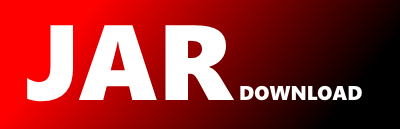
com.twilio.rest.messaging.v1.SessionCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.messaging.v1;
import com.twilio.base.Creator;
import com.twilio.converter.DateConverter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
/**
* PLEASE NOTE that this class contains preview products that are subject to
* change. Use them with caution. If you currently do not have developer preview
* access, please contact [email protected].
*/
public class SessionCreator extends Creator {
private final String messagingServiceSid;
private String friendlyName;
private String attributes;
private DateTime dateCreated;
private DateTime dateUpdated;
private String createdBy;
/**
* Construct a new SessionCreator.
*
* @param messagingServiceSid The unique id of the SMS Service this session
* belongs to.
*/
public SessionCreator(final String messagingServiceSid) {
this.messagingServiceSid = messagingServiceSid;
}
/**
* The human-readable name of this session. Optional..
*
* @param friendlyName The human-readable name of this session.
* @return this
*/
public SessionCreator setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* An optional string metadata field you can use to store any data you wish. The
* string value must contain structurally valid JSON if specified. **Note**
* that if the attributes are not set "{}" will be returned..
*
* @param attributes An optional string metadata field you can use to store any
* data you wish.
* @return this
*/
public SessionCreator setAttributes(final String attributes) {
this.attributes = attributes;
return this;
}
/**
* The date that this resource was created..
*
* @param dateCreated The date that this resource was created.
* @return this
*/
public SessionCreator setDateCreated(final DateTime dateCreated) {
this.dateCreated = dateCreated;
return this;
}
/**
* The date that this resource was last updated..
*
* @param dateUpdated The date that this resource was last updated.
* @return this
*/
public SessionCreator setDateUpdated(final DateTime dateUpdated) {
this.dateUpdated = dateUpdated;
return this;
}
/**
* Identity of the session's creator. If the Session was created through the
* API, the value will be `system`.
*
* @param createdBy Identity of the session's creator.
* @return this
*/
public SessionCreator setCreatedBy(final String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created Session
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public Session create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.MESSAGING.toString(),
"/v1/Sessions",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("Session creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return Session.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (messagingServiceSid != null) {
request.addPostParam("MessagingServiceSid", messagingServiceSid);
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (attributes != null) {
request.addPostParam("Attributes", attributes);
}
if (dateCreated != null) {
request.addPostParam("DateCreated", dateCreated.toString());
}
if (dateUpdated != null) {
request.addPostParam("DateUpdated", dateUpdated.toString());
}
if (createdBy != null) {
request.addPostParam("CreatedBy", createdBy);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy