.com/compositions, where compositions is the path where you want compositions to be stored.
* @return this
*/
public CompositionSettingsCreator setAwsS3Url(final String awsS3Url) {
return setAwsS3Url(Promoter.uriFromString(awsS3Url));
}
/**
* `true|false` When set to `true`, all Compositions will be written to the
* `AwsS3Url` specified above. When set to `false`, all Compositions will be
* stored in Twilio's cloud..
*
* @param awsStorageEnabled true|false When set to true, all Compositions will
* be written to the AwsS3Url specified above. When set
* to false, all Compositions will be stored in
* Twilio's cloud.
* @return this
*/
public CompositionSettingsCreator setAwsStorageEnabled(final Boolean awsStorageEnabled) {
this.awsStorageEnabled = awsStorageEnabled;
return this;
}
/**
* `true|false` When set to `true`, all Compositions will be stored encrypted.
* Dafault value is `false`.
*
* @param encryptionEnabled true|false When set to true, all Compositions will
* be stored encrypted.
* @return this
*/
public CompositionSettingsCreator setEncryptionEnabled(final Boolean encryptionEnabled) {
this.encryptionEnabled = encryptionEnabled;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created CompositionSettings
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public CompositionSettings create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.VIDEO.toString(),
"/v1/CompositionSettings/Default",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("CompositionSettings creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return CompositionSettings.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (awsCredentialsSid != null) {
request.addPostParam("AwsCredentialsSid", awsCredentialsSid);
}
if (encryptionKeySid != null) {
request.addPostParam("EncryptionKeySid", encryptionKeySid);
}
if (awsS3Url != null) {
request.addPostParam("AwsS3Url", awsS3Url.toString());
}
if (awsStorageEnabled != null) {
request.addPostParam("AwsStorageEnabled", awsStorageEnabled.toString());
}
if (encryptionEnabled != null) {
request.addPostParam("EncryptionEnabled", encryptionEnabled.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy