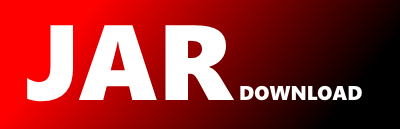
com.twilio.rest.notify.v1.service.Notification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.notify.v1.service;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.common.base.MoreObjects;
import com.twilio.base.Resource;
import com.twilio.converter.Converter;
import com.twilio.converter.DateConverter;
import com.twilio.converter.Promoter;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
import org.joda.time.DateTime;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* PLEASE NOTE that this class contains beta products that are subject to
* change. Use them with caution.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class Notification extends Resource {
private static final long serialVersionUID = 275926337068118L;
public enum Priority {
HIGH("high"),
LOW("low");
private final String value;
private Priority(final String value) {
this.value = value;
}
public String toString() {
return value;
}
/**
* Generate a Priority from a string.
* @param value string value
* @return generated Priority
*/
@JsonCreator
public static Priority forValue(final String value) {
return Promoter.enumFromString(value, Priority.values());
}
}
/**
* Create a NotificationCreator to execute create.
*
* @param pathServiceSid The SID of the Service to create the resource under
* @return NotificationCreator capable of executing the create
*/
public static NotificationCreator creator(final String pathServiceSid) {
return new NotificationCreator(pathServiceSid);
}
/**
* Converts a JSON String into a Notification object using the provided
* ObjectMapper.
*
* @param json Raw JSON String
* @param objectMapper Jackson ObjectMapper
* @return Notification object represented by the provided JSON
*/
public static Notification fromJson(final String json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Notification.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
/**
* Converts a JSON InputStream into a Notification object using the provided
* ObjectMapper.
*
* @param json Raw JSON InputStream
* @param objectMapper Jackson ObjectMapper
* @return Notification object represented by the provided JSON
*/
public static Notification fromJson(final InputStream json, final ObjectMapper objectMapper) {
// Convert all checked exceptions to Runtime
try {
return objectMapper.readValue(json, Notification.class);
} catch (final JsonMappingException | JsonParseException e) {
throw new ApiException(e.getMessage(), e);
} catch (final IOException e) {
throw new ApiConnectionException(e.getMessage(), e);
}
}
private final String sid;
private final String accountSid;
private final String serviceSid;
private final DateTime dateCreated;
private final List identities;
private final List tags;
private final List segments;
private final Notification.Priority priority;
private final Integer ttl;
private final String title;
private final String body;
private final String sound;
private final String action;
private final Map data;
private final Map apn;
private final Map gcm;
private final Map fcm;
private final Map sms;
private final Map facebookMessenger;
private final Map alexa;
@JsonCreator
private Notification(@JsonProperty("sid")
final String sid,
@JsonProperty("account_sid")
final String accountSid,
@JsonProperty("service_sid")
final String serviceSid,
@JsonProperty("date_created")
final String dateCreated,
@JsonProperty("identities")
final List identities,
@JsonProperty("tags")
final List tags,
@JsonProperty("segments")
final List segments,
@JsonProperty("priority")
final Notification.Priority priority,
@JsonProperty("ttl")
final Integer ttl,
@JsonProperty("title")
final String title,
@JsonProperty("body")
final String body,
@JsonProperty("sound")
final String sound,
@JsonProperty("action")
final String action,
@JsonProperty("data")
final Map data,
@JsonProperty("apn")
final Map apn,
@JsonProperty("gcm")
final Map gcm,
@JsonProperty("fcm")
final Map fcm,
@JsonProperty("sms")
final Map sms,
@JsonProperty("facebook_messenger")
final Map facebookMessenger,
@JsonProperty("alexa")
final Map alexa) {
this.sid = sid;
this.accountSid = accountSid;
this.serviceSid = serviceSid;
this.dateCreated = DateConverter.iso8601DateTimeFromString(dateCreated);
this.identities = identities;
this.tags = tags;
this.segments = segments;
this.priority = priority;
this.ttl = ttl;
this.title = title;
this.body = body;
this.sound = sound;
this.action = action;
this.data = data;
this.apn = apn;
this.gcm = gcm;
this.fcm = fcm;
this.sms = sms;
this.facebookMessenger = facebookMessenger;
this.alexa = alexa;
}
/**
* Returns The The unique string that identifies the resource.
*
* @return The unique string that identifies the resource
*/
public final String getSid() {
return this.sid;
}
/**
* Returns The The SID of the Account that created the resource.
*
* @return The SID of the Account that created the resource
*/
public final String getAccountSid() {
return this.accountSid;
}
/**
* Returns The The SID of the Service that the resource is associated with.
*
* @return The SID of the Service that the resource is associated with
*/
public final String getServiceSid() {
return this.serviceSid;
}
/**
* Returns The The RFC 2822 date and time in GMT when the resource was created.
*
* @return The RFC 2822 date and time in GMT when the resource was created
*/
public final DateTime getDateCreated() {
return this.dateCreated;
}
/**
* Returns The The list of identity values of the Users to notify.
*
* @return The list of identity values of the Users to notify
*/
public final List getIdentities() {
return this.identities;
}
/**
* Returns The The tags that select the Bindings to notify.
*
* @return The tags that select the Bindings to notify
*/
public final List getTags() {
return this.tags;
}
/**
* Returns The The list of Segments to notify.
*
* @return The list of Segments to notify
*/
public final List getSegments() {
return this.segments;
}
/**
* Returns The The priority of the notification.
*
* @return The priority of the notification
*/
public final Notification.Priority getPriority() {
return this.priority;
}
/**
* Returns The How long, in seconds, the notification is valid.
*
* @return How long, in seconds, the notification is valid
*/
public final Integer getTtl() {
return this.ttl;
}
/**
* Returns The The notification title.
*
* @return The notification title
*/
public final String getTitle() {
return this.title;
}
/**
* Returns The The notification body text.
*
* @return The notification body text
*/
public final String getBody() {
return this.body;
}
/**
* Returns The The name of the sound to be played for the notification.
*
* @return The name of the sound to be played for the notification
*/
public final String getSound() {
return this.sound;
}
/**
* Returns The The actions to display for the notification.
*
* @return The actions to display for the notification
*/
public final String getAction() {
return this.action;
}
/**
* Returns The The custom key-value pairs of the notification's payload.
*
* @return The custom key-value pairs of the notification's payload
*/
public final Map getData() {
return this.data;
}
/**
* Returns The The APNS-specific payload that overrides corresponding attributes
* in a generic payload for APNS Bindings.
*
* @return The APNS-specific payload that overrides corresponding attributes in
* a generic payload for APNS Bindings
*/
public final Map getApn() {
return this.apn;
}
/**
* Returns The The GCM-specific payload that overrides corresponding attributes
* in generic payload for GCM Bindings.
*
* @return The GCM-specific payload that overrides corresponding attributes in
* generic payload for GCM Bindings
*/
public final Map getGcm() {
return this.gcm;
}
/**
* Returns The The FCM-specific payload that overrides corresponding attributes
* in generic payload for FCM Bindings.
*
* @return The FCM-specific payload that overrides corresponding attributes in
* generic payload for FCM Bindings
*/
public final Map getFcm() {
return this.fcm;
}
/**
* Returns The The SMS-specific payload that overrides corresponding attributes
* in generic payload for SMS Bindings.
*
* @return The SMS-specific payload that overrides corresponding attributes in
* generic payload for SMS Bindings
*/
public final Map getSms() {
return this.sms;
}
/**
* Returns The Deprecated.
*
* @return Deprecated
*/
public final Map getFacebookMessenger() {
return this.facebookMessenger;
}
/**
* Returns The Deprecated.
*
* @return Deprecated
*/
public final Map getAlexa() {
return this.alexa;
}
@Override
public boolean equals(final Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Notification other = (Notification) o;
return Objects.equals(sid, other.sid) &&
Objects.equals(accountSid, other.accountSid) &&
Objects.equals(serviceSid, other.serviceSid) &&
Objects.equals(dateCreated, other.dateCreated) &&
Objects.equals(identities, other.identities) &&
Objects.equals(tags, other.tags) &&
Objects.equals(segments, other.segments) &&
Objects.equals(priority, other.priority) &&
Objects.equals(ttl, other.ttl) &&
Objects.equals(title, other.title) &&
Objects.equals(body, other.body) &&
Objects.equals(sound, other.sound) &&
Objects.equals(action, other.action) &&
Objects.equals(data, other.data) &&
Objects.equals(apn, other.apn) &&
Objects.equals(gcm, other.gcm) &&
Objects.equals(fcm, other.fcm) &&
Objects.equals(sms, other.sms) &&
Objects.equals(facebookMessenger, other.facebookMessenger) &&
Objects.equals(alexa, other.alexa);
}
@Override
public int hashCode() {
return Objects.hash(sid,
accountSid,
serviceSid,
dateCreated,
identities,
tags,
segments,
priority,
ttl,
title,
body,
sound,
action,
data,
apn,
gcm,
fcm,
sms,
facebookMessenger,
alexa);
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("sid", sid)
.add("accountSid", accountSid)
.add("serviceSid", serviceSid)
.add("dateCreated", dateCreated)
.add("identities", identities)
.add("tags", tags)
.add("segments", segments)
.add("priority", priority)
.add("ttl", ttl)
.add("title", title)
.add("body", body)
.add("sound", sound)
.add("action", action)
.add("data", data)
.add("apn", apn)
.add("gcm", gcm)
.add("fcm", fcm)
.add("sms", sms)
.add("facebookMessenger", facebookMessenger)
.add("alexa", alexa)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy