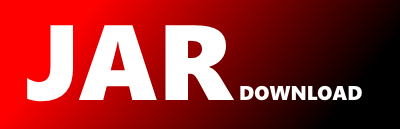
com.twilio.rest.accounts.v1.credential.PublicKeyCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of twilio Show documentation
Show all versions of twilio Show documentation
Twilio Java Helper Library
/**
* This code was generated by
* \ / _ _ _| _ _
* | (_)\/(_)(_|\/| |(/_ v1.0.0
* / /
*/
package com.twilio.rest.accounts.v1.credential;
import com.twilio.base.Creator;
import com.twilio.exception.ApiConnectionException;
import com.twilio.exception.ApiException;
import com.twilio.exception.RestException;
import com.twilio.http.HttpMethod;
import com.twilio.http.Request;
import com.twilio.http.Response;
import com.twilio.http.TwilioRestClient;
import com.twilio.rest.Domains;
public class PublicKeyCreator extends Creator {
private final String publicKey;
private String friendlyName;
private String accountSid;
/**
* Construct a new PublicKeyCreator.
*
* @param publicKey A URL encoded representation of the public key
*/
public PublicKeyCreator(final String publicKey) {
this.publicKey = publicKey;
}
/**
* A descriptive string that you create to describe the resource. It can be up
* to 64 characters long..
*
* @param friendlyName A string to describe the resource
* @return this
*/
public PublicKeyCreator setFriendlyName(final String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* The SID of the Subaccount that this Credential should be associated with.
* Must be a valid Subaccount of the account issuing the request.
*
* @param accountSid The Subaccount this Credential should be associated with.
* @return this
*/
public PublicKeyCreator setAccountSid(final String accountSid) {
this.accountSid = accountSid;
return this;
}
/**
* Make the request to the Twilio API to perform the create.
*
* @param client TwilioRestClient with which to make the request
* @return Created PublicKey
*/
@Override
@SuppressWarnings("checkstyle:linelength")
public PublicKey create(final TwilioRestClient client) {
Request request = new Request(
HttpMethod.POST,
Domains.ACCOUNTS.toString(),
"/v1/Credentials/PublicKeys",
client.getRegion()
);
addPostParams(request);
Response response = client.request(request);
if (response == null) {
throw new ApiConnectionException("PublicKey creation failed: Unable to connect to server");
} else if (!TwilioRestClient.SUCCESS.apply(response.getStatusCode())) {
RestException restException = RestException.fromJson(response.getStream(), client.getObjectMapper());
if (restException == null) {
throw new ApiException("Server Error, no content");
}
throw new ApiException(
restException.getMessage(),
restException.getCode(),
restException.getMoreInfo(),
restException.getStatus(),
null
);
}
return PublicKey.fromJson(response.getStream(), client.getObjectMapper());
}
/**
* Add the requested post parameters to the Request.
*
* @param request Request to add post params to
*/
private void addPostParams(final Request request) {
if (publicKey != null) {
request.addPostParam("PublicKey", publicKey.toString());
}
if (friendlyName != null) {
request.addPostParam("FriendlyName", friendlyName);
}
if (accountSid != null) {
request.addPostParam("AccountSid", accountSid);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy