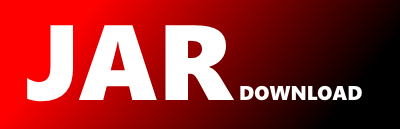
com.twilio.rest.flexapi.v1.FlexFlowUpdater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.flexapi.v1;
import com.twilio.base.Updater;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import java.net.URI;
public class FlexFlowUpdater extends Updater {
private final String pathSid;
private String friendlyName;
private String chatServiceSid;
private FlexFlow.ChannelType channelType;
private String contactIdentity;
private Boolean enabled;
private FlexFlow.IntegrationType integrationType;
private String integrationFlowSid;
private URI integrationUrl;
private String integrationWorkspaceSid;
private String integrationWorkflowSid;
private String integrationChannel;
private Integer integrationTimeout;
private Integer integrationPriority;
private Boolean integrationCreationOnMessage;
private Boolean longLived;
/**
* Construct a new FlexFlowUpdater.
*
* @param pathSid The unique ID of the FlexFlow
*/
public FlexFlowUpdater(final String pathSid) {
this.pathSid = pathSid;
}
/**
* Human readable description of this FlexFlow.
*
* @param friendlyName Human readable description of this FlexFlow
* @return this
*/
public FlexFlowUpdater setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The unique SID identifier of the chat service.
*
* @param chatServiceSid Service Sid.
* @return this
*/
public FlexFlowUpdater setChatServiceSid(final String chatServiceSid) {
this.chatServiceSid = chatServiceSid;
return this;
}
/**
* Channel type (web | facebook | sms).
*
* @param channelType Channel type
* @return this
*/
public FlexFlowUpdater setChannelType(final FlexFlow.ChannelType channelType) {
this.channelType = channelType;
return this;
}
/**
* Channel contact Identity (number / contact).
*
* @param contactIdentity Channel contact Identity
* @return this
*/
public FlexFlowUpdater setContactIdentity(final String contactIdentity) {
this.contactIdentity = contactIdentity;
return this;
}
/**
* Boolean flag for enabling or disabling the FlexFlow.
*
* @param enabled Boolean flag for enabling or disabling the FlexFlow
* @return this
*/
public FlexFlowUpdater setEnabled(final Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Integration type (studio | external | task).
*
* @param integrationType Integration type
* @return this
*/
public FlexFlowUpdater setIntegrationType(final FlexFlow.IntegrationType integrationType) {
this.integrationType = integrationType;
return this;
}
/**
* The unique SID identifier of the Flow for Studio integration type.
*
* @param integrationFlowSid Flow Sid.
* @return this
*/
public FlexFlowUpdater setIntegrationFlowSid(final String integrationFlowSid) {
this.integrationFlowSid = integrationFlowSid;
return this;
}
/**
* External Webhook Url for External integration type.
*
* @param integrationUrl External Webhook Url
* @return this
*/
public FlexFlowUpdater setIntegrationUrl(final URI integrationUrl) {
this.integrationUrl = integrationUrl;
return this;
}
/**
* External Webhook Url for External integration type.
*
* @param integrationUrl External Webhook Url
* @return this
*/
public FlexFlowUpdater setIntegrationUrl(final String integrationUrl) {
return setIntegrationUrl(Promoter.uriFromString(integrationUrl));
}
/**
* Workspace Sid for a new task for Task integration type.
*
* @param integrationWorkspaceSid Workspace Sid for a new task
* @return this
*/
public FlexFlowUpdater setIntegrationWorkspaceSid(final String integrationWorkspaceSid) {
this.integrationWorkspaceSid = integrationWorkspaceSid;
return this;
}
/**
* Workflow Sid for a new task for Task integration type.
*
* @param integrationWorkflowSid Workflow Sid for a new task
* @return this
*/
public FlexFlowUpdater setIntegrationWorkflowSid(final String integrationWorkflowSid) {
this.integrationWorkflowSid = integrationWorkflowSid;
return this;
}
/**
* Task Channel for a new task for Task integration type (default is 'default').
*
* @param integrationChannel Task Channel for a new task
* @return this
*/
public FlexFlowUpdater setIntegrationChannel(final String integrationChannel) {
this.integrationChannel = integrationChannel;
return this;
}
/**
* Task timeout in seconds for a new task for Task integration type (default
* 86400).
*
* @param integrationTimeout Task timeout in seconds for a new task
* @return this
*/
public FlexFlowUpdater setIntegrationTimeout(final Integer integrationTimeout) {
this.integrationTimeout = integrationTimeout;
return this;
}
/**
* Task priority for a new task for Task integration type (default 0).
*
* @param integrationPriority Task priority for a new task
* @return this
*/
public FlexFlowUpdater setIntegrationPriority(final Integer integrationPriority) {
this.integrationPriority = integrationPriority;
return this;
}
/**
* Flag for task creation, either creating task with the channel, or if true
* create task whwn first message arrives (for Task integration type).
*
* @param integrationCreationOnMessage Flag for task creation
* @return this
*/
public FlexFlowUpdater setIntegrationCreationOnMessage(final Boolean integrationCreationOnMessage) {
this.integrationCreationOnMessage = integrationCreationOnMessage;
return this;
}
/**
* Default Flag defining whether the new channels created are long lived or not.
*
* @param longLived Long Lived flag for new Channel
* @return this
*/
public FlexFlowUpdater setLongLived(final Boolean longLived) {
this.longLived = longLived;
return this;
}
/**
* Make the request to the Twilio API to perform the update.
*
* @param client TwilioRestClient with which to make the request
* @return Updated FlexFlow
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public FlexFlow update(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.FLEXAPI.toString(),
"/v1/FlexFlows/" + this.pathSid + "",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("FlexFlow update failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return FlexFlow.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (chatServiceSid != null) {
request.addPostParam("ChatServiceSid", chatServiceSid);
}
if (channelType != null) {
request.addPostParam("ChannelType", channelType.toString());
}
if (contactIdentity != null) {
request.addPostParam("ContactIdentity", contactIdentity);
}
if (enabled != null) {
request.addPostParam("Enabled", enabled.toString());
}
if (integrationType != null) {
request.addPostParam("IntegrationType", integrationType.toString());
}
if (integrationFlowSid != null) {
request.addPostParam("Integration.FlowSid", integrationFlowSid);
}
if (integrationUrl != null) {
request.addPostParam("Integration.Url", integrationUrl.toString());
}
if (integrationWorkspaceSid != null) {
request.addPostParam("Integration.WorkspaceSid", integrationWorkspaceSid);
}
if (integrationWorkflowSid != null) {
request.addPostParam("Integration.WorkflowSid", integrationWorkflowSid);
}
if (integrationChannel != null) {
request.addPostParam("Integration.Channel", integrationChannel);
}
if (integrationTimeout != null) {
request.addPostParam("Integration.Timeout", integrationTimeout.toString());
}
if (integrationPriority != null) {
request.addPostParam("Integration.Priority", integrationPriority.toString());
}
if (integrationCreationOnMessage != null) {
request.addPostParam("Integration.CreationOnMessage", integrationCreationOnMessage.toString());
}
if (longLived != null) {
request.addPostParam("LongLived", longLived.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy