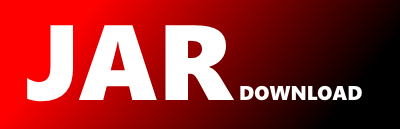
com.twilio.converter.PrefixedCollapsibleMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
package com.twilio.converter;
import com.google.common.base.Joiner;
import com.google.common.collect.Lists;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PrefixedCollapsibleMap {
private static Map flatten(
Map map,
Map result,
List previous
) {
for (Map.Entry entry : map.entrySet()) {
List next = Lists.newArrayList(previous);
next.add(entry.getKey());
if (entry.getValue() instanceof Map) {
flatten((Map)entry.getValue(), result, next);
} else {
result.put(Joiner.on('.').join(next), entry.getValue().toString());
}
}
return result;
}
/**
* Flatten a Map of String, Object into a Map of String, String where keys are '.' separated
* and prepends a key.
*
* @param map map to transform
* @param prefix key to prepend
* @return flattened map
*/
public static Map serialize(Map map, String prefix) {
if (map == null || map.isEmpty()) {
return Collections.emptyMap();
}
Map flattened = flatten(map, new HashMap(), new ArrayList());
Map result = new HashMap<>();
for (Map.Entry entry : flattened.entrySet()) {
result.put(prefix + "." + entry.getKey(), entry.getValue());
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy